mirror of
https://github.com/torvalds/linux
synced 2024-07-22 19:21:07 +00:00
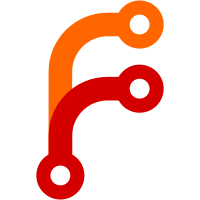
gcc-11 points out a mismatch between the declaration and the definition
of poly1305_core_setkey():
lib/crypto/poly1305-donna32.c:13:67: error: argument 2 of type ‘const u8[16]’ {aka ‘const unsigned char[16]’} with mismatched bound [-Werror=array-parameter=]
13 | void poly1305_core_setkey(struct poly1305_core_key *key, const u8 raw_key[16])
| ~~~~~~~~~^~~~~~~~~~~
In file included from lib/crypto/poly1305-donna32.c:11:
include/crypto/internal/poly1305.h:21:68: note: previously declared as ‘const u8 *’ {aka ‘const unsigned char *’}
21 | void poly1305_core_setkey(struct poly1305_core_key *key, const u8 *raw_key);
This is harmless in principle, as the calling conventions are the same,
but the more specific prototype allows better type checking in the
caller.
Change the declaration to match the actual function definition.
The poly1305_simd_init() is a bit suspicious here, as it previously
had a 32-byte argument type, but looks like it needs to take the
16-byte POLY1305_BLOCK_SIZE array instead.
Fixes: 1c08a10436
("crypto: poly1305 - add new 32 and 64-bit generic versions")
Signed-off-by: Arnd Bergmann <arnd@arndb.de>
Reviewed-by: Ard Biesheuvel <ardb@kernel.org>
Reviewed-by: Eric Biggers <ebiggers@google.com>
Signed-off-by: Herbert Xu <herbert@gondor.apana.org.au>
100 lines
2.4 KiB
C
100 lines
2.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* Common values for the Poly1305 algorithm
|
|
*/
|
|
|
|
#ifndef _CRYPTO_POLY1305_H
|
|
#define _CRYPTO_POLY1305_H
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/crypto.h>
|
|
|
|
#define POLY1305_BLOCK_SIZE 16
|
|
#define POLY1305_KEY_SIZE 32
|
|
#define POLY1305_DIGEST_SIZE 16
|
|
|
|
/* The poly1305_key and poly1305_state types are mostly opaque and
|
|
* implementation-defined. Limbs might be in base 2^64 or base 2^26, or
|
|
* different yet. The union type provided keeps these 64-bit aligned for the
|
|
* case in which this is implemented using 64x64 multiplies.
|
|
*/
|
|
|
|
struct poly1305_key {
|
|
union {
|
|
u32 r[5];
|
|
u64 r64[3];
|
|
};
|
|
};
|
|
|
|
struct poly1305_core_key {
|
|
struct poly1305_key key;
|
|
struct poly1305_key precomputed_s;
|
|
};
|
|
|
|
struct poly1305_state {
|
|
union {
|
|
u32 h[5];
|
|
u64 h64[3];
|
|
};
|
|
};
|
|
|
|
struct poly1305_desc_ctx {
|
|
/* partial buffer */
|
|
u8 buf[POLY1305_BLOCK_SIZE];
|
|
/* bytes used in partial buffer */
|
|
unsigned int buflen;
|
|
/* how many keys have been set in r[] */
|
|
unsigned short rset;
|
|
/* whether s[] has been set */
|
|
bool sset;
|
|
/* finalize key */
|
|
u32 s[4];
|
|
/* accumulator */
|
|
struct poly1305_state h;
|
|
/* key */
|
|
union {
|
|
struct poly1305_key opaque_r[CONFIG_CRYPTO_LIB_POLY1305_RSIZE];
|
|
struct poly1305_core_key core_r;
|
|
};
|
|
};
|
|
|
|
void poly1305_init_arch(struct poly1305_desc_ctx *desc,
|
|
const u8 key[POLY1305_KEY_SIZE]);
|
|
void poly1305_init_generic(struct poly1305_desc_ctx *desc,
|
|
const u8 key[POLY1305_KEY_SIZE]);
|
|
|
|
static inline void poly1305_init(struct poly1305_desc_ctx *desc, const u8 *key)
|
|
{
|
|
if (IS_ENABLED(CONFIG_CRYPTO_ARCH_HAVE_LIB_POLY1305))
|
|
poly1305_init_arch(desc, key);
|
|
else
|
|
poly1305_init_generic(desc, key);
|
|
}
|
|
|
|
void poly1305_update_arch(struct poly1305_desc_ctx *desc, const u8 *src,
|
|
unsigned int nbytes);
|
|
void poly1305_update_generic(struct poly1305_desc_ctx *desc, const u8 *src,
|
|
unsigned int nbytes);
|
|
|
|
static inline void poly1305_update(struct poly1305_desc_ctx *desc,
|
|
const u8 *src, unsigned int nbytes)
|
|
{
|
|
if (IS_ENABLED(CONFIG_CRYPTO_ARCH_HAVE_LIB_POLY1305))
|
|
poly1305_update_arch(desc, src, nbytes);
|
|
else
|
|
poly1305_update_generic(desc, src, nbytes);
|
|
}
|
|
|
|
void poly1305_final_arch(struct poly1305_desc_ctx *desc, u8 *digest);
|
|
void poly1305_final_generic(struct poly1305_desc_ctx *desc, u8 *digest);
|
|
|
|
static inline void poly1305_final(struct poly1305_desc_ctx *desc, u8 *digest)
|
|
{
|
|
if (IS_ENABLED(CONFIG_CRYPTO_ARCH_HAVE_LIB_POLY1305))
|
|
poly1305_final_arch(desc, digest);
|
|
else
|
|
poly1305_final_generic(desc, digest);
|
|
}
|
|
|
|
#endif
|