mirror of
https://gitlab.com/qemu-project/qemu
synced 2024-07-21 02:15:04 +00:00
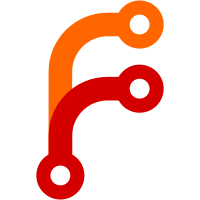
Since QEMU 7.1 we don't support Ubuntu 18.04 anymore, so the last big important Linux distro that did not have a pre-packaged libslirp has been dismissed. All other major distros seem to have a libslirp package in their distribution already - according to repology.org: Fedora 35: 4.6.1 CentOS 8 (RHEL-8): 4.4.0 Debian 11: 4.4.0 OpenSUSE Leap 15.3: 4.3.1 Ubuntu LTS 20.04: 4.1.0 FreeBSD Ports: 4.7.0 NetBSD pkgsrc: 4.7.0 Homebrew: 4.7.0 MSYS2 mingw: 4.7.0 The only one that was still missing a libslirp package is OpenBSD - but the next version (OpenBSD 7.2 which will be shipped in October) is going to include a libslirp package. Since QEMU 7.2 will be published after OpenBSD 7.2, we should be fine there, too. So there is no real urgent need for keeping the slirp submodule in the QEMU tree anymore. Thus let's drop the slirp submodule now and rely on the libslirp packages from the distributions instead. Message-Id: <20220824151122.704946-7-thuth@redhat.com> Acked-by: Samuel Thibault <samuel.thibault@ens-lyon.org> Signed-off-by: Thomas Huth <thuth@redhat.com>
74 lines
2.1 KiB
Bash
Executable file
74 lines
2.1 KiB
Bash
Executable file
#!/bin/bash
|
|
#
|
|
# Author: Fam Zheng <famz@redhat.com>
|
|
#
|
|
# Archive source tree, including submodules. This is created for test code to
|
|
# export the source files, in order to be built in a different environment,
|
|
# such as in a docker instance or VM.
|
|
#
|
|
# This code is licensed under the GPL version 2 or later. See
|
|
# the COPYING file in the top-level directory.
|
|
|
|
error() {
|
|
printf %s\\n "$*" >&2
|
|
exit 1
|
|
}
|
|
|
|
if test $# -lt 1; then
|
|
error "Usage: $0 <output tarball>"
|
|
fi
|
|
|
|
tar_file=$(realpath "$1")
|
|
sub_tdir=$(mktemp -d "${tar_file%.tar}.sub.XXXXXXXX")
|
|
sub_file="${sub_tdir}/submodule.tar"
|
|
|
|
# We want a predictable list of submodules for builds, that is
|
|
# independent of what the developer currently has initialized
|
|
# in their checkout, because the build environment is completely
|
|
# different to the host OS.
|
|
submodules="dtc meson ui/keycodemapdb"
|
|
submodules="$submodules tests/fp/berkeley-softfloat-3 tests/fp/berkeley-testfloat-3"
|
|
sub_deinit=""
|
|
|
|
function cleanup() {
|
|
local status=$?
|
|
rm -rf "$sub_tdir"
|
|
if test "$sub_deinit" != ""; then
|
|
git submodule deinit $sub_deinit
|
|
fi
|
|
exit $status
|
|
}
|
|
trap "cleanup" 0 1 2 3 15
|
|
|
|
function tree_ish() {
|
|
local retval='HEAD'
|
|
if ! git diff-index --quiet --ignore-submodules=all HEAD -- &>/dev/null
|
|
then
|
|
retval=$(git stash create)
|
|
fi
|
|
echo "$retval"
|
|
}
|
|
|
|
git archive --format tar "$(tree_ish)" > "$tar_file"
|
|
test $? -ne 0 && error "failed to archive qemu"
|
|
for sm in $submodules; do
|
|
status="$(git submodule status "$sm")"
|
|
smhash="${status#[ +-]}"
|
|
smhash="${smhash%% *}"
|
|
case "$status" in
|
|
-*)
|
|
sub_deinit="$sub_deinit $sm"
|
|
git submodule update --init "$sm"
|
|
test $? -ne 0 && error "failed to update submodule $sm"
|
|
;;
|
|
+*)
|
|
echo "WARNING: submodule $sm is out of sync"
|
|
;;
|
|
esac
|
|
(cd $sm; git archive --format tar --prefix "$sm/" $(tree_ish)) > "$sub_file"
|
|
test $? -ne 0 && error "failed to archive submodule $sm ($smhash)"
|
|
tar --concatenate --file "$tar_file" "$sub_file"
|
|
test $? -ne 0 && error "failed append submodule $sm to $tar_file"
|
|
done
|
|
exit 0
|