mirror of
https://github.com/SerenityOS/serenity
synced 2024-07-21 10:05:32 +00:00
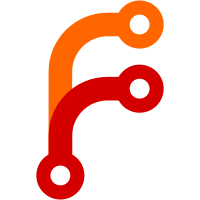
By constraining two implementations, the compiler will select the best fitting one. All this will require is duplicating the implementation and simplifying for the `void` case. This constraining also informs both the caller and compiler by passing the callback parameter types as part of the constraint (e.g.: `IterationFunction<int>`). Some `for_each` functions in LibELF only take functions which return `void`. This is a minimal correctness check, as it removes one way for a function to incompletely do something. There seems to be a possible idiom where inside a lambda, a `return;` is the same as `continue;` in a for-loop.
67 lines
1.2 KiB
C++
67 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2020, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/IterationDecision.h>
|
|
#include <AK/StdLibExtras.h>
|
|
|
|
namespace AK::Concepts {
|
|
|
|
#if defined(__cpp_concepts) && !defined(__COVERITY__)
|
|
|
|
template<typename T>
|
|
concept Integral = IsIntegral<T>;
|
|
|
|
template<typename T>
|
|
concept FloatingPoint = IsFloatingPoint<T>;
|
|
|
|
template<typename T>
|
|
concept Arithmetic = IsArithmetic<T>;
|
|
|
|
template<typename T>
|
|
concept Signed = IsSigned<T>;
|
|
|
|
template<typename T>
|
|
concept Unsigned = IsUnsigned<T>;
|
|
|
|
template<typename T, typename U>
|
|
concept SameAs = IsSame<T, U>;
|
|
|
|
template<typename Func, typename... Args>
|
|
concept VoidFunction = requires(Func func, Args... args)
|
|
{
|
|
{
|
|
func(args...)
|
|
}
|
|
->SameAs<void>;
|
|
};
|
|
|
|
template<typename Func, typename... Args>
|
|
concept IteratorFunction = requires(Func func, Args... args)
|
|
{
|
|
{
|
|
func(args...)
|
|
}
|
|
->SameAs<IterationDecision>;
|
|
};
|
|
|
|
#endif
|
|
|
|
}
|
|
|
|
#if defined(__cpp_concepts) && !defined(__COVERITY__)
|
|
|
|
using AK::Concepts::Arithmetic;
|
|
using AK::Concepts::FloatingPoint;
|
|
using AK::Concepts::Integral;
|
|
using AK::Concepts::IteratorFunction;
|
|
using AK::Concepts::Signed;
|
|
using AK::Concepts::Unsigned;
|
|
using AK::Concepts::VoidFunction;
|
|
|
|
#endif
|