mirror of
https://github.com/SerenityOS/serenity
synced 2024-09-28 20:24:32 +00:00
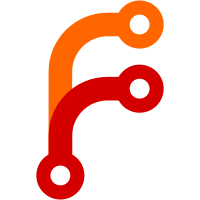
InodeVMObjects now track dirty and clean pages. This tracking of dirty and clean pages is used by the msync and purge syscalls. dirty page tracking works using the following rules: * when a new InodeVMObject is made, all pages are marked clean. * writes to clean InodeVMObject pages will cause a page fault, the fault handler will mark the page as dirty. * writes to dirty InodeVMObject pages do not cause page faults. * if msync is called, only dirty pages are flushed to storage (and marked clean). * if purge syscall is called, only clean pages are discarded.
48 lines
1.2 KiB
C++
48 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/Bitmap.h>
|
|
#include <Kernel/Memory/VMObject.h>
|
|
#include <Kernel/UnixTypes.h>
|
|
|
|
namespace Kernel::Memory {
|
|
|
|
class InodeVMObject : public VMObject {
|
|
public:
|
|
virtual ~InodeVMObject() override;
|
|
|
|
Inode& inode() { return *m_inode; }
|
|
Inode const& inode() const { return *m_inode; }
|
|
|
|
size_t amount_dirty() const;
|
|
size_t amount_clean() const;
|
|
|
|
bool is_page_dirty(size_t page_index) const;
|
|
void set_page_dirty(size_t page_index, bool is_dirty);
|
|
|
|
int release_all_clean_pages();
|
|
int try_release_clean_pages(int page_amount);
|
|
|
|
u32 writable_mappings() const;
|
|
|
|
protected:
|
|
explicit InodeVMObject(Inode&, FixedArray<RefPtr<PhysicalRAMPage>>&&, Bitmap dirty_pages);
|
|
explicit InodeVMObject(InodeVMObject const&, FixedArray<RefPtr<PhysicalRAMPage>>&&, Bitmap dirty_pages);
|
|
|
|
InodeVMObject& operator=(InodeVMObject const&) = delete;
|
|
InodeVMObject& operator=(InodeVMObject&&) = delete;
|
|
InodeVMObject(InodeVMObject&&) = delete;
|
|
|
|
virtual bool is_inode() const final { return true; }
|
|
|
|
NonnullRefPtr<Inode> const m_inode;
|
|
Bitmap m_dirty_pages;
|
|
};
|
|
|
|
}
|