mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 01:55:07 +00:00
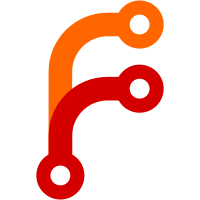
We have a new, improved string type coming up in AK (OOM aware, no null state), and while it's going to use UTF-8, the name UTF8String is a mouthful - so let's free up the String name by renaming the existing class. Making the old one have an annoying name will hopefully also help with quick adoption :^)
53 lines
1.2 KiB
C++
53 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2018-2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/DeprecatedString.h>
|
|
#include <AK/ScopeGuard.h>
|
|
#include <LibCore/MappedFile.h>
|
|
#include <LibCore/System.h>
|
|
#include <fcntl.h>
|
|
#include <sys/mman.h>
|
|
#include <unistd.h>
|
|
|
|
namespace Core {
|
|
|
|
ErrorOr<NonnullRefPtr<MappedFile>> MappedFile::map(StringView path)
|
|
{
|
|
auto fd = TRY(Core::System::open(path, O_RDONLY | O_CLOEXEC, 0));
|
|
return map_from_fd_and_close(fd, path);
|
|
}
|
|
|
|
ErrorOr<NonnullRefPtr<MappedFile>> MappedFile::map_from_fd_and_close(int fd, [[maybe_unused]] StringView path)
|
|
{
|
|
TRY(Core::System::fcntl(fd, F_SETFD, FD_CLOEXEC));
|
|
|
|
ScopeGuard fd_close_guard = [fd] {
|
|
close(fd);
|
|
};
|
|
|
|
auto stat = TRY(Core::System::fstat(fd));
|
|
auto size = stat.st_size;
|
|
|
|
auto* ptr = TRY(Core::System::mmap(nullptr, size, PROT_READ, MAP_SHARED, fd, 0, 0, path));
|
|
|
|
return adopt_ref(*new MappedFile(ptr, size));
|
|
}
|
|
|
|
MappedFile::MappedFile(void* ptr, size_t size)
|
|
: m_data(ptr)
|
|
, m_size(size)
|
|
{
|
|
}
|
|
|
|
MappedFile::~MappedFile()
|
|
{
|
|
auto res = Core::System::munmap(m_data, m_size);
|
|
if (res.is_error())
|
|
dbgln("Failed to unmap MappedFile (@ {:p}): {}", m_data, res.error());
|
|
}
|
|
|
|
}
|