mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 01:55:07 +00:00
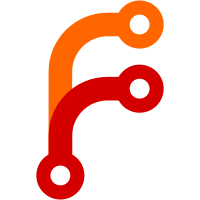
This removes the direct dependency on sys/time.h from ElapsedTimer, and makes the code a lot cleaner by using the helpers from AK::Time for time math and getting the current timestamp.
45 lines
806 B
C++
45 lines
806 B
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/Assertions.h>
|
|
#include <AK/Time.h>
|
|
#include <LibCore/ElapsedTimer.h>
|
|
|
|
namespace Core {
|
|
|
|
ElapsedTimer ElapsedTimer::start_new()
|
|
{
|
|
ElapsedTimer timer;
|
|
timer.start();
|
|
return timer;
|
|
}
|
|
|
|
void ElapsedTimer::start()
|
|
{
|
|
m_valid = true;
|
|
m_origin_time = m_precise ? Time::now_monotonic() : Time::now_monotonic_coarse();
|
|
}
|
|
|
|
void ElapsedTimer::reset()
|
|
{
|
|
m_valid = false;
|
|
m_origin_time = {};
|
|
}
|
|
|
|
i64 ElapsedTimer::elapsed() const
|
|
{
|
|
return elapsed_time().to_milliseconds();
|
|
}
|
|
|
|
Time ElapsedTimer::elapsed_time() const
|
|
{
|
|
VERIFY(is_valid());
|
|
auto now = m_precise ? Time::now_monotonic() : Time::now_monotonic_coarse();
|
|
return now - m_origin_time;
|
|
}
|
|
|
|
}
|