mirror of
https://github.com/torvalds/linux
synced 2024-07-22 19:21:07 +00:00
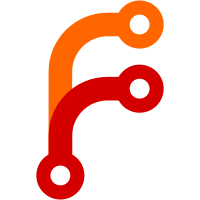
This behaves like IORING_OP_READ, except: 1) It only supports pollable files (eg pipes, sockets, etc). Note that for sockets, you probably want to use recv/recvmsg with multishot instead. 2) It supports multishot mode, meaning it will repeatedly trigger a read and fill a buffer when data is available. This allows similar use to recv/recvmsg but on non-sockets, where a single request will repeatedly post a CQE whenever data is read from it. 3) Because of #2, it must be used with provided buffers. This is uniformly true across any request type that supports multishot and transfers data, with the reason being that it's obviously not possible to pass in a single buffer for the data, as multiple reads may very well trigger before an application has a chance to process previous CQEs and the data passed from them. Reviewed-by: Gabriel Krisman Bertazi <krisman@suse.de> Signed-off-by: Jens Axboe <axboe@kernel.dk>
28 lines
921 B
C
28 lines
921 B
C
// SPDX-License-Identifier: GPL-2.0
|
|
|
|
#include <linux/pagemap.h>
|
|
|
|
struct io_rw_state {
|
|
struct iov_iter iter;
|
|
struct iov_iter_state iter_state;
|
|
struct iovec fast_iov[UIO_FASTIOV];
|
|
};
|
|
|
|
struct io_async_rw {
|
|
struct io_rw_state s;
|
|
const struct iovec *free_iovec;
|
|
size_t bytes_done;
|
|
struct wait_page_queue wpq;
|
|
};
|
|
|
|
int io_prep_rw(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_read(struct io_kiocb *req, unsigned int issue_flags);
|
|
int io_readv_prep_async(struct io_kiocb *req);
|
|
int io_write(struct io_kiocb *req, unsigned int issue_flags);
|
|
int io_writev_prep_async(struct io_kiocb *req);
|
|
void io_readv_writev_cleanup(struct io_kiocb *req);
|
|
void io_rw_fail(struct io_kiocb *req);
|
|
void io_req_rw_complete(struct io_kiocb *req, struct io_tw_state *ts);
|
|
int io_read_mshot_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_read_mshot(struct io_kiocb *req, unsigned int issue_flags);
|