mirror of
https://github.com/SerenityOS/serenity
synced 2024-10-07 16:40:59 +00:00
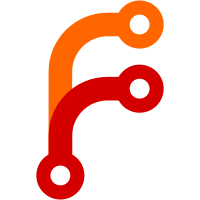
These types can be picked up by including <AK/Types.h>: * u8, u16, u32, u64 (unsigned) * i8, i16, i32, i64 (signed)
32 lines
866 B
C++
32 lines
866 B
C++
#pragma once
|
|
|
|
#include <AK/NetworkOrdered.h>
|
|
#include <Kernel/Net/MACAddress.h>
|
|
|
|
class [[gnu::packed]] EthernetFrameHeader
|
|
{
|
|
public:
|
|
EthernetFrameHeader() {}
|
|
~EthernetFrameHeader() {}
|
|
|
|
MACAddress destination() const { return m_destination; }
|
|
void set_destination(const MACAddress& address) { m_destination = address; }
|
|
|
|
MACAddress source() const { return m_source; }
|
|
void set_source(const MACAddress& address) { m_source = address; }
|
|
|
|
u16 ether_type() const { return m_ether_type; }
|
|
void set_ether_type(u16 ether_type) { m_ether_type = ether_type; }
|
|
|
|
const void* payload() const { return &m_payload[0]; }
|
|
void* payload() { return &m_payload[0]; }
|
|
|
|
private:
|
|
MACAddress m_destination;
|
|
MACAddress m_source;
|
|
NetworkOrdered<u16> m_ether_type;
|
|
u32 m_payload[0];
|
|
};
|
|
|
|
static_assert(sizeof(EthernetFrameHeader) == 14);
|