mirror of
https://github.com/torvalds/linux
synced 2024-07-22 11:10:46 +00:00
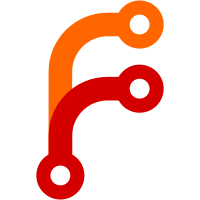
Each peer's endpoint contains a dst_cache entry that takes a reference
to another netdev. When the containing namespace exits, we take down the
socket and prevent future sockets from being created (by setting
creating_net to NULL), which removes that potential reference on the
netns. However, it doesn't release references to the netns that a netdev
cached in dst_cache might be taking, so the netns still might fail to
exit. Since the socket is gimped anyway, we can simply clear all the
dst_caches (by way of clearing the endpoint src), which will release all
references.
However, the current dst_cache_reset function only releases those
references lazily. But it turns out that all of our usages of
wg_socket_clear_peer_endpoint_src are called from contexts that are not
exactly high-speed or bottle-necked. For example, when there's
connection difficulty, or when userspace is reconfiguring the interface.
And in particular for this patch, when the netns is exiting. So for
those cases, it makes more sense to call dst_release immediately. For
that, we add a small helper function to dst_cache.
This patch also adds a test to netns.sh from Hangbin Liu to ensure this
doesn't regress.
Tested-by: Hangbin Liu <liuhangbin@gmail.com>
Reported-by: Xiumei Mu <xmu@redhat.com>
Cc: Toke Høiland-Jørgensen <toke@redhat.com>
Cc: Paolo Abeni <pabeni@redhat.com>
Fixes: 900575aa33
("wireguard: device: avoid circular netns references")
Signed-off-by: Jason A. Donenfeld <Jason@zx2c4.com>
Signed-off-by: Jakub Kicinski <kuba@kernel.org>
110 lines
3 KiB
C
110 lines
3 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _NET_DST_CACHE_H
|
|
#define _NET_DST_CACHE_H
|
|
|
|
#include <linux/jiffies.h>
|
|
#include <net/dst.h>
|
|
#if IS_ENABLED(CONFIG_IPV6)
|
|
#include <net/ip6_fib.h>
|
|
#endif
|
|
|
|
struct dst_cache {
|
|
struct dst_cache_pcpu __percpu *cache;
|
|
unsigned long reset_ts;
|
|
};
|
|
|
|
/**
|
|
* dst_cache_get - perform cache lookup
|
|
* @dst_cache: the cache
|
|
*
|
|
* The caller should use dst_cache_get_ip4() if it need to retrieve the
|
|
* source address to be used when xmitting to the cached dst.
|
|
* local BH must be disabled.
|
|
*/
|
|
struct dst_entry *dst_cache_get(struct dst_cache *dst_cache);
|
|
|
|
/**
|
|
* dst_cache_get_ip4 - perform cache lookup and fetch ipv4 source address
|
|
* @dst_cache: the cache
|
|
* @saddr: return value for the retrieved source address
|
|
*
|
|
* local BH must be disabled.
|
|
*/
|
|
struct rtable *dst_cache_get_ip4(struct dst_cache *dst_cache, __be32 *saddr);
|
|
|
|
/**
|
|
* dst_cache_set_ip4 - store the ipv4 dst into the cache
|
|
* @dst_cache: the cache
|
|
* @dst: the entry to be cached
|
|
* @saddr: the source address to be stored inside the cache
|
|
*
|
|
* local BH must be disabled.
|
|
*/
|
|
void dst_cache_set_ip4(struct dst_cache *dst_cache, struct dst_entry *dst,
|
|
__be32 saddr);
|
|
|
|
#if IS_ENABLED(CONFIG_IPV6)
|
|
|
|
/**
|
|
* dst_cache_set_ip6 - store the ipv6 dst into the cache
|
|
* @dst_cache: the cache
|
|
* @dst: the entry to be cached
|
|
* @saddr: the source address to be stored inside the cache
|
|
*
|
|
* local BH must be disabled.
|
|
*/
|
|
void dst_cache_set_ip6(struct dst_cache *dst_cache, struct dst_entry *dst,
|
|
const struct in6_addr *saddr);
|
|
|
|
/**
|
|
* dst_cache_get_ip6 - perform cache lookup and fetch ipv6 source address
|
|
* @dst_cache: the cache
|
|
* @saddr: return value for the retrieved source address
|
|
*
|
|
* local BH must be disabled.
|
|
*/
|
|
struct dst_entry *dst_cache_get_ip6(struct dst_cache *dst_cache,
|
|
struct in6_addr *saddr);
|
|
#endif
|
|
|
|
/**
|
|
* dst_cache_reset - invalidate the cache contents
|
|
* @dst_cache: the cache
|
|
*
|
|
* This does not free the cached dst to avoid races and contentions.
|
|
* the dst will be freed on later cache lookup.
|
|
*/
|
|
static inline void dst_cache_reset(struct dst_cache *dst_cache)
|
|
{
|
|
dst_cache->reset_ts = jiffies;
|
|
}
|
|
|
|
/**
|
|
* dst_cache_reset_now - invalidate the cache contents immediately
|
|
* @dst_cache: the cache
|
|
*
|
|
* The caller must be sure there are no concurrent users, as this frees
|
|
* all dst_cache users immediately, rather than waiting for the next
|
|
* per-cpu usage like dst_cache_reset does. Most callers should use the
|
|
* higher speed lazily-freed dst_cache_reset function instead.
|
|
*/
|
|
void dst_cache_reset_now(struct dst_cache *dst_cache);
|
|
|
|
/**
|
|
* dst_cache_init - initialize the cache, allocating the required storage
|
|
* @dst_cache: the cache
|
|
* @gfp: allocation flags
|
|
*/
|
|
int dst_cache_init(struct dst_cache *dst_cache, gfp_t gfp);
|
|
|
|
/**
|
|
* dst_cache_destroy - empty the cache and free the allocated storage
|
|
* @dst_cache: the cache
|
|
*
|
|
* No synchronization is enforced: it must be called only when the cache
|
|
* is unsed.
|
|
*/
|
|
void dst_cache_destroy(struct dst_cache *dst_cache);
|
|
|
|
#endif
|