mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 10:36:24 +00:00
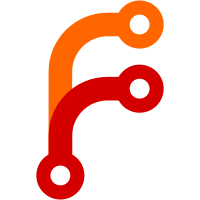
Also run it across the whole tree to get everything using the One True Style. We don't yet run this in an automated fashion as it's a little slow, but there is a snippet to do so in makeall.sh.
54 lines
794 B
C++
54 lines
794 B
C++
#pragma once
|
|
|
|
#include "Assertions.h"
|
|
#include "RetainPtr.h"
|
|
#include "Retainable.h"
|
|
|
|
namespace AK {
|
|
|
|
template<typename T>
|
|
class Weakable;
|
|
template<typename T>
|
|
class WeakPtr;
|
|
|
|
template<typename T>
|
|
class WeakLink : public Retainable<WeakLink<T>> {
|
|
friend class Weakable<T>;
|
|
|
|
public:
|
|
T* ptr() { return static_cast<T*>(m_ptr); }
|
|
const T* ptr() const { return static_cast<const T*>(m_ptr); }
|
|
|
|
private:
|
|
explicit WeakLink(T& weakable)
|
|
: m_ptr(&weakable)
|
|
{
|
|
}
|
|
T* m_ptr;
|
|
};
|
|
|
|
template<typename T>
|
|
class Weakable {
|
|
private:
|
|
class Link;
|
|
|
|
public:
|
|
WeakPtr<T> make_weak_ptr();
|
|
|
|
protected:
|
|
Weakable() {}
|
|
|
|
~Weakable()
|
|
{
|
|
if (m_link)
|
|
m_link->m_ptr = nullptr;
|
|
}
|
|
|
|
private:
|
|
RetainPtr<WeakLink<T>> m_link;
|
|
};
|
|
|
|
}
|
|
|
|
using AK::Weakable;
|