mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 01:55:07 +00:00
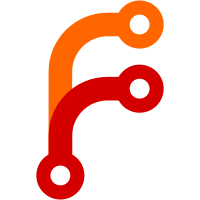
These instances were detected by searching for files that include stdlib.h, but don't match the regex: \\b(_abort|abort|abs|aligned_alloc|arc4random|arc4random_buf|arc4random_ uniform|atexit|atof|atoi|atol|atoll|bsearch|calloc|clearenv|div|div_t|ex it|_Exit|EXIT_FAILURE|EXIT_SUCCESS|free|getenv|getprogname|grantpt|labs| ldiv|ldiv_t|llabs|lldiv|lldiv_t|malloc|malloc_good_size|malloc_size|mble n|mbstowcs|mbtowc|mkdtemp|mkstemp|mkstemps|mktemp|posix_memalign|posix_o penpt|ptsname|ptsname_r|putenv|qsort|qsort_r|rand|RAND_MAX|random|reallo c|realpath|secure_getenv|serenity_dump_malloc_stats|serenity_setenv|sete nv|setprogname|srand|srandom|strtod|strtof|strtol|strtold|strtoll|strtou l|strtoull|system|unlockpt|unsetenv|wcstombs|wctomb)\\b (Without the linebreaks.) This regex is pessimistic, so there might be more files that don't actually use anything from the stdlib. In theory, one might use LibCPP to detect things like this automatically, but let's do this one step after another.
46 lines
1.1 KiB
C++
46 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2020, Peter Elliott <pelliott@serenityos.org>
|
|
* Copyright (c) 2021, Emanuele Torre <torreemanuele6@gmail.com>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <LibCore/GetPassword.h>
|
|
#include <LibCore/System.h>
|
|
#include <stdio.h>
|
|
#include <termios.h>
|
|
#include <unistd.h>
|
|
|
|
namespace Core {
|
|
|
|
ErrorOr<SecretString> get_password(StringView prompt)
|
|
{
|
|
TRY(Core::System::write(STDOUT_FILENO, prompt.bytes()));
|
|
|
|
auto original = TRY(Core::System::tcgetattr(STDIN_FILENO));
|
|
|
|
termios no_echo = original;
|
|
no_echo.c_lflag &= ~ECHO;
|
|
TRY(Core::System::tcsetattr(STDIN_FILENO, TCSAFLUSH, no_echo));
|
|
|
|
char* password = nullptr;
|
|
size_t n = 0;
|
|
|
|
auto line_length = getline(&password, &n, stdin);
|
|
auto saved_errno = errno;
|
|
|
|
tcsetattr(STDIN_FILENO, TCSAFLUSH, &original);
|
|
putchar('\n');
|
|
|
|
if (line_length < 0)
|
|
return Error::from_errno(saved_errno);
|
|
|
|
VERIFY(line_length != 0);
|
|
|
|
// Remove trailing '\n' read by getline().
|
|
password[line_length - 1] = '\0';
|
|
|
|
return SecretString::take_ownership(password, line_length);
|
|
}
|
|
}
|