mirror of
https://github.com/torvalds/linux
synced 2024-07-22 19:21:07 +00:00
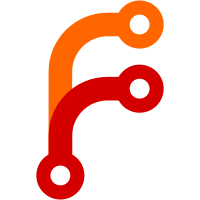
An optional counter is a driver-specific counter that may be dynamically enabled/disabled. This enhancement allows drivers to expose counters which are, for example, mutually exclusive and cannot be enabled at the same time, counters that might degrades performance, optional debug counters, etc. Optional counters are marked with IB_STAT_FLAG_OPTIONAL flag. They are not exported in sysfs, and must be at the end of all stats, otherwise the attr->show() in sysfs would get wrong indexes for hwcounters that are behind optional counters. Link: https://lore.kernel.org/r/20211008122439.166063-7-markzhang@nvidia.com Signed-off-by: Aharon Landau <aharonl@nvidia.com> Signed-off-by: Neta Ostrovsky <netao@nvidia.com> Signed-off-by: Leon Romanovsky <leonro@nvidia.com> Signed-off-by: Mark Zhang <markzhang@nvidia.com> Signed-off-by: Jason Gunthorpe <jgg@nvidia.com>
69 lines
1.9 KiB
C
69 lines
1.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 OR Linux-OpenIB */
|
|
/*
|
|
* Copyright (c) 2019 Mellanox Technologies. All rights reserved.
|
|
*/
|
|
|
|
#ifndef _RDMA_COUNTER_H_
|
|
#define _RDMA_COUNTER_H_
|
|
|
|
#include <linux/mutex.h>
|
|
#include <linux/pid_namespace.h>
|
|
|
|
#include <rdma/restrack.h>
|
|
#include <rdma/rdma_netlink.h>
|
|
|
|
struct ib_device;
|
|
struct ib_qp;
|
|
|
|
struct auto_mode_param {
|
|
int qp_type;
|
|
};
|
|
|
|
struct rdma_counter_mode {
|
|
enum rdma_nl_counter_mode mode;
|
|
enum rdma_nl_counter_mask mask;
|
|
struct auto_mode_param param;
|
|
};
|
|
|
|
struct rdma_port_counter {
|
|
struct rdma_counter_mode mode;
|
|
struct rdma_hw_stats *hstats;
|
|
unsigned int num_counters;
|
|
struct mutex lock;
|
|
};
|
|
|
|
struct rdma_counter {
|
|
struct rdma_restrack_entry res;
|
|
struct ib_device *device;
|
|
uint32_t id;
|
|
struct kref kref;
|
|
struct rdma_counter_mode mode;
|
|
struct mutex lock;
|
|
struct rdma_hw_stats *stats;
|
|
u32 port;
|
|
};
|
|
|
|
void rdma_counter_init(struct ib_device *dev);
|
|
void rdma_counter_release(struct ib_device *dev);
|
|
int rdma_counter_set_auto_mode(struct ib_device *dev, u32 port,
|
|
enum rdma_nl_counter_mask mask,
|
|
struct netlink_ext_ack *extack);
|
|
int rdma_counter_bind_qp_auto(struct ib_qp *qp, u32 port);
|
|
int rdma_counter_unbind_qp(struct ib_qp *qp, bool force);
|
|
|
|
int rdma_counter_query_stats(struct rdma_counter *counter);
|
|
u64 rdma_counter_get_hwstat_value(struct ib_device *dev, u32 port, u32 index);
|
|
int rdma_counter_bind_qpn(struct ib_device *dev, u32 port,
|
|
u32 qp_num, u32 counter_id);
|
|
int rdma_counter_bind_qpn_alloc(struct ib_device *dev, u32 port,
|
|
u32 qp_num, u32 *counter_id);
|
|
int rdma_counter_unbind_qpn(struct ib_device *dev, u32 port,
|
|
u32 qp_num, u32 counter_id);
|
|
int rdma_counter_get_mode(struct ib_device *dev, u32 port,
|
|
enum rdma_nl_counter_mode *mode,
|
|
enum rdma_nl_counter_mask *mask);
|
|
|
|
int rdma_counter_modify(struct ib_device *dev, u32 port,
|
|
unsigned int index, bool enable);
|
|
#endif /* _RDMA_COUNTER_H_ */
|