mirror of
https://github.com/torvalds/linux
synced 2024-09-23 12:56:39 +00:00
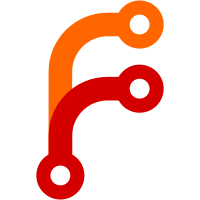
Currently we disable preemption in copy_to_user_page; a behaviour that we inherited from the 32-bit arm code. This was necessary for older cores without broadcast data cache maintenance, and ensured that cache lines were dirtied and cleaned by the same CPU. On these systems dirty cache line migration was not possible, so this was sufficient to guarantee coherency. On contemporary systems, cache coherence protocols permit (dirty) cache lines to migrate between CPUs as a result of speculation, prefetching, and other behaviours. To account for this, in ARMv8 data cache maintenance operations are broadcast and affect all data caches in the domain associated with the VA (i.e. ISH for kernel and user mappings). In __switch_to we ensure that tasks can be safely migrated in the middle of a maintenance sequence, using a dsb(ish) to ensure prior explicit memory accesses are observed and cache maintenance operations are completed before a task can be run on another CPU. Given the above, it is not necessary to disable preemption in copy_to_user_page. This patch removes the preempt_{disable,enable} calls, permitting preemption. Signed-off-by: Mark Rutland <mark.rutland@arm.com> Cc: Will Deacon <will.deacon@arm.com> Signed-off-by: Catalin Marinas <catalin.marinas@arm.com>
101 lines
2.7 KiB
C
101 lines
2.7 KiB
C
/*
|
|
* Based on arch/arm/mm/flush.c
|
|
*
|
|
* Copyright (C) 1995-2002 Russell King
|
|
* Copyright (C) 2012 ARM Ltd.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <linux/export.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/pagemap.h>
|
|
|
|
#include <asm/cacheflush.h>
|
|
#include <asm/cachetype.h>
|
|
#include <asm/tlbflush.h>
|
|
|
|
#include "mm.h"
|
|
|
|
void flush_cache_range(struct vm_area_struct *vma, unsigned long start,
|
|
unsigned long end)
|
|
{
|
|
if (vma->vm_flags & VM_EXEC)
|
|
__flush_icache_all();
|
|
}
|
|
|
|
static void sync_icache_aliases(void *kaddr, unsigned long len)
|
|
{
|
|
unsigned long addr = (unsigned long)kaddr;
|
|
|
|
if (icache_is_aliasing()) {
|
|
__clean_dcache_area_pou(kaddr, len);
|
|
__flush_icache_all();
|
|
} else {
|
|
flush_icache_range(addr, addr + len);
|
|
}
|
|
}
|
|
|
|
static void flush_ptrace_access(struct vm_area_struct *vma, struct page *page,
|
|
unsigned long uaddr, void *kaddr,
|
|
unsigned long len)
|
|
{
|
|
if (vma->vm_flags & VM_EXEC)
|
|
sync_icache_aliases(kaddr, len);
|
|
}
|
|
|
|
/*
|
|
* Copy user data from/to a page which is mapped into a different processes
|
|
* address space. Really, we want to allow our "user space" model to handle
|
|
* this.
|
|
*/
|
|
void copy_to_user_page(struct vm_area_struct *vma, struct page *page,
|
|
unsigned long uaddr, void *dst, const void *src,
|
|
unsigned long len)
|
|
{
|
|
memcpy(dst, src, len);
|
|
flush_ptrace_access(vma, page, uaddr, dst, len);
|
|
}
|
|
|
|
void __sync_icache_dcache(pte_t pte, unsigned long addr)
|
|
{
|
|
struct page *page = pte_page(pte);
|
|
|
|
/* no flushing needed for anonymous pages */
|
|
if (!page_mapping(page))
|
|
return;
|
|
|
|
if (!test_and_set_bit(PG_dcache_clean, &page->flags))
|
|
sync_icache_aliases(page_address(page),
|
|
PAGE_SIZE << compound_order(page));
|
|
else if (icache_is_aivivt())
|
|
__flush_icache_all();
|
|
}
|
|
|
|
/*
|
|
* This function is called when a page has been modified by the kernel. Mark
|
|
* it as dirty for later flushing when mapped in user space (if executable,
|
|
* see __sync_icache_dcache).
|
|
*/
|
|
void flush_dcache_page(struct page *page)
|
|
{
|
|
if (test_bit(PG_dcache_clean, &page->flags))
|
|
clear_bit(PG_dcache_clean, &page->flags);
|
|
}
|
|
EXPORT_SYMBOL(flush_dcache_page);
|
|
|
|
/*
|
|
* Additional functions defined in assembly.
|
|
*/
|
|
EXPORT_SYMBOL(flush_icache_range);
|