mirror of
https://gitlab.com/qemu-project/qemu
synced 2024-07-22 02:44:53 +00:00
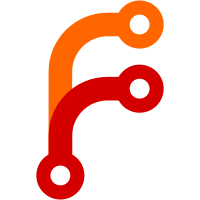
The CPU / CPU state are forward declared. $ git grep -E 'struct [A-Za-z]+CPU\ \*' target/arm/hvf_arm.h:16:void hvf_arm_set_cpu_features_from_host(struct ARMCPU *cpu); target/openrisc/cpu.h:234: int (*cpu_openrisc_map_address_code)(struct OpenRISCCPU *cpu, target/openrisc/cpu.h:238: int (*cpu_openrisc_map_address_data)(struct OpenRISCCPU *cpu, $ git grep -E 'struct CPU[A-Za-z0-9]+State\ \*' target/mips/internal.h:137: int (*map_address)(struct CPUMIPSState *env, hwaddr *physical, int *prot, target/mips/internal.h:139: void (*helper_tlbwi)(struct CPUMIPSState *env); target/mips/internal.h:140: void (*helper_tlbwr)(struct CPUMIPSState *env); target/mips/internal.h:141: void (*helper_tlbp)(struct CPUMIPSState *env); target/mips/internal.h:142: void (*helper_tlbr)(struct CPUMIPSState *env); target/mips/internal.h:143: void (*helper_tlbinv)(struct CPUMIPSState *env); target/mips/internal.h:144: void (*helper_tlbinvf)(struct CPUMIPSState *env); target/xtensa/cpu.h:347: struct CPUXtensaState *env; ... Reviewed-by: Richard Henderson <richard.henderson@linaro.org> Signed-off-by: Philippe Mathieu-Daudé <f4bug@amsat.org> Message-Id: <20220214183144.27402-12-f4bug@amsat.org>
51 lines
2 KiB
C
51 lines
2 KiB
C
/*
|
|
* Copyright (C) 2016 Veertu Inc,
|
|
* Copyright (C) 2017 Google Inc,
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef X86_EMU_H
|
|
#define X86_EMU_H
|
|
|
|
#include "x86.h"
|
|
#include "x86_decode.h"
|
|
#include "cpu.h"
|
|
|
|
void init_emu(void);
|
|
bool exec_instruction(CPUX86State *env, struct x86_decode *ins);
|
|
|
|
void load_regs(struct CPUState *cpu);
|
|
void store_regs(struct CPUState *cpu);
|
|
|
|
void simulate_rdmsr(struct CPUState *cpu);
|
|
void simulate_wrmsr(struct CPUState *cpu);
|
|
|
|
target_ulong read_reg(CPUX86State *env, int reg, int size);
|
|
void write_reg(CPUX86State *env, int reg, target_ulong val, int size);
|
|
target_ulong read_val_from_reg(target_ulong reg_ptr, int size);
|
|
void write_val_to_reg(target_ulong reg_ptr, target_ulong val, int size);
|
|
void write_val_ext(CPUX86State *env, target_ulong ptr, target_ulong val, int size);
|
|
uint8_t *read_mmio(CPUX86State *env, target_ulong ptr, int bytes);
|
|
target_ulong read_val_ext(CPUX86State *env, target_ulong ptr, int size);
|
|
|
|
void exec_movzx(CPUX86State *env, struct x86_decode *decode);
|
|
void exec_shl(CPUX86State *env, struct x86_decode *decode);
|
|
void exec_movsx(CPUX86State *env, struct x86_decode *decode);
|
|
void exec_ror(CPUX86State *env, struct x86_decode *decode);
|
|
void exec_rol(CPUX86State *env, struct x86_decode *decode);
|
|
void exec_rcl(CPUX86State *env, struct x86_decode *decode);
|
|
void exec_rcr(CPUX86State *env, struct x86_decode *decode);
|
|
#endif
|