mirror of
https://gitlab.com/qemu-project/qemu
synced 2024-11-02 22:41:07 +00:00
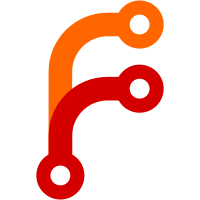
Introduce a new crypto/ directory that will (eventually) contain all the cryptographic related code. This initially defines a wrapper for initializing gnutls and for computing hashes with gnutls. The former ensures that gnutls is guaranteed to be initialized exactly once in QEMU regardless of CLI args. The block quorum code currently fails to initialize gnutls so it only works by luck, if VNC server TLS is not requested. The hash APIs avoids the need to litter the rest of the code with preprocessor checks and simplifies callers by allocating the correct amount of memory for the requested hash. Signed-off-by: Daniel P. Berrange <berrange@redhat.com> Message-Id: <1435770638-25715-2-git-send-email-berrange@redhat.com> Signed-off-by: Paolo Bonzini <pbonzini@redhat.com>
5650 lines
145 KiB
Bash
Executable file
5650 lines
145 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# qemu configure script (c) 2003 Fabrice Bellard
|
|
#
|
|
|
|
# Unset some variables known to interfere with behavior of common tools,
|
|
# just as autoconf does.
|
|
CLICOLOR_FORCE= GREP_OPTIONS=
|
|
unset CLICOLOR_FORCE GREP_OPTIONS
|
|
|
|
# Temporary directory used for files created while
|
|
# configure runs. Since it is in the build directory
|
|
# we can safely blow away any previous version of it
|
|
# (and we need not jump through hoops to try to delete
|
|
# it when configure exits.)
|
|
TMPDIR1="config-temp"
|
|
rm -rf "${TMPDIR1}"
|
|
mkdir -p "${TMPDIR1}"
|
|
if [ $? -ne 0 ]; then
|
|
echo "ERROR: failed to create temporary directory"
|
|
exit 1
|
|
fi
|
|
|
|
TMPB="qemu-conf"
|
|
TMPC="${TMPDIR1}/${TMPB}.c"
|
|
TMPO="${TMPDIR1}/${TMPB}.o"
|
|
TMPCXX="${TMPDIR1}/${TMPB}.cxx"
|
|
TMPL="${TMPDIR1}/${TMPB}.lo"
|
|
TMPA="${TMPDIR1}/lib${TMPB}.la"
|
|
TMPE="${TMPDIR1}/${TMPB}.exe"
|
|
|
|
rm -f config.log
|
|
|
|
# Print a helpful header at the top of config.log
|
|
echo "# QEMU configure log $(date)" >> config.log
|
|
printf "# Configured with:" >> config.log
|
|
printf " '%s'" "$0" "$@" >> config.log
|
|
echo >> config.log
|
|
echo "#" >> config.log
|
|
|
|
error_exit() {
|
|
echo
|
|
echo "ERROR: $1"
|
|
while test -n "$2"; do
|
|
echo " $2"
|
|
shift
|
|
done
|
|
echo
|
|
exit 1
|
|
}
|
|
|
|
do_compiler() {
|
|
# Run the compiler, capturing its output to the log. First argument
|
|
# is compiler binary to execute.
|
|
local compiler="$1"
|
|
shift
|
|
echo $compiler "$@" >> config.log
|
|
$compiler "$@" >> config.log 2>&1 || return $?
|
|
# Test passed. If this is an --enable-werror build, rerun
|
|
# the test with -Werror and bail out if it fails. This
|
|
# makes warning-generating-errors in configure test code
|
|
# obvious to developers.
|
|
if test "$werror" != "yes"; then
|
|
return 0
|
|
fi
|
|
# Don't bother rerunning the compile if we were already using -Werror
|
|
case "$*" in
|
|
*-Werror*)
|
|
return 0
|
|
;;
|
|
esac
|
|
echo $compiler -Werror "$@" >> config.log
|
|
$compiler -Werror "$@" >> config.log 2>&1 && return $?
|
|
error_exit "configure test passed without -Werror but failed with -Werror." \
|
|
"This is probably a bug in the configure script. The failing command" \
|
|
"will be at the bottom of config.log." \
|
|
"You can run configure with --disable-werror to bypass this check."
|
|
}
|
|
|
|
do_cc() {
|
|
do_compiler "$cc" "$@"
|
|
}
|
|
|
|
do_cxx() {
|
|
do_compiler "$cxx" "$@"
|
|
}
|
|
|
|
update_cxxflags() {
|
|
# Set QEMU_CXXFLAGS from QEMU_CFLAGS by filtering out those
|
|
# options which some versions of GCC's C++ compiler complain about
|
|
# because they only make sense for C programs.
|
|
QEMU_CXXFLAGS=
|
|
for arg in $QEMU_CFLAGS; do
|
|
case $arg in
|
|
-Wstrict-prototypes|-Wmissing-prototypes|-Wnested-externs|\
|
|
-Wold-style-declaration|-Wold-style-definition|-Wredundant-decls)
|
|
;;
|
|
*)
|
|
QEMU_CXXFLAGS=${QEMU_CXXFLAGS:+$QEMU_CXXFLAGS }$arg
|
|
;;
|
|
esac
|
|
done
|
|
}
|
|
|
|
compile_object() {
|
|
local_cflags="$1"
|
|
do_cc $QEMU_CFLAGS $local_cflags -c -o $TMPO $TMPC
|
|
}
|
|
|
|
compile_prog() {
|
|
local_cflags="$1"
|
|
local_ldflags="$2"
|
|
do_cc $QEMU_CFLAGS $local_cflags -o $TMPE $TMPC $LDFLAGS $local_ldflags
|
|
}
|
|
|
|
do_libtool() {
|
|
local mode=$1
|
|
shift
|
|
# Run the compiler, capturing its output to the log.
|
|
echo $libtool $mode --tag=CC $cc "$@" >> config.log
|
|
$libtool $mode --tag=CC $cc "$@" >> config.log 2>&1 || return $?
|
|
# Test passed. If this is an --enable-werror build, rerun
|
|
# the test with -Werror and bail out if it fails. This
|
|
# makes warning-generating-errors in configure test code
|
|
# obvious to developers.
|
|
if test "$werror" != "yes"; then
|
|
return 0
|
|
fi
|
|
# Don't bother rerunning the compile if we were already using -Werror
|
|
case "$*" in
|
|
*-Werror*)
|
|
return 0
|
|
;;
|
|
esac
|
|
echo $libtool $mode --tag=CC $cc -Werror "$@" >> config.log
|
|
$libtool $mode --tag=CC $cc -Werror "$@" >> config.log 2>&1 && return $?
|
|
error_exit "configure test passed without -Werror but failed with -Werror." \
|
|
"This is probably a bug in the configure script. The failing command" \
|
|
"will be at the bottom of config.log." \
|
|
"You can run configure with --disable-werror to bypass this check."
|
|
}
|
|
|
|
libtool_prog() {
|
|
do_libtool --mode=compile $QEMU_CFLAGS -c -fPIE -DPIE -o $TMPO $TMPC || return $?
|
|
do_libtool --mode=link $LDFLAGS -o $TMPA $TMPL -rpath /usr/local/lib
|
|
}
|
|
|
|
# symbolically link $1 to $2. Portable version of "ln -sf".
|
|
symlink() {
|
|
rm -rf "$2"
|
|
mkdir -p "$(dirname "$2")"
|
|
ln -s "$1" "$2"
|
|
}
|
|
|
|
# check whether a command is available to this shell (may be either an
|
|
# executable or a builtin)
|
|
has() {
|
|
type "$1" >/dev/null 2>&1
|
|
}
|
|
|
|
# search for an executable in PATH
|
|
path_of() {
|
|
local_command="$1"
|
|
local_ifs="$IFS"
|
|
local_dir=""
|
|
|
|
# pathname has a dir component?
|
|
if [ "${local_command#*/}" != "$local_command" ]; then
|
|
if [ -x "$local_command" ] && [ ! -d "$local_command" ]; then
|
|
echo "$local_command"
|
|
return 0
|
|
fi
|
|
fi
|
|
if [ -z "$local_command" ]; then
|
|
return 1
|
|
fi
|
|
|
|
IFS=:
|
|
for local_dir in $PATH; do
|
|
if [ -x "$local_dir/$local_command" ] && [ ! -d "$local_dir/$local_command" ]; then
|
|
echo "$local_dir/$local_command"
|
|
IFS="${local_ifs:-$(printf ' \t\n')}"
|
|
return 0
|
|
fi
|
|
done
|
|
# not found
|
|
IFS="${local_ifs:-$(printf ' \t\n')}"
|
|
return 1
|
|
}
|
|
|
|
have_backend () {
|
|
echo "$trace_backends" | grep "$1" >/dev/null
|
|
}
|
|
|
|
# default parameters
|
|
source_path=`dirname "$0"`
|
|
cpu=""
|
|
iasl="iasl"
|
|
interp_prefix="/usr/gnemul/qemu-%M"
|
|
static="no"
|
|
cross_prefix=""
|
|
audio_drv_list=""
|
|
block_drv_rw_whitelist=""
|
|
block_drv_ro_whitelist=""
|
|
host_cc="cc"
|
|
libs_softmmu=""
|
|
libs_tools=""
|
|
audio_pt_int=""
|
|
audio_win_int=""
|
|
cc_i386=i386-pc-linux-gnu-gcc
|
|
libs_qga=""
|
|
debug_info="yes"
|
|
stack_protector=""
|
|
|
|
# Don't accept a target_list environment variable.
|
|
unset target_list
|
|
|
|
# Default value for a variable defining feature "foo".
|
|
# * foo="no" feature will only be used if --enable-foo arg is given
|
|
# * foo="" feature will be searched for, and if found, will be used
|
|
# unless --disable-foo is given
|
|
# * foo="yes" this value will only be set by --enable-foo flag.
|
|
# feature will searched for,
|
|
# if not found, configure exits with error
|
|
#
|
|
# Always add --enable-foo and --disable-foo command line args.
|
|
# Distributions want to ensure that several features are compiled in, and it
|
|
# is impossible without a --enable-foo that exits if a feature is not found.
|
|
|
|
bluez=""
|
|
brlapi=""
|
|
curl=""
|
|
curses=""
|
|
docs=""
|
|
fdt=""
|
|
netmap="no"
|
|
pixman=""
|
|
sdl=""
|
|
sdlabi="1.2"
|
|
virtfs=""
|
|
vnc="yes"
|
|
sparse="no"
|
|
uuid=""
|
|
vde=""
|
|
vnc_tls=""
|
|
vnc_sasl=""
|
|
vnc_jpeg=""
|
|
vnc_png=""
|
|
vnc_ws=""
|
|
xen=""
|
|
xen_ctrl_version=""
|
|
xen_pci_passthrough=""
|
|
linux_aio=""
|
|
cap_ng=""
|
|
attr=""
|
|
libattr=""
|
|
xfs=""
|
|
|
|
vhost_net="no"
|
|
vhost_scsi="no"
|
|
kvm="no"
|
|
rdma=""
|
|
gprof="no"
|
|
debug_tcg="no"
|
|
debug="no"
|
|
strip_opt="yes"
|
|
tcg_interpreter="no"
|
|
bigendian="no"
|
|
mingw32="no"
|
|
gcov="no"
|
|
gcov_tool="gcov"
|
|
EXESUF=""
|
|
DSOSUF=".so"
|
|
LDFLAGS_SHARED="-shared"
|
|
modules="no"
|
|
prefix="/usr/local"
|
|
mandir="\${prefix}/share/man"
|
|
datadir="\${prefix}/share"
|
|
qemu_docdir="\${prefix}/share/doc/qemu"
|
|
bindir="\${prefix}/bin"
|
|
libdir="\${prefix}/lib"
|
|
libexecdir="\${prefix}/libexec"
|
|
includedir="\${prefix}/include"
|
|
sysconfdir="\${prefix}/etc"
|
|
local_statedir="\${prefix}/var"
|
|
confsuffix="/qemu"
|
|
slirp="yes"
|
|
oss_lib=""
|
|
bsd="no"
|
|
linux="no"
|
|
solaris="no"
|
|
profiler="no"
|
|
cocoa="no"
|
|
softmmu="yes"
|
|
linux_user="no"
|
|
bsd_user="no"
|
|
guest_base="yes"
|
|
aix="no"
|
|
blobs="yes"
|
|
pkgversion=""
|
|
pie=""
|
|
zero_malloc=""
|
|
qom_cast_debug="yes"
|
|
trace_backends="nop"
|
|
trace_file="trace"
|
|
spice=""
|
|
rbd=""
|
|
smartcard_nss=""
|
|
libusb=""
|
|
usb_redir=""
|
|
opengl=""
|
|
zlib="yes"
|
|
lzo=""
|
|
snappy=""
|
|
bzip2=""
|
|
guest_agent=""
|
|
guest_agent_with_vss="no"
|
|
guest_agent_msi=""
|
|
vss_win32_sdk=""
|
|
win_sdk="no"
|
|
want_tools="yes"
|
|
libiscsi=""
|
|
libnfs=""
|
|
coroutine=""
|
|
coroutine_pool=""
|
|
seccomp=""
|
|
glusterfs=""
|
|
glusterfs_discard="no"
|
|
glusterfs_zerofill="no"
|
|
archipelago="no"
|
|
gtk=""
|
|
gtkabi=""
|
|
gnutls=""
|
|
gnutls_hash=""
|
|
vte=""
|
|
tpm="yes"
|
|
libssh2=""
|
|
vhdx=""
|
|
quorum=""
|
|
numa=""
|
|
tcmalloc="no"
|
|
|
|
# parse CC options first
|
|
for opt do
|
|
optarg=`expr "x$opt" : 'x[^=]*=\(.*\)'`
|
|
case "$opt" in
|
|
--cross-prefix=*) cross_prefix="$optarg"
|
|
;;
|
|
--cc=*) CC="$optarg"
|
|
;;
|
|
--cxx=*) CXX="$optarg"
|
|
;;
|
|
--source-path=*) source_path="$optarg"
|
|
;;
|
|
--cpu=*) cpu="$optarg"
|
|
;;
|
|
--extra-cflags=*) QEMU_CFLAGS="$QEMU_CFLAGS $optarg"
|
|
EXTRA_CFLAGS="$optarg"
|
|
;;
|
|
--extra-ldflags=*) LDFLAGS="$LDFLAGS $optarg"
|
|
EXTRA_LDFLAGS="$optarg"
|
|
;;
|
|
--enable-debug-info) debug_info="yes"
|
|
;;
|
|
--disable-debug-info) debug_info="no"
|
|
;;
|
|
esac
|
|
done
|
|
# OS specific
|
|
# Using uname is really, really broken. Once we have the right set of checks
|
|
# we can eliminate its usage altogether.
|
|
|
|
# Preferred compiler:
|
|
# ${CC} (if set)
|
|
# ${cross_prefix}gcc (if cross-prefix specified)
|
|
# system compiler
|
|
if test -z "${CC}${cross_prefix}"; then
|
|
cc="$host_cc"
|
|
else
|
|
cc="${CC-${cross_prefix}gcc}"
|
|
fi
|
|
|
|
if test -z "${CXX}${cross_prefix}"; then
|
|
cxx="c++"
|
|
else
|
|
cxx="${CXX-${cross_prefix}g++}"
|
|
fi
|
|
|
|
ar="${AR-${cross_prefix}ar}"
|
|
as="${AS-${cross_prefix}as}"
|
|
cpp="${CPP-$cc -E}"
|
|
objcopy="${OBJCOPY-${cross_prefix}objcopy}"
|
|
ld="${LD-${cross_prefix}ld}"
|
|
libtool="${LIBTOOL-${cross_prefix}libtool}"
|
|
nm="${NM-${cross_prefix}nm}"
|
|
strip="${STRIP-${cross_prefix}strip}"
|
|
windres="${WINDRES-${cross_prefix}windres}"
|
|
pkg_config_exe="${PKG_CONFIG-${cross_prefix}pkg-config}"
|
|
query_pkg_config() {
|
|
"${pkg_config_exe}" ${QEMU_PKG_CONFIG_FLAGS} "$@"
|
|
}
|
|
pkg_config=query_pkg_config
|
|
sdl_config="${SDL_CONFIG-${cross_prefix}sdl-config}"
|
|
sdl2_config="${SDL2_CONFIG-${cross_prefix}sdl2-config}"
|
|
|
|
# If the user hasn't specified ARFLAGS, default to 'rv', just as make does.
|
|
ARFLAGS="${ARFLAGS-rv}"
|
|
|
|
# default flags for all hosts
|
|
QEMU_CFLAGS="-fno-strict-aliasing -fno-common $QEMU_CFLAGS"
|
|
QEMU_CFLAGS="-Wall -Wundef -Wwrite-strings -Wmissing-prototypes $QEMU_CFLAGS"
|
|
QEMU_CFLAGS="-Wstrict-prototypes -Wredundant-decls $QEMU_CFLAGS"
|
|
QEMU_CFLAGS="-D_GNU_SOURCE -D_FILE_OFFSET_BITS=64 -D_LARGEFILE_SOURCE $QEMU_CFLAGS"
|
|
QEMU_INCLUDES="-I. -I\$(SRC_PATH) -I\$(SRC_PATH)/include"
|
|
if test "$debug_info" = "yes"; then
|
|
CFLAGS="-g $CFLAGS"
|
|
LDFLAGS="-g $LDFLAGS"
|
|
fi
|
|
|
|
# make source path absolute
|
|
source_path=`cd "$source_path"; pwd`
|
|
|
|
# running configure in the source tree?
|
|
# we know that's the case if configure is there.
|
|
if test -f "./configure"; then
|
|
pwd_is_source_path="y"
|
|
else
|
|
pwd_is_source_path="n"
|
|
fi
|
|
|
|
check_define() {
|
|
cat > $TMPC <<EOF
|
|
#if !defined($1)
|
|
#error $1 not defined
|
|
#endif
|
|
int main(void) { return 0; }
|
|
EOF
|
|
compile_object
|
|
}
|
|
|
|
check_include() {
|
|
cat > $TMPC <<EOF
|
|
#include <$1>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
compile_object
|
|
}
|
|
|
|
write_c_skeleton() {
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
}
|
|
|
|
if check_define __linux__ ; then
|
|
targetos="Linux"
|
|
elif check_define _WIN32 ; then
|
|
targetos='MINGW32'
|
|
elif check_define __OpenBSD__ ; then
|
|
targetos='OpenBSD'
|
|
elif check_define __sun__ ; then
|
|
targetos='SunOS'
|
|
elif check_define __HAIKU__ ; then
|
|
targetos='Haiku'
|
|
else
|
|
targetos=`uname -s`
|
|
fi
|
|
|
|
# Some host OSes need non-standard checks for which CPU to use.
|
|
# Note that these checks are broken for cross-compilation: if you're
|
|
# cross-compiling to one of these OSes then you'll need to specify
|
|
# the correct CPU with the --cpu option.
|
|
case $targetos in
|
|
Darwin)
|
|
# on Leopard most of the system is 32-bit, so we have to ask the kernel if we can
|
|
# run 64-bit userspace code.
|
|
# If the user didn't specify a CPU explicitly and the kernel says this is
|
|
# 64 bit hw, then assume x86_64. Otherwise fall through to the usual detection code.
|
|
if test -z "$cpu" && test "$(sysctl -n hw.optional.x86_64)" = "1"; then
|
|
cpu="x86_64"
|
|
fi
|
|
;;
|
|
SunOS)
|
|
# `uname -m` returns i86pc even on an x86_64 box, so default based on isainfo
|
|
if test -z "$cpu" && test "$(isainfo -k)" = "amd64"; then
|
|
cpu="x86_64"
|
|
fi
|
|
esac
|
|
|
|
if test ! -z "$cpu" ; then
|
|
# command line argument
|
|
:
|
|
elif check_define __i386__ ; then
|
|
cpu="i386"
|
|
elif check_define __x86_64__ ; then
|
|
if check_define __ILP32__ ; then
|
|
cpu="x32"
|
|
else
|
|
cpu="x86_64"
|
|
fi
|
|
elif check_define __sparc__ ; then
|
|
if check_define __arch64__ ; then
|
|
cpu="sparc64"
|
|
else
|
|
cpu="sparc"
|
|
fi
|
|
elif check_define _ARCH_PPC ; then
|
|
if check_define _ARCH_PPC64 ; then
|
|
cpu="ppc64"
|
|
else
|
|
cpu="ppc"
|
|
fi
|
|
elif check_define __mips__ ; then
|
|
cpu="mips"
|
|
elif check_define __ia64__ ; then
|
|
cpu="ia64"
|
|
elif check_define __s390__ ; then
|
|
if check_define __s390x__ ; then
|
|
cpu="s390x"
|
|
else
|
|
cpu="s390"
|
|
fi
|
|
elif check_define __arm__ ; then
|
|
cpu="arm"
|
|
elif check_define __aarch64__ ; then
|
|
cpu="aarch64"
|
|
elif check_define __hppa__ ; then
|
|
cpu="hppa"
|
|
else
|
|
cpu=`uname -m`
|
|
fi
|
|
|
|
ARCH=
|
|
# Normalise host CPU name and set ARCH.
|
|
# Note that this case should only have supported host CPUs, not guests.
|
|
case "$cpu" in
|
|
ia64|ppc|ppc64|s390|s390x|sparc64|x32)
|
|
cpu="$cpu"
|
|
;;
|
|
i386|i486|i586|i686|i86pc|BePC)
|
|
cpu="i386"
|
|
;;
|
|
x86_64|amd64)
|
|
cpu="x86_64"
|
|
;;
|
|
armv*b|armv*l|arm)
|
|
cpu="arm"
|
|
;;
|
|
aarch64)
|
|
cpu="aarch64"
|
|
;;
|
|
mips*)
|
|
cpu="mips"
|
|
;;
|
|
sparc|sun4[cdmuv])
|
|
cpu="sparc"
|
|
;;
|
|
*)
|
|
# This will result in either an error or falling back to TCI later
|
|
ARCH=unknown
|
|
;;
|
|
esac
|
|
if test -z "$ARCH"; then
|
|
ARCH="$cpu"
|
|
fi
|
|
|
|
# OS specific
|
|
|
|
# host *BSD for user mode
|
|
HOST_VARIANT_DIR=""
|
|
|
|
case $targetos in
|
|
CYGWIN*)
|
|
mingw32="yes"
|
|
QEMU_CFLAGS="-mno-cygwin $QEMU_CFLAGS"
|
|
audio_possible_drivers="sdl"
|
|
audio_drv_list="sdl"
|
|
;;
|
|
MINGW32*)
|
|
mingw32="yes"
|
|
audio_possible_drivers="dsound sdl"
|
|
if check_include dsound.h; then
|
|
audio_drv_list="dsound"
|
|
else
|
|
audio_drv_list=""
|
|
fi
|
|
;;
|
|
GNU/kFreeBSD)
|
|
bsd="yes"
|
|
audio_drv_list="oss"
|
|
audio_possible_drivers="oss sdl pa"
|
|
;;
|
|
FreeBSD)
|
|
bsd="yes"
|
|
make="${MAKE-gmake}"
|
|
audio_drv_list="oss"
|
|
audio_possible_drivers="oss sdl pa"
|
|
# needed for kinfo_getvmmap(3) in libutil.h
|
|
LIBS="-lutil $LIBS"
|
|
netmap="" # enable netmap autodetect
|
|
HOST_VARIANT_DIR="freebsd"
|
|
;;
|
|
DragonFly)
|
|
bsd="yes"
|
|
make="${MAKE-gmake}"
|
|
audio_drv_list="oss"
|
|
audio_possible_drivers="oss sdl pa"
|
|
HOST_VARIANT_DIR="dragonfly"
|
|
;;
|
|
NetBSD)
|
|
bsd="yes"
|
|
make="${MAKE-gmake}"
|
|
audio_drv_list="oss"
|
|
audio_possible_drivers="oss sdl"
|
|
oss_lib="-lossaudio"
|
|
HOST_VARIANT_DIR="netbsd"
|
|
;;
|
|
OpenBSD)
|
|
bsd="yes"
|
|
make="${MAKE-gmake}"
|
|
audio_drv_list="sdl"
|
|
audio_possible_drivers="sdl"
|
|
HOST_VARIANT_DIR="openbsd"
|
|
;;
|
|
Darwin)
|
|
bsd="yes"
|
|
darwin="yes"
|
|
LDFLAGS_SHARED="-bundle -undefined dynamic_lookup"
|
|
if [ "$cpu" = "x86_64" ] ; then
|
|
QEMU_CFLAGS="-arch x86_64 $QEMU_CFLAGS"
|
|
LDFLAGS="-arch x86_64 $LDFLAGS"
|
|
fi
|
|
cocoa="yes"
|
|
audio_drv_list="coreaudio"
|
|
audio_possible_drivers="coreaudio sdl"
|
|
LDFLAGS="-framework CoreFoundation -framework IOKit $LDFLAGS"
|
|
libs_softmmu="-F/System/Library/Frameworks -framework Cocoa -framework IOKit $libs_softmmu"
|
|
# Disable attempts to use ObjectiveC features in os/object.h since they
|
|
# won't work when we're compiling with gcc as a C compiler.
|
|
QEMU_CFLAGS="-DOS_OBJECT_USE_OBJC=0 $QEMU_CFLAGS"
|
|
HOST_VARIANT_DIR="darwin"
|
|
;;
|
|
SunOS)
|
|
solaris="yes"
|
|
make="${MAKE-gmake}"
|
|
install="${INSTALL-ginstall}"
|
|
ld="gld"
|
|
smbd="${SMBD-/usr/sfw/sbin/smbd}"
|
|
needs_libsunmath="no"
|
|
solarisrev=`uname -r | cut -f2 -d.`
|
|
if [ "$cpu" = "i386" -o "$cpu" = "x86_64" ] ; then
|
|
if test "$solarisrev" -le 9 ; then
|
|
if test -f /opt/SUNWspro/prod/lib/libsunmath.so.1; then
|
|
needs_libsunmath="yes"
|
|
QEMU_CFLAGS="-I/opt/SUNWspro/prod/include/cc $QEMU_CFLAGS"
|
|
LDFLAGS="-L/opt/SUNWspro/prod/lib -R/opt/SUNWspro/prod/lib $LDFLAGS"
|
|
LIBS="-lsunmath $LIBS"
|
|
else
|
|
error_exit "QEMU will not link correctly on Solaris 8/X86 or 9/x86 without" \
|
|
"libsunmath from the Sun Studio compilers tools, due to a lack of" \
|
|
"C99 math features in libm.so in Solaris 8/x86 and Solaris 9/x86" \
|
|
"Studio 11 can be downloaded from www.sun.com."
|
|
fi
|
|
fi
|
|
fi
|
|
if test -f /usr/include/sys/soundcard.h ; then
|
|
audio_drv_list="oss"
|
|
fi
|
|
audio_possible_drivers="oss sdl"
|
|
# needed for CMSG_ macros in sys/socket.h
|
|
QEMU_CFLAGS="-D_XOPEN_SOURCE=600 $QEMU_CFLAGS"
|
|
# needed for TIOCWIN* defines in termios.h
|
|
QEMU_CFLAGS="-D__EXTENSIONS__ $QEMU_CFLAGS"
|
|
QEMU_CFLAGS="-std=gnu99 $QEMU_CFLAGS"
|
|
solarisnetlibs="-lsocket -lnsl -lresolv"
|
|
LIBS="$solarisnetlibs $LIBS"
|
|
libs_qga="$solarisnetlibs $libs_qga"
|
|
;;
|
|
AIX)
|
|
aix="yes"
|
|
make="${MAKE-gmake}"
|
|
;;
|
|
Haiku)
|
|
haiku="yes"
|
|
QEMU_CFLAGS="-DB_USE_POSITIVE_POSIX_ERRORS $QEMU_CFLAGS"
|
|
LIBS="-lposix_error_mapper -lnetwork $LIBS"
|
|
;;
|
|
*)
|
|
audio_drv_list="oss"
|
|
audio_possible_drivers="oss alsa sdl pa"
|
|
linux="yes"
|
|
linux_user="yes"
|
|
kvm="yes"
|
|
vhost_net="yes"
|
|
vhost_scsi="yes"
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/linux-headers -I$(pwd)/linux-headers $QEMU_INCLUDES"
|
|
;;
|
|
esac
|
|
|
|
if [ "$bsd" = "yes" ] ; then
|
|
if [ "$darwin" != "yes" ] ; then
|
|
bsd_user="yes"
|
|
fi
|
|
fi
|
|
|
|
: ${make=${MAKE-make}}
|
|
: ${install=${INSTALL-install}}
|
|
: ${python=${PYTHON-python}}
|
|
: ${smbd=${SMBD-/usr/sbin/smbd}}
|
|
|
|
# Default objcc to clang if available, otherwise use CC
|
|
if has clang; then
|
|
objcc=clang
|
|
else
|
|
objcc="$cc"
|
|
fi
|
|
|
|
if test "$mingw32" = "yes" ; then
|
|
EXESUF=".exe"
|
|
DSOSUF=".dll"
|
|
QEMU_CFLAGS="-DWIN32_LEAN_AND_MEAN -DWINVER=0x501 $QEMU_CFLAGS"
|
|
# enable C99/POSIX format strings (needs mingw32-runtime 3.15 or later)
|
|
QEMU_CFLAGS="-D__USE_MINGW_ANSI_STDIO=1 $QEMU_CFLAGS"
|
|
LIBS="-lwinmm -lws2_32 -liphlpapi $LIBS"
|
|
write_c_skeleton;
|
|
if compile_prog "" "-liberty" ; then
|
|
LIBS="-liberty $LIBS"
|
|
fi
|
|
prefix="c:/Program Files/QEMU"
|
|
mandir="\${prefix}"
|
|
datadir="\${prefix}"
|
|
qemu_docdir="\${prefix}"
|
|
bindir="\${prefix}"
|
|
sysconfdir="\${prefix}"
|
|
local_statedir=
|
|
confsuffix=""
|
|
libs_qga="-lws2_32 -lwinmm -lpowrprof $libs_qga"
|
|
fi
|
|
|
|
werror=""
|
|
|
|
for opt do
|
|
optarg=`expr "x$opt" : 'x[^=]*=\(.*\)'`
|
|
case "$opt" in
|
|
--help|-h) show_help=yes
|
|
;;
|
|
--version|-V) exec cat $source_path/VERSION
|
|
;;
|
|
--prefix=*) prefix="$optarg"
|
|
;;
|
|
--interp-prefix=*) interp_prefix="$optarg"
|
|
;;
|
|
--source-path=*)
|
|
;;
|
|
--cross-prefix=*)
|
|
;;
|
|
--cc=*)
|
|
;;
|
|
--host-cc=*) host_cc="$optarg"
|
|
;;
|
|
--cxx=*)
|
|
;;
|
|
--iasl=*) iasl="$optarg"
|
|
;;
|
|
--objcc=*) objcc="$optarg"
|
|
;;
|
|
--make=*) make="$optarg"
|
|
;;
|
|
--install=*) install="$optarg"
|
|
;;
|
|
--python=*) python="$optarg"
|
|
;;
|
|
--gcov=*) gcov_tool="$optarg"
|
|
;;
|
|
--smbd=*) smbd="$optarg"
|
|
;;
|
|
--extra-cflags=*)
|
|
;;
|
|
--extra-ldflags=*)
|
|
;;
|
|
--enable-debug-info)
|
|
;;
|
|
--disable-debug-info)
|
|
;;
|
|
--enable-modules)
|
|
modules="yes"
|
|
;;
|
|
--cpu=*)
|
|
;;
|
|
--target-list=*) target_list="$optarg"
|
|
;;
|
|
--enable-trace-backends=*) trace_backends="$optarg"
|
|
;;
|
|
# XXX: backwards compatibility
|
|
--enable-trace-backend=*) trace_backends="$optarg"
|
|
;;
|
|
--with-trace-file=*) trace_file="$optarg"
|
|
;;
|
|
--enable-gprof) gprof="yes"
|
|
;;
|
|
--enable-gcov) gcov="yes"
|
|
;;
|
|
--static)
|
|
static="yes"
|
|
LDFLAGS="-static $LDFLAGS"
|
|
QEMU_PKG_CONFIG_FLAGS="--static $QEMU_PKG_CONFIG_FLAGS"
|
|
;;
|
|
--mandir=*) mandir="$optarg"
|
|
;;
|
|
--bindir=*) bindir="$optarg"
|
|
;;
|
|
--libdir=*) libdir="$optarg"
|
|
;;
|
|
--libexecdir=*) libexecdir="$optarg"
|
|
;;
|
|
--includedir=*) includedir="$optarg"
|
|
;;
|
|
--datadir=*) datadir="$optarg"
|
|
;;
|
|
--with-confsuffix=*) confsuffix="$optarg"
|
|
;;
|
|
--docdir=*) qemu_docdir="$optarg"
|
|
;;
|
|
--sysconfdir=*) sysconfdir="$optarg"
|
|
;;
|
|
--localstatedir=*) local_statedir="$optarg"
|
|
;;
|
|
--sbindir=*|--sharedstatedir=*|\
|
|
--oldincludedir=*|--datarootdir=*|--infodir=*|--localedir=*|\
|
|
--htmldir=*|--dvidir=*|--pdfdir=*|--psdir=*)
|
|
# These switches are silently ignored, for compatibility with
|
|
# autoconf-generated configure scripts. This allows QEMU's
|
|
# configure to be used by RPM and similar macros that set
|
|
# lots of directory switches by default.
|
|
;;
|
|
--with-system-pixman) pixman="system"
|
|
;;
|
|
--without-system-pixman) pixman="internal"
|
|
;;
|
|
--without-pixman) pixman="none"
|
|
;;
|
|
--disable-sdl) sdl="no"
|
|
;;
|
|
--enable-sdl) sdl="yes"
|
|
;;
|
|
--with-sdlabi=*) sdlabi="$optarg"
|
|
;;
|
|
--disable-qom-cast-debug) qom_cast_debug="no"
|
|
;;
|
|
--enable-qom-cast-debug) qom_cast_debug="yes"
|
|
;;
|
|
--disable-virtfs) virtfs="no"
|
|
;;
|
|
--enable-virtfs) virtfs="yes"
|
|
;;
|
|
--disable-vnc) vnc="no"
|
|
;;
|
|
--enable-vnc) vnc="yes"
|
|
;;
|
|
--oss-lib=*) oss_lib="$optarg"
|
|
;;
|
|
--audio-drv-list=*) audio_drv_list="$optarg"
|
|
;;
|
|
--block-drv-rw-whitelist=*|--block-drv-whitelist=*) block_drv_rw_whitelist=`echo "$optarg" | sed -e 's/,/ /g'`
|
|
;;
|
|
--block-drv-ro-whitelist=*) block_drv_ro_whitelist=`echo "$optarg" | sed -e 's/,/ /g'`
|
|
;;
|
|
--enable-debug-tcg) debug_tcg="yes"
|
|
;;
|
|
--disable-debug-tcg) debug_tcg="no"
|
|
;;
|
|
--enable-debug)
|
|
# Enable debugging options that aren't excessively noisy
|
|
debug_tcg="yes"
|
|
debug="yes"
|
|
strip_opt="no"
|
|
;;
|
|
--enable-sparse) sparse="yes"
|
|
;;
|
|
--disable-sparse) sparse="no"
|
|
;;
|
|
--disable-strip) strip_opt="no"
|
|
;;
|
|
--disable-vnc-tls) vnc_tls="no"
|
|
;;
|
|
--enable-vnc-tls) vnc_tls="yes"
|
|
;;
|
|
--disable-vnc-sasl) vnc_sasl="no"
|
|
;;
|
|
--enable-vnc-sasl) vnc_sasl="yes"
|
|
;;
|
|
--disable-vnc-jpeg) vnc_jpeg="no"
|
|
;;
|
|
--enable-vnc-jpeg) vnc_jpeg="yes"
|
|
;;
|
|
--disable-vnc-png) vnc_png="no"
|
|
;;
|
|
--enable-vnc-png) vnc_png="yes"
|
|
;;
|
|
--disable-vnc-ws) vnc_ws="no"
|
|
;;
|
|
--enable-vnc-ws) vnc_ws="yes"
|
|
;;
|
|
--disable-slirp) slirp="no"
|
|
;;
|
|
--disable-uuid) uuid="no"
|
|
;;
|
|
--enable-uuid) uuid="yes"
|
|
;;
|
|
--disable-vde) vde="no"
|
|
;;
|
|
--enable-vde) vde="yes"
|
|
;;
|
|
--disable-netmap) netmap="no"
|
|
;;
|
|
--enable-netmap) netmap="yes"
|
|
;;
|
|
--disable-xen) xen="no"
|
|
;;
|
|
--enable-xen) xen="yes"
|
|
;;
|
|
--disable-xen-pci-passthrough) xen_pci_passthrough="no"
|
|
;;
|
|
--enable-xen-pci-passthrough) xen_pci_passthrough="yes"
|
|
;;
|
|
--disable-brlapi) brlapi="no"
|
|
;;
|
|
--enable-brlapi) brlapi="yes"
|
|
;;
|
|
--disable-bluez) bluez="no"
|
|
;;
|
|
--enable-bluez) bluez="yes"
|
|
;;
|
|
--disable-kvm) kvm="no"
|
|
;;
|
|
--enable-kvm) kvm="yes"
|
|
;;
|
|
--disable-tcg-interpreter) tcg_interpreter="no"
|
|
;;
|
|
--enable-tcg-interpreter) tcg_interpreter="yes"
|
|
;;
|
|
--disable-cap-ng) cap_ng="no"
|
|
;;
|
|
--enable-cap-ng) cap_ng="yes"
|
|
;;
|
|
--disable-spice) spice="no"
|
|
;;
|
|
--enable-spice) spice="yes"
|
|
;;
|
|
--disable-libiscsi) libiscsi="no"
|
|
;;
|
|
--enable-libiscsi) libiscsi="yes"
|
|
;;
|
|
--disable-libnfs) libnfs="no"
|
|
;;
|
|
--enable-libnfs) libnfs="yes"
|
|
;;
|
|
--enable-profiler) profiler="yes"
|
|
;;
|
|
--disable-cocoa) cocoa="no"
|
|
;;
|
|
--enable-cocoa)
|
|
cocoa="yes" ;
|
|
sdl="no" ;
|
|
audio_drv_list="coreaudio `echo $audio_drv_list | sed s,coreaudio,,g`"
|
|
;;
|
|
--disable-system) softmmu="no"
|
|
;;
|
|
--enable-system) softmmu="yes"
|
|
;;
|
|
--disable-user)
|
|
linux_user="no" ;
|
|
bsd_user="no" ;
|
|
;;
|
|
--enable-user) ;;
|
|
--disable-linux-user) linux_user="no"
|
|
;;
|
|
--enable-linux-user) linux_user="yes"
|
|
;;
|
|
--disable-bsd-user) bsd_user="no"
|
|
;;
|
|
--enable-bsd-user) bsd_user="yes"
|
|
;;
|
|
--enable-guest-base) guest_base="yes"
|
|
;;
|
|
--disable-guest-base) guest_base="no"
|
|
;;
|
|
--enable-pie) pie="yes"
|
|
;;
|
|
--disable-pie) pie="no"
|
|
;;
|
|
--enable-werror) werror="yes"
|
|
;;
|
|
--disable-werror) werror="no"
|
|
;;
|
|
--enable-stack-protector) stack_protector="yes"
|
|
;;
|
|
--disable-stack-protector) stack_protector="no"
|
|
;;
|
|
--disable-curses) curses="no"
|
|
;;
|
|
--enable-curses) curses="yes"
|
|
;;
|
|
--disable-curl) curl="no"
|
|
;;
|
|
--enable-curl) curl="yes"
|
|
;;
|
|
--disable-fdt) fdt="no"
|
|
;;
|
|
--enable-fdt) fdt="yes"
|
|
;;
|
|
--disable-linux-aio) linux_aio="no"
|
|
;;
|
|
--enable-linux-aio) linux_aio="yes"
|
|
;;
|
|
--disable-attr) attr="no"
|
|
;;
|
|
--enable-attr) attr="yes"
|
|
;;
|
|
--disable-blobs) blobs="no"
|
|
;;
|
|
--with-pkgversion=*) pkgversion=" ($optarg)"
|
|
;;
|
|
--with-coroutine=*) coroutine="$optarg"
|
|
;;
|
|
--disable-coroutine-pool) coroutine_pool="no"
|
|
;;
|
|
--enable-coroutine-pool) coroutine_pool="yes"
|
|
;;
|
|
--disable-docs) docs="no"
|
|
;;
|
|
--enable-docs) docs="yes"
|
|
;;
|
|
--disable-vhost-net) vhost_net="no"
|
|
;;
|
|
--enable-vhost-net) vhost_net="yes"
|
|
;;
|
|
--disable-vhost-scsi) vhost_scsi="no"
|
|
;;
|
|
--enable-vhost-scsi) vhost_scsi="yes"
|
|
;;
|
|
--disable-opengl) opengl="no"
|
|
;;
|
|
--enable-opengl) opengl="yes"
|
|
;;
|
|
--disable-rbd) rbd="no"
|
|
;;
|
|
--enable-rbd) rbd="yes"
|
|
;;
|
|
--disable-xfsctl) xfs="no"
|
|
;;
|
|
--enable-xfsctl) xfs="yes"
|
|
;;
|
|
--disable-smartcard-nss) smartcard_nss="no"
|
|
;;
|
|
--enable-smartcard-nss) smartcard_nss="yes"
|
|
;;
|
|
--disable-libusb) libusb="no"
|
|
;;
|
|
--enable-libusb) libusb="yes"
|
|
;;
|
|
--disable-usb-redir) usb_redir="no"
|
|
;;
|
|
--enable-usb-redir) usb_redir="yes"
|
|
;;
|
|
--disable-zlib-test) zlib="no"
|
|
;;
|
|
--disable-lzo) lzo="no"
|
|
;;
|
|
--enable-lzo) lzo="yes"
|
|
;;
|
|
--disable-snappy) snappy="no"
|
|
;;
|
|
--enable-snappy) snappy="yes"
|
|
;;
|
|
--disable-bzip2) bzip2="no"
|
|
;;
|
|
--enable-bzip2) bzip2="yes"
|
|
;;
|
|
--enable-guest-agent) guest_agent="yes"
|
|
;;
|
|
--disable-guest-agent) guest_agent="no"
|
|
;;
|
|
--enable-guest-agent-msi) guest_agent_msi="yes"
|
|
;;
|
|
--disable-guest-agent-msi) guest_agent_msi="no"
|
|
;;
|
|
--with-vss-sdk) vss_win32_sdk=""
|
|
;;
|
|
--with-vss-sdk=*) vss_win32_sdk="$optarg"
|
|
;;
|
|
--without-vss-sdk) vss_win32_sdk="no"
|
|
;;
|
|
--with-win-sdk) win_sdk=""
|
|
;;
|
|
--with-win-sdk=*) win_sdk="$optarg"
|
|
;;
|
|
--without-win-sdk) win_sdk="no"
|
|
;;
|
|
--enable-tools) want_tools="yes"
|
|
;;
|
|
--disable-tools) want_tools="no"
|
|
;;
|
|
--enable-seccomp) seccomp="yes"
|
|
;;
|
|
--disable-seccomp) seccomp="no"
|
|
;;
|
|
--disable-glusterfs) glusterfs="no"
|
|
;;
|
|
--enable-glusterfs) glusterfs="yes"
|
|
;;
|
|
--disable-archipelago) archipelago="no"
|
|
;;
|
|
--enable-archipelago) archipelago="yes"
|
|
;;
|
|
--disable-virtio-blk-data-plane|--enable-virtio-blk-data-plane)
|
|
echo "$0: $opt is obsolete, virtio-blk data-plane is always on" >&2
|
|
;;
|
|
--disable-gtk) gtk="no"
|
|
;;
|
|
--enable-gtk) gtk="yes"
|
|
;;
|
|
--disable-gnutls) gnutls="no"
|
|
;;
|
|
--enable-gnutls) gnutls="yes"
|
|
;;
|
|
--enable-rdma) rdma="yes"
|
|
;;
|
|
--disable-rdma) rdma="no"
|
|
;;
|
|
--with-gtkabi=*) gtkabi="$optarg"
|
|
;;
|
|
--disable-vte) vte="no"
|
|
;;
|
|
--enable-vte) vte="yes"
|
|
;;
|
|
--disable-tpm) tpm="no"
|
|
;;
|
|
--enable-tpm) tpm="yes"
|
|
;;
|
|
--disable-libssh2) libssh2="no"
|
|
;;
|
|
--enable-libssh2) libssh2="yes"
|
|
;;
|
|
--enable-vhdx) vhdx="yes"
|
|
;;
|
|
--disable-vhdx) vhdx="no"
|
|
;;
|
|
--disable-quorum) quorum="no"
|
|
;;
|
|
--enable-quorum) quorum="yes"
|
|
;;
|
|
--disable-numa) numa="no"
|
|
;;
|
|
--enable-numa) numa="yes"
|
|
;;
|
|
--disable-tcmalloc) tcmalloc="no"
|
|
;;
|
|
--enable-tcmalloc) tcmalloc="yes"
|
|
;;
|
|
*)
|
|
echo "ERROR: unknown option $opt"
|
|
echo "Try '$0 --help' for more information"
|
|
exit 1
|
|
;;
|
|
esac
|
|
done
|
|
|
|
if ! has $python; then
|
|
error_exit "Python not found. Use --python=/path/to/python"
|
|
fi
|
|
|
|
# Note that if the Python conditional here evaluates True we will exit
|
|
# with status 1 which is a shell 'false' value.
|
|
if ! $python -c 'import sys; sys.exit(sys.version_info < (2,4) or sys.version_info >= (3,))'; then
|
|
error_exit "Cannot use '$python', Python 2.4 or later is required." \
|
|
"Note that Python 3 or later is not yet supported." \
|
|
"Use --python=/path/to/python to specify a supported Python."
|
|
fi
|
|
|
|
# The -B switch was added in Python 2.6.
|
|
# If it is supplied, compiled files are not written.
|
|
# Use it for Python versions which support it.
|
|
if $python -B -c 'import sys; sys.exit(0)' 2>/dev/null; then
|
|
python="$python -B"
|
|
fi
|
|
|
|
case "$cpu" in
|
|
ppc)
|
|
CPU_CFLAGS="-m32"
|
|
LDFLAGS="-m32 $LDFLAGS"
|
|
;;
|
|
ppc64)
|
|
CPU_CFLAGS="-m64"
|
|
LDFLAGS="-m64 $LDFLAGS"
|
|
;;
|
|
sparc)
|
|
LDFLAGS="-m32 $LDFLAGS"
|
|
CPU_CFLAGS="-m32 -mcpu=ultrasparc"
|
|
;;
|
|
sparc64)
|
|
LDFLAGS="-m64 $LDFLAGS"
|
|
CPU_CFLAGS="-m64 -mcpu=ultrasparc"
|
|
;;
|
|
s390)
|
|
CPU_CFLAGS="-m31"
|
|
LDFLAGS="-m31 $LDFLAGS"
|
|
;;
|
|
s390x)
|
|
CPU_CFLAGS="-m64"
|
|
LDFLAGS="-m64 $LDFLAGS"
|
|
;;
|
|
i386)
|
|
CPU_CFLAGS="-m32"
|
|
LDFLAGS="-m32 $LDFLAGS"
|
|
cc_i386='$(CC) -m32'
|
|
;;
|
|
x86_64)
|
|
CPU_CFLAGS="-m64"
|
|
LDFLAGS="-m64 $LDFLAGS"
|
|
cc_i386='$(CC) -m32'
|
|
;;
|
|
x32)
|
|
CPU_CFLAGS="-mx32"
|
|
LDFLAGS="-mx32 $LDFLAGS"
|
|
cc_i386='$(CC) -m32'
|
|
;;
|
|
# No special flags required for other host CPUs
|
|
esac
|
|
|
|
QEMU_CFLAGS="$CPU_CFLAGS $QEMU_CFLAGS"
|
|
EXTRA_CFLAGS="$CPU_CFLAGS $EXTRA_CFLAGS"
|
|
|
|
default_target_list=""
|
|
|
|
mak_wilds=""
|
|
|
|
if [ "$softmmu" = "yes" ]; then
|
|
mak_wilds="${mak_wilds} $source_path/default-configs/*-softmmu.mak"
|
|
fi
|
|
if [ "$linux_user" = "yes" ]; then
|
|
mak_wilds="${mak_wilds} $source_path/default-configs/*-linux-user.mak"
|
|
fi
|
|
if [ "$bsd_user" = "yes" ]; then
|
|
mak_wilds="${mak_wilds} $source_path/default-configs/*-bsd-user.mak"
|
|
fi
|
|
|
|
for config in $mak_wilds; do
|
|
default_target_list="${default_target_list} $(basename "$config" .mak)"
|
|
done
|
|
|
|
if test x"$show_help" = x"yes" ; then
|
|
cat << EOF
|
|
|
|
Usage: configure [options]
|
|
Options: [defaults in brackets after descriptions]
|
|
|
|
Standard options:
|
|
--help print this message
|
|
--prefix=PREFIX install in PREFIX [$prefix]
|
|
--interp-prefix=PREFIX where to find shared libraries, etc.
|
|
use %M for cpu name [$interp_prefix]
|
|
--target-list=LIST set target list (default: build everything)
|
|
$(echo Available targets: $default_target_list | \
|
|
fold -s -w 53 | sed -e 's/^/ /')
|
|
|
|
Advanced options (experts only):
|
|
--source-path=PATH path of source code [$source_path]
|
|
--cross-prefix=PREFIX use PREFIX for compile tools [$cross_prefix]
|
|
--cc=CC use C compiler CC [$cc]
|
|
--iasl=IASL use ACPI compiler IASL [$iasl]
|
|
--host-cc=CC use C compiler CC [$host_cc] for code run at
|
|
build time
|
|
--cxx=CXX use C++ compiler CXX [$cxx]
|
|
--objcc=OBJCC use Objective-C compiler OBJCC [$objcc]
|
|
--extra-cflags=CFLAGS append extra C compiler flags QEMU_CFLAGS
|
|
--extra-ldflags=LDFLAGS append extra linker flags LDFLAGS
|
|
--make=MAKE use specified make [$make]
|
|
--install=INSTALL use specified install [$install]
|
|
--python=PYTHON use specified python [$python]
|
|
--smbd=SMBD use specified smbd [$smbd]
|
|
--static enable static build [$static]
|
|
--mandir=PATH install man pages in PATH
|
|
--datadir=PATH install firmware in PATH$confsuffix
|
|
--docdir=PATH install documentation in PATH$confsuffix
|
|
--bindir=PATH install binaries in PATH
|
|
--libdir=PATH install libraries in PATH
|
|
--sysconfdir=PATH install config in PATH$confsuffix
|
|
--localstatedir=PATH install local state in PATH (set at runtime on win32)
|
|
--with-confsuffix=SUFFIX suffix for QEMU data inside datadir/libdir/sysconfdir [$confsuffix]
|
|
--enable-debug enable common debug build options
|
|
--disable-strip disable stripping binaries
|
|
--disable-werror disable compilation abort on warning
|
|
--disable-stack-protector disable compiler-provided stack protection
|
|
--audio-drv-list=LIST set audio drivers list:
|
|
Available drivers: $audio_possible_drivers
|
|
--block-drv-whitelist=L Same as --block-drv-rw-whitelist=L
|
|
--block-drv-rw-whitelist=L
|
|
set block driver read-write whitelist
|
|
(affects only QEMU, not qemu-img)
|
|
--block-drv-ro-whitelist=L
|
|
set block driver read-only whitelist
|
|
(affects only QEMU, not qemu-img)
|
|
--enable-trace-backends=B Set trace backend
|
|
Available backends: $($python $source_path/scripts/tracetool.py --list-backends)
|
|
--with-trace-file=NAME Full PATH,NAME of file to store traces
|
|
Default:trace-<pid>
|
|
--disable-slirp disable SLIRP userspace network connectivity
|
|
--enable-tcg-interpreter enable TCG with bytecode interpreter (TCI)
|
|
--oss-lib path to OSS library
|
|
--cpu=CPU Build for host CPU [$cpu]
|
|
--with-coroutine=BACKEND coroutine backend. Supported options:
|
|
gthread, ucontext, sigaltstack, windows
|
|
--enable-gcov enable test coverage analysis with gcov
|
|
--gcov=GCOV use specified gcov [$gcov_tool]
|
|
--disable-blobs disable installing provided firmware blobs
|
|
--with-vss-sdk=SDK-path enable Windows VSS support in QEMU Guest Agent
|
|
--with-win-sdk=SDK-path path to Windows Platform SDK (to build VSS .tlb)
|
|
|
|
Optional features, enabled with --enable-FEATURE and
|
|
disabled with --disable-FEATURE, default is enabled if available:
|
|
|
|
system all system emulation targets
|
|
user supported user emulation targets
|
|
linux-user all linux usermode emulation targets
|
|
bsd-user all BSD usermode emulation targets
|
|
guest-base GUEST_BASE support for usermode emulation targets
|
|
docs build documentation
|
|
guest-agent build the QEMU Guest Agent
|
|
guest-agent-msi build guest agent Windows MSI installation package
|
|
pie Position Independent Executables
|
|
modules modules support
|
|
debug-tcg TCG debugging (default is disabled)
|
|
debug-info debugging information
|
|
sparse sparse checker
|
|
|
|
gnutls GNUTLS cryptography support
|
|
sdl SDL UI
|
|
--with-sdlabi select preferred SDL ABI 1.2 or 2.0
|
|
gtk gtk UI
|
|
--with-gtkabi select preferred GTK ABI 2.0 or 3.0
|
|
vte vte support for the gtk UI
|
|
curses curses UI
|
|
vnc VNC UI support
|
|
vnc-tls TLS encryption for VNC server
|
|
vnc-sasl SASL encryption for VNC server
|
|
vnc-jpeg JPEG lossy compression for VNC server
|
|
vnc-png PNG compression for VNC server
|
|
vnc-ws Websockets support for VNC server
|
|
cocoa Cocoa UI (Mac OS X only)
|
|
virtfs VirtFS
|
|
xen xen backend driver support
|
|
xen-pci-passthrough
|
|
brlapi BrlAPI (Braile)
|
|
curl curl connectivity
|
|
fdt fdt device tree
|
|
bluez bluez stack connectivity
|
|
kvm KVM acceleration support
|
|
rdma RDMA-based migration support
|
|
uuid uuid support
|
|
vde support for vde network
|
|
netmap support for netmap network
|
|
linux-aio Linux AIO support
|
|
cap-ng libcap-ng support
|
|
attr attr and xattr support
|
|
vhost-net vhost-net acceleration support
|
|
spice spice
|
|
rbd rados block device (rbd)
|
|
libiscsi iscsi support
|
|
libnfs nfs support
|
|
smartcard-nss smartcard nss support
|
|
libusb libusb (for usb passthrough)
|
|
usb-redir usb network redirection support
|
|
lzo support of lzo compression library
|
|
snappy support of snappy compression library
|
|
bzip2 support of bzip2 compression library
|
|
(for reading bzip2-compressed dmg images)
|
|
seccomp seccomp support
|
|
coroutine-pool coroutine freelist (better performance)
|
|
glusterfs GlusterFS backend
|
|
archipelago Archipelago backend
|
|
tpm TPM support
|
|
libssh2 ssh block device support
|
|
vhdx support for the Microsoft VHDX image format
|
|
quorum quorum block filter support
|
|
numa libnuma support
|
|
tcmalloc tcmalloc support
|
|
|
|
NOTE: The object files are built at the place where configure is launched
|
|
EOF
|
|
exit 0
|
|
fi
|
|
|
|
# Now we have handled --enable-tcg-interpreter and know we're not just
|
|
# printing the help message, bail out if the host CPU isn't supported.
|
|
if test "$ARCH" = "unknown"; then
|
|
if test "$tcg_interpreter" = "yes" ; then
|
|
echo "Unsupported CPU = $cpu, will use TCG with TCI (experimental)"
|
|
ARCH=tci
|
|
else
|
|
error_exit "Unsupported CPU = $cpu, try --enable-tcg-interpreter"
|
|
fi
|
|
fi
|
|
|
|
# Consult white-list to determine whether to enable werror
|
|
# by default. Only enable by default for git builds
|
|
z_version=`cut -f3 -d. $source_path/VERSION`
|
|
|
|
if test -z "$werror" ; then
|
|
if test -d "$source_path/.git" -a \
|
|
"$linux" = "yes" ; then
|
|
werror="yes"
|
|
else
|
|
werror="no"
|
|
fi
|
|
fi
|
|
|
|
# check that the C compiler works.
|
|
write_c_skeleton;
|
|
if compile_object ; then
|
|
: C compiler works ok
|
|
else
|
|
error_exit "\"$cc\" either does not exist or does not work"
|
|
fi
|
|
|
|
# Check that the C++ compiler exists and works with the C compiler
|
|
if has $cxx; then
|
|
cat > $TMPC <<EOF
|
|
int c_function(void);
|
|
int main(void) { return c_function(); }
|
|
EOF
|
|
|
|
compile_object
|
|
|
|
cat > $TMPCXX <<EOF
|
|
extern "C" {
|
|
int c_function(void);
|
|
}
|
|
int c_function(void) { return 42; }
|
|
EOF
|
|
|
|
update_cxxflags
|
|
|
|
if do_cxx $QEMU_CXXFLAGS -o $TMPE $TMPCXX $TMPO $LDFLAGS; then
|
|
# C++ compiler $cxx works ok with C compiler $cc
|
|
:
|
|
else
|
|
echo "C++ compiler $cxx does not work with C compiler $cc"
|
|
echo "Disabling C++ specific optional code"
|
|
cxx=
|
|
fi
|
|
else
|
|
echo "No C++ compiler available; disabling C++ specific optional code"
|
|
cxx=
|
|
fi
|
|
|
|
gcc_flags="-Wold-style-declaration -Wold-style-definition -Wtype-limits"
|
|
gcc_flags="-Wformat-security -Wformat-y2k -Winit-self -Wignored-qualifiers $gcc_flags"
|
|
gcc_flags="-Wmissing-include-dirs -Wempty-body -Wnested-externs $gcc_flags"
|
|
gcc_flags="-Wendif-labels $gcc_flags"
|
|
gcc_flags="-Wno-initializer-overrides $gcc_flags"
|
|
gcc_flags="-Wno-string-plus-int $gcc_flags"
|
|
# Note that we do not add -Werror to gcc_flags here, because that would
|
|
# enable it for all configure tests. If a configure test failed due
|
|
# to -Werror this would just silently disable some features,
|
|
# so it's too error prone.
|
|
|
|
cc_has_warning_flag() {
|
|
write_c_skeleton;
|
|
|
|
# Use the positive sense of the flag when testing for -Wno-wombat
|
|
# support (gcc will happily accept the -Wno- form of unknown
|
|
# warning options).
|
|
optflag="$(echo $1 | sed -e 's/^-Wno-/-W/')"
|
|
compile_prog "-Werror $optflag" ""
|
|
}
|
|
|
|
for flag in $gcc_flags; do
|
|
if cc_has_warning_flag $flag ; then
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $flag"
|
|
fi
|
|
done
|
|
|
|
if test "$stack_protector" != "no"; then
|
|
gcc_flags="-fstack-protector-strong -fstack-protector-all"
|
|
sp_on=0
|
|
for flag in $gcc_flags; do
|
|
# We need to check both a compile and a link, since some compiler
|
|
# setups fail only on a .c->.o compile and some only at link time
|
|
if do_cc $QEMU_CFLAGS -Werror $flag -c -o $TMPO $TMPC &&
|
|
compile_prog "-Werror $flag" ""; then
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $flag"
|
|
LIBTOOLFLAGS="$LIBTOOLFLAGS -Wc,$flag"
|
|
sp_on=1
|
|
break
|
|
fi
|
|
done
|
|
if test "$stack_protector" = yes; then
|
|
if test $sp_on = 0; then
|
|
error_exit "Stack protector not supported"
|
|
fi
|
|
fi
|
|
fi
|
|
|
|
# Workaround for http://gcc.gnu.org/PR55489. Happens with -fPIE/-fPIC and
|
|
# large functions that use global variables. The bug is in all releases of
|
|
# GCC, but it became particularly acute in 4.6.x and 4.7.x. It is fixed in
|
|
# 4.7.3 and 4.8.0. We should be able to delete this at the end of 2013.
|
|
cat > $TMPC << EOF
|
|
#if __GNUC__ == 4 && (__GNUC_MINOR__ == 6 || (__GNUC_MINOR__ == 7 && __GNUC_PATCHLEVEL__ <= 2))
|
|
int main(void) { return 0; }
|
|
#else
|
|
#error No bug in this compiler.
|
|
#endif
|
|
EOF
|
|
if compile_prog "-Werror -fno-gcse" "" ; then
|
|
TRANSLATE_OPT_CFLAGS=-fno-gcse
|
|
fi
|
|
|
|
if test "$static" = "yes" ; then
|
|
if test "$modules" = "yes" ; then
|
|
error_exit "static and modules are mutually incompatible"
|
|
fi
|
|
if test "$pie" = "yes" ; then
|
|
error_exit "static and pie are mutually incompatible"
|
|
else
|
|
pie="no"
|
|
fi
|
|
fi
|
|
|
|
# Unconditional check for compiler __thread support
|
|
cat > $TMPC << EOF
|
|
static __thread int tls_var;
|
|
int main(void) { return tls_var; }
|
|
EOF
|
|
|
|
if ! compile_prog "-Werror" "" ; then
|
|
error_exit "Your compiler does not support the __thread specifier for " \
|
|
"Thread-Local Storage (TLS). Please upgrade to a version that does."
|
|
fi
|
|
|
|
if test "$pie" = ""; then
|
|
case "$cpu-$targetos" in
|
|
i386-Linux|x86_64-Linux|x32-Linux|i386-OpenBSD|x86_64-OpenBSD)
|
|
;;
|
|
*)
|
|
pie="no"
|
|
;;
|
|
esac
|
|
fi
|
|
|
|
if test "$pie" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
|
|
#ifdef __linux__
|
|
# define THREAD __thread
|
|
#else
|
|
# define THREAD
|
|
#endif
|
|
|
|
static THREAD int tls_var;
|
|
|
|
int main(void) { return tls_var; }
|
|
|
|
EOF
|
|
if compile_prog "-fPIE -DPIE" "-pie"; then
|
|
QEMU_CFLAGS="-fPIE -DPIE $QEMU_CFLAGS"
|
|
LDFLAGS="-pie $LDFLAGS"
|
|
pie="yes"
|
|
if compile_prog "" "-Wl,-z,relro -Wl,-z,now" ; then
|
|
LDFLAGS="-Wl,-z,relro -Wl,-z,now $LDFLAGS"
|
|
fi
|
|
else
|
|
if test "$pie" = "yes"; then
|
|
error_exit "PIE not available due to missing toolchain support"
|
|
else
|
|
echo "Disabling PIE due to missing toolchain support"
|
|
pie="no"
|
|
fi
|
|
fi
|
|
|
|
if compile_prog "-Werror -fno-pie" "-nopie"; then
|
|
CFLAGS_NOPIE="-fno-pie"
|
|
LDFLAGS_NOPIE="-nopie"
|
|
fi
|
|
fi
|
|
|
|
# check for broken gcc and libtool in RHEL5
|
|
if test -n "$libtool" -a "$pie" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
|
|
void *f(unsigned char *buf, int len);
|
|
void *g(unsigned char *buf, int len);
|
|
|
|
void *
|
|
f(unsigned char *buf, int len)
|
|
{
|
|
return (void*)0L;
|
|
}
|
|
|
|
void *
|
|
g(unsigned char *buf, int len)
|
|
{
|
|
return f(buf, len);
|
|
}
|
|
|
|
EOF
|
|
if ! libtool_prog; then
|
|
echo "Disabling libtool due to broken toolchain support"
|
|
libtool=
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# __sync_fetch_and_and requires at least -march=i486. Many toolchains
|
|
# use i686 as default anyway, but for those that don't, an explicit
|
|
# specification is necessary
|
|
|
|
if test "$cpu" = "i386"; then
|
|
cat > $TMPC << EOF
|
|
static int sfaa(int *ptr)
|
|
{
|
|
return __sync_fetch_and_and(ptr, 0);
|
|
}
|
|
|
|
int main(void)
|
|
{
|
|
int val = 42;
|
|
val = __sync_val_compare_and_swap(&val, 0, 1);
|
|
sfaa(&val);
|
|
return val;
|
|
}
|
|
EOF
|
|
if ! compile_prog "" "" ; then
|
|
QEMU_CFLAGS="-march=i486 $QEMU_CFLAGS"
|
|
fi
|
|
fi
|
|
|
|
#########################################
|
|
# Solaris specific configure tool chain decisions
|
|
|
|
if test "$solaris" = "yes" ; then
|
|
if has $install; then
|
|
:
|
|
else
|
|
error_exit "Solaris install program not found. Use --install=/usr/ucb/install or" \
|
|
"install fileutils from www.blastwave.org using pkg-get -i fileutils" \
|
|
"to get ginstall which is used by default (which lives in /opt/csw/bin)"
|
|
fi
|
|
if test "`path_of $install`" = "/usr/sbin/install" ; then
|
|
error_exit "Solaris /usr/sbin/install is not an appropriate install program." \
|
|
"try ginstall from the GNU fileutils available from www.blastwave.org" \
|
|
"using pkg-get -i fileutils, or use --install=/usr/ucb/install"
|
|
fi
|
|
if has ar; then
|
|
:
|
|
else
|
|
if test -f /usr/ccs/bin/ar ; then
|
|
error_exit "No path includes ar" \
|
|
"Add /usr/ccs/bin to your path and rerun configure"
|
|
fi
|
|
error_exit "No path includes ar"
|
|
fi
|
|
fi
|
|
|
|
if test -z "${target_list+xxx}" ; then
|
|
target_list="$default_target_list"
|
|
else
|
|
target_list=`echo "$target_list" | sed -e 's/,/ /g'`
|
|
fi
|
|
|
|
# Check that we recognised the target name; this allows a more
|
|
# friendly error message than if we let it fall through.
|
|
for target in $target_list; do
|
|
case " $default_target_list " in
|
|
*" $target "*)
|
|
;;
|
|
*)
|
|
error_exit "Unknown target name '$target'"
|
|
;;
|
|
esac
|
|
done
|
|
|
|
# see if system emulation was really requested
|
|
case " $target_list " in
|
|
*"-softmmu "*) softmmu=yes
|
|
;;
|
|
*) softmmu=no
|
|
;;
|
|
esac
|
|
|
|
feature_not_found() {
|
|
feature=$1
|
|
remedy=$2
|
|
|
|
error_exit "User requested feature $feature" \
|
|
"configure was not able to find it." \
|
|
"$remedy"
|
|
}
|
|
|
|
# ---
|
|
# big/little endian test
|
|
cat > $TMPC << EOF
|
|
short big_endian[] = { 0x4269, 0x4765, 0x4e64, 0x4961, 0x4e00, 0, };
|
|
short little_endian[] = { 0x694c, 0x7454, 0x654c, 0x6e45, 0x6944, 0x6e41, 0, };
|
|
extern int foo(short *, short *);
|
|
int main(int argc, char *argv[]) {
|
|
return foo(big_endian, little_endian);
|
|
}
|
|
EOF
|
|
|
|
if compile_object ; then
|
|
if grep -q BiGeNdIaN $TMPO ; then
|
|
bigendian="yes"
|
|
elif grep -q LiTtLeEnDiAn $TMPO ; then
|
|
bigendian="no"
|
|
else
|
|
echo big/little test failed
|
|
fi
|
|
else
|
|
echo big/little test failed
|
|
fi
|
|
|
|
##########################################
|
|
# L2TPV3 probe
|
|
|
|
cat > $TMPC <<EOF
|
|
#include <sys/socket.h>
|
|
#include <linux/ip.h>
|
|
int main(void) { return sizeof(struct mmsghdr); }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
l2tpv3=yes
|
|
else
|
|
l2tpv3=no
|
|
fi
|
|
|
|
##########################################
|
|
# pkg-config probe
|
|
|
|
if ! has "$pkg_config_exe"; then
|
|
error_exit "pkg-config binary '$pkg_config_exe' not found"
|
|
fi
|
|
|
|
##########################################
|
|
# NPTL probe
|
|
|
|
if test "$linux_user" = "yes"; then
|
|
cat > $TMPC <<EOF
|
|
#include <sched.h>
|
|
#include <linux/futex.h>
|
|
int main(void) {
|
|
#if !defined(CLONE_SETTLS) || !defined(FUTEX_WAIT)
|
|
#error bork
|
|
#endif
|
|
return 0;
|
|
}
|
|
EOF
|
|
if ! compile_object ; then
|
|
feature_not_found "nptl" "Install glibc and linux kernel headers."
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# zlib check
|
|
|
|
if test "$zlib" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <zlib.h>
|
|
int main(void) { zlibVersion(); return 0; }
|
|
EOF
|
|
if compile_prog "" "-lz" ; then
|
|
:
|
|
else
|
|
error_exit "zlib check failed" \
|
|
"Make sure to have the zlib libs and headers installed."
|
|
fi
|
|
fi
|
|
LIBS="$LIBS -lz"
|
|
|
|
##########################################
|
|
# lzo check
|
|
|
|
if test "$lzo" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <lzo/lzo1x.h>
|
|
int main(void) { lzo_version(); return 0; }
|
|
EOF
|
|
if compile_prog "" "-llzo2" ; then
|
|
libs_softmmu="$libs_softmmu -llzo2"
|
|
lzo="yes"
|
|
else
|
|
if test "$lzo" = "yes"; then
|
|
feature_not_found "liblzo2" "Install liblzo2 devel"
|
|
fi
|
|
lzo="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# snappy check
|
|
|
|
if test "$snappy" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <snappy-c.h>
|
|
int main(void) { snappy_max_compressed_length(4096); return 0; }
|
|
EOF
|
|
if compile_prog "" "-lsnappy" ; then
|
|
libs_softmmu="$libs_softmmu -lsnappy"
|
|
snappy="yes"
|
|
else
|
|
if test "$snappy" = "yes"; then
|
|
feature_not_found "libsnappy" "Install libsnappy devel"
|
|
fi
|
|
snappy="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# bzip2 check
|
|
|
|
if test "$bzip2" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <bzlib.h>
|
|
int main(void) { BZ2_bzlibVersion(); return 0; }
|
|
EOF
|
|
if compile_prog "" "-lbz2" ; then
|
|
bzip2="yes"
|
|
else
|
|
if test "$bzip2" = "yes"; then
|
|
feature_not_found "libbzip2" "Install libbzip2 devel"
|
|
fi
|
|
bzip2="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# libseccomp check
|
|
|
|
if test "$seccomp" != "no" ; then
|
|
if test "$cpu" = "i386" || test "$cpu" = "x86_64" &&
|
|
$pkg_config --atleast-version=2.1.1 libseccomp; then
|
|
libs_softmmu="$libs_softmmu `$pkg_config --libs libseccomp`"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS `$pkg_config --cflags libseccomp`"
|
|
seccomp="yes"
|
|
else
|
|
if test "$seccomp" = "yes"; then
|
|
feature_not_found "libseccomp" "Install libseccomp devel >= 2.1.1"
|
|
fi
|
|
seccomp="no"
|
|
fi
|
|
fi
|
|
##########################################
|
|
# xen probe
|
|
|
|
if test "$xen" != "no" ; then
|
|
xen_libs="-lxenstore -lxenctrl -lxenguest"
|
|
|
|
# First we test whether Xen headers and libraries are available.
|
|
# If no, we are done and there is no Xen support.
|
|
# If yes, more tests are run to detect the Xen version.
|
|
|
|
# Xen (any)
|
|
cat > $TMPC <<EOF
|
|
#include <xenctrl.h>
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
if ! compile_prog "" "$xen_libs" ; then
|
|
# Xen not found
|
|
if test "$xen" = "yes" ; then
|
|
feature_not_found "xen" "Install xen devel"
|
|
fi
|
|
xen=no
|
|
|
|
# Xen unstable
|
|
elif
|
|
cat > $TMPC <<EOF &&
|
|
#include <xenctrl.h>
|
|
#include <xenstore.h>
|
|
#include <stdint.h>
|
|
#include <xen/hvm/hvm_info_table.h>
|
|
#if !defined(HVM_MAX_VCPUS)
|
|
# error HVM_MAX_VCPUS not defined
|
|
#endif
|
|
int main(void) {
|
|
xc_interface *xc;
|
|
xs_daemon_open();
|
|
xc = xc_interface_open(0, 0, 0);
|
|
xc_hvm_set_mem_type(0, 0, HVMMEM_ram_ro, 0, 0);
|
|
xc_gnttab_open(NULL, 0);
|
|
xc_domain_add_to_physmap(0, 0, XENMAPSPACE_gmfn, 0, 0);
|
|
xc_hvm_inject_msi(xc, 0, 0xf0000000, 0x00000000);
|
|
xc_hvm_create_ioreq_server(xc, 0, 0, NULL);
|
|
return 0;
|
|
}
|
|
EOF
|
|
compile_prog "" "$xen_libs"
|
|
then
|
|
xen_ctrl_version=450
|
|
xen=yes
|
|
|
|
elif
|
|
cat > $TMPC <<EOF &&
|
|
#include <xenctrl.h>
|
|
#include <xenstore.h>
|
|
#include <stdint.h>
|
|
#include <xen/hvm/hvm_info_table.h>
|
|
#if !defined(HVM_MAX_VCPUS)
|
|
# error HVM_MAX_VCPUS not defined
|
|
#endif
|
|
int main(void) {
|
|
xc_interface *xc;
|
|
xs_daemon_open();
|
|
xc = xc_interface_open(0, 0, 0);
|
|
xc_hvm_set_mem_type(0, 0, HVMMEM_ram_ro, 0, 0);
|
|
xc_gnttab_open(NULL, 0);
|
|
xc_domain_add_to_physmap(0, 0, XENMAPSPACE_gmfn, 0, 0);
|
|
xc_hvm_inject_msi(xc, 0, 0xf0000000, 0x00000000);
|
|
return 0;
|
|
}
|
|
EOF
|
|
compile_prog "" "$xen_libs"
|
|
then
|
|
xen_ctrl_version=420
|
|
xen=yes
|
|
|
|
elif
|
|
cat > $TMPC <<EOF &&
|
|
#include <xenctrl.h>
|
|
#include <xs.h>
|
|
#include <stdint.h>
|
|
#include <xen/hvm/hvm_info_table.h>
|
|
#if !defined(HVM_MAX_VCPUS)
|
|
# error HVM_MAX_VCPUS not defined
|
|
#endif
|
|
int main(void) {
|
|
xs_daemon_open();
|
|
xc_interface_open(0, 0, 0);
|
|
xc_hvm_set_mem_type(0, 0, HVMMEM_ram_ro, 0, 0);
|
|
xc_gnttab_open(NULL, 0);
|
|
xc_domain_add_to_physmap(0, 0, XENMAPSPACE_gmfn, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
compile_prog "" "$xen_libs"
|
|
then
|
|
xen_ctrl_version=410
|
|
xen=yes
|
|
|
|
# Xen 4.0.0
|
|
elif
|
|
cat > $TMPC <<EOF &&
|
|
#include <xenctrl.h>
|
|
#include <xs.h>
|
|
#include <stdint.h>
|
|
#include <xen/hvm/hvm_info_table.h>
|
|
#if !defined(HVM_MAX_VCPUS)
|
|
# error HVM_MAX_VCPUS not defined
|
|
#endif
|
|
int main(void) {
|
|
struct xen_add_to_physmap xatp = {
|
|
.domid = 0, .space = XENMAPSPACE_gmfn, .idx = 0, .gpfn = 0,
|
|
};
|
|
xs_daemon_open();
|
|
xc_interface_open();
|
|
xc_gnttab_open();
|
|
xc_hvm_set_mem_type(0, 0, HVMMEM_ram_ro, 0, 0);
|
|
xc_memory_op(0, XENMEM_add_to_physmap, &xatp);
|
|
return 0;
|
|
}
|
|
EOF
|
|
compile_prog "" "$xen_libs"
|
|
then
|
|
xen_ctrl_version=400
|
|
xen=yes
|
|
|
|
# Xen 3.4.0
|
|
elif
|
|
cat > $TMPC <<EOF &&
|
|
#include <xenctrl.h>
|
|
#include <xs.h>
|
|
int main(void) {
|
|
struct xen_add_to_physmap xatp = {
|
|
.domid = 0, .space = XENMAPSPACE_gmfn, .idx = 0, .gpfn = 0,
|
|
};
|
|
xs_daemon_open();
|
|
xc_interface_open();
|
|
xc_gnttab_open();
|
|
xc_hvm_set_mem_type(0, 0, HVMMEM_ram_ro, 0, 0);
|
|
xc_memory_op(0, XENMEM_add_to_physmap, &xatp);
|
|
return 0;
|
|
}
|
|
EOF
|
|
compile_prog "" "$xen_libs"
|
|
then
|
|
xen_ctrl_version=340
|
|
xen=yes
|
|
|
|
# Xen 3.3.0
|
|
elif
|
|
cat > $TMPC <<EOF &&
|
|
#include <xenctrl.h>
|
|
#include <xs.h>
|
|
int main(void) {
|
|
xs_daemon_open();
|
|
xc_interface_open();
|
|
xc_gnttab_open();
|
|
xc_hvm_set_mem_type(0, 0, HVMMEM_ram_ro, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
compile_prog "" "$xen_libs"
|
|
then
|
|
xen_ctrl_version=330
|
|
xen=yes
|
|
|
|
# Xen version unsupported
|
|
else
|
|
if test "$xen" = "yes" ; then
|
|
feature_not_found "xen (unsupported version)" "Install supported xen (e.g. 4.0, 3.4, 3.3)"
|
|
fi
|
|
xen=no
|
|
fi
|
|
|
|
if test "$xen" = yes; then
|
|
libs_softmmu="$xen_libs $libs_softmmu"
|
|
fi
|
|
fi
|
|
|
|
if test "$xen_pci_passthrough" != "no"; then
|
|
if test "$xen" = "yes" && test "$linux" = "yes" &&
|
|
test "$xen_ctrl_version" -ge 340; then
|
|
xen_pci_passthrough=yes
|
|
else
|
|
if test "$xen_pci_passthrough" = "yes"; then
|
|
if test "$xen_ctrl_version" -lt 340; then
|
|
error_exit "User requested feature Xen PCI Passthrough" \
|
|
"This feature does not work with Xen 3.3"
|
|
fi
|
|
error_exit "User requested feature Xen PCI Passthrough" \
|
|
" but this feature requires /sys from Linux"
|
|
fi
|
|
xen_pci_passthrough=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# libtool probe
|
|
|
|
if ! has $libtool; then
|
|
libtool=
|
|
fi
|
|
|
|
# MacOSX ships with a libtool which isn't the GNU one; weed this
|
|
# out by checking whether libtool supports the --version switch
|
|
if test -n "$libtool"; then
|
|
if ! "$libtool" --version >/dev/null 2>&1; then
|
|
libtool=
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# Sparse probe
|
|
if test "$sparse" != "no" ; then
|
|
if has cgcc; then
|
|
sparse=yes
|
|
else
|
|
if test "$sparse" = "yes" ; then
|
|
feature_not_found "sparse" "Install sparse binary"
|
|
fi
|
|
sparse=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# X11 probe
|
|
x11_cflags=
|
|
x11_libs=-lX11
|
|
if $pkg_config --exists "x11"; then
|
|
x11_cflags=`$pkg_config --cflags x11`
|
|
x11_libs=`$pkg_config --libs x11`
|
|
fi
|
|
|
|
##########################################
|
|
# GTK probe
|
|
|
|
if test "$gtkabi" = ""; then
|
|
# The GTK ABI was not specified explicitly, so try whether 2.0 is available.
|
|
# Use 3.0 as a fallback if that is available.
|
|
if $pkg_config --exists "gtk+-2.0 >= 2.18.0"; then
|
|
gtkabi=2.0
|
|
elif $pkg_config --exists "gtk+-3.0 >= 3.0.0"; then
|
|
gtkabi=3.0
|
|
else
|
|
gtkabi=2.0
|
|
fi
|
|
fi
|
|
|
|
if test "$gtk" != "no"; then
|
|
gtkpackage="gtk+-$gtkabi"
|
|
gtkx11package="gtk+-x11-$gtkabi"
|
|
if test "$gtkabi" = "3.0" ; then
|
|
gtkversion="3.0.0"
|
|
else
|
|
gtkversion="2.18.0"
|
|
fi
|
|
if $pkg_config --exists "$gtkpackage >= $gtkversion"; then
|
|
gtk_cflags=`$pkg_config --cflags $gtkpackage`
|
|
gtk_libs=`$pkg_config --libs $gtkpackage`
|
|
if $pkg_config --exists "$gtkx11package >= $gtkversion"; then
|
|
gtk_cflags="$gtk_cflags $x11_cflags"
|
|
gtk_libs="$gtk_libs $x11_libs"
|
|
fi
|
|
libs_softmmu="$gtk_libs $libs_softmmu"
|
|
gtk="yes"
|
|
elif test "$gtk" = "yes"; then
|
|
feature_not_found "gtk" "Install gtk2 or gtk3 devel"
|
|
else
|
|
gtk="no"
|
|
fi
|
|
fi
|
|
|
|
|
|
##########################################
|
|
# GNUTLS probe
|
|
|
|
if test "$gnutls" != "no"; then
|
|
if $pkg_config --exists "gnutls"; then
|
|
gnutls_cflags=`$pkg_config --cflags gnutls`
|
|
gnutls_libs=`$pkg_config --libs gnutls`
|
|
libs_softmmu="$gnutls_libs $libs_softmmu"
|
|
libs_tools="$gnutls_libs $libs_tools"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $gnutls_cflags"
|
|
gnutls="yes"
|
|
|
|
# gnutls_hash_init requires >= 2.9.10
|
|
if $pkg_config --exists "gnutls >= 2.9.10"; then
|
|
gnutls_hash="yes"
|
|
else
|
|
gnutls_hash="no"
|
|
fi
|
|
elif test "$gnutls" = "yes"; then
|
|
feature_not_found "gnutls" "Install gnutls devel"
|
|
else
|
|
gnutls="no"
|
|
gnutls_hash="no"
|
|
fi
|
|
else
|
|
gnutls_hash="no"
|
|
fi
|
|
|
|
|
|
##########################################
|
|
# VTE probe
|
|
|
|
if test "$vte" != "no"; then
|
|
if test "$gtkabi" = "3.0"; then
|
|
vtepackage="vte-2.90"
|
|
vteversion="0.32.0"
|
|
else
|
|
vtepackage="vte"
|
|
vteversion="0.24.0"
|
|
fi
|
|
if $pkg_config --exists "$vtepackage >= $vteversion"; then
|
|
vte_cflags=`$pkg_config --cflags $vtepackage`
|
|
vte_libs=`$pkg_config --libs $vtepackage`
|
|
libs_softmmu="$vte_libs $libs_softmmu"
|
|
vte="yes"
|
|
elif test "$vte" = "yes"; then
|
|
if test "$gtkabi" = "3.0"; then
|
|
feature_not_found "vte" "Install libvte-2.90 devel"
|
|
else
|
|
feature_not_found "vte" "Install libvte devel"
|
|
fi
|
|
else
|
|
vte="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# SDL probe
|
|
|
|
# Look for sdl configuration program (pkg-config or sdl-config). Try
|
|
# sdl-config even without cross prefix, and favour pkg-config over sdl-config.
|
|
|
|
if test $sdlabi = "2.0"; then
|
|
sdl_config=$sdl2_config
|
|
sdlname=sdl2
|
|
sdlconfigname=sdl2_config
|
|
else
|
|
sdlname=sdl
|
|
sdlconfigname=sdl_config
|
|
fi
|
|
|
|
if test "`basename $sdl_config`" != $sdlconfigname && ! has ${sdl_config}; then
|
|
sdl_config=$sdlconfigname
|
|
fi
|
|
|
|
if $pkg_config $sdlname --exists; then
|
|
sdlconfig="$pkg_config $sdlname"
|
|
_sdlversion=`$sdlconfig --modversion 2>/dev/null | sed 's/[^0-9]//g'`
|
|
elif has ${sdl_config}; then
|
|
sdlconfig="$sdl_config"
|
|
_sdlversion=`$sdlconfig --version | sed 's/[^0-9]//g'`
|
|
else
|
|
if test "$sdl" = "yes" ; then
|
|
feature_not_found "sdl" "Install SDL devel"
|
|
fi
|
|
sdl=no
|
|
fi
|
|
if test -n "$cross_prefix" && test "$(basename "$sdlconfig")" = sdl-config; then
|
|
echo warning: using "\"$sdlconfig\"" to detect cross-compiled sdl >&2
|
|
fi
|
|
|
|
sdl_too_old=no
|
|
if test "$sdl" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <SDL.h>
|
|
#undef main /* We don't want SDL to override our main() */
|
|
int main( void ) { return SDL_Init (SDL_INIT_VIDEO); }
|
|
EOF
|
|
sdl_cflags=`$sdlconfig --cflags 2> /dev/null`
|
|
if test "$static" = "yes" ; then
|
|
sdl_libs=`$sdlconfig --static-libs 2>/dev/null`
|
|
else
|
|
sdl_libs=`$sdlconfig --libs 2> /dev/null`
|
|
fi
|
|
if compile_prog "$sdl_cflags" "$sdl_libs" ; then
|
|
if test "$_sdlversion" -lt 121 ; then
|
|
sdl_too_old=yes
|
|
else
|
|
if test "$cocoa" = "no" ; then
|
|
sdl=yes
|
|
fi
|
|
fi
|
|
|
|
# static link with sdl ? (note: sdl.pc's --static --libs is broken)
|
|
if test "$sdl" = "yes" -a "$static" = "yes" ; then
|
|
if test $? = 0 && echo $sdl_libs | grep -- -laa > /dev/null; then
|
|
sdl_libs="$sdl_libs `aalib-config --static-libs 2>/dev/null`"
|
|
sdl_cflags="$sdl_cflags `aalib-config --cflags 2>/dev/null`"
|
|
fi
|
|
if compile_prog "$sdl_cflags" "$sdl_libs" ; then
|
|
:
|
|
else
|
|
sdl=no
|
|
fi
|
|
fi # static link
|
|
else # sdl not found
|
|
if test "$sdl" = "yes" ; then
|
|
feature_not_found "sdl" "Install SDL devel"
|
|
fi
|
|
sdl=no
|
|
fi # sdl compile test
|
|
fi
|
|
|
|
if test "$sdl" = "yes" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <SDL.h>
|
|
#if defined(SDL_VIDEO_DRIVER_X11)
|
|
#include <X11/XKBlib.h>
|
|
#else
|
|
#error No x11 support
|
|
#endif
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if compile_prog "$sdl_cflags $x11_cflags" "$sdl_libs $x11_libs" ; then
|
|
sdl_cflags="$sdl_cflags $x11_cflags"
|
|
sdl_libs="$sdl_libs $x11_libs"
|
|
fi
|
|
libs_softmmu="$sdl_libs $libs_softmmu"
|
|
fi
|
|
|
|
##########################################
|
|
# RDMA needs OpenFabrics libraries
|
|
if test "$rdma" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <rdma/rdma_cma.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
rdma_libs="-lrdmacm -libverbs"
|
|
if compile_prog "" "$rdma_libs" ; then
|
|
rdma="yes"
|
|
libs_softmmu="$libs_softmmu $rdma_libs"
|
|
else
|
|
if test "$rdma" = "yes" ; then
|
|
error_exit \
|
|
" OpenFabrics librdmacm/libibverbs not present." \
|
|
" Your options:" \
|
|
" (1) Fast: Install infiniband packages from your distro." \
|
|
" (2) Cleanest: Install libraries from www.openfabrics.org" \
|
|
" (3) Also: Install softiwarp if you don't have RDMA hardware"
|
|
fi
|
|
rdma="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# VNC TLS/WS detection
|
|
if test "$vnc" = "yes" -a \( "$vnc_tls" != "no" -o "$vnc_ws" != "no" \) ; then
|
|
cat > $TMPC <<EOF
|
|
#include <gnutls/gnutls.h>
|
|
int main(void) { gnutls_session_t s; gnutls_init(&s, GNUTLS_SERVER); return 0; }
|
|
EOF
|
|
vnc_tls_cflags=`$pkg_config --cflags gnutls 2> /dev/null`
|
|
vnc_tls_libs=`$pkg_config --libs gnutls 2> /dev/null`
|
|
if compile_prog "$vnc_tls_cflags" "$vnc_tls_libs" ; then
|
|
if test "$vnc_tls" != "no" ; then
|
|
vnc_tls=yes
|
|
fi
|
|
if test "$vnc_ws" != "no" ; then
|
|
vnc_ws=yes
|
|
fi
|
|
libs_softmmu="$vnc_tls_libs $libs_softmmu"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $vnc_tls_cflags"
|
|
else
|
|
if test "$vnc_tls" = "yes" ; then
|
|
feature_not_found "vnc-tls" "Install gnutls devel"
|
|
fi
|
|
if test "$vnc_ws" = "yes" ; then
|
|
feature_not_found "vnc-ws" "Install gnutls devel"
|
|
fi
|
|
vnc_tls=no
|
|
vnc_ws=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# Quorum probe (check for gnutls)
|
|
if test "$quorum" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <gnutls/gnutls.h>
|
|
#include <gnutls/crypto.h>
|
|
int main(void) {char data[4096], digest[32];
|
|
gnutls_hash_fast(GNUTLS_DIG_SHA256, data, 4096, digest);
|
|
return 0;
|
|
}
|
|
EOF
|
|
quorum_tls_cflags=`$pkg_config --cflags gnutls 2> /dev/null`
|
|
quorum_tls_libs=`$pkg_config --libs gnutls 2> /dev/null`
|
|
if compile_prog "$quorum_tls_cflags" "$quorum_tls_libs" ; then
|
|
qcow_tls=yes
|
|
libs_softmmu="$quorum_tls_libs $libs_softmmu"
|
|
libs_tools="$quorum_tls_libs $libs_softmmu"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $quorum_tls_cflags"
|
|
quorum="yes"
|
|
else
|
|
if test "$quorum" = "yes"; then
|
|
feature_not_found "gnutls" "gnutls > 2.10.0 required to compile Quorum"
|
|
fi
|
|
quorum="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# VNC SASL detection
|
|
if test "$vnc" = "yes" -a "$vnc_sasl" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <sasl/sasl.h>
|
|
#include <stdio.h>
|
|
int main(void) { sasl_server_init(NULL, "qemu"); return 0; }
|
|
EOF
|
|
# Assuming Cyrus-SASL installed in /usr prefix
|
|
vnc_sasl_cflags=""
|
|
vnc_sasl_libs="-lsasl2"
|
|
if compile_prog "$vnc_sasl_cflags" "$vnc_sasl_libs" ; then
|
|
vnc_sasl=yes
|
|
libs_softmmu="$vnc_sasl_libs $libs_softmmu"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $vnc_sasl_cflags"
|
|
else
|
|
if test "$vnc_sasl" = "yes" ; then
|
|
feature_not_found "vnc-sasl" "Install Cyrus SASL devel"
|
|
fi
|
|
vnc_sasl=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# VNC JPEG detection
|
|
if test "$vnc" = "yes" -a "$vnc_jpeg" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <jpeglib.h>
|
|
int main(void) { struct jpeg_compress_struct s; jpeg_create_compress(&s); return 0; }
|
|
EOF
|
|
vnc_jpeg_cflags=""
|
|
vnc_jpeg_libs="-ljpeg"
|
|
if compile_prog "$vnc_jpeg_cflags" "$vnc_jpeg_libs" ; then
|
|
vnc_jpeg=yes
|
|
libs_softmmu="$vnc_jpeg_libs $libs_softmmu"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $vnc_jpeg_cflags"
|
|
else
|
|
if test "$vnc_jpeg" = "yes" ; then
|
|
feature_not_found "vnc-jpeg" "Install libjpeg-turbo devel"
|
|
fi
|
|
vnc_jpeg=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# VNC PNG detection
|
|
if test "$vnc" = "yes" -a "$vnc_png" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
//#include <stdio.h>
|
|
#include <png.h>
|
|
#include <stddef.h>
|
|
int main(void) {
|
|
png_structp png_ptr;
|
|
png_ptr = png_create_write_struct(PNG_LIBPNG_VER_STRING, NULL, NULL, NULL);
|
|
return png_ptr != 0;
|
|
}
|
|
EOF
|
|
if $pkg_config libpng --exists; then
|
|
vnc_png_cflags=`$pkg_config libpng --cflags`
|
|
vnc_png_libs=`$pkg_config libpng --libs`
|
|
else
|
|
vnc_png_cflags=""
|
|
vnc_png_libs="-lpng"
|
|
fi
|
|
if compile_prog "$vnc_png_cflags" "$vnc_png_libs" ; then
|
|
vnc_png=yes
|
|
libs_softmmu="$vnc_png_libs $libs_softmmu"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $vnc_png_cflags"
|
|
else
|
|
if test "$vnc_png" = "yes" ; then
|
|
feature_not_found "vnc-png" "Install libpng devel"
|
|
fi
|
|
vnc_png=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# fnmatch() probe, used for ACL routines
|
|
fnmatch="no"
|
|
cat > $TMPC << EOF
|
|
#include <fnmatch.h>
|
|
int main(void)
|
|
{
|
|
fnmatch("foo", "foo", 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
fnmatch="yes"
|
|
fi
|
|
|
|
##########################################
|
|
# uuid_generate() probe, used for vdi block driver
|
|
# Note that on some systems (notably MacOSX) no extra library
|
|
# need be linked to get the uuid functions.
|
|
if test "$uuid" != "no" ; then
|
|
uuid_libs="-luuid"
|
|
cat > $TMPC << EOF
|
|
#include <uuid/uuid.h>
|
|
int main(void)
|
|
{
|
|
uuid_t my_uuid;
|
|
uuid_generate(my_uuid);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
uuid="yes"
|
|
elif compile_prog "" "$uuid_libs" ; then
|
|
uuid="yes"
|
|
libs_softmmu="$uuid_libs $libs_softmmu"
|
|
libs_tools="$uuid_libs $libs_tools"
|
|
else
|
|
if test "$uuid" = "yes" ; then
|
|
feature_not_found "uuid" "Install libuuid devel"
|
|
fi
|
|
uuid=no
|
|
fi
|
|
fi
|
|
|
|
if test "$vhdx" = "yes" ; then
|
|
if test "$uuid" = "no" ; then
|
|
error_exit "uuid required for VHDX support"
|
|
fi
|
|
elif test "$vhdx" != "no" ; then
|
|
if test "$uuid" = "yes" ; then
|
|
vhdx=yes
|
|
else
|
|
vhdx=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# xfsctl() probe, used for raw-posix
|
|
if test "$xfs" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <stddef.h> /* NULL */
|
|
#include <xfs/xfs.h>
|
|
int main(void)
|
|
{
|
|
xfsctl(NULL, 0, 0, NULL);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
xfs="yes"
|
|
else
|
|
if test "$xfs" = "yes" ; then
|
|
feature_not_found "xfs" "Instal xfsprogs/xfslibs devel"
|
|
fi
|
|
xfs=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# vde libraries probe
|
|
if test "$vde" != "no" ; then
|
|
vde_libs="-lvdeplug"
|
|
cat > $TMPC << EOF
|
|
#include <libvdeplug.h>
|
|
int main(void)
|
|
{
|
|
struct vde_open_args a = {0, 0, 0};
|
|
char s[] = "";
|
|
vde_open(s, s, &a);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "$vde_libs" ; then
|
|
vde=yes
|
|
libs_softmmu="$vde_libs $libs_softmmu"
|
|
libs_tools="$vde_libs $libs_tools"
|
|
else
|
|
if test "$vde" = "yes" ; then
|
|
feature_not_found "vde" "Install vde (Virtual Distributed Ethernet) devel"
|
|
fi
|
|
vde=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# netmap support probe
|
|
# Apart from looking for netmap headers, we make sure that the host API version
|
|
# supports the netmap backend (>=11). The upper bound (15) is meant to simulate
|
|
# a minor/major version number. Minor new features will be marked with values up
|
|
# to 15, and if something happens that requires a change to the backend we will
|
|
# move above 15, submit the backend fixes and modify this two bounds.
|
|
if test "$netmap" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <inttypes.h>
|
|
#include <net/if.h>
|
|
#include <net/netmap.h>
|
|
#include <net/netmap_user.h>
|
|
#if (NETMAP_API < 11) || (NETMAP_API > 15)
|
|
#error
|
|
#endif
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
netmap=yes
|
|
else
|
|
if test "$netmap" = "yes" ; then
|
|
feature_not_found "netmap"
|
|
fi
|
|
netmap=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# libcap-ng library probe
|
|
if test "$cap_ng" != "no" ; then
|
|
cap_libs="-lcap-ng"
|
|
cat > $TMPC << EOF
|
|
#include <cap-ng.h>
|
|
int main(void)
|
|
{
|
|
capng_capability_to_name(CAPNG_EFFECTIVE);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "$cap_libs" ; then
|
|
cap_ng=yes
|
|
libs_tools="$cap_libs $libs_tools"
|
|
else
|
|
if test "$cap_ng" = "yes" ; then
|
|
feature_not_found "cap_ng" "Install libcap-ng devel"
|
|
fi
|
|
cap_ng=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# Sound support libraries probe
|
|
|
|
audio_drv_probe()
|
|
{
|
|
drv=$1
|
|
hdr=$2
|
|
lib=$3
|
|
exp=$4
|
|
cfl=$5
|
|
cat > $TMPC << EOF
|
|
#include <$hdr>
|
|
int main(void) { $exp }
|
|
EOF
|
|
if compile_prog "$cfl" "$lib" ; then
|
|
:
|
|
else
|
|
error_exit "$drv check failed" \
|
|
"Make sure to have the $drv libs and headers installed."
|
|
fi
|
|
}
|
|
|
|
audio_drv_list=`echo "$audio_drv_list" | sed -e 's/,/ /g'`
|
|
for drv in $audio_drv_list; do
|
|
case $drv in
|
|
alsa)
|
|
audio_drv_probe $drv alsa/asoundlib.h -lasound \
|
|
"return snd_pcm_close((snd_pcm_t *)0);"
|
|
libs_softmmu="-lasound $libs_softmmu"
|
|
;;
|
|
|
|
pa)
|
|
audio_drv_probe $drv pulse/mainloop.h "-lpulse" \
|
|
"pa_mainloop *m = 0; pa_mainloop_free (m); return 0;"
|
|
libs_softmmu="-lpulse $libs_softmmu"
|
|
audio_pt_int="yes"
|
|
;;
|
|
|
|
coreaudio)
|
|
libs_softmmu="-framework CoreAudio $libs_softmmu"
|
|
;;
|
|
|
|
dsound)
|
|
libs_softmmu="-lole32 -ldxguid $libs_softmmu"
|
|
audio_win_int="yes"
|
|
;;
|
|
|
|
oss)
|
|
libs_softmmu="$oss_lib $libs_softmmu"
|
|
;;
|
|
|
|
sdl|wav)
|
|
# XXX: Probes for CoreAudio, DirectSound, SDL(?)
|
|
;;
|
|
|
|
*)
|
|
echo "$audio_possible_drivers" | grep -q "\<$drv\>" || {
|
|
error_exit "Unknown driver '$drv' selected" \
|
|
"Possible drivers are: $audio_possible_drivers"
|
|
}
|
|
;;
|
|
esac
|
|
done
|
|
|
|
##########################################
|
|
# BrlAPI probe
|
|
|
|
if test "$brlapi" != "no" ; then
|
|
brlapi_libs="-lbrlapi"
|
|
cat > $TMPC << EOF
|
|
#include <brlapi.h>
|
|
#include <stddef.h>
|
|
int main( void ) { return brlapi__openConnection (NULL, NULL, NULL); }
|
|
EOF
|
|
if compile_prog "" "$brlapi_libs" ; then
|
|
brlapi=yes
|
|
libs_softmmu="$brlapi_libs $libs_softmmu"
|
|
else
|
|
if test "$brlapi" = "yes" ; then
|
|
feature_not_found "brlapi" "Install brlapi devel"
|
|
fi
|
|
brlapi=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# curses probe
|
|
if test "$curses" != "no" ; then
|
|
if test "$mingw32" = "yes" ; then
|
|
curses_list="-lpdcurses"
|
|
else
|
|
curses_list="$($pkg_config --libs ncurses 2>/dev/null):-lncurses:-lcurses"
|
|
fi
|
|
curses_found=no
|
|
cat > $TMPC << EOF
|
|
#include <curses.h>
|
|
int main(void) {
|
|
const char *s = curses_version();
|
|
resize_term(0, 0);
|
|
return s != 0;
|
|
}
|
|
EOF
|
|
IFS=:
|
|
for curses_lib in $curses_list; do
|
|
unset IFS
|
|
if compile_prog "" "$curses_lib" ; then
|
|
curses_found=yes
|
|
libs_softmmu="$curses_lib $libs_softmmu"
|
|
break
|
|
fi
|
|
done
|
|
unset IFS
|
|
if test "$curses_found" = "yes" ; then
|
|
curses=yes
|
|
else
|
|
if test "$curses" = "yes" ; then
|
|
feature_not_found "curses" "Install ncurses devel"
|
|
fi
|
|
curses=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# curl probe
|
|
if test "$curl" != "no" ; then
|
|
if $pkg_config libcurl --exists; then
|
|
curlconfig="$pkg_config libcurl"
|
|
else
|
|
curlconfig=curl-config
|
|
fi
|
|
cat > $TMPC << EOF
|
|
#include <curl/curl.h>
|
|
int main(void) { curl_easy_init(); curl_multi_setopt(0, 0, 0); return 0; }
|
|
EOF
|
|
curl_cflags=`$curlconfig --cflags 2>/dev/null`
|
|
curl_libs=`$curlconfig --libs 2>/dev/null`
|
|
if compile_prog "$curl_cflags" "$curl_libs" ; then
|
|
curl=yes
|
|
else
|
|
if test "$curl" = "yes" ; then
|
|
feature_not_found "curl" "Install libcurl devel"
|
|
fi
|
|
curl=no
|
|
fi
|
|
fi # test "$curl"
|
|
|
|
##########################################
|
|
# bluez support probe
|
|
if test "$bluez" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <bluetooth/bluetooth.h>
|
|
int main(void) { return bt_error(0); }
|
|
EOF
|
|
bluez_cflags=`$pkg_config --cflags bluez 2> /dev/null`
|
|
bluez_libs=`$pkg_config --libs bluez 2> /dev/null`
|
|
if compile_prog "$bluez_cflags" "$bluez_libs" ; then
|
|
bluez=yes
|
|
libs_softmmu="$bluez_libs $libs_softmmu"
|
|
else
|
|
if test "$bluez" = "yes" ; then
|
|
feature_not_found "bluez" "Install bluez-libs/libbluetooth devel"
|
|
fi
|
|
bluez="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# glib support probe
|
|
|
|
glib_req_ver=2.22
|
|
glib_modules=gthread-2.0
|
|
if test "$modules" = yes; then
|
|
glib_modules="$glib_modules gmodule-2.0"
|
|
fi
|
|
|
|
for i in $glib_modules; do
|
|
if $pkg_config --atleast-version=$glib_req_ver $i; then
|
|
glib_cflags=`$pkg_config --cflags $i`
|
|
glib_libs=`$pkg_config --libs $i`
|
|
CFLAGS="$glib_cflags $CFLAGS"
|
|
LIBS="$glib_libs $LIBS"
|
|
libs_qga="$glib_libs $libs_qga"
|
|
else
|
|
error_exit "glib-$glib_req_ver $i is required to compile QEMU"
|
|
fi
|
|
done
|
|
|
|
# g_test_trap_subprocess added in 2.38. Used by some tests.
|
|
glib_subprocess=yes
|
|
if ! $pkg_config --atleast-version=2.38 glib-2.0; then
|
|
glib_subprocess=no
|
|
fi
|
|
|
|
# Silence clang 3.5.0 warnings about glib attribute __alloc_size__ usage
|
|
cat > $TMPC << EOF
|
|
#include <glib.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if ! compile_prog "$glib_cflags -Werror" "$glib_libs" ; then
|
|
if cc_has_warning_flag "-Wno-unknown-attributes"; then
|
|
glib_cflags="-Wno-unknown-attributes $glib_cflags"
|
|
CFLAGS="-Wno-unknown-attributes $CFLAGS"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# SHA command probe for modules
|
|
if test "$modules" = yes; then
|
|
shacmd_probe="sha1sum sha1 shasum"
|
|
for c in $shacmd_probe; do
|
|
if has $c; then
|
|
shacmd="$c"
|
|
break
|
|
fi
|
|
done
|
|
if test "$shacmd" = ""; then
|
|
error_exit "one of the checksum commands is required to enable modules: $shacmd_probe"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# pixman support probe
|
|
|
|
if test "$pixman" = ""; then
|
|
if test "$want_tools" = "no" -a "$softmmu" = "no"; then
|
|
pixman="none"
|
|
elif $pkg_config --atleast-version=0.21.8 pixman-1 > /dev/null 2>&1; then
|
|
pixman="system"
|
|
else
|
|
pixman="internal"
|
|
fi
|
|
fi
|
|
if test "$pixman" = "none"; then
|
|
if test "$want_tools" != "no" -o "$softmmu" != "no"; then
|
|
error_exit "pixman disabled but system emulation or tools build" \
|
|
"enabled. You can turn off pixman only if you also" \
|
|
"disable all system emulation targets and the tools" \
|
|
"build with '--disable-tools --disable-system'."
|
|
fi
|
|
pixman_cflags=
|
|
pixman_libs=
|
|
elif test "$pixman" = "system"; then
|
|
# pixman version has been checked above
|
|
pixman_cflags=`$pkg_config --cflags pixman-1`
|
|
pixman_libs=`$pkg_config --libs pixman-1`
|
|
else
|
|
if test ! -d ${source_path}/pixman/pixman; then
|
|
error_exit "pixman >= 0.21.8 not present. Your options:" \
|
|
" (1) Preferred: Install the pixman devel package (any recent" \
|
|
" distro should have packages as Xorg needs pixman too)." \
|
|
" (2) Fetch the pixman submodule, using:" \
|
|
" git submodule update --init pixman"
|
|
fi
|
|
mkdir -p pixman/pixman
|
|
pixman_cflags="-I\$(SRC_PATH)/pixman/pixman -I\$(BUILD_DIR)/pixman/pixman"
|
|
pixman_libs="-L\$(BUILD_DIR)/pixman/pixman/.libs -lpixman-1"
|
|
fi
|
|
|
|
##########################################
|
|
# libcap probe
|
|
|
|
if test "$cap" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <sys/capability.h>
|
|
int main(void) { cap_t caps; caps = cap_init(); return caps != NULL; }
|
|
EOF
|
|
if compile_prog "" "-lcap" ; then
|
|
cap=yes
|
|
else
|
|
cap=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# pthread probe
|
|
PTHREADLIBS_LIST="-pthread -lpthread -lpthreadGC2"
|
|
|
|
pthread=no
|
|
cat > $TMPC << EOF
|
|
#include <pthread.h>
|
|
static void *f(void *p) { return NULL; }
|
|
int main(void) {
|
|
pthread_t thread;
|
|
pthread_create(&thread, 0, f, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
pthread=yes
|
|
else
|
|
for pthread_lib in $PTHREADLIBS_LIST; do
|
|
if compile_prog "" "$pthread_lib" ; then
|
|
pthread=yes
|
|
found=no
|
|
for lib_entry in $LIBS; do
|
|
if test "$lib_entry" = "$pthread_lib"; then
|
|
found=yes
|
|
break
|
|
fi
|
|
done
|
|
if test "$found" = "no"; then
|
|
LIBS="$pthread_lib $LIBS"
|
|
fi
|
|
break
|
|
fi
|
|
done
|
|
fi
|
|
|
|
if test "$mingw32" != yes -a "$pthread" = no; then
|
|
error_exit "pthread check failed" \
|
|
"Make sure to have the pthread libs and headers installed."
|
|
fi
|
|
|
|
# check for pthread_setname_np
|
|
pthread_setname_np=no
|
|
cat > $TMPC << EOF
|
|
#include <pthread.h>
|
|
|
|
static void *f(void *p) { return NULL; }
|
|
int main(void)
|
|
{
|
|
pthread_t thread;
|
|
pthread_create(&thread, 0, f, 0);
|
|
pthread_setname_np(thread, "QEMU");
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "$pthread_lib" ; then
|
|
pthread_setname_np=yes
|
|
fi
|
|
|
|
##########################################
|
|
# rbd probe
|
|
if test "$rbd" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <rbd/librbd.h>
|
|
int main(void) {
|
|
rados_t cluster;
|
|
rados_create(&cluster, NULL);
|
|
return 0;
|
|
}
|
|
EOF
|
|
rbd_libs="-lrbd -lrados"
|
|
if compile_prog "" "$rbd_libs" ; then
|
|
rbd=yes
|
|
else
|
|
if test "$rbd" = "yes" ; then
|
|
feature_not_found "rados block device" "Install librbd/ceph devel"
|
|
fi
|
|
rbd=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# libssh2 probe
|
|
min_libssh2_version=1.2.8
|
|
if test "$libssh2" != "no" ; then
|
|
if $pkg_config --atleast-version=$min_libssh2_version libssh2; then
|
|
libssh2_cflags=`$pkg_config libssh2 --cflags`
|
|
libssh2_libs=`$pkg_config libssh2 --libs`
|
|
libssh2=yes
|
|
else
|
|
if test "$libssh2" = "yes" ; then
|
|
error_exit "libssh2 >= $min_libssh2_version required for --enable-libssh2"
|
|
fi
|
|
libssh2=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# libssh2_sftp_fsync probe
|
|
|
|
if test "$libssh2" = "yes"; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <libssh2.h>
|
|
#include <libssh2_sftp.h>
|
|
int main(void) {
|
|
LIBSSH2_SESSION *session;
|
|
LIBSSH2_SFTP *sftp;
|
|
LIBSSH2_SFTP_HANDLE *sftp_handle;
|
|
session = libssh2_session_init ();
|
|
sftp = libssh2_sftp_init (session);
|
|
sftp_handle = libssh2_sftp_open (sftp, "/", 0, 0);
|
|
libssh2_sftp_fsync (sftp_handle);
|
|
return 0;
|
|
}
|
|
EOF
|
|
# libssh2_cflags/libssh2_libs defined in previous test.
|
|
if compile_prog "$libssh2_cflags" "$libssh2_libs" ; then
|
|
QEMU_CFLAGS="-DHAS_LIBSSH2_SFTP_FSYNC $QEMU_CFLAGS"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# linux-aio probe
|
|
|
|
if test "$linux_aio" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <libaio.h>
|
|
#include <sys/eventfd.h>
|
|
#include <stddef.h>
|
|
int main(void) { io_setup(0, NULL); io_set_eventfd(NULL, 0); eventfd(0, 0); return 0; }
|
|
EOF
|
|
if compile_prog "" "-laio" ; then
|
|
linux_aio=yes
|
|
else
|
|
if test "$linux_aio" = "yes" ; then
|
|
feature_not_found "linux AIO" "Install libaio devel"
|
|
fi
|
|
linux_aio=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# TPM passthrough is only on x86 Linux
|
|
|
|
if test "$targetos" = Linux && test "$cpu" = i386 -o "$cpu" = x86_64; then
|
|
tpm_passthrough=$tpm
|
|
else
|
|
tpm_passthrough=no
|
|
fi
|
|
|
|
##########################################
|
|
# attr probe
|
|
|
|
if test "$attr" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <sys/types.h>
|
|
#ifdef CONFIG_LIBATTR
|
|
#include <attr/xattr.h>
|
|
#else
|
|
#include <sys/xattr.h>
|
|
#endif
|
|
int main(void) { getxattr(NULL, NULL, NULL, 0); setxattr(NULL, NULL, NULL, 0, 0); return 0; }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
attr=yes
|
|
# Older distros have <attr/xattr.h>, and need -lattr:
|
|
elif compile_prog "-DCONFIG_LIBATTR" "-lattr" ; then
|
|
attr=yes
|
|
LIBS="-lattr $LIBS"
|
|
libattr=yes
|
|
else
|
|
if test "$attr" = "yes" ; then
|
|
feature_not_found "ATTR" "Install libc6 or libattr devel"
|
|
fi
|
|
attr=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# iovec probe
|
|
cat > $TMPC <<EOF
|
|
#include <sys/types.h>
|
|
#include <sys/uio.h>
|
|
#include <unistd.h>
|
|
int main(void) { return sizeof(struct iovec); }
|
|
EOF
|
|
iovec=no
|
|
if compile_prog "" "" ; then
|
|
iovec=yes
|
|
fi
|
|
|
|
##########################################
|
|
# preadv probe
|
|
cat > $TMPC <<EOF
|
|
#include <sys/types.h>
|
|
#include <sys/uio.h>
|
|
#include <unistd.h>
|
|
int main(void) { return preadv(0, 0, 0, 0); }
|
|
EOF
|
|
preadv=no
|
|
if compile_prog "" "" ; then
|
|
preadv=yes
|
|
fi
|
|
|
|
##########################################
|
|
# fdt probe
|
|
# fdt support is mandatory for at least some target architectures,
|
|
# so insist on it if we're building those system emulators.
|
|
fdt_required=no
|
|
for target in $target_list; do
|
|
case $target in
|
|
aarch64*-softmmu|arm*-softmmu|ppc*-softmmu|microblaze*-softmmu)
|
|
fdt_required=yes
|
|
;;
|
|
esac
|
|
done
|
|
|
|
if test "$fdt_required" = "yes"; then
|
|
if test "$fdt" = "no"; then
|
|
error_exit "fdt disabled but some requested targets require it." \
|
|
"You can turn off fdt only if you also disable all the system emulation" \
|
|
"targets which need it (by specifying a cut down --target-list)."
|
|
fi
|
|
fdt=yes
|
|
fi
|
|
|
|
if test "$fdt" != "no" ; then
|
|
fdt_libs="-lfdt"
|
|
# explicitly check for libfdt_env.h as it is missing in some stable installs
|
|
# and test for required functions to make sure we are on a version >= 1.4.0
|
|
cat > $TMPC << EOF
|
|
#include <libfdt.h>
|
|
#include <libfdt_env.h>
|
|
int main(void) { fdt_get_property_by_offset(0, 0, 0); return 0; }
|
|
EOF
|
|
if compile_prog "" "$fdt_libs" ; then
|
|
# system DTC is good - use it
|
|
fdt=yes
|
|
elif test -d ${source_path}/dtc/libfdt ; then
|
|
# have submodule DTC - use it
|
|
fdt=yes
|
|
dtc_internal="yes"
|
|
mkdir -p dtc
|
|
if [ "$pwd_is_source_path" != "y" ] ; then
|
|
symlink "$source_path/dtc/Makefile" "dtc/Makefile"
|
|
symlink "$source_path/dtc/scripts" "dtc/scripts"
|
|
fi
|
|
fdt_cflags="-I\$(SRC_PATH)/dtc/libfdt"
|
|
fdt_libs="-L\$(BUILD_DIR)/dtc/libfdt $fdt_libs"
|
|
elif test "$fdt" = "yes" ; then
|
|
# have neither and want - prompt for system/submodule install
|
|
error_exit "DTC (libfdt) version >= 1.4.0 not present. Your options:" \
|
|
" (1) Preferred: Install the DTC (libfdt) devel package" \
|
|
" (2) Fetch the DTC submodule, using:" \
|
|
" git submodule update --init dtc"
|
|
else
|
|
# don't have and don't want
|
|
fdt_libs=
|
|
fdt=no
|
|
fi
|
|
fi
|
|
|
|
libs_softmmu="$libs_softmmu $fdt_libs"
|
|
|
|
##########################################
|
|
# opengl probe (for sdl2, milkymist-tmu2)
|
|
|
|
# GLX probe, used by milkymist-tmu2
|
|
# this is temporary, code will be switched to egl mid-term.
|
|
cat > $TMPC << EOF
|
|
#include <X11/Xlib.h>
|
|
#include <GL/gl.h>
|
|
#include <GL/glx.h>
|
|
int main(void) { glBegin(0); glXQueryVersion(0,0,0); return 0; }
|
|
EOF
|
|
if compile_prog "" "-lGL -lX11" ; then
|
|
have_glx=yes
|
|
else
|
|
have_glx=no
|
|
fi
|
|
|
|
if test "$opengl" != "no" ; then
|
|
opengl_pkgs="gl glesv2 epoxy egl"
|
|
if $pkg_config $opengl_pkgs x11 && test "$have_glx" = "yes"; then
|
|
opengl_cflags="$($pkg_config --cflags $opengl_pkgs) $x11_cflags"
|
|
opengl_libs="$($pkg_config --libs $opengl_pkgs) $x11_libs"
|
|
opengl=yes
|
|
else
|
|
if test "$opengl" = "yes" ; then
|
|
feature_not_found "opengl" "Please install opengl (mesa) devel pkgs: $opengl_pkgs"
|
|
fi
|
|
opengl_cflags=""
|
|
opengl_libs=""
|
|
opengl=no
|
|
fi
|
|
fi
|
|
|
|
|
|
##########################################
|
|
# archipelago probe
|
|
if test "$archipelago" != "no" ; then
|
|
cat > $TMPC <<EOF
|
|
#include <stdio.h>
|
|
#include <xseg/xseg.h>
|
|
#include <xseg/protocol.h>
|
|
int main(void) {
|
|
xseg_initialize();
|
|
return 0;
|
|
}
|
|
EOF
|
|
archipelago_libs=-lxseg
|
|
if compile_prog "" "$archipelago_libs"; then
|
|
archipelago="yes"
|
|
libs_tools="$archipelago_libs $libs_tools"
|
|
libs_softmmu="$archipelago_libs $libs_softmmu"
|
|
|
|
echo "WARNING: Please check the licenses of QEMU and libxseg carefully."
|
|
echo "GPLv3 versions of libxseg may not be compatible with QEMU's"
|
|
echo "license and therefore prevent redistribution."
|
|
echo
|
|
echo "To disable Archipelago, use --disable-archipelago"
|
|
else
|
|
if test "$archipelago" = "yes" ; then
|
|
feature_not_found "Archipelago backend support" "Install libxseg devel"
|
|
fi
|
|
archipelago="no"
|
|
fi
|
|
fi
|
|
|
|
|
|
##########################################
|
|
# glusterfs probe
|
|
if test "$glusterfs" != "no" ; then
|
|
if $pkg_config --atleast-version=3 glusterfs-api; then
|
|
glusterfs="yes"
|
|
glusterfs_cflags=`$pkg_config --cflags glusterfs-api`
|
|
glusterfs_libs=`$pkg_config --libs glusterfs-api`
|
|
if $pkg_config --atleast-version=5 glusterfs-api; then
|
|
glusterfs_discard="yes"
|
|
fi
|
|
if $pkg_config --atleast-version=6 glusterfs-api; then
|
|
glusterfs_zerofill="yes"
|
|
fi
|
|
else
|
|
if test "$glusterfs" = "yes" ; then
|
|
feature_not_found "GlusterFS backend support" \
|
|
"Install glusterfs-api devel >= 3"
|
|
fi
|
|
glusterfs="no"
|
|
fi
|
|
fi
|
|
|
|
# Check for inotify functions when we are building linux-user
|
|
# emulator. This is done because older glibc versions don't
|
|
# have syscall stubs for these implemented. In that case we
|
|
# don't provide them even if kernel supports them.
|
|
#
|
|
inotify=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/inotify.h>
|
|
|
|
int
|
|
main(void)
|
|
{
|
|
/* try to start inotify */
|
|
return inotify_init();
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
inotify=yes
|
|
fi
|
|
|
|
inotify1=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/inotify.h>
|
|
|
|
int
|
|
main(void)
|
|
{
|
|
/* try to start inotify */
|
|
return inotify_init1(0);
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
inotify1=yes
|
|
fi
|
|
|
|
# check if utimensat and futimens are supported
|
|
utimens=no
|
|
cat > $TMPC << EOF
|
|
#define _ATFILE_SOURCE
|
|
#include <stddef.h>
|
|
#include <fcntl.h>
|
|
#include <sys/stat.h>
|
|
|
|
int main(void)
|
|
{
|
|
utimensat(AT_FDCWD, "foo", NULL, 0);
|
|
futimens(0, NULL);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
utimens=yes
|
|
fi
|
|
|
|
# check if pipe2 is there
|
|
pipe2=no
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
#include <fcntl.h>
|
|
|
|
int main(void)
|
|
{
|
|
int pipefd[2];
|
|
return pipe2(pipefd, O_CLOEXEC);
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
pipe2=yes
|
|
fi
|
|
|
|
# check if accept4 is there
|
|
accept4=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/socket.h>
|
|
#include <stddef.h>
|
|
|
|
int main(void)
|
|
{
|
|
accept4(0, NULL, NULL, SOCK_CLOEXEC);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
accept4=yes
|
|
fi
|
|
|
|
# check if tee/splice is there. vmsplice was added same time.
|
|
splice=no
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
#include <fcntl.h>
|
|
#include <limits.h>
|
|
|
|
int main(void)
|
|
{
|
|
int len, fd = 0;
|
|
len = tee(STDIN_FILENO, STDOUT_FILENO, INT_MAX, SPLICE_F_NONBLOCK);
|
|
splice(STDIN_FILENO, NULL, fd, NULL, len, SPLICE_F_MOVE);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
splice=yes
|
|
fi
|
|
|
|
##########################################
|
|
# libnuma probe
|
|
|
|
if test "$numa" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <numa.h>
|
|
int main(void) { return numa_available(); }
|
|
EOF
|
|
|
|
if compile_prog "" "-lnuma" ; then
|
|
numa=yes
|
|
libs_softmmu="-lnuma $libs_softmmu"
|
|
else
|
|
if test "$numa" = "yes" ; then
|
|
feature_not_found "numa" "install numactl devel"
|
|
fi
|
|
numa=no
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# tcmalloc probe
|
|
|
|
if test "$tcmalloc" = "yes" ; then
|
|
cat > $TMPC << EOF
|
|
#include <stdlib.h>
|
|
int main(void) { malloc(1); return 0; }
|
|
EOF
|
|
|
|
if compile_prog "" "-ltcmalloc" ; then
|
|
LIBS="-ltcmalloc $LIBS"
|
|
else
|
|
feature_not_found "tcmalloc" "install gperftools devel"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# signalfd probe
|
|
signalfd="no"
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
#include <sys/syscall.h>
|
|
#include <signal.h>
|
|
int main(void) { return syscall(SYS_signalfd, -1, NULL, _NSIG / 8); }
|
|
EOF
|
|
|
|
if compile_prog "" "" ; then
|
|
signalfd=yes
|
|
fi
|
|
|
|
# check if eventfd is supported
|
|
eventfd=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/eventfd.h>
|
|
|
|
int main(void)
|
|
{
|
|
return eventfd(0, EFD_NONBLOCK | EFD_CLOEXEC);
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
eventfd=yes
|
|
fi
|
|
|
|
# check for fallocate
|
|
fallocate=no
|
|
cat > $TMPC << EOF
|
|
#include <fcntl.h>
|
|
|
|
int main(void)
|
|
{
|
|
fallocate(0, 0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
fallocate=yes
|
|
fi
|
|
|
|
# check for fallocate hole punching
|
|
fallocate_punch_hole=no
|
|
cat > $TMPC << EOF
|
|
#include <fcntl.h>
|
|
#include <linux/falloc.h>
|
|
|
|
int main(void)
|
|
{
|
|
fallocate(0, FALLOC_FL_PUNCH_HOLE | FALLOC_FL_KEEP_SIZE, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
fallocate_punch_hole=yes
|
|
fi
|
|
|
|
# check that fallocate supports range zeroing inside the file
|
|
fallocate_zero_range=no
|
|
cat > $TMPC << EOF
|
|
#include <fcntl.h>
|
|
#include <linux/falloc.h>
|
|
|
|
int main(void)
|
|
{
|
|
fallocate(0, FALLOC_FL_ZERO_RANGE, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
fallocate_zero_range=yes
|
|
fi
|
|
|
|
# check for posix_fallocate
|
|
posix_fallocate=no
|
|
cat > $TMPC << EOF
|
|
#include <fcntl.h>
|
|
|
|
int main(void)
|
|
{
|
|
posix_fallocate(0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
posix_fallocate=yes
|
|
fi
|
|
|
|
# check for sync_file_range
|
|
sync_file_range=no
|
|
cat > $TMPC << EOF
|
|
#include <fcntl.h>
|
|
|
|
int main(void)
|
|
{
|
|
sync_file_range(0, 0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
sync_file_range=yes
|
|
fi
|
|
|
|
# check for linux/fiemap.h and FS_IOC_FIEMAP
|
|
fiemap=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/ioctl.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/fiemap.h>
|
|
|
|
int main(void)
|
|
{
|
|
ioctl(0, FS_IOC_FIEMAP, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
fiemap=yes
|
|
fi
|
|
|
|
# check for dup3
|
|
dup3=no
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
|
|
int main(void)
|
|
{
|
|
dup3(0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
dup3=yes
|
|
fi
|
|
|
|
# check for ppoll support
|
|
ppoll=no
|
|
cat > $TMPC << EOF
|
|
#include <poll.h>
|
|
|
|
int main(void)
|
|
{
|
|
struct pollfd pfd = { .fd = 0, .events = 0, .revents = 0 };
|
|
ppoll(&pfd, 1, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
ppoll=yes
|
|
fi
|
|
|
|
# check for prctl(PR_SET_TIMERSLACK , ... ) support
|
|
prctl_pr_set_timerslack=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/prctl.h>
|
|
|
|
int main(void)
|
|
{
|
|
prctl(PR_SET_TIMERSLACK, 1, 0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
prctl_pr_set_timerslack=yes
|
|
fi
|
|
|
|
# check for epoll support
|
|
epoll=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/epoll.h>
|
|
|
|
int main(void)
|
|
{
|
|
epoll_create(0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
epoll=yes
|
|
fi
|
|
|
|
# epoll_create1 and epoll_pwait are later additions
|
|
# so we must check separately for their presence
|
|
epoll_create1=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/epoll.h>
|
|
|
|
int main(void)
|
|
{
|
|
/* Note that we use epoll_create1 as a value, not as
|
|
* a function being called. This is necessary so that on
|
|
* old SPARC glibc versions where the function was present in
|
|
* the library but not declared in the header file we will
|
|
* fail the configure check. (Otherwise we will get a compiler
|
|
* warning but not an error, and will proceed to fail the
|
|
* qemu compile where we compile with -Werror.)
|
|
*/
|
|
return (int)(uintptr_t)&epoll_create1;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
epoll_create1=yes
|
|
fi
|
|
|
|
epoll_pwait=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/epoll.h>
|
|
|
|
int main(void)
|
|
{
|
|
epoll_pwait(0, 0, 0, 0, 0);
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
epoll_pwait=yes
|
|
fi
|
|
|
|
# check for sendfile support
|
|
sendfile=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/sendfile.h>
|
|
|
|
int main(void)
|
|
{
|
|
return sendfile(0, 0, 0, 0);
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
sendfile=yes
|
|
fi
|
|
|
|
# check for timerfd support (glibc 2.8 and newer)
|
|
timerfd=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/timerfd.h>
|
|
|
|
int main(void)
|
|
{
|
|
return(timerfd_create(CLOCK_REALTIME, 0));
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
timerfd=yes
|
|
fi
|
|
|
|
# check for setns and unshare support
|
|
setns=no
|
|
cat > $TMPC << EOF
|
|
#include <sched.h>
|
|
|
|
int main(void)
|
|
{
|
|
int ret;
|
|
ret = setns(0, 0);
|
|
ret = unshare(0);
|
|
return ret;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
setns=yes
|
|
fi
|
|
|
|
# Check if tools are available to build documentation.
|
|
if test "$docs" != "no" ; then
|
|
if has makeinfo && has pod2man; then
|
|
docs=yes
|
|
else
|
|
if test "$docs" = "yes" ; then
|
|
feature_not_found "docs" "Install texinfo and Perl/perl-podlators"
|
|
fi
|
|
docs=no
|
|
fi
|
|
fi
|
|
|
|
# Search for bswap_32 function
|
|
byteswap_h=no
|
|
cat > $TMPC << EOF
|
|
#include <byteswap.h>
|
|
int main(void) { return bswap_32(0); }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
byteswap_h=yes
|
|
fi
|
|
|
|
# Search for bswap32 function
|
|
bswap_h=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/endian.h>
|
|
#include <sys/types.h>
|
|
#include <machine/bswap.h>
|
|
int main(void) { return bswap32(0); }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
bswap_h=yes
|
|
fi
|
|
|
|
##########################################
|
|
# Do we have libiscsi >= 1.9.0
|
|
if test "$libiscsi" != "no" ; then
|
|
if $pkg_config --atleast-version=1.9.0 libiscsi; then
|
|
libiscsi="yes"
|
|
libiscsi_cflags=$($pkg_config --cflags libiscsi)
|
|
libiscsi_libs=$($pkg_config --libs libiscsi)
|
|
else
|
|
if test "$libiscsi" = "yes" ; then
|
|
feature_not_found "libiscsi" "Install libiscsi >= 1.9.0"
|
|
fi
|
|
libiscsi="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# Do we need libm
|
|
cat > $TMPC << EOF
|
|
#include <math.h>
|
|
int main(int argc, char **argv) { return isnan(sin((double)argc)); }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
:
|
|
elif compile_prog "" "-lm" ; then
|
|
LIBS="-lm $LIBS"
|
|
libs_qga="-lm $libs_qga"
|
|
else
|
|
error_exit "libm check failed"
|
|
fi
|
|
|
|
##########################################
|
|
# Do we need librt
|
|
# uClibc provides 2 versions of clock_gettime(), one with realtime
|
|
# support and one without. This means that the clock_gettime() don't
|
|
# need -lrt. We still need it for timer_create() so we check for this
|
|
# function in addition.
|
|
cat > $TMPC <<EOF
|
|
#include <signal.h>
|
|
#include <time.h>
|
|
int main(void) {
|
|
timer_create(CLOCK_REALTIME, NULL, NULL);
|
|
return clock_gettime(CLOCK_REALTIME, NULL);
|
|
}
|
|
EOF
|
|
|
|
if compile_prog "" "" ; then
|
|
:
|
|
# we need pthread for static linking. use previous pthread test result
|
|
elif compile_prog "" "$pthread_lib -lrt" ; then
|
|
LIBS="$LIBS -lrt"
|
|
libs_qga="$libs_qga -lrt"
|
|
fi
|
|
|
|
if test "$darwin" != "yes" -a "$mingw32" != "yes" -a "$solaris" != yes -a \
|
|
"$aix" != "yes" -a "$haiku" != "yes" ; then
|
|
libs_softmmu="-lutil $libs_softmmu"
|
|
fi
|
|
|
|
##########################################
|
|
# spice probe
|
|
if test "$spice" != "no" ; then
|
|
cat > $TMPC << EOF
|
|
#include <spice.h>
|
|
int main(void) { spice_server_new(); return 0; }
|
|
EOF
|
|
spice_cflags=$($pkg_config --cflags spice-protocol spice-server 2>/dev/null)
|
|
spice_libs=$($pkg_config --libs spice-protocol spice-server 2>/dev/null)
|
|
if $pkg_config --atleast-version=0.12.0 spice-server && \
|
|
$pkg_config --atleast-version=0.12.3 spice-protocol && \
|
|
compile_prog "$spice_cflags" "$spice_libs" ; then
|
|
spice="yes"
|
|
libs_softmmu="$libs_softmmu $spice_libs"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $spice_cflags"
|
|
spice_protocol_version=$($pkg_config --modversion spice-protocol)
|
|
spice_server_version=$($pkg_config --modversion spice-server)
|
|
else
|
|
if test "$spice" = "yes" ; then
|
|
feature_not_found "spice" \
|
|
"Install spice-server(>=0.12.0) and spice-protocol(>=0.12.3) devel"
|
|
fi
|
|
spice="no"
|
|
fi
|
|
fi
|
|
|
|
# check for libcacard for smartcard support
|
|
smartcard_cflags=""
|
|
# TODO - what's the minimal nss version we support?
|
|
if test "$smartcard_nss" != "no"; then
|
|
cat > $TMPC << EOF
|
|
#include <pk11pub.h>
|
|
int main(void) { PK11_FreeSlot(0); return 0; }
|
|
EOF
|
|
# FIXME: do not include $glib_* in here
|
|
nss_libs="$($pkg_config --libs nss 2>/dev/null) $glib_libs"
|
|
nss_cflags="$($pkg_config --cflags nss 2>/dev/null) $glib_cflags"
|
|
test_cflags="$nss_cflags"
|
|
# The header files in nss < 3.13.3 have a bug which causes them to
|
|
# emit a warning. If we're going to compile QEMU with -Werror, then
|
|
# test that the headers don't have this bug. Otherwise we would pass
|
|
# the configure test but fail to compile QEMU later.
|
|
if test "$werror" = "yes"; then
|
|
test_cflags="-Werror $test_cflags"
|
|
fi
|
|
if test -n "$libtool" &&
|
|
$pkg_config --atleast-version=3.12.8 nss && \
|
|
compile_prog "$test_cflags" "$nss_libs"; then
|
|
smartcard_nss="yes"
|
|
else
|
|
if test "$smartcard_nss" = "yes"; then
|
|
feature_not_found "nss" "Install nss devel >= 3.12.8"
|
|
fi
|
|
smartcard_nss="no"
|
|
fi
|
|
fi
|
|
|
|
# check for libusb
|
|
if test "$libusb" != "no" ; then
|
|
if $pkg_config --atleast-version=1.0.13 libusb-1.0; then
|
|
libusb="yes"
|
|
libusb_cflags=$($pkg_config --cflags libusb-1.0)
|
|
libusb_libs=$($pkg_config --libs libusb-1.0)
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $libusb_cflags"
|
|
libs_softmmu="$libs_softmmu $libusb_libs"
|
|
else
|
|
if test "$libusb" = "yes"; then
|
|
feature_not_found "libusb" "Install libusb devel >= 1.0.13"
|
|
fi
|
|
libusb="no"
|
|
fi
|
|
fi
|
|
|
|
# check for usbredirparser for usb network redirection support
|
|
if test "$usb_redir" != "no" ; then
|
|
if $pkg_config --atleast-version=0.6 libusbredirparser-0.5; then
|
|
usb_redir="yes"
|
|
usb_redir_cflags=$($pkg_config --cflags libusbredirparser-0.5)
|
|
usb_redir_libs=$($pkg_config --libs libusbredirparser-0.5)
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $usb_redir_cflags"
|
|
libs_softmmu="$libs_softmmu $usb_redir_libs"
|
|
else
|
|
if test "$usb_redir" = "yes"; then
|
|
feature_not_found "usb-redir" "Install usbredir devel"
|
|
fi
|
|
usb_redir="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# check if we have VSS SDK headers for win
|
|
|
|
if test "$mingw32" = "yes" -a "$guest_agent" != "no" -a "$vss_win32_sdk" != "no" ; then
|
|
case "$vss_win32_sdk" in
|
|
"") vss_win32_include="-I$source_path" ;;
|
|
*\ *) # The SDK is installed in "Program Files" by default, but we cannot
|
|
# handle path with spaces. So we symlink the headers into ".sdk/vss".
|
|
vss_win32_include="-I$source_path/.sdk/vss"
|
|
symlink "$vss_win32_sdk/inc" "$source_path/.sdk/vss/inc"
|
|
;;
|
|
*) vss_win32_include="-I$vss_win32_sdk"
|
|
esac
|
|
cat > $TMPC << EOF
|
|
#define __MIDL_user_allocate_free_DEFINED__
|
|
#include <inc/win2003/vss.h>
|
|
int main(void) { return VSS_CTX_BACKUP; }
|
|
EOF
|
|
if compile_prog "$vss_win32_include" "" ; then
|
|
guest_agent_with_vss="yes"
|
|
QEMU_CFLAGS="$QEMU_CFLAGS $vss_win32_include"
|
|
libs_qga="-lole32 -loleaut32 -lshlwapi -luuid -lstdc++ -Wl,--enable-stdcall-fixup $libs_qga"
|
|
else
|
|
if test "$vss_win32_sdk" != "" ; then
|
|
echo "ERROR: Please download and install Microsoft VSS SDK:"
|
|
echo "ERROR: http://www.microsoft.com/en-us/download/details.aspx?id=23490"
|
|
echo "ERROR: On POSIX-systems, you can extract the SDK headers by:"
|
|
echo "ERROR: scripts/extract-vsssdk-headers setup.exe"
|
|
echo "ERROR: The headers are extracted in the directory \`inc'."
|
|
feature_not_found "VSS support"
|
|
fi
|
|
guest_agent_with_vss="no"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# lookup Windows platform SDK (if not specified)
|
|
# The SDK is needed only to build .tlb (type library) file of guest agent
|
|
# VSS provider from the source. It is usually unnecessary because the
|
|
# pre-compiled .tlb file is included.
|
|
|
|
if test "$mingw32" = "yes" -a "$guest_agent" != "no" -a "$guest_agent_with_vss" = "yes" ; then
|
|
if test -z "$win_sdk"; then
|
|
programfiles="$PROGRAMFILES"
|
|
test -n "$PROGRAMW6432" && programfiles="$PROGRAMW6432"
|
|
if test -n "$programfiles"; then
|
|
win_sdk=$(ls -d "$programfiles/Microsoft SDKs/Windows/v"* | tail -1) 2>/dev/null
|
|
else
|
|
feature_not_found "Windows SDK"
|
|
fi
|
|
elif test "$win_sdk" = "no"; then
|
|
win_sdk=""
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# Guest agent Window MSI package
|
|
|
|
if test "$guest_agent" != yes; then
|
|
if test "$guest_agent_msi" = yes; then
|
|
error_exit "MSI guest agent package requires guest agent enabled"
|
|
fi
|
|
guest_agent_msi=no
|
|
elif test "$mingw32" != "yes"; then
|
|
if test "$guest_agent_msi" = "yes"; then
|
|
error_exit "MSI guest agent package is available only for MinGW Windows cross-compilation"
|
|
fi
|
|
guest_agent_msi=no
|
|
elif ! has wixl; then
|
|
if test "$guest_agent_msi" = "yes"; then
|
|
error_exit "MSI guest agent package requires wixl tool installed ( usually from msitools package )"
|
|
fi
|
|
guest_agent_msi=no
|
|
fi
|
|
|
|
if test "$guest_agent_msi" != "no"; then
|
|
if test "$guest_agent_with_vss" = "yes"; then
|
|
QEMU_GA_MSI_WITH_VSS="-D InstallVss"
|
|
fi
|
|
|
|
if test "$QEMU_GA_MANUFACTURER" = ""; then
|
|
QEMU_GA_MANUFACTURER=QEMU
|
|
fi
|
|
|
|
if test "$QEMU_GA_DISTRO" = ""; then
|
|
QEMU_GA_DISTRO=Linux
|
|
fi
|
|
|
|
if test "$QEMU_GA_VERSION" = ""; then
|
|
QEMU_GA_VERSION=`cat $source_path/VERSION`
|
|
fi
|
|
|
|
QEMU_GA_MSI_MINGW_DLL_PATH="-D Mingw_dlls=`$pkg_config --variable=prefix glib-2.0`/bin"
|
|
|
|
case "$cpu" in
|
|
x86_64)
|
|
QEMU_GA_MSI_ARCH="-a x64 -D Arch=64"
|
|
;;
|
|
i386)
|
|
QEMU_GA_MSI_ARCH="-D Arch=32"
|
|
;;
|
|
*)
|
|
error_exit "CPU $cpu not supported for building installation package"
|
|
;;
|
|
esac
|
|
fi
|
|
|
|
##########################################
|
|
# check if we have fdatasync
|
|
|
|
fdatasync=no
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
int main(void) {
|
|
#if defined(_POSIX_SYNCHRONIZED_IO) && _POSIX_SYNCHRONIZED_IO > 0
|
|
return fdatasync(0);
|
|
#else
|
|
#error Not supported
|
|
#endif
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
fdatasync=yes
|
|
fi
|
|
|
|
##########################################
|
|
# check if we have madvise
|
|
|
|
madvise=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/types.h>
|
|
#include <sys/mman.h>
|
|
#include <stddef.h>
|
|
int main(void) { return madvise(NULL, 0, MADV_DONTNEED); }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
madvise=yes
|
|
fi
|
|
|
|
##########################################
|
|
# check if we have posix_madvise
|
|
|
|
posix_madvise=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/mman.h>
|
|
#include <stddef.h>
|
|
int main(void) { return posix_madvise(NULL, 0, POSIX_MADV_DONTNEED); }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
posix_madvise=yes
|
|
fi
|
|
|
|
##########################################
|
|
# check if we have usable SIGEV_THREAD_ID
|
|
|
|
sigev_thread_id=no
|
|
cat > $TMPC << EOF
|
|
#include <signal.h>
|
|
int main(void) {
|
|
struct sigevent ev;
|
|
ev.sigev_notify = SIGEV_THREAD_ID;
|
|
ev._sigev_un._tid = 0;
|
|
asm volatile("" : : "g"(&ev));
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
sigev_thread_id=yes
|
|
fi
|
|
|
|
##########################################
|
|
# check if trace backend exists
|
|
|
|
$python "$source_path/scripts/tracetool.py" "--backends=$trace_backends" --check-backends > /dev/null 2> /dev/null
|
|
if test "$?" -ne 0 ; then
|
|
error_exit "invalid trace backends" \
|
|
"Please choose supported trace backends."
|
|
fi
|
|
|
|
##########################################
|
|
# For 'ust' backend, test if ust headers are present
|
|
if have_backend "ust"; then
|
|
cat > $TMPC << EOF
|
|
#include <lttng/tracepoint.h>
|
|
int main(void) { return 0; }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
if $pkg_config lttng-ust --exists; then
|
|
lttng_ust_libs=`$pkg_config --libs lttng-ust`
|
|
else
|
|
lttng_ust_libs="-llttng-ust"
|
|
fi
|
|
if $pkg_config liburcu-bp --exists; then
|
|
urcu_bp_libs=`$pkg_config --libs liburcu-bp`
|
|
else
|
|
urcu_bp_libs="-lurcu-bp"
|
|
fi
|
|
|
|
LIBS="$lttng_ust_libs $urcu_bp_libs $LIBS"
|
|
libs_qga="$lttng_ust_libs $urcu_bp_libs $libs_qga"
|
|
else
|
|
error_exit "Trace backend 'ust' missing lttng-ust header files"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# For 'dtrace' backend, test if 'dtrace' command is present
|
|
if have_backend "dtrace"; then
|
|
if ! has 'dtrace' ; then
|
|
error_exit "dtrace command is not found in PATH $PATH"
|
|
fi
|
|
trace_backend_stap="no"
|
|
if has 'stap' ; then
|
|
trace_backend_stap="yes"
|
|
fi
|
|
fi
|
|
|
|
##########################################
|
|
# check and set a backend for coroutine
|
|
|
|
# We prefer ucontext, but it's not always possible. The fallback
|
|
# is sigcontext. gthread is not selectable except explicitly, because
|
|
# it is not functional enough to run QEMU proper. (It is occasionally
|
|
# useful for debugging purposes.) On Windows the only valid backend
|
|
# is the Windows-specific one.
|
|
|
|
ucontext_works=no
|
|
if test "$darwin" != "yes"; then
|
|
cat > $TMPC << EOF
|
|
#include <ucontext.h>
|
|
#ifdef __stub_makecontext
|
|
#error Ignoring glibc stub makecontext which will always fail
|
|
#endif
|
|
int main(void) { makecontext(0, 0, 0); return 0; }
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
ucontext_works=yes
|
|
fi
|
|
fi
|
|
|
|
if test "$coroutine" = ""; then
|
|
if test "$mingw32" = "yes"; then
|
|
coroutine=win32
|
|
elif test "$ucontext_works" = "yes"; then
|
|
coroutine=ucontext
|
|
else
|
|
coroutine=sigaltstack
|
|
fi
|
|
else
|
|
case $coroutine in
|
|
windows)
|
|
if test "$mingw32" != "yes"; then
|
|
error_exit "'windows' coroutine backend only valid for Windows"
|
|
fi
|
|
# Unfortunately the user visible backend name doesn't match the
|
|
# coroutine-*.c filename for this case, so we have to adjust it here.
|
|
coroutine=win32
|
|
;;
|
|
ucontext)
|
|
if test "$ucontext_works" != "yes"; then
|
|
feature_not_found "ucontext"
|
|
fi
|
|
;;
|
|
gthread|sigaltstack)
|
|
if test "$mingw32" = "yes"; then
|
|
error_exit "only the 'windows' coroutine backend is valid for Windows"
|
|
fi
|
|
;;
|
|
*)
|
|
error_exit "unknown coroutine backend $coroutine"
|
|
;;
|
|
esac
|
|
fi
|
|
|
|
if test "$coroutine_pool" = ""; then
|
|
if test "$coroutine" = "gthread"; then
|
|
coroutine_pool=no
|
|
else
|
|
coroutine_pool=yes
|
|
fi
|
|
fi
|
|
if test "$coroutine" = "gthread" -a "$coroutine_pool" = "yes"; then
|
|
error_exit "'gthread' coroutine backend does not support pool (use --disable-coroutine-pool)"
|
|
fi
|
|
|
|
##########################################
|
|
# check if we have open_by_handle_at
|
|
|
|
open_by_handle_at=no
|
|
cat > $TMPC << EOF
|
|
#include <fcntl.h>
|
|
#if !defined(AT_EMPTY_PATH)
|
|
# error missing definition
|
|
#else
|
|
int main(void) { struct file_handle fh; return open_by_handle_at(0, &fh, 0); }
|
|
#endif
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
open_by_handle_at=yes
|
|
fi
|
|
|
|
########################################
|
|
# check if we have linux/magic.h
|
|
|
|
linux_magic_h=no
|
|
cat > $TMPC << EOF
|
|
#include <linux/magic.h>
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
linux_magic_h=yes
|
|
fi
|
|
|
|
########################################
|
|
# check whether we can disable warning option with a pragma (this is needed
|
|
# to silence warnings in the headers of some versions of external libraries).
|
|
# This test has to be compiled with -Werror as otherwise an unknown pragma is
|
|
# only a warning.
|
|
#
|
|
# If we can't selectively disable warning in the code, disable -Werror so that
|
|
# the build doesn't fail anyway.
|
|
|
|
pragma_disable_unused_but_set=no
|
|
cat > $TMPC << EOF
|
|
#pragma GCC diagnostic push
|
|
#pragma GCC diagnostic ignored "-Wunused-but-set-variable"
|
|
#pragma GCC diagnostic ignored "-Wstrict-prototypes"
|
|
#pragma GCC diagnostic pop
|
|
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "-Werror" "" ; then
|
|
pragma_diagnostic_available=yes
|
|
else
|
|
werror=no
|
|
fi
|
|
|
|
########################################
|
|
# check if we have valgrind/valgrind.h
|
|
|
|
valgrind_h=no
|
|
cat > $TMPC << EOF
|
|
#include <valgrind/valgrind.h>
|
|
int main(void) {
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
valgrind_h=yes
|
|
fi
|
|
|
|
########################################
|
|
# check if environ is declared
|
|
|
|
has_environ=no
|
|
cat > $TMPC << EOF
|
|
#include <unistd.h>
|
|
int main(void) {
|
|
environ = 0;
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
has_environ=yes
|
|
fi
|
|
|
|
########################################
|
|
# check if cpuid.h is usable.
|
|
|
|
cpuid_h=no
|
|
cat > $TMPC << EOF
|
|
#include <cpuid.h>
|
|
int main(void) {
|
|
unsigned a, b, c, d;
|
|
int max = __get_cpuid_max(0, 0);
|
|
|
|
if (max >= 1) {
|
|
__cpuid(1, a, b, c, d);
|
|
}
|
|
|
|
if (max >= 7) {
|
|
__cpuid_count(7, 0, a, b, c, d);
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
cpuid_h=yes
|
|
fi
|
|
|
|
########################################
|
|
# check if __[u]int128_t is usable.
|
|
|
|
int128=no
|
|
cat > $TMPC << EOF
|
|
#if defined(__clang_major__) && defined(__clang_minor__)
|
|
# if ((__clang_major__ < 3) || (__clang_major__ == 3) && (__clang_minor__ < 2))
|
|
# error __int128_t does not work in CLANG before 3.2
|
|
# endif
|
|
#endif
|
|
__int128_t a;
|
|
__uint128_t b;
|
|
int main (void) {
|
|
a = a + b;
|
|
b = a * b;
|
|
a = a * a;
|
|
return 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
int128=yes
|
|
fi
|
|
|
|
########################################
|
|
# check if getauxval is available.
|
|
|
|
getauxval=no
|
|
cat > $TMPC << EOF
|
|
#include <sys/auxv.h>
|
|
int main(void) {
|
|
return getauxval(AT_HWCAP) == 0;
|
|
}
|
|
EOF
|
|
if compile_prog "" "" ; then
|
|
getauxval=yes
|
|
fi
|
|
|
|
########################################
|
|
# check if ccache is interfering with
|
|
# semantic analysis of macros
|
|
|
|
ccache_cpp2=no
|
|
cat > $TMPC << EOF
|
|
static const int Z = 1;
|
|
#define fn() ({ Z; })
|
|
#define TAUT(X) ((X) == Z)
|
|
#define PAREN(X, Y) (X == Y)
|
|
#define ID(X) (X)
|
|
int main(int argc, char *argv[])
|
|
{
|
|
int x = 0, y = 0;
|
|
x = ID(x);
|
|
x = fn();
|
|
fn();
|
|
if (PAREN(x, y)) return 0;
|
|
if (TAUT(Z)) return 0;
|
|
return 0;
|
|
}
|
|
EOF
|
|
|
|
if ! compile_object "-Werror"; then
|
|
ccache_cpp2=yes
|
|
fi
|
|
|
|
##########################################
|
|
# End of CC checks
|
|
# After here, no more $cc or $ld runs
|
|
|
|
if test "$gcov" = "yes" ; then
|
|
CFLAGS="-fprofile-arcs -ftest-coverage -g $CFLAGS"
|
|
LDFLAGS="-fprofile-arcs -ftest-coverage $LDFLAGS"
|
|
elif test "$debug" = "no" ; then
|
|
CFLAGS="-O2 -U_FORTIFY_SOURCE -D_FORTIFY_SOURCE=2 $CFLAGS"
|
|
fi
|
|
|
|
##########################################
|
|
# Do we have libnfs
|
|
if test "$libnfs" != "no" ; then
|
|
if $pkg_config --atleast-version=1.9.3 libnfs; then
|
|
libnfs="yes"
|
|
libnfs_libs=$($pkg_config --libs libnfs)
|
|
LIBS="$LIBS $libnfs_libs"
|
|
else
|
|
if test "$libnfs" = "yes" ; then
|
|
feature_not_found "libnfs" "Install libnfs devel >= 1.9.3"
|
|
fi
|
|
libnfs="no"
|
|
fi
|
|
fi
|
|
|
|
# Disable zero malloc errors for official releases unless explicitly told to
|
|
# enable/disable
|
|
if test -z "$zero_malloc" ; then
|
|
if test "$z_version" = "50" ; then
|
|
zero_malloc="no"
|
|
else
|
|
zero_malloc="yes"
|
|
fi
|
|
fi
|
|
|
|
# Now we've finished running tests it's OK to add -Werror to the compiler flags
|
|
if test "$werror" = "yes"; then
|
|
QEMU_CFLAGS="-Werror $QEMU_CFLAGS"
|
|
fi
|
|
|
|
if test "$solaris" = "no" ; then
|
|
if $ld --version 2>/dev/null | grep "GNU ld" >/dev/null 2>/dev/null ; then
|
|
LDFLAGS="-Wl,--warn-common $LDFLAGS"
|
|
fi
|
|
fi
|
|
|
|
# test if pod2man has --utf8 option
|
|
if pod2man --help | grep -q utf8; then
|
|
POD2MAN="pod2man --utf8"
|
|
else
|
|
POD2MAN="pod2man"
|
|
fi
|
|
|
|
# Use ASLR, no-SEH and DEP if available
|
|
if test "$mingw32" = "yes" ; then
|
|
for flag in --dynamicbase --no-seh --nxcompat; do
|
|
if $ld --help 2>/dev/null | grep ".$flag" >/dev/null 2>/dev/null ; then
|
|
LDFLAGS="-Wl,$flag $LDFLAGS"
|
|
fi
|
|
done
|
|
fi
|
|
|
|
qemu_confdir=$sysconfdir$confsuffix
|
|
qemu_moddir=$libdir$confsuffix
|
|
qemu_datadir=$datadir$confsuffix
|
|
qemu_localedir="$datadir/locale"
|
|
|
|
tools=""
|
|
if test "$want_tools" = "yes" ; then
|
|
tools="qemu-img\$(EXESUF) qemu-io\$(EXESUF) $tools"
|
|
if [ "$linux" = "yes" -o "$bsd" = "yes" -o "$solaris" = "yes" ] ; then
|
|
tools="qemu-nbd\$(EXESUF) $tools"
|
|
fi
|
|
fi
|
|
if test "$softmmu" = yes ; then
|
|
if test "$virtfs" != no ; then
|
|
if test "$cap" = yes && test "$linux" = yes && test "$attr" = yes ; then
|
|
virtfs=yes
|
|
tools="$tools fsdev/virtfs-proxy-helper\$(EXESUF)"
|
|
else
|
|
if test "$virtfs" = yes; then
|
|
error_exit "VirtFS is supported only on Linux and requires libcap-devel and libattr-devel"
|
|
fi
|
|
virtfs=no
|
|
fi
|
|
fi
|
|
fi
|
|
if [ "$guest_agent" != "no" ]; then
|
|
if [ "$linux" = "yes" -o "$bsd" = "yes" -o "$solaris" = "yes" -o "$mingw32" = "yes" ] ; then
|
|
tools="qemu-ga\$(EXESUF) $tools"
|
|
if [ "$mingw32" = "yes" -a "$guest_agent_with_vss" = "yes" ]; then
|
|
tools="qga/vss-win32/qga-vss.dll qga/vss-win32/qga-vss.tlb $tools"
|
|
fi
|
|
guest_agent=yes
|
|
elif [ "$guest_agent" != yes ]; then
|
|
guest_agent=no
|
|
else
|
|
error_exit "Guest agent is not supported on this platform"
|
|
fi
|
|
fi
|
|
|
|
# Mac OS X ships with a broken assembler
|
|
roms=
|
|
if test \( "$cpu" = "i386" -o "$cpu" = "x86_64" \) -a \
|
|
"$targetos" != "Darwin" -a "$targetos" != "SunOS" -a \
|
|
"$softmmu" = yes ; then
|
|
roms="optionrom"
|
|
fi
|
|
if test "$cpu" = "ppc64" -a "$targetos" != "Darwin" ; then
|
|
roms="$roms spapr-rtas"
|
|
fi
|
|
|
|
if test "$cpu" = "s390x" ; then
|
|
roms="$roms s390-ccw"
|
|
fi
|
|
|
|
# Probe for the need for relocating the user-only binary.
|
|
if test "$pie" = "no" ; then
|
|
textseg_addr=
|
|
case "$cpu" in
|
|
arm | i386 | ppc* | s390* | sparc* | x86_64 | x32)
|
|
# ??? Rationale for choosing this address
|
|
textseg_addr=0x60000000
|
|
;;
|
|
mips)
|
|
# A 256M aligned address, high in the address space, with enough
|
|
# room for the code_gen_buffer above it before the stack.
|
|
textseg_addr=0x60000000
|
|
;;
|
|
esac
|
|
if [ -n "$textseg_addr" ]; then
|
|
cat > $TMPC <<EOF
|
|
int main(void) { return 0; }
|
|
EOF
|
|
textseg_ldflags="-Wl,-Ttext-segment=$textseg_addr"
|
|
if ! compile_prog "" "$textseg_ldflags"; then
|
|
# In case ld does not support -Ttext-segment, edit the default linker
|
|
# script via sed to set the .text start addr. This is needed on FreeBSD
|
|
# at least.
|
|
$ld --verbose | sed \
|
|
-e '1,/==================================================/d' \
|
|
-e '/==================================================/,$d' \
|
|
-e "s/[.] = [0-9a-fx]* [+] SIZEOF_HEADERS/. = $textseg_addr + SIZEOF_HEADERS/" \
|
|
-e "s/__executable_start = [0-9a-fx]*/__executable_start = $textseg_addr/" > config-host.ld
|
|
textseg_ldflags="-Wl,-T../config-host.ld"
|
|
fi
|
|
fi
|
|
fi
|
|
|
|
# prepend pixman and ftd flags after all config tests are done
|
|
QEMU_CFLAGS="$pixman_cflags $fdt_cflags $QEMU_CFLAGS"
|
|
libs_softmmu="$pixman_libs $libs_softmmu"
|
|
|
|
echo "Install prefix $prefix"
|
|
echo "BIOS directory `eval echo $qemu_datadir`"
|
|
echo "binary directory `eval echo $bindir`"
|
|
echo "library directory `eval echo $libdir`"
|
|
echo "module directory `eval echo $qemu_moddir`"
|
|
echo "libexec directory `eval echo $libexecdir`"
|
|
echo "include directory `eval echo $includedir`"
|
|
echo "config directory `eval echo $sysconfdir`"
|
|
if test "$mingw32" = "no" ; then
|
|
echo "local state directory `eval echo $local_statedir`"
|
|
echo "Manual directory `eval echo $mandir`"
|
|
echo "ELF interp prefix $interp_prefix"
|
|
else
|
|
echo "local state directory queried at runtime"
|
|
echo "Windows SDK $win_sdk"
|
|
fi
|
|
echo "Source path $source_path"
|
|
echo "C compiler $cc"
|
|
echo "Host C compiler $host_cc"
|
|
echo "C++ compiler $cxx"
|
|
echo "Objective-C compiler $objcc"
|
|
echo "ARFLAGS $ARFLAGS"
|
|
echo "CFLAGS $CFLAGS"
|
|
echo "QEMU_CFLAGS $QEMU_CFLAGS"
|
|
echo "LDFLAGS $LDFLAGS"
|
|
echo "make $make"
|
|
echo "install $install"
|
|
echo "python $python"
|
|
if test "$slirp" = "yes" ; then
|
|
echo "smbd $smbd"
|
|
fi
|
|
echo "module support $modules"
|
|
echo "host CPU $cpu"
|
|
echo "host big endian $bigendian"
|
|
echo "target list $target_list"
|
|
echo "tcg debug enabled $debug_tcg"
|
|
echo "gprof enabled $gprof"
|
|
echo "sparse enabled $sparse"
|
|
echo "strip binaries $strip_opt"
|
|
echo "profiler $profiler"
|
|
echo "static build $static"
|
|
if test "$darwin" = "yes" ; then
|
|
echo "Cocoa support $cocoa"
|
|
fi
|
|
echo "pixman $pixman"
|
|
echo "SDL support $sdl"
|
|
echo "GTK support $gtk"
|
|
echo "GNUTLS support $gnutls"
|
|
echo "GNUTLS hash $gnutls_hash"
|
|
echo "VTE support $vte"
|
|
echo "curses support $curses"
|
|
echo "curl support $curl"
|
|
echo "mingw32 support $mingw32"
|
|
echo "Audio drivers $audio_drv_list"
|
|
echo "Block whitelist (rw) $block_drv_rw_whitelist"
|
|
echo "Block whitelist (ro) $block_drv_ro_whitelist"
|
|
echo "VirtFS support $virtfs"
|
|
echo "VNC support $vnc"
|
|
if test "$vnc" = "yes" ; then
|
|
echo "VNC TLS support $vnc_tls"
|
|
echo "VNC SASL support $vnc_sasl"
|
|
echo "VNC JPEG support $vnc_jpeg"
|
|
echo "VNC PNG support $vnc_png"
|
|
echo "VNC WS support $vnc_ws"
|
|
fi
|
|
if test -n "$sparc_cpu"; then
|
|
echo "Target Sparc Arch $sparc_cpu"
|
|
fi
|
|
echo "xen support $xen"
|
|
if test "$xen" = "yes" ; then
|
|
echo "xen ctrl version $xen_ctrl_version"
|
|
fi
|
|
echo "brlapi support $brlapi"
|
|
echo "bluez support $bluez"
|
|
echo "Documentation $docs"
|
|
echo "GUEST_BASE $guest_base"
|
|
echo "PIE $pie"
|
|
echo "vde support $vde"
|
|
echo "netmap support $netmap"
|
|
echo "Linux AIO support $linux_aio"
|
|
echo "ATTR/XATTR support $attr"
|
|
echo "Install blobs $blobs"
|
|
echo "KVM support $kvm"
|
|
echo "RDMA support $rdma"
|
|
echo "TCG interpreter $tcg_interpreter"
|
|
echo "fdt support $fdt"
|
|
echo "preadv support $preadv"
|
|
echo "fdatasync $fdatasync"
|
|
echo "madvise $madvise"
|
|
echo "posix_madvise $posix_madvise"
|
|
echo "sigev_thread_id $sigev_thread_id"
|
|
echo "uuid support $uuid"
|
|
echo "libcap-ng support $cap_ng"
|
|
echo "vhost-net support $vhost_net"
|
|
echo "vhost-scsi support $vhost_scsi"
|
|
echo "Trace backends $trace_backends"
|
|
if test "$trace_backend" = "simple"; then
|
|
echo "Trace output file $trace_file-<pid>"
|
|
fi
|
|
if test "$spice" = "yes"; then
|
|
echo "spice support $spice ($spice_protocol_version/$spice_server_version)"
|
|
else
|
|
echo "spice support $spice"
|
|
fi
|
|
echo "rbd support $rbd"
|
|
echo "xfsctl support $xfs"
|
|
echo "nss used $smartcard_nss"
|
|
echo "libusb $libusb"
|
|
echo "usb net redir $usb_redir"
|
|
echo "OpenGL support $opengl"
|
|
echo "libiscsi support $libiscsi"
|
|
echo "libnfs support $libnfs"
|
|
echo "build guest agent $guest_agent"
|
|
echo "QGA VSS support $guest_agent_with_vss"
|
|
echo "seccomp support $seccomp"
|
|
echo "coroutine backend $coroutine"
|
|
echo "coroutine pool $coroutine_pool"
|
|
echo "GlusterFS support $glusterfs"
|
|
echo "Archipelago support $archipelago"
|
|
echo "gcov $gcov_tool"
|
|
echo "gcov enabled $gcov"
|
|
echo "TPM support $tpm"
|
|
echo "libssh2 support $libssh2"
|
|
echo "TPM passthrough $tpm_passthrough"
|
|
echo "QOM debugging $qom_cast_debug"
|
|
echo "vhdx $vhdx"
|
|
echo "Quorum $quorum"
|
|
echo "lzo support $lzo"
|
|
echo "snappy support $snappy"
|
|
echo "bzip2 support $bzip2"
|
|
echo "NUMA host support $numa"
|
|
echo "tcmalloc support $tcmalloc"
|
|
|
|
if test "$sdl_too_old" = "yes"; then
|
|
echo "-> Your SDL version is too old - please upgrade to have SDL support"
|
|
fi
|
|
|
|
config_host_mak="config-host.mak"
|
|
|
|
echo "# Automatically generated by configure - do not modify" >config-all-disas.mak
|
|
|
|
echo "# Automatically generated by configure - do not modify" > $config_host_mak
|
|
echo >> $config_host_mak
|
|
|
|
echo all: >> $config_host_mak
|
|
echo "prefix=$prefix" >> $config_host_mak
|
|
echo "bindir=$bindir" >> $config_host_mak
|
|
echo "libdir=$libdir" >> $config_host_mak
|
|
echo "libexecdir=$libexecdir" >> $config_host_mak
|
|
echo "includedir=$includedir" >> $config_host_mak
|
|
echo "mandir=$mandir" >> $config_host_mak
|
|
echo "sysconfdir=$sysconfdir" >> $config_host_mak
|
|
echo "qemu_confdir=$qemu_confdir" >> $config_host_mak
|
|
echo "qemu_datadir=$qemu_datadir" >> $config_host_mak
|
|
echo "qemu_docdir=$qemu_docdir" >> $config_host_mak
|
|
echo "qemu_moddir=$qemu_moddir" >> $config_host_mak
|
|
if test "$mingw32" = "no" ; then
|
|
echo "qemu_localstatedir=$local_statedir" >> $config_host_mak
|
|
fi
|
|
echo "qemu_helperdir=$libexecdir" >> $config_host_mak
|
|
echo "extra_cflags=$EXTRA_CFLAGS" >> $config_host_mak
|
|
echo "extra_ldflags=$EXTRA_LDFLAGS" >> $config_host_mak
|
|
echo "qemu_localedir=$qemu_localedir" >> $config_host_mak
|
|
echo "libs_softmmu=$libs_softmmu" >> $config_host_mak
|
|
|
|
echo "ARCH=$ARCH" >> $config_host_mak
|
|
|
|
if test "$debug_tcg" = "yes" ; then
|
|
echo "CONFIG_DEBUG_TCG=y" >> $config_host_mak
|
|
fi
|
|
if test "$strip_opt" = "yes" ; then
|
|
echo "STRIP=${strip}" >> $config_host_mak
|
|
fi
|
|
if test "$bigendian" = "yes" ; then
|
|
echo "HOST_WORDS_BIGENDIAN=y" >> $config_host_mak
|
|
fi
|
|
if test "$mingw32" = "yes" ; then
|
|
echo "CONFIG_WIN32=y" >> $config_host_mak
|
|
rc_version=`cat $source_path/VERSION`
|
|
version_major=${rc_version%%.*}
|
|
rc_version=${rc_version#*.}
|
|
version_minor=${rc_version%%.*}
|
|
rc_version=${rc_version#*.}
|
|
version_subminor=${rc_version%%.*}
|
|
version_micro=0
|
|
echo "CONFIG_FILEVERSION=$version_major,$version_minor,$version_subminor,$version_micro" >> $config_host_mak
|
|
echo "CONFIG_PRODUCTVERSION=$version_major,$version_minor,$version_subminor,$version_micro" >> $config_host_mak
|
|
if test "$guest_agent_with_vss" = "yes" ; then
|
|
echo "CONFIG_QGA_VSS=y" >> $config_host_mak
|
|
echo "WIN_SDK=\"$win_sdk\"" >> $config_host_mak
|
|
fi
|
|
if test "$guest_agent_msi" != "no"; then
|
|
echo "QEMU_GA_MSI_ENABLED=yes" >> $config_host_mak
|
|
echo "QEMU_GA_MSI_MINGW_DLL_PATH=${QEMU_GA_MSI_MINGW_DLL_PATH}" >> $config_host_mak
|
|
echo "QEMU_GA_MSI_WITH_VSS=${QEMU_GA_MSI_WITH_VSS}" >> $config_host_mak
|
|
echo "QEMU_GA_MSI_ARCH=${QEMU_GA_MSI_ARCH}" >> $config_host_mak
|
|
echo "QEMU_GA_MANUFACTURER=${QEMU_GA_MANUFACTURER}" >> $config_host_mak
|
|
echo "QEMU_GA_DISTRO=${QEMU_GA_DISTRO}" >> $config_host_mak
|
|
echo "QEMU_GA_VERSION=${QEMU_GA_VERSION}" >> $config_host_mak
|
|
fi
|
|
else
|
|
echo "CONFIG_POSIX=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$linux" = "yes" ; then
|
|
echo "CONFIG_LINUX=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$darwin" = "yes" ; then
|
|
echo "CONFIG_DARWIN=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$aix" = "yes" ; then
|
|
echo "CONFIG_AIX=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$solaris" = "yes" ; then
|
|
echo "CONFIG_SOLARIS=y" >> $config_host_mak
|
|
echo "CONFIG_SOLARIS_VERSION=$solarisrev" >> $config_host_mak
|
|
if test "$needs_libsunmath" = "yes" ; then
|
|
echo "CONFIG_NEEDS_LIBSUNMATH=y" >> $config_host_mak
|
|
fi
|
|
fi
|
|
if test "$haiku" = "yes" ; then
|
|
echo "CONFIG_HAIKU=y" >> $config_host_mak
|
|
fi
|
|
if test "$static" = "yes" ; then
|
|
echo "CONFIG_STATIC=y" >> $config_host_mak
|
|
fi
|
|
if test "$profiler" = "yes" ; then
|
|
echo "CONFIG_PROFILER=y" >> $config_host_mak
|
|
fi
|
|
if test "$slirp" = "yes" ; then
|
|
echo "CONFIG_SLIRP=y" >> $config_host_mak
|
|
echo "CONFIG_SMBD_COMMAND=\"$smbd\"" >> $config_host_mak
|
|
fi
|
|
if test "$vde" = "yes" ; then
|
|
echo "CONFIG_VDE=y" >> $config_host_mak
|
|
fi
|
|
if test "$netmap" = "yes" ; then
|
|
echo "CONFIG_NETMAP=y" >> $config_host_mak
|
|
fi
|
|
if test "$l2tpv3" = "yes" ; then
|
|
echo "CONFIG_L2TPV3=y" >> $config_host_mak
|
|
fi
|
|
if test "$cap_ng" = "yes" ; then
|
|
echo "CONFIG_LIBCAP=y" >> $config_host_mak
|
|
fi
|
|
echo "CONFIG_AUDIO_DRIVERS=$audio_drv_list" >> $config_host_mak
|
|
for drv in $audio_drv_list; do
|
|
def=CONFIG_`echo $drv | LC_ALL=C tr '[a-z]' '[A-Z]'`
|
|
echo "$def=y" >> $config_host_mak
|
|
done
|
|
if test "$audio_pt_int" = "yes" ; then
|
|
echo "CONFIG_AUDIO_PT_INT=y" >> $config_host_mak
|
|
fi
|
|
if test "$audio_win_int" = "yes" ; then
|
|
echo "CONFIG_AUDIO_WIN_INT=y" >> $config_host_mak
|
|
fi
|
|
echo "CONFIG_BDRV_RW_WHITELIST=$block_drv_rw_whitelist" >> $config_host_mak
|
|
echo "CONFIG_BDRV_RO_WHITELIST=$block_drv_ro_whitelist" >> $config_host_mak
|
|
if test "$vnc" = "yes" ; then
|
|
echo "CONFIG_VNC=y" >> $config_host_mak
|
|
fi
|
|
if test "$vnc_tls" = "yes" ; then
|
|
echo "CONFIG_VNC_TLS=y" >> $config_host_mak
|
|
fi
|
|
if test "$vnc_sasl" = "yes" ; then
|
|
echo "CONFIG_VNC_SASL=y" >> $config_host_mak
|
|
fi
|
|
if test "$vnc_jpeg" = "yes" ; then
|
|
echo "CONFIG_VNC_JPEG=y" >> $config_host_mak
|
|
fi
|
|
if test "$vnc_png" = "yes" ; then
|
|
echo "CONFIG_VNC_PNG=y" >> $config_host_mak
|
|
fi
|
|
if test "$vnc_ws" = "yes" ; then
|
|
echo "CONFIG_VNC_WS=y" >> $config_host_mak
|
|
echo "VNC_WS_CFLAGS=$vnc_ws_cflags" >> $config_host_mak
|
|
fi
|
|
if test "$fnmatch" = "yes" ; then
|
|
echo "CONFIG_FNMATCH=y" >> $config_host_mak
|
|
fi
|
|
if test "$uuid" = "yes" ; then
|
|
echo "CONFIG_UUID=y" >> $config_host_mak
|
|
fi
|
|
if test "$xfs" = "yes" ; then
|
|
echo "CONFIG_XFS=y" >> $config_host_mak
|
|
fi
|
|
qemu_version=`head $source_path/VERSION`
|
|
echo "VERSION=$qemu_version" >>$config_host_mak
|
|
echo "PKGVERSION=$pkgversion" >>$config_host_mak
|
|
echo "SRC_PATH=$source_path" >> $config_host_mak
|
|
echo "TARGET_DIRS=$target_list" >> $config_host_mak
|
|
if [ "$docs" = "yes" ] ; then
|
|
echo "BUILD_DOCS=yes" >> $config_host_mak
|
|
fi
|
|
if test "$modules" = "yes"; then
|
|
# $shacmd can generate a hash started with digit, which the compiler doesn't
|
|
# like as an symbol. So prefix it with an underscore
|
|
echo "CONFIG_STAMP=_`(echo $qemu_version; echo $pkgversion; cat $0) | $shacmd - | cut -f1 -d\ `" >> $config_host_mak
|
|
echo "CONFIG_MODULES=y" >> $config_host_mak
|
|
fi
|
|
if test "$sdl" = "yes" ; then
|
|
echo "CONFIG_SDL=y" >> $config_host_mak
|
|
echo "CONFIG_SDLABI=$sdlabi" >> $config_host_mak
|
|
echo "SDL_CFLAGS=$sdl_cflags" >> $config_host_mak
|
|
fi
|
|
if test "$cocoa" = "yes" ; then
|
|
echo "CONFIG_COCOA=y" >> $config_host_mak
|
|
fi
|
|
if test "$curses" = "yes" ; then
|
|
echo "CONFIG_CURSES=y" >> $config_host_mak
|
|
fi
|
|
if test "$utimens" = "yes" ; then
|
|
echo "CONFIG_UTIMENSAT=y" >> $config_host_mak
|
|
fi
|
|
if test "$pipe2" = "yes" ; then
|
|
echo "CONFIG_PIPE2=y" >> $config_host_mak
|
|
fi
|
|
if test "$accept4" = "yes" ; then
|
|
echo "CONFIG_ACCEPT4=y" >> $config_host_mak
|
|
fi
|
|
if test "$splice" = "yes" ; then
|
|
echo "CONFIG_SPLICE=y" >> $config_host_mak
|
|
fi
|
|
if test "$eventfd" = "yes" ; then
|
|
echo "CONFIG_EVENTFD=y" >> $config_host_mak
|
|
fi
|
|
if test "$fallocate" = "yes" ; then
|
|
echo "CONFIG_FALLOCATE=y" >> $config_host_mak
|
|
fi
|
|
if test "$fallocate_punch_hole" = "yes" ; then
|
|
echo "CONFIG_FALLOCATE_PUNCH_HOLE=y" >> $config_host_mak
|
|
fi
|
|
if test "$fallocate_zero_range" = "yes" ; then
|
|
echo "CONFIG_FALLOCATE_ZERO_RANGE=y" >> $config_host_mak
|
|
fi
|
|
if test "$posix_fallocate" = "yes" ; then
|
|
echo "CONFIG_POSIX_FALLOCATE=y" >> $config_host_mak
|
|
fi
|
|
if test "$sync_file_range" = "yes" ; then
|
|
echo "CONFIG_SYNC_FILE_RANGE=y" >> $config_host_mak
|
|
fi
|
|
if test "$fiemap" = "yes" ; then
|
|
echo "CONFIG_FIEMAP=y" >> $config_host_mak
|
|
fi
|
|
if test "$dup3" = "yes" ; then
|
|
echo "CONFIG_DUP3=y" >> $config_host_mak
|
|
fi
|
|
if test "$ppoll" = "yes" ; then
|
|
echo "CONFIG_PPOLL=y" >> $config_host_mak
|
|
fi
|
|
if test "$prctl_pr_set_timerslack" = "yes" ; then
|
|
echo "CONFIG_PRCTL_PR_SET_TIMERSLACK=y" >> $config_host_mak
|
|
fi
|
|
if test "$epoll" = "yes" ; then
|
|
echo "CONFIG_EPOLL=y" >> $config_host_mak
|
|
fi
|
|
if test "$epoll_create1" = "yes" ; then
|
|
echo "CONFIG_EPOLL_CREATE1=y" >> $config_host_mak
|
|
fi
|
|
if test "$epoll_pwait" = "yes" ; then
|
|
echo "CONFIG_EPOLL_PWAIT=y" >> $config_host_mak
|
|
fi
|
|
if test "$sendfile" = "yes" ; then
|
|
echo "CONFIG_SENDFILE=y" >> $config_host_mak
|
|
fi
|
|
if test "$timerfd" = "yes" ; then
|
|
echo "CONFIG_TIMERFD=y" >> $config_host_mak
|
|
fi
|
|
if test "$setns" = "yes" ; then
|
|
echo "CONFIG_SETNS=y" >> $config_host_mak
|
|
fi
|
|
if test "$inotify" = "yes" ; then
|
|
echo "CONFIG_INOTIFY=y" >> $config_host_mak
|
|
fi
|
|
if test "$inotify1" = "yes" ; then
|
|
echo "CONFIG_INOTIFY1=y" >> $config_host_mak
|
|
fi
|
|
if test "$byteswap_h" = "yes" ; then
|
|
echo "CONFIG_BYTESWAP_H=y" >> $config_host_mak
|
|
fi
|
|
if test "$bswap_h" = "yes" ; then
|
|
echo "CONFIG_MACHINE_BSWAP_H=y" >> $config_host_mak
|
|
fi
|
|
if test "$curl" = "yes" ; then
|
|
echo "CONFIG_CURL=m" >> $config_host_mak
|
|
echo "CURL_CFLAGS=$curl_cflags" >> $config_host_mak
|
|
echo "CURL_LIBS=$curl_libs" >> $config_host_mak
|
|
fi
|
|
if test "$brlapi" = "yes" ; then
|
|
echo "CONFIG_BRLAPI=y" >> $config_host_mak
|
|
fi
|
|
if test "$bluez" = "yes" ; then
|
|
echo "CONFIG_BLUEZ=y" >> $config_host_mak
|
|
echo "BLUEZ_CFLAGS=$bluez_cflags" >> $config_host_mak
|
|
fi
|
|
if test "$glib_subprocess" = "yes" ; then
|
|
echo "CONFIG_HAS_GLIB_SUBPROCESS_TESTS=y" >> $config_host_mak
|
|
fi
|
|
echo "GLIB_CFLAGS=$glib_cflags" >> $config_host_mak
|
|
if test "$gtk" = "yes" ; then
|
|
echo "CONFIG_GTK=y" >> $config_host_mak
|
|
echo "CONFIG_GTKABI=$gtkabi" >> $config_host_mak
|
|
echo "GTK_CFLAGS=$gtk_cflags" >> $config_host_mak
|
|
fi
|
|
if test "$gnutls" = "yes" ; then
|
|
echo "CONFIG_GNUTLS=y" >> $config_host_mak
|
|
fi
|
|
if test "$gnutls_hash" = "yes" ; then
|
|
echo "CONFIG_GNUTLS_HASH=y" >> $config_host_mak
|
|
fi
|
|
if test "$vte" = "yes" ; then
|
|
echo "CONFIG_VTE=y" >> $config_host_mak
|
|
echo "VTE_CFLAGS=$vte_cflags" >> $config_host_mak
|
|
fi
|
|
if test "$xen" = "yes" ; then
|
|
echo "CONFIG_XEN_BACKEND=y" >> $config_host_mak
|
|
echo "CONFIG_XEN_CTRL_INTERFACE_VERSION=$xen_ctrl_version" >> $config_host_mak
|
|
fi
|
|
if test "$linux_aio" = "yes" ; then
|
|
echo "CONFIG_LINUX_AIO=y" >> $config_host_mak
|
|
fi
|
|
if test "$attr" = "yes" ; then
|
|
echo "CONFIG_ATTR=y" >> $config_host_mak
|
|
fi
|
|
if test "$libattr" = "yes" ; then
|
|
echo "CONFIG_LIBATTR=y" >> $config_host_mak
|
|
fi
|
|
if test "$virtfs" = "yes" ; then
|
|
echo "CONFIG_VIRTFS=y" >> $config_host_mak
|
|
fi
|
|
if test "$vhost_scsi" = "yes" ; then
|
|
echo "CONFIG_VHOST_SCSI=y" >> $config_host_mak
|
|
fi
|
|
if test "$vhost_net" = "yes" ; then
|
|
echo "CONFIG_VHOST_NET_USED=y" >> $config_host_mak
|
|
fi
|
|
if test "$blobs" = "yes" ; then
|
|
echo "INSTALL_BLOBS=yes" >> $config_host_mak
|
|
fi
|
|
if test "$iovec" = "yes" ; then
|
|
echo "CONFIG_IOVEC=y" >> $config_host_mak
|
|
fi
|
|
if test "$preadv" = "yes" ; then
|
|
echo "CONFIG_PREADV=y" >> $config_host_mak
|
|
fi
|
|
if test "$fdt" = "yes" ; then
|
|
echo "CONFIG_FDT=y" >> $config_host_mak
|
|
fi
|
|
if test "$signalfd" = "yes" ; then
|
|
echo "CONFIG_SIGNALFD=y" >> $config_host_mak
|
|
fi
|
|
if test "$tcg_interpreter" = "yes" ; then
|
|
echo "CONFIG_TCG_INTERPRETER=y" >> $config_host_mak
|
|
fi
|
|
if test "$fdatasync" = "yes" ; then
|
|
echo "CONFIG_FDATASYNC=y" >> $config_host_mak
|
|
fi
|
|
if test "$madvise" = "yes" ; then
|
|
echo "CONFIG_MADVISE=y" >> $config_host_mak
|
|
fi
|
|
if test "$posix_madvise" = "yes" ; then
|
|
echo "CONFIG_POSIX_MADVISE=y" >> $config_host_mak
|
|
fi
|
|
if test "$sigev_thread_id" = "yes" ; then
|
|
echo "CONFIG_SIGEV_THREAD_ID=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$spice" = "yes" ; then
|
|
echo "CONFIG_SPICE=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$smartcard_nss" = "yes" ; then
|
|
echo "CONFIG_SMARTCARD_NSS=y" >> $config_host_mak
|
|
echo "NSS_LIBS=$nss_libs" >> $config_host_mak
|
|
echo "NSS_CFLAGS=$nss_cflags" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$libusb" = "yes" ; then
|
|
echo "CONFIG_USB_LIBUSB=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$usb_redir" = "yes" ; then
|
|
echo "CONFIG_USB_REDIR=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$opengl" = "yes" ; then
|
|
echo "CONFIG_OPENGL=y" >> $config_host_mak
|
|
echo "OPENGL_CFLAGS=$opengl_cflags" >> $config_host_mak
|
|
echo "OPENGL_LIBS=$opengl_libs" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$lzo" = "yes" ; then
|
|
echo "CONFIG_LZO=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$snappy" = "yes" ; then
|
|
echo "CONFIG_SNAPPY=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$bzip2" = "yes" ; then
|
|
echo "CONFIG_BZIP2=y" >> $config_host_mak
|
|
echo "BZIP2_LIBS=-lbz2" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$libiscsi" = "yes" ; then
|
|
echo "CONFIG_LIBISCSI=m" >> $config_host_mak
|
|
echo "LIBISCSI_CFLAGS=$libiscsi_cflags" >> $config_host_mak
|
|
echo "LIBISCSI_LIBS=$libiscsi_libs" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$libnfs" = "yes" ; then
|
|
echo "CONFIG_LIBNFS=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$seccomp" = "yes"; then
|
|
echo "CONFIG_SECCOMP=y" >> $config_host_mak
|
|
fi
|
|
|
|
# XXX: suppress that
|
|
if [ "$bsd" = "yes" ] ; then
|
|
echo "CONFIG_BSD=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$zero_malloc" = "yes" ; then
|
|
echo "CONFIG_ZERO_MALLOC=y" >> $config_host_mak
|
|
fi
|
|
if test "$qom_cast_debug" = "yes" ; then
|
|
echo "CONFIG_QOM_CAST_DEBUG=y" >> $config_host_mak
|
|
fi
|
|
if test "$rbd" = "yes" ; then
|
|
echo "CONFIG_RBD=m" >> $config_host_mak
|
|
echo "RBD_CFLAGS=$rbd_cflags" >> $config_host_mak
|
|
echo "RBD_LIBS=$rbd_libs" >> $config_host_mak
|
|
fi
|
|
|
|
echo "CONFIG_COROUTINE_BACKEND=$coroutine" >> $config_host_mak
|
|
if test "$coroutine_pool" = "yes" ; then
|
|
echo "CONFIG_COROUTINE_POOL=1" >> $config_host_mak
|
|
else
|
|
echo "CONFIG_COROUTINE_POOL=0" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$open_by_handle_at" = "yes" ; then
|
|
echo "CONFIG_OPEN_BY_HANDLE=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$linux_magic_h" = "yes" ; then
|
|
echo "CONFIG_LINUX_MAGIC_H=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$pragma_diagnostic_available" = "yes" ; then
|
|
echo "CONFIG_PRAGMA_DIAGNOSTIC_AVAILABLE=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$valgrind_h" = "yes" ; then
|
|
echo "CONFIG_VALGRIND_H=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$has_environ" = "yes" ; then
|
|
echo "CONFIG_HAS_ENVIRON=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$cpuid_h" = "yes" ; then
|
|
echo "CONFIG_CPUID_H=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$int128" = "yes" ; then
|
|
echo "CONFIG_INT128=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$getauxval" = "yes" ; then
|
|
echo "CONFIG_GETAUXVAL=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$glusterfs" = "yes" ; then
|
|
echo "CONFIG_GLUSTERFS=m" >> $config_host_mak
|
|
echo "GLUSTERFS_CFLAGS=$glusterfs_cflags" >> $config_host_mak
|
|
echo "GLUSTERFS_LIBS=$glusterfs_libs" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$glusterfs_discard" = "yes" ; then
|
|
echo "CONFIG_GLUSTERFS_DISCARD=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$glusterfs_zerofill" = "yes" ; then
|
|
echo "CONFIG_GLUSTERFS_ZEROFILL=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$archipelago" = "yes" ; then
|
|
echo "CONFIG_ARCHIPELAGO=m" >> $config_host_mak
|
|
echo "ARCHIPELAGO_LIBS=$archipelago_libs" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$libssh2" = "yes" ; then
|
|
echo "CONFIG_LIBSSH2=m" >> $config_host_mak
|
|
echo "LIBSSH2_CFLAGS=$libssh2_cflags" >> $config_host_mak
|
|
echo "LIBSSH2_LIBS=$libssh2_libs" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$quorum" = "yes" ; then
|
|
echo "CONFIG_QUORUM=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$vhdx" = "yes" ; then
|
|
echo "CONFIG_VHDX=y" >> $config_host_mak
|
|
fi
|
|
|
|
# USB host support
|
|
if test "$libusb" = "yes"; then
|
|
echo "HOST_USB=libusb legacy" >> $config_host_mak
|
|
else
|
|
echo "HOST_USB=stub" >> $config_host_mak
|
|
fi
|
|
|
|
# TPM passthrough support?
|
|
if test "$tpm" = "yes"; then
|
|
echo 'CONFIG_TPM=$(CONFIG_SOFTMMU)' >> $config_host_mak
|
|
if test "$tpm_passthrough" = "yes"; then
|
|
echo "CONFIG_TPM_PASSTHROUGH=y" >> $config_host_mak
|
|
fi
|
|
fi
|
|
|
|
echo "TRACE_BACKENDS=$trace_backends" >> $config_host_mak
|
|
if have_backend "nop"; then
|
|
echo "CONFIG_TRACE_NOP=y" >> $config_host_mak
|
|
fi
|
|
if have_backend "simple"; then
|
|
echo "CONFIG_TRACE_SIMPLE=y" >> $config_host_mak
|
|
# Set the appropriate trace file.
|
|
trace_file="\"$trace_file-\" FMT_pid"
|
|
fi
|
|
if have_backend "stderr"; then
|
|
echo "CONFIG_TRACE_STDERR=y" >> $config_host_mak
|
|
fi
|
|
if have_backend "ust"; then
|
|
echo "CONFIG_TRACE_UST=y" >> $config_host_mak
|
|
fi
|
|
if have_backend "dtrace"; then
|
|
echo "CONFIG_TRACE_DTRACE=y" >> $config_host_mak
|
|
if test "$trace_backend_stap" = "yes" ; then
|
|
echo "CONFIG_TRACE_SYSTEMTAP=y" >> $config_host_mak
|
|
fi
|
|
fi
|
|
if have_backend "ftrace"; then
|
|
if test "$linux" = "yes" ; then
|
|
echo "CONFIG_TRACE_FTRACE=y" >> $config_host_mak
|
|
else
|
|
feature_not_found "ftrace(trace backend)" "ftrace requires Linux"
|
|
fi
|
|
fi
|
|
echo "CONFIG_TRACE_FILE=$trace_file" >> $config_host_mak
|
|
|
|
if test "$rdma" = "yes" ; then
|
|
echo "CONFIG_RDMA=y" >> $config_host_mak
|
|
fi
|
|
|
|
# Hold two types of flag:
|
|
# CONFIG_THREAD_SETNAME_BYTHREAD - we've got a way of setting the name on
|
|
# a thread we have a handle to
|
|
# CONFIG_PTHREAD_SETNAME_NP - A way of doing it on a particular
|
|
# platform
|
|
if test "$pthread_setname_np" = "yes" ; then
|
|
echo "CONFIG_THREAD_SETNAME_BYTHREAD=y" >> $config_host_mak
|
|
echo "CONFIG_PTHREAD_SETNAME_NP=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$tcg_interpreter" = "yes"; then
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg/tci $QEMU_INCLUDES"
|
|
elif test "$ARCH" = "sparc64" ; then
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg/sparc $QEMU_INCLUDES"
|
|
elif test "$ARCH" = "s390x" ; then
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg/s390 $QEMU_INCLUDES"
|
|
elif test "$ARCH" = "x86_64" -o "$ARCH" = "x32" ; then
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg/i386 $QEMU_INCLUDES"
|
|
elif test "$ARCH" = "ppc64" ; then
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg/ppc $QEMU_INCLUDES"
|
|
else
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg/\$(ARCH) $QEMU_INCLUDES"
|
|
fi
|
|
QEMU_INCLUDES="-I\$(SRC_PATH)/tcg $QEMU_INCLUDES"
|
|
|
|
echo "TOOLS=$tools" >> $config_host_mak
|
|
echo "ROMS=$roms" >> $config_host_mak
|
|
echo "MAKE=$make" >> $config_host_mak
|
|
echo "INSTALL=$install" >> $config_host_mak
|
|
echo "INSTALL_DIR=$install -d -m 0755" >> $config_host_mak
|
|
echo "INSTALL_DATA=$install -c -m 0644" >> $config_host_mak
|
|
if test -n "$libtool"; then
|
|
echo "INSTALL_PROG=\$(LIBTOOL) --mode=install $install -c -m 0755" >> $config_host_mak
|
|
echo "INSTALL_LIB=\$(LIBTOOL) --mode=install $install -c -m 0644" >> $config_host_mak
|
|
else
|
|
echo "INSTALL_PROG=$install -c -m 0755" >> $config_host_mak
|
|
echo "INSTALL_LIB=$install -c -m 0644" >> $config_host_mak
|
|
fi
|
|
echo "PYTHON=$python" >> $config_host_mak
|
|
echo "CC=$cc" >> $config_host_mak
|
|
if $iasl -h > /dev/null 2>&1; then
|
|
echo "IASL=$iasl" >> $config_host_mak
|
|
fi
|
|
echo "CC_I386=$cc_i386" >> $config_host_mak
|
|
echo "HOST_CC=$host_cc" >> $config_host_mak
|
|
echo "CXX=$cxx" >> $config_host_mak
|
|
echo "OBJCC=$objcc" >> $config_host_mak
|
|
echo "AR=$ar" >> $config_host_mak
|
|
echo "ARFLAGS=$ARFLAGS" >> $config_host_mak
|
|
echo "AS=$as" >> $config_host_mak
|
|
echo "CPP=$cpp" >> $config_host_mak
|
|
echo "OBJCOPY=$objcopy" >> $config_host_mak
|
|
echo "LD=$ld" >> $config_host_mak
|
|
echo "NM=$nm" >> $config_host_mak
|
|
echo "WINDRES=$windres" >> $config_host_mak
|
|
echo "LIBTOOL=$libtool" >> $config_host_mak
|
|
echo "CFLAGS=$CFLAGS" >> $config_host_mak
|
|
echo "CFLAGS_NOPIE=$CFLAGS_NOPIE" >> $config_host_mak
|
|
echo "QEMU_CFLAGS=$QEMU_CFLAGS" >> $config_host_mak
|
|
echo "QEMU_INCLUDES=$QEMU_INCLUDES" >> $config_host_mak
|
|
if test "$sparse" = "yes" ; then
|
|
echo "CC := REAL_CC=\"\$(CC)\" cgcc" >> $config_host_mak
|
|
echo "CPP := REAL_CC=\"\$(CPP)\" cgcc" >> $config_host_mak
|
|
echo "CXX := REAL_CC=\"\$(CXX)\" cgcc" >> $config_host_mak
|
|
echo "HOST_CC := REAL_CC=\"\$(HOST_CC)\" cgcc" >> $config_host_mak
|
|
echo "QEMU_CFLAGS += -Wbitwise -Wno-transparent-union -Wno-old-initializer -Wno-non-pointer-null" >> $config_host_mak
|
|
fi
|
|
if test "$cross_prefix" != ""; then
|
|
echo "AUTOCONF_HOST := --host=${cross_prefix%-}" >> $config_host_mak
|
|
else
|
|
echo "AUTOCONF_HOST := " >> $config_host_mak
|
|
fi
|
|
echo "LDFLAGS=$LDFLAGS" >> $config_host_mak
|
|
echo "LDFLAGS_NOPIE=$LDFLAGS_NOPIE" >> $config_host_mak
|
|
echo "LIBTOOLFLAGS=$LIBTOOLFLAGS" >> $config_host_mak
|
|
echo "LIBS+=$LIBS" >> $config_host_mak
|
|
echo "LIBS_TOOLS+=$libs_tools" >> $config_host_mak
|
|
echo "EXESUF=$EXESUF" >> $config_host_mak
|
|
echo "DSOSUF=$DSOSUF" >> $config_host_mak
|
|
echo "LDFLAGS_SHARED=$LDFLAGS_SHARED" >> $config_host_mak
|
|
echo "LIBS_QGA+=$libs_qga" >> $config_host_mak
|
|
echo "POD2MAN=$POD2MAN" >> $config_host_mak
|
|
echo "TRANSLATE_OPT_CFLAGS=$TRANSLATE_OPT_CFLAGS" >> $config_host_mak
|
|
if test "$gcov" = "yes" ; then
|
|
echo "CONFIG_GCOV=y" >> $config_host_mak
|
|
echo "GCOV=$gcov_tool" >> $config_host_mak
|
|
fi
|
|
|
|
# use included Linux headers
|
|
if test "$linux" = "yes" ; then
|
|
mkdir -p linux-headers
|
|
case "$cpu" in
|
|
i386|x86_64|x32)
|
|
linux_arch=x86
|
|
;;
|
|
ppcemb|ppc|ppc64)
|
|
linux_arch=powerpc
|
|
;;
|
|
s390x)
|
|
linux_arch=s390
|
|
;;
|
|
aarch64)
|
|
linux_arch=arm64
|
|
;;
|
|
mips64)
|
|
linux_arch=mips
|
|
;;
|
|
*)
|
|
# For most CPUs the kernel architecture name and QEMU CPU name match.
|
|
linux_arch="$cpu"
|
|
;;
|
|
esac
|
|
# For non-KVM architectures we will not have asm headers
|
|
if [ -e "$source_path/linux-headers/asm-$linux_arch" ]; then
|
|
symlink "$source_path/linux-headers/asm-$linux_arch" linux-headers/asm
|
|
fi
|
|
fi
|
|
|
|
for target in $target_list; do
|
|
target_dir="$target"
|
|
config_target_mak=$target_dir/config-target.mak
|
|
target_name=`echo $target | cut -d '-' -f 1`
|
|
target_bigendian="no"
|
|
|
|
case "$target_name" in
|
|
armeb|lm32|m68k|microblaze|mips|mipsn32|mips64|moxie|or32|ppc|ppcemb|ppc64|ppc64abi32|s390x|sh4eb|sparc|sparc64|sparc32plus|xtensaeb)
|
|
target_bigendian=yes
|
|
;;
|
|
esac
|
|
target_softmmu="no"
|
|
target_user_only="no"
|
|
target_linux_user="no"
|
|
target_bsd_user="no"
|
|
case "$target" in
|
|
${target_name}-softmmu)
|
|
target_softmmu="yes"
|
|
;;
|
|
${target_name}-linux-user)
|
|
if test "$linux" != "yes" ; then
|
|
error_exit "Target '$target' is only available on a Linux host"
|
|
fi
|
|
target_user_only="yes"
|
|
target_linux_user="yes"
|
|
;;
|
|
${target_name}-bsd-user)
|
|
if test "$bsd" != "yes" ; then
|
|
error_exit "Target '$target' is only available on a BSD host"
|
|
fi
|
|
target_user_only="yes"
|
|
target_bsd_user="yes"
|
|
;;
|
|
*)
|
|
error_exit "Target '$target' not recognised"
|
|
exit 1
|
|
;;
|
|
esac
|
|
|
|
mkdir -p $target_dir
|
|
echo "# Automatically generated by configure - do not modify" > $config_target_mak
|
|
|
|
bflt="no"
|
|
interp_prefix1=`echo "$interp_prefix" | sed "s/%M/$target_name/g"`
|
|
gdb_xml_files=""
|
|
|
|
TARGET_ARCH="$target_name"
|
|
TARGET_BASE_ARCH=""
|
|
TARGET_ABI_DIR=""
|
|
|
|
case "$target_name" in
|
|
i386)
|
|
;;
|
|
x86_64)
|
|
TARGET_BASE_ARCH=i386
|
|
;;
|
|
alpha)
|
|
;;
|
|
arm|armeb)
|
|
TARGET_ARCH=arm
|
|
bflt="yes"
|
|
gdb_xml_files="arm-core.xml arm-vfp.xml arm-vfp3.xml arm-neon.xml"
|
|
;;
|
|
aarch64)
|
|
TARGET_BASE_ARCH=arm
|
|
bflt="yes"
|
|
gdb_xml_files="aarch64-core.xml aarch64-fpu.xml arm-core.xml arm-vfp.xml arm-vfp3.xml arm-neon.xml"
|
|
;;
|
|
cris)
|
|
;;
|
|
lm32)
|
|
;;
|
|
m68k)
|
|
bflt="yes"
|
|
gdb_xml_files="cf-core.xml cf-fp.xml"
|
|
;;
|
|
microblaze|microblazeel)
|
|
TARGET_ARCH=microblaze
|
|
bflt="yes"
|
|
;;
|
|
mips|mipsel)
|
|
TARGET_ARCH=mips
|
|
echo "TARGET_ABI_MIPSO32=y" >> $config_target_mak
|
|
;;
|
|
mipsn32|mipsn32el)
|
|
TARGET_ARCH=mips64
|
|
TARGET_BASE_ARCH=mips
|
|
echo "TARGET_ABI_MIPSN32=y" >> $config_target_mak
|
|
echo "TARGET_ABI32=y" >> $config_target_mak
|
|
;;
|
|
mips64|mips64el)
|
|
TARGET_ARCH=mips64
|
|
TARGET_BASE_ARCH=mips
|
|
echo "TARGET_ABI_MIPSN64=y" >> $config_target_mak
|
|
;;
|
|
moxie)
|
|
;;
|
|
or32)
|
|
TARGET_ARCH=openrisc
|
|
TARGET_BASE_ARCH=openrisc
|
|
;;
|
|
ppc)
|
|
gdb_xml_files="power-core.xml power-fpu.xml power-altivec.xml power-spe.xml"
|
|
;;
|
|
ppcemb)
|
|
TARGET_BASE_ARCH=ppc
|
|
TARGET_ABI_DIR=ppc
|
|
gdb_xml_files="power-core.xml power-fpu.xml power-altivec.xml power-spe.xml"
|
|
;;
|
|
ppc64)
|
|
TARGET_BASE_ARCH=ppc
|
|
TARGET_ABI_DIR=ppc
|
|
gdb_xml_files="power64-core.xml power-fpu.xml power-altivec.xml power-spe.xml"
|
|
;;
|
|
ppc64le)
|
|
TARGET_ARCH=ppc64
|
|
TARGET_BASE_ARCH=ppc
|
|
TARGET_ABI_DIR=ppc
|
|
gdb_xml_files="power64-core.xml power-fpu.xml power-altivec.xml power-spe.xml"
|
|
;;
|
|
ppc64abi32)
|
|
TARGET_ARCH=ppc64
|
|
TARGET_BASE_ARCH=ppc
|
|
TARGET_ABI_DIR=ppc
|
|
echo "TARGET_ABI32=y" >> $config_target_mak
|
|
gdb_xml_files="power64-core.xml power-fpu.xml power-altivec.xml power-spe.xml"
|
|
;;
|
|
sh4|sh4eb)
|
|
TARGET_ARCH=sh4
|
|
bflt="yes"
|
|
;;
|
|
sparc)
|
|
;;
|
|
sparc64)
|
|
TARGET_BASE_ARCH=sparc
|
|
;;
|
|
sparc32plus)
|
|
TARGET_ARCH=sparc64
|
|
TARGET_BASE_ARCH=sparc
|
|
TARGET_ABI_DIR=sparc
|
|
echo "TARGET_ABI32=y" >> $config_target_mak
|
|
;;
|
|
s390x)
|
|
gdb_xml_files="s390x-core64.xml s390-acr.xml s390-fpr.xml s390-vx.xml"
|
|
;;
|
|
tricore)
|
|
;;
|
|
unicore32)
|
|
;;
|
|
xtensa|xtensaeb)
|
|
TARGET_ARCH=xtensa
|
|
;;
|
|
*)
|
|
error_exit "Unsupported target CPU"
|
|
;;
|
|
esac
|
|
# TARGET_BASE_ARCH needs to be defined after TARGET_ARCH
|
|
if [ "$TARGET_BASE_ARCH" = "" ]; then
|
|
TARGET_BASE_ARCH=$TARGET_ARCH
|
|
fi
|
|
|
|
symlink "$source_path/Makefile.target" "$target_dir/Makefile"
|
|
|
|
upper() {
|
|
echo "$@"| LC_ALL=C tr '[a-z]' '[A-Z]'
|
|
}
|
|
|
|
target_arch_name="`upper $TARGET_ARCH`"
|
|
echo "TARGET_$target_arch_name=y" >> $config_target_mak
|
|
echo "TARGET_NAME=$target_name" >> $config_target_mak
|
|
echo "TARGET_BASE_ARCH=$TARGET_BASE_ARCH" >> $config_target_mak
|
|
if [ "$TARGET_ABI_DIR" = "" ]; then
|
|
TARGET_ABI_DIR=$TARGET_ARCH
|
|
fi
|
|
echo "TARGET_ABI_DIR=$TARGET_ABI_DIR" >> $config_target_mak
|
|
if [ "$HOST_VARIANT_DIR" != "" ]; then
|
|
echo "HOST_VARIANT_DIR=$HOST_VARIANT_DIR" >> $config_target_mak
|
|
fi
|
|
case "$target_name" in
|
|
i386|x86_64)
|
|
if test "$xen" = "yes" -a "$target_softmmu" = "yes" ; then
|
|
echo "CONFIG_XEN=y" >> $config_target_mak
|
|
if test "$xen_pci_passthrough" = yes; then
|
|
echo "CONFIG_XEN_PCI_PASSTHROUGH=y" >> "$config_target_mak"
|
|
fi
|
|
fi
|
|
;;
|
|
*)
|
|
esac
|
|
case "$target_name" in
|
|
aarch64|arm|i386|x86_64|ppcemb|ppc|ppc64|s390x|mipsel|mips)
|
|
# Make sure the target and host cpus are compatible
|
|
if test "$kvm" = "yes" -a "$target_softmmu" = "yes" -a \
|
|
\( "$target_name" = "$cpu" -o \
|
|
\( "$target_name" = "ppcemb" -a "$cpu" = "ppc" \) -o \
|
|
\( "$target_name" = "ppc64" -a "$cpu" = "ppc" \) -o \
|
|
\( "$target_name" = "ppc" -a "$cpu" = "ppc64" \) -o \
|
|
\( "$target_name" = "ppcemb" -a "$cpu" = "ppc64" \) -o \
|
|
\( "$target_name" = "mipsel" -a "$cpu" = "mips" \) -o \
|
|
\( "$target_name" = "x86_64" -a "$cpu" = "i386" \) -o \
|
|
\( "$target_name" = "i386" -a "$cpu" = "x86_64" \) -o \
|
|
\( "$target_name" = "x86_64" -a "$cpu" = "x32" \) -o \
|
|
\( "$target_name" = "i386" -a "$cpu" = "x32" \) \) ; then
|
|
echo "CONFIG_KVM=y" >> $config_target_mak
|
|
if test "$vhost_net" = "yes" ; then
|
|
echo "CONFIG_VHOST_NET=y" >> $config_target_mak
|
|
fi
|
|
fi
|
|
esac
|
|
if test "$target_bigendian" = "yes" ; then
|
|
echo "TARGET_WORDS_BIGENDIAN=y" >> $config_target_mak
|
|
fi
|
|
if test "$target_softmmu" = "yes" ; then
|
|
echo "CONFIG_SOFTMMU=y" >> $config_target_mak
|
|
fi
|
|
if test "$target_user_only" = "yes" ; then
|
|
echo "CONFIG_USER_ONLY=y" >> $config_target_mak
|
|
echo "CONFIG_QEMU_INTERP_PREFIX=\"$interp_prefix1\"" >> $config_target_mak
|
|
fi
|
|
if test "$target_linux_user" = "yes" ; then
|
|
echo "CONFIG_LINUX_USER=y" >> $config_target_mak
|
|
fi
|
|
list=""
|
|
if test ! -z "$gdb_xml_files" ; then
|
|
for x in $gdb_xml_files; do
|
|
list="$list $source_path/gdb-xml/$x"
|
|
done
|
|
echo "TARGET_XML_FILES=$list" >> $config_target_mak
|
|
fi
|
|
|
|
if test "$target_user_only" = "yes" -a "$bflt" = "yes"; then
|
|
echo "TARGET_HAS_BFLT=y" >> $config_target_mak
|
|
fi
|
|
if test "$target_user_only" = "yes" -a "$guest_base" = "yes"; then
|
|
echo "CONFIG_USE_GUEST_BASE=y" >> $config_target_mak
|
|
fi
|
|
if test "$target_bsd_user" = "yes" ; then
|
|
echo "CONFIG_BSD_USER=y" >> $config_target_mak
|
|
fi
|
|
|
|
# generate QEMU_CFLAGS/LDFLAGS for targets
|
|
|
|
cflags=""
|
|
ldflags=""
|
|
|
|
for i in $ARCH $TARGET_BASE_ARCH ; do
|
|
case "$i" in
|
|
alpha)
|
|
echo "CONFIG_ALPHA_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_ALPHA_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
aarch64)
|
|
if test -n "${cxx}"; then
|
|
echo "CONFIG_ARM_A64_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_ARM_A64_DIS=y" >> config-all-disas.mak
|
|
fi
|
|
;;
|
|
arm)
|
|
echo "CONFIG_ARM_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_ARM_DIS=y" >> config-all-disas.mak
|
|
if test -n "${cxx}"; then
|
|
echo "CONFIG_ARM_A64_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_ARM_A64_DIS=y" >> config-all-disas.mak
|
|
fi
|
|
;;
|
|
cris)
|
|
echo "CONFIG_CRIS_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_CRIS_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
hppa)
|
|
echo "CONFIG_HPPA_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_HPPA_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
i386|x86_64|x32)
|
|
echo "CONFIG_I386_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_I386_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
ia64*)
|
|
echo "CONFIG_IA64_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_IA64_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
lm32)
|
|
echo "CONFIG_LM32_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_LM32_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
m68k)
|
|
echo "CONFIG_M68K_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_M68K_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
microblaze*)
|
|
echo "CONFIG_MICROBLAZE_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_MICROBLAZE_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
mips*)
|
|
echo "CONFIG_MIPS_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_MIPS_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
moxie*)
|
|
echo "CONFIG_MOXIE_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_MOXIE_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
or32)
|
|
echo "CONFIG_OPENRISC_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_OPENRISC_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
ppc*)
|
|
echo "CONFIG_PPC_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_PPC_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
s390*)
|
|
echo "CONFIG_S390_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_S390_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
sh4)
|
|
echo "CONFIG_SH4_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_SH4_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
sparc*)
|
|
echo "CONFIG_SPARC_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_SPARC_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
xtensa*)
|
|
echo "CONFIG_XTENSA_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_XTENSA_DIS=y" >> config-all-disas.mak
|
|
;;
|
|
esac
|
|
done
|
|
if test "$tcg_interpreter" = "yes" ; then
|
|
echo "CONFIG_TCI_DIS=y" >> $config_target_mak
|
|
echo "CONFIG_TCI_DIS=y" >> config-all-disas.mak
|
|
fi
|
|
|
|
case "$ARCH" in
|
|
alpha)
|
|
# Ensure there's only a single GP
|
|
cflags="-msmall-data $cflags"
|
|
;;
|
|
esac
|
|
|
|
if test "$gprof" = "yes" ; then
|
|
echo "TARGET_GPROF=yes" >> $config_target_mak
|
|
if test "$target_linux_user" = "yes" ; then
|
|
cflags="-p $cflags"
|
|
ldflags="-p $ldflags"
|
|
fi
|
|
if test "$target_softmmu" = "yes" ; then
|
|
ldflags="-p $ldflags"
|
|
echo "GPROF_CFLAGS=-p" >> $config_target_mak
|
|
fi
|
|
fi
|
|
|
|
if test "$target_linux_user" = "yes" -o "$target_bsd_user" = "yes" ; then
|
|
ldflags="$ldflags $textseg_ldflags"
|
|
fi
|
|
|
|
echo "LDFLAGS+=$ldflags" >> $config_target_mak
|
|
echo "QEMU_CFLAGS+=$cflags" >> $config_target_mak
|
|
|
|
done # for target in $targets
|
|
|
|
if [ "$pixman" = "internal" ]; then
|
|
echo "config-host.h: subdir-pixman" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$rdma" = "yes" ; then
|
|
echo "CONFIG_RDMA=y" >> $config_host_mak
|
|
fi
|
|
|
|
if [ "$dtc_internal" = "yes" ]; then
|
|
echo "config-host.h: subdir-dtc" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$numa" = "yes"; then
|
|
echo "CONFIG_NUMA=y" >> $config_host_mak
|
|
fi
|
|
|
|
if test "$ccache_cpp2" = "yes"; then
|
|
echo "export CCACHE_CPP2=y" >> $config_host_mak
|
|
fi
|
|
|
|
# build tree in object directory in case the source is not in the current directory
|
|
DIRS="tests tests/tcg tests/tcg/cris tests/tcg/lm32 tests/libqos tests/qapi-schema tests/tcg/xtensa tests/qemu-iotests"
|
|
DIRS="$DIRS fsdev"
|
|
DIRS="$DIRS pc-bios/optionrom pc-bios/spapr-rtas pc-bios/s390-ccw"
|
|
DIRS="$DIRS roms/seabios roms/vgabios"
|
|
DIRS="$DIRS qapi-generated"
|
|
FILES="Makefile tests/tcg/Makefile qdict-test-data.txt"
|
|
FILES="$FILES tests/tcg/cris/Makefile tests/tcg/cris/.gdbinit"
|
|
FILES="$FILES tests/tcg/lm32/Makefile tests/tcg/xtensa/Makefile po/Makefile"
|
|
FILES="$FILES pc-bios/optionrom/Makefile pc-bios/keymaps"
|
|
FILES="$FILES pc-bios/spapr-rtas/Makefile"
|
|
FILES="$FILES pc-bios/s390-ccw/Makefile"
|
|
FILES="$FILES roms/seabios/Makefile roms/vgabios/Makefile"
|
|
FILES="$FILES pc-bios/qemu-icon.bmp"
|
|
for bios_file in \
|
|
$source_path/pc-bios/*.bin \
|
|
$source_path/pc-bios/*.aml \
|
|
$source_path/pc-bios/*.rom \
|
|
$source_path/pc-bios/*.dtb \
|
|
$source_path/pc-bios/*.img \
|
|
$source_path/pc-bios/openbios-* \
|
|
$source_path/pc-bios/u-boot.* \
|
|
$source_path/pc-bios/palcode-*
|
|
do
|
|
FILES="$FILES pc-bios/`basename $bios_file`"
|
|
done
|
|
for test_file in `find $source_path/tests/acpi-test-data -type f`
|
|
do
|
|
FILES="$FILES tests/acpi-test-data`echo $test_file | sed -e 's/.*acpi-test-data//'`"
|
|
done
|
|
mkdir -p $DIRS
|
|
for f in $FILES ; do
|
|
if [ -e "$source_path/$f" ] && [ "$pwd_is_source_path" != "y" ]; then
|
|
symlink "$source_path/$f" "$f"
|
|
fi
|
|
done
|
|
|
|
# temporary config to build submodules
|
|
for rom in seabios vgabios ; do
|
|
config_mak=roms/$rom/config.mak
|
|
echo "# Automatically generated by configure - do not modify" > $config_mak
|
|
echo "SRC_PATH=$source_path/roms/$rom" >> $config_mak
|
|
echo "AS=$as" >> $config_mak
|
|
echo "CC=$cc" >> $config_mak
|
|
echo "BCC=bcc" >> $config_mak
|
|
echo "CPP=$cpp" >> $config_mak
|
|
echo "OBJCOPY=objcopy" >> $config_mak
|
|
echo "IASL=$iasl" >> $config_mak
|
|
echo "LD=$ld" >> $config_mak
|
|
done
|
|
|
|
# set up qemu-iotests in this build directory
|
|
iotests_common_env="tests/qemu-iotests/common.env"
|
|
iotests_check="tests/qemu-iotests/check"
|
|
|
|
echo "# Automatically generated by configure - do not modify" > "$iotests_common_env"
|
|
echo >> "$iotests_common_env"
|
|
echo "export PYTHON='$python'" >> "$iotests_common_env"
|
|
|
|
if [ ! -e "$iotests_check" ]; then
|
|
symlink "$source_path/$iotests_check" "$iotests_check"
|
|
fi
|
|
|
|
# Save the configure command line for later reuse.
|
|
cat <<EOD >config.status
|
|
#!/bin/sh
|
|
# Generated by configure.
|
|
# Run this file to recreate the current configuration.
|
|
# Compiler output produced by configure, useful for debugging
|
|
# configure, is in config.log if it exists.
|
|
EOD
|
|
printf "exec" >>config.status
|
|
printf " '%s'" "$0" "$@" >>config.status
|
|
echo >>config.status
|
|
chmod +x config.status
|
|
|
|
rm -r "$TMPDIR1"
|