mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 01:55:07 +00:00
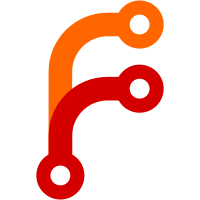
This allows us to use our nice syscall wrappers, avoids some accidental string copying, and starts to unlink us from the old DeprecatedString.
59 lines
1.2 KiB
C++
59 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2020-2021, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include "TempFile.h"
|
|
#include <AK/Random.h>
|
|
#include <LibCore/File.h>
|
|
#include <fcntl.h>
|
|
#include <stdlib.h>
|
|
#include <sys/stat.h>
|
|
|
|
namespace Core {
|
|
|
|
NonnullOwnPtr<TempFile> TempFile::create(Type type)
|
|
{
|
|
return adopt_own(*new TempFile(type));
|
|
}
|
|
|
|
DeprecatedString TempFile::create_temp(Type type)
|
|
{
|
|
char name_template[] = "/tmp/tmp.XXXXXX";
|
|
switch (type) {
|
|
case Type::File: {
|
|
auto fd = mkstemp(name_template);
|
|
VERIFY(fd >= 0);
|
|
close(fd);
|
|
break;
|
|
}
|
|
case Type::Directory: {
|
|
auto fd = mkdtemp(name_template);
|
|
VERIFY(fd != nullptr);
|
|
break;
|
|
}
|
|
}
|
|
return DeprecatedString { name_template };
|
|
}
|
|
|
|
TempFile::TempFile(Type type)
|
|
: m_type(type)
|
|
, m_path(create_temp(type))
|
|
{
|
|
}
|
|
|
|
TempFile::~TempFile()
|
|
{
|
|
File::RecursionMode recursion_allowed { File::RecursionMode::Disallowed };
|
|
if (m_type == Type::Directory)
|
|
recursion_allowed = File::RecursionMode::Allowed;
|
|
|
|
auto rc = File::remove(m_path, recursion_allowed);
|
|
if (rc.is_error()) {
|
|
warnln("File::remove failed: {}", rc.error().string_literal());
|
|
}
|
|
}
|
|
|
|
}
|