mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 10:05:32 +00:00
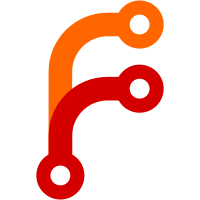
SPDX License Identifiers are a more compact / standardized way of representing file license information. See: https://spdx.dev/resources/use/#identifiers This was done with the `ambr` search and replace tool. ambr --no-parent-ignore --key-from-file --rep-from-file key.txt rep.txt *
50 lines
1.2 KiB
C++
50 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2020-2021, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Assertions.h>
|
|
#include <AK/Platform.h>
|
|
#include <AK/StdLibExtras.h>
|
|
|
|
namespace AK {
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE bool is(InputType& input)
|
|
{
|
|
if constexpr (requires { input.template fast_is<OutputType>(); }) {
|
|
return input.template fast_is<OutputType>();
|
|
}
|
|
return dynamic_cast<CopyConst<InputType, OutputType>*>(&input);
|
|
}
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE bool is(InputType* input)
|
|
{
|
|
return input && is<OutputType>(*input);
|
|
}
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE CopyConst<InputType, OutputType>* downcast(InputType* input)
|
|
{
|
|
static_assert(IsBaseOf<InputType, OutputType>);
|
|
VERIFY(!input || is<OutputType>(*input));
|
|
return static_cast<CopyConst<InputType, OutputType>*>(input);
|
|
}
|
|
|
|
template<typename OutputType, typename InputType>
|
|
ALWAYS_INLINE CopyConst<InputType, OutputType>& downcast(InputType& input)
|
|
{
|
|
static_assert(IsBaseOf<InputType, OutputType>);
|
|
VERIFY(is<OutputType>(input));
|
|
return static_cast<CopyConst<InputType, OutputType>&>(input);
|
|
}
|
|
|
|
}
|
|
|
|
using AK::downcast;
|
|
using AK::is;
|