mirror of
https://gitlab.com/qemu-project/qemu
synced 2024-07-21 18:34:33 +00:00
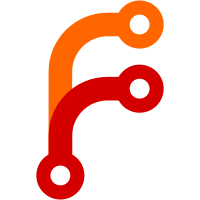
Pass an error object as the third parameter to "notifier with return" notifiers, so clients no longer need to bundle an error object in the opaque data. The new parameter is used in a later patch. Signed-off-by: Steve Sistare <steven.sistare@oracle.com> Reviewed-by: Peter Xu <peterx@redhat.com> Reviewed-by: David Hildenbrand <david@redhat.com> Link: https://lore.kernel.org/r/1708622920-68779-2-git-send-email-steven.sistare@oracle.com Signed-off-by: Peter Xu <peterx@redhat.com>
78 lines
1.8 KiB
C
78 lines
1.8 KiB
C
/*
|
|
* Notifier lists
|
|
*
|
|
* Copyright IBM, Corp. 2010
|
|
*
|
|
* Authors:
|
|
* Anthony Liguori <aliguori@us.ibm.com>
|
|
*
|
|
* This work is licensed under the terms of the GNU GPL, version 2. See
|
|
* the COPYING file in the top-level directory.
|
|
*
|
|
* Contributions after 2012-01-13 are licensed under the terms of the
|
|
* GNU GPL, version 2 or (at your option) any later version.
|
|
*/
|
|
|
|
#include "qemu/osdep.h"
|
|
#include "qemu/notify.h"
|
|
|
|
void notifier_list_init(NotifierList *list)
|
|
{
|
|
QLIST_INIT(&list->notifiers);
|
|
}
|
|
|
|
void notifier_list_add(NotifierList *list, Notifier *notifier)
|
|
{
|
|
QLIST_INSERT_HEAD(&list->notifiers, notifier, node);
|
|
}
|
|
|
|
void notifier_remove(Notifier *notifier)
|
|
{
|
|
QLIST_REMOVE(notifier, node);
|
|
}
|
|
|
|
void notifier_list_notify(NotifierList *list, void *data)
|
|
{
|
|
Notifier *notifier, *next;
|
|
|
|
QLIST_FOREACH_SAFE(notifier, &list->notifiers, node, next) {
|
|
notifier->notify(notifier, data);
|
|
}
|
|
}
|
|
|
|
bool notifier_list_empty(NotifierList *list)
|
|
{
|
|
return QLIST_EMPTY(&list->notifiers);
|
|
}
|
|
|
|
void notifier_with_return_list_init(NotifierWithReturnList *list)
|
|
{
|
|
QLIST_INIT(&list->notifiers);
|
|
}
|
|
|
|
void notifier_with_return_list_add(NotifierWithReturnList *list,
|
|
NotifierWithReturn *notifier)
|
|
{
|
|
QLIST_INSERT_HEAD(&list->notifiers, notifier, node);
|
|
}
|
|
|
|
void notifier_with_return_remove(NotifierWithReturn *notifier)
|
|
{
|
|
QLIST_REMOVE(notifier, node);
|
|
}
|
|
|
|
int notifier_with_return_list_notify(NotifierWithReturnList *list, void *data,
|
|
Error **errp)
|
|
{
|
|
NotifierWithReturn *notifier, *next;
|
|
int ret = 0;
|
|
|
|
QLIST_FOREACH_SAFE(notifier, &list->notifiers, node, next) {
|
|
ret = notifier->notify(notifier, data, errp);
|
|
if (ret != 0) {
|
|
break;
|
|
}
|
|
}
|
|
return ret;
|
|
}
|