mirror of
https://gitlab.com/qemu-project/qemu
synced 2024-09-30 00:04:37 +00:00
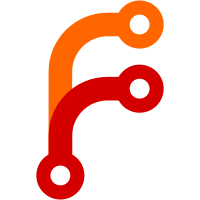
Each virtual console in the SDL2 frontend has a key state map. When switching windows with GUI keys we have to release all pressed modifier keys in the currently active window, because after the switch the now inactive window no longer receives the key release events. To reproduce the issue open a text editor in the SDL UI and then press Ctrl-Alt-2 to open a Compat Monitor Console. Close the console with the mouse. Try to enter text in the text editor and notice that the modifier keys Ctrl and Alt are stuck and need to be pressed once to be released. Tested-by: Howard Spoelstra <hsp.cat7@gmail.com> Signed-off-by: Volker Rümelin <vr_qemu@t-online.de> Tested-by: Bernhard Beschow <shentey@gmail.com> Message-ID: <20240909061552.6122-2-vr_qemu@t-online.de> Signed-off-by: Philippe Mathieu-Daudé <philmd@linaro.org>
66 lines
2.3 KiB
C
66 lines
2.3 KiB
C
/*
|
|
* QEMU SDL display driver
|
|
*
|
|
* Copyright (c) 2003 Fabrice Bellard
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
|
* of this software and associated documentation files (the "Software"), to deal
|
|
* in the Software without restriction, including without limitation the rights
|
|
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
|
* copies of the Software, and to permit persons to whom the Software is
|
|
* furnished to do so, subject to the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice shall be included in
|
|
* all copies or substantial portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
|
|
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
|
* THE SOFTWARE.
|
|
*/
|
|
/* Ported SDL 1.2 code to 2.0 by Dave Airlie. */
|
|
|
|
#include "qemu/osdep.h"
|
|
#include "ui/console.h"
|
|
#include "ui/input.h"
|
|
#include "ui/sdl2.h"
|
|
#include "trace.h"
|
|
|
|
void sdl2_process_key(struct sdl2_console *scon,
|
|
SDL_KeyboardEvent *ev)
|
|
{
|
|
int qcode;
|
|
QemuConsole *con = scon->dcl.con;
|
|
|
|
if (ev->keysym.scancode >= qemu_input_map_usb_to_qcode_len) {
|
|
return;
|
|
}
|
|
qcode = qemu_input_map_usb_to_qcode[ev->keysym.scancode];
|
|
trace_sdl2_process_key(ev->keysym.scancode, qcode,
|
|
ev->type == SDL_KEYDOWN ? "down" : "up");
|
|
qkbd_state_key_event(scon->kbd, qcode, ev->type == SDL_KEYDOWN);
|
|
|
|
if (QEMU_IS_TEXT_CONSOLE(con)) {
|
|
QemuTextConsole *s = QEMU_TEXT_CONSOLE(con);
|
|
bool ctrl = qkbd_state_modifier_get(scon->kbd, QKBD_MOD_CTRL);
|
|
if (ev->type == SDL_KEYDOWN) {
|
|
switch (qcode) {
|
|
case Q_KEY_CODE_RET:
|
|
qemu_text_console_put_keysym(s, '\n');
|
|
break;
|
|
default:
|
|
qemu_text_console_put_qcode(s, qcode, ctrl);
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
void sdl2_release_modifiers(struct sdl2_console *scon)
|
|
{
|
|
qkbd_state_lift_all_keys(scon->kbd);
|
|
}
|