mirror of
https://github.com/torvalds/linux
synced 2024-10-22 03:09:41 +00:00
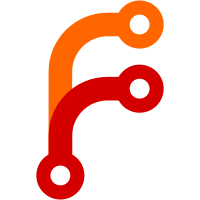
SCIF0 and SCIF1 are used as debug serial ports. Enable them and configure pinmuxing appropriately. We can now remove the clkdev registration hack for SCIF devices from the Lager reference board file. Signed-off-by: Laurent Pinchart <laurent.pinchart+renesas@ideasonboard.com> [horms+renesas@verge.net.au: updated changelog to remove references to device renaming] [horms+renesas@verge.net.au: resolved conflicts] Signed-off-by: Simon Horman <horms+renesas@verge.net.au>
138 lines
3.8 KiB
C
138 lines
3.8 KiB
C
/*
|
|
* Lager board support - Reference DT implementation
|
|
*
|
|
* Copyright (C) 2013 Renesas Solutions Corp.
|
|
* Copyright (C) 2013 Simon Horman
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; version 2 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#include <linux/dma-mapping.h>
|
|
#include <linux/init.h>
|
|
#include <linux/of_platform.h>
|
|
#include <linux/platform_data/rcar-du.h>
|
|
#include <mach/clock.h>
|
|
#include <mach/common.h>
|
|
#include <mach/irqs.h>
|
|
#include <mach/rcar-gen2.h>
|
|
#include <mach/r8a7790.h>
|
|
#include <asm/mach/arch.h>
|
|
|
|
/* DU */
|
|
static struct rcar_du_encoder_data lager_du_encoders[] = {
|
|
{
|
|
.type = RCAR_DU_ENCODER_VGA,
|
|
.output = RCAR_DU_OUTPUT_DPAD0,
|
|
}, {
|
|
.type = RCAR_DU_ENCODER_NONE,
|
|
.output = RCAR_DU_OUTPUT_LVDS1,
|
|
.connector.lvds.panel = {
|
|
.width_mm = 210,
|
|
.height_mm = 158,
|
|
.mode = {
|
|
.clock = 65000,
|
|
.hdisplay = 1024,
|
|
.hsync_start = 1048,
|
|
.hsync_end = 1184,
|
|
.htotal = 1344,
|
|
.vdisplay = 768,
|
|
.vsync_start = 771,
|
|
.vsync_end = 777,
|
|
.vtotal = 806,
|
|
.flags = 0,
|
|
},
|
|
},
|
|
},
|
|
};
|
|
|
|
static struct rcar_du_platform_data lager_du_pdata = {
|
|
.encoders = lager_du_encoders,
|
|
.num_encoders = ARRAY_SIZE(lager_du_encoders),
|
|
};
|
|
|
|
static const struct resource du_resources[] __initconst = {
|
|
DEFINE_RES_MEM(0xfeb00000, 0x70000),
|
|
DEFINE_RES_MEM_NAMED(0xfeb90000, 0x1c, "lvds.0"),
|
|
DEFINE_RES_MEM_NAMED(0xfeb94000, 0x1c, "lvds.1"),
|
|
DEFINE_RES_IRQ(gic_spi(256)),
|
|
DEFINE_RES_IRQ(gic_spi(268)),
|
|
DEFINE_RES_IRQ(gic_spi(269)),
|
|
};
|
|
|
|
static void __init lager_add_du_device(void)
|
|
{
|
|
struct platform_device_info info = {
|
|
.name = "rcar-du-r8a7790",
|
|
.id = -1,
|
|
.res = du_resources,
|
|
.num_res = ARRAY_SIZE(du_resources),
|
|
.data = &lager_du_pdata,
|
|
.size_data = sizeof(lager_du_pdata),
|
|
.dma_mask = DMA_BIT_MASK(32),
|
|
};
|
|
|
|
platform_device_register_full(&info);
|
|
}
|
|
|
|
/*
|
|
* This is a really crude hack to provide clkdev support to platform
|
|
* devices until they get moved to DT.
|
|
*/
|
|
static const struct clk_name clk_names[] __initconst = {
|
|
{ "cmt0", "fck", "sh-cmt-48-gen2.0" },
|
|
{ "du0", "du.0", "rcar-du-r8a7790" },
|
|
{ "du1", "du.1", "rcar-du-r8a7790" },
|
|
{ "du2", "du.2", "rcar-du-r8a7790" },
|
|
{ "lvds0", "lvds.0", "rcar-du-r8a7790" },
|
|
{ "lvds1", "lvds.1", "rcar-du-r8a7790" },
|
|
};
|
|
|
|
/*
|
|
* This is a really crude hack to work around core platform clock issues
|
|
*/
|
|
static const struct clk_name clk_enables[] __initconst = {
|
|
{ "ether", NULL, "ee700000.ethernet" },
|
|
{ "msiof1", NULL, "e6e10000.spi" },
|
|
{ "mmcif1", NULL, "ee220000.mmc" },
|
|
{ "qspi_mod", NULL, "e6b10000.spi" },
|
|
{ "sdhi0", NULL, "ee100000.sd" },
|
|
{ "sdhi2", NULL, "ee140000.sd" },
|
|
{ "thermal", NULL, "e61f0000.thermal" },
|
|
};
|
|
|
|
static void __init lager_add_standard_devices(void)
|
|
{
|
|
shmobile_clk_workaround(clk_names, ARRAY_SIZE(clk_names), false);
|
|
shmobile_clk_workaround(clk_enables, ARRAY_SIZE(clk_enables), true);
|
|
r8a7790_add_dt_devices();
|
|
of_platform_populate(NULL, of_default_bus_match_table, NULL, NULL);
|
|
|
|
lager_add_du_device();
|
|
}
|
|
|
|
static const char *lager_boards_compat_dt[] __initdata = {
|
|
"renesas,lager",
|
|
"renesas,lager-reference",
|
|
NULL,
|
|
};
|
|
|
|
DT_MACHINE_START(LAGER_DT, "lager")
|
|
.smp = smp_ops(r8a7790_smp_ops),
|
|
.init_early = r8a7790_init_early,
|
|
.init_time = rcar_gen2_timer_init,
|
|
.init_machine = lager_add_standard_devices,
|
|
.init_late = shmobile_init_late,
|
|
.dt_compat = lager_boards_compat_dt,
|
|
MACHINE_END
|