mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-22 18:46:18 +00:00
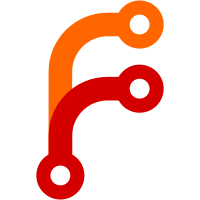
Previously we would not run destructors for items in a CircularQueue, which would lead to memory leaks. This patch fixes that, and also adds a basic unit test for the class.
26 lines
490 B
C++
26 lines
490 B
C++
#pragma once
|
|
|
|
#include <AK/Assertions.h>
|
|
#include <AK/CircularQueue.h>
|
|
#include <AK/Types.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename T, int Capacity>
|
|
class CircularDeque : public CircularQueue<T, Capacity> {
|
|
public:
|
|
T dequeue_end()
|
|
{
|
|
ASSERT(!this->is_empty());
|
|
auto& slot = this->elements()[(this->m_head + this->m_size - 1) % Capacity];
|
|
T value = move(slot);
|
|
slot.~T();
|
|
this->m_size--;
|
|
return value;
|
|
}
|
|
};
|
|
|
|
}
|
|
|
|
using AK::CircularDeque;
|