mirror of
https://github.com/torvalds/linux
synced 2024-09-22 12:26:27 +00:00
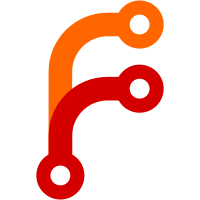
Rewrite the vmap allocator to use rbtrees and lazy tlb flushing, and provide a fast, scalable percpu frontend for small vmaps (requires a slightly different API, though). The biggest problem with vmap is actually vunmap. Presently this requires a global kernel TLB flush, which on most architectures is a broadcast IPI to all CPUs to flush the cache. This is all done under a global lock. As the number of CPUs increases, so will the number of vunmaps a scaled workload will want to perform, and so will the cost of a global TLB flush. This gives terrible quadratic scalability characteristics. Another problem is that the entire vmap subsystem works under a single lock. It is a rwlock, but it is actually taken for write in all the fast paths, and the read locking would likely never be run concurrently anyway, so it's just pointless. This is a rewrite of vmap subsystem to solve those problems. The existing vmalloc API is implemented on top of the rewritten subsystem. The TLB flushing problem is solved by using lazy TLB unmapping. vmap addresses do not have to be flushed immediately when they are vunmapped, because the kernel will not reuse them again (would be a use-after-free) until they are reallocated. So the addresses aren't allocated again until a subsequent TLB flush. A single TLB flush then can flush multiple vunmaps from each CPU. XEN and PAT and such do not like deferred TLB flushing because they can't always handle multiple aliasing virtual addresses to a physical address. They now call vm_unmap_aliases() in order to flush any deferred mappings. That call is very expensive (well, actually not a lot more expensive than a single vunmap under the old scheme), however it should be OK if not called too often. The virtual memory extent information is stored in an rbtree rather than a linked list to improve the algorithmic scalability. There is a per-CPU allocator for small vmaps, which amortizes or avoids global locking. To use the per-CPU interface, the vm_map_ram / vm_unmap_ram interfaces must be used in place of vmap and vunmap. Vmalloc does not use these interfaces at the moment, so it will not be quite so scalable (although it will use lazy TLB flushing). As a quick test of performance, I ran a test that loops in the kernel, linearly mapping then touching then unmapping 4 pages. Different numbers of tests were run in parallel on an 4 core, 2 socket opteron. Results are in nanoseconds per map+touch+unmap. threads vanilla vmap rewrite 1 14700 2900 2 33600 3000 4 49500 2800 8 70631 2900 So with a 8 cores, the rewritten version is already 25x faster. In a slightly more realistic test (although with an older and less scalable version of the patch), I ripped the not-very-good vunmap batching code out of XFS, and implemented the large buffer mapping with vm_map_ram and vm_unmap_ram... along with a couple of other tricks, I was able to speed up a large directory workload by 20x on a 64 CPU system. I believe vmap/vunmap is actually sped up a lot more than 20x on such a system, but I'm running into other locks now. vmap is pretty well blown off the profiles. Before: 1352059 total 0.1401 798784 _write_lock 8320.6667 <- vmlist_lock 529313 default_idle 1181.5022 15242 smp_call_function 15.8771 <- vmap tlb flushing 2472 __get_vm_area_node 1.9312 <- vmap 1762 remove_vm_area 4.5885 <- vunmap 316 map_vm_area 0.2297 <- vmap 312 kfree 0.1950 300 _spin_lock 3.1250 252 sn_send_IPI_phys 0.4375 <- tlb flushing 238 vmap 0.8264 <- vmap 216 find_lock_page 0.5192 196 find_next_bit 0.3603 136 sn2_send_IPI 0.2024 130 pio_phys_write_mmr 2.0312 118 unmap_kernel_range 0.1229 After: 78406 total 0.0081 40053 default_idle 89.4040 33576 ia64_spinlock_contention 349.7500 1650 _spin_lock 17.1875 319 __reg_op 0.5538 281 _atomic_dec_and_lock 1.0977 153 mutex_unlock 1.5938 123 iget_locked 0.1671 117 xfs_dir_lookup 0.1662 117 dput 0.1406 114 xfs_iget_core 0.0268 92 xfs_da_hashname 0.1917 75 d_alloc 0.0670 68 vmap_page_range 0.0462 <- vmap 58 kmem_cache_alloc 0.0604 57 memset 0.0540 52 rb_next 0.1625 50 __copy_user 0.0208 49 bitmap_find_free_region 0.2188 <- vmap 46 ia64_sn_udelay 0.1106 45 find_inode_fast 0.1406 42 memcmp 0.2188 42 finish_task_switch 0.1094 42 __d_lookup 0.0410 40 radix_tree_lookup_slot 0.1250 37 _spin_unlock_irqrestore 0.3854 36 xfs_bmapi 0.0050 36 kmem_cache_free 0.0256 35 xfs_vn_getattr 0.0322 34 radix_tree_lookup 0.1062 33 __link_path_walk 0.0035 31 xfs_da_do_buf 0.0091 30 _xfs_buf_find 0.0204 28 find_get_page 0.0875 27 xfs_iread 0.0241 27 __strncpy_from_user 0.2812 26 _xfs_buf_initialize 0.0406 24 _xfs_buf_lookup_pages 0.0179 24 vunmap_page_range 0.0250 <- vunmap 23 find_lock_page 0.0799 22 vm_map_ram 0.0087 <- vmap 20 kfree 0.0125 19 put_page 0.0330 18 __kmalloc 0.0176 17 xfs_da_node_lookup_int 0.0086 17 _read_lock 0.0885 17 page_waitqueue 0.0664 vmap has gone from being the top 5 on the profiles and flushing the crap out of all TLBs, to using less than 1% of kernel time. [akpm@linux-foundation.org: cleanups, section fix] [akpm@linux-foundation.org: fix build on alpha] Signed-off-by: Nick Piggin <npiggin@suse.de> Cc: Jeremy Fitzhardinge <jeremy@goop.org> Cc: Krzysztof Helt <krzysztof.h1@poczta.fm> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
889 lines
21 KiB
C
889 lines
21 KiB
C
/*
|
|
* linux/init/main.c
|
|
*
|
|
* Copyright (C) 1991, 1992 Linus Torvalds
|
|
*
|
|
* GK 2/5/95 - Changed to support mounting root fs via NFS
|
|
* Added initrd & change_root: Werner Almesberger & Hans Lermen, Feb '96
|
|
* Moan early if gcc is old, avoiding bogus kernels - Paul Gortmaker, May '96
|
|
* Simplified starting of init: Michael A. Griffith <grif@acm.org>
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/module.h>
|
|
#include <linux/proc_fs.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/syscalls.h>
|
|
#include <linux/string.h>
|
|
#include <linux/ctype.h>
|
|
#include <linux/delay.h>
|
|
#include <linux/utsname.h>
|
|
#include <linux/ioport.h>
|
|
#include <linux/init.h>
|
|
#include <linux/smp_lock.h>
|
|
#include <linux/initrd.h>
|
|
#include <linux/bootmem.h>
|
|
#include <linux/tty.h>
|
|
#include <linux/gfp.h>
|
|
#include <linux/percpu.h>
|
|
#include <linux/kmod.h>
|
|
#include <linux/vmalloc.h>
|
|
#include <linux/kernel_stat.h>
|
|
#include <linux/start_kernel.h>
|
|
#include <linux/security.h>
|
|
#include <linux/smp.h>
|
|
#include <linux/workqueue.h>
|
|
#include <linux/profile.h>
|
|
#include <linux/rcupdate.h>
|
|
#include <linux/moduleparam.h>
|
|
#include <linux/kallsyms.h>
|
|
#include <linux/writeback.h>
|
|
#include <linux/cpu.h>
|
|
#include <linux/cpuset.h>
|
|
#include <linux/cgroup.h>
|
|
#include <linux/efi.h>
|
|
#include <linux/tick.h>
|
|
#include <linux/interrupt.h>
|
|
#include <linux/taskstats_kern.h>
|
|
#include <linux/delayacct.h>
|
|
#include <linux/unistd.h>
|
|
#include <linux/rmap.h>
|
|
#include <linux/mempolicy.h>
|
|
#include <linux/key.h>
|
|
#include <linux/unwind.h>
|
|
#include <linux/buffer_head.h>
|
|
#include <linux/debug_locks.h>
|
|
#include <linux/debugobjects.h>
|
|
#include <linux/lockdep.h>
|
|
#include <linux/pid_namespace.h>
|
|
#include <linux/device.h>
|
|
#include <linux/kthread.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/signal.h>
|
|
#include <linux/idr.h>
|
|
|
|
#include <asm/io.h>
|
|
#include <asm/bugs.h>
|
|
#include <asm/setup.h>
|
|
#include <asm/sections.h>
|
|
#include <asm/cacheflush.h>
|
|
|
|
#ifdef CONFIG_X86_LOCAL_APIC
|
|
#include <asm/smp.h>
|
|
#endif
|
|
|
|
/*
|
|
* This is one of the first .c files built. Error out early if we have compiler
|
|
* trouble.
|
|
*/
|
|
|
|
#if __GNUC__ == 4 && __GNUC_MINOR__ == 1 && __GNUC_PATCHLEVEL__ == 0
|
|
#warning gcc-4.1.0 is known to miscompile the kernel. A different compiler version is recommended.
|
|
#endif
|
|
|
|
static int kernel_init(void *);
|
|
|
|
extern void init_IRQ(void);
|
|
extern void fork_init(unsigned long);
|
|
extern void mca_init(void);
|
|
extern void sbus_init(void);
|
|
extern void prio_tree_init(void);
|
|
extern void radix_tree_init(void);
|
|
extern void free_initmem(void);
|
|
#ifdef CONFIG_ACPI
|
|
extern void acpi_early_init(void);
|
|
#else
|
|
static inline void acpi_early_init(void) { }
|
|
#endif
|
|
#ifndef CONFIG_DEBUG_RODATA
|
|
static inline void mark_rodata_ro(void) { }
|
|
#endif
|
|
|
|
#ifdef CONFIG_TC
|
|
extern void tc_init(void);
|
|
#endif
|
|
|
|
enum system_states system_state;
|
|
EXPORT_SYMBOL(system_state);
|
|
|
|
/*
|
|
* Boot command-line arguments
|
|
*/
|
|
#define MAX_INIT_ARGS CONFIG_INIT_ENV_ARG_LIMIT
|
|
#define MAX_INIT_ENVS CONFIG_INIT_ENV_ARG_LIMIT
|
|
|
|
extern void time_init(void);
|
|
/* Default late time init is NULL. archs can override this later. */
|
|
void (*late_time_init)(void);
|
|
extern void softirq_init(void);
|
|
|
|
/* Untouched command line saved by arch-specific code. */
|
|
char __initdata boot_command_line[COMMAND_LINE_SIZE];
|
|
/* Untouched saved command line (eg. for /proc) */
|
|
char *saved_command_line;
|
|
/* Command line for parameter parsing */
|
|
static char *static_command_line;
|
|
|
|
static char *execute_command;
|
|
static char *ramdisk_execute_command;
|
|
|
|
#ifdef CONFIG_SMP
|
|
/* Setup configured maximum number of CPUs to activate */
|
|
unsigned int __initdata setup_max_cpus = NR_CPUS;
|
|
|
|
/*
|
|
* Setup routine for controlling SMP activation
|
|
*
|
|
* Command-line option of "nosmp" or "maxcpus=0" will disable SMP
|
|
* activation entirely (the MPS table probe still happens, though).
|
|
*
|
|
* Command-line option of "maxcpus=<NUM>", where <NUM> is an integer
|
|
* greater than 0, limits the maximum number of CPUs activated in
|
|
* SMP mode to <NUM>.
|
|
*/
|
|
#ifndef CONFIG_X86_IO_APIC
|
|
static inline void disable_ioapic_setup(void) {};
|
|
#endif
|
|
|
|
static int __init nosmp(char *str)
|
|
{
|
|
setup_max_cpus = 0;
|
|
disable_ioapic_setup();
|
|
return 0;
|
|
}
|
|
|
|
early_param("nosmp", nosmp);
|
|
|
|
static int __init maxcpus(char *str)
|
|
{
|
|
get_option(&str, &setup_max_cpus);
|
|
if (setup_max_cpus == 0)
|
|
disable_ioapic_setup();
|
|
|
|
return 0;
|
|
}
|
|
|
|
early_param("maxcpus", maxcpus);
|
|
#else
|
|
#define setup_max_cpus NR_CPUS
|
|
#endif
|
|
|
|
/*
|
|
* If set, this is an indication to the drivers that reset the underlying
|
|
* device before going ahead with the initialization otherwise driver might
|
|
* rely on the BIOS and skip the reset operation.
|
|
*
|
|
* This is useful if kernel is booting in an unreliable environment.
|
|
* For ex. kdump situaiton where previous kernel has crashed, BIOS has been
|
|
* skipped and devices will be in unknown state.
|
|
*/
|
|
unsigned int reset_devices;
|
|
EXPORT_SYMBOL(reset_devices);
|
|
|
|
static int __init set_reset_devices(char *str)
|
|
{
|
|
reset_devices = 1;
|
|
return 1;
|
|
}
|
|
|
|
__setup("reset_devices", set_reset_devices);
|
|
|
|
static char * argv_init[MAX_INIT_ARGS+2] = { "init", NULL, };
|
|
char * envp_init[MAX_INIT_ENVS+2] = { "HOME=/", "TERM=linux", NULL, };
|
|
static const char *panic_later, *panic_param;
|
|
|
|
extern struct obs_kernel_param __setup_start[], __setup_end[];
|
|
|
|
static int __init obsolete_checksetup(char *line)
|
|
{
|
|
struct obs_kernel_param *p;
|
|
int had_early_param = 0;
|
|
|
|
p = __setup_start;
|
|
do {
|
|
int n = strlen(p->str);
|
|
if (!strncmp(line, p->str, n)) {
|
|
if (p->early) {
|
|
/* Already done in parse_early_param?
|
|
* (Needs exact match on param part).
|
|
* Keep iterating, as we can have early
|
|
* params and __setups of same names 8( */
|
|
if (line[n] == '\0' || line[n] == '=')
|
|
had_early_param = 1;
|
|
} else if (!p->setup_func) {
|
|
printk(KERN_WARNING "Parameter %s is obsolete,"
|
|
" ignored\n", p->str);
|
|
return 1;
|
|
} else if (p->setup_func(line + n))
|
|
return 1;
|
|
}
|
|
p++;
|
|
} while (p < __setup_end);
|
|
|
|
return had_early_param;
|
|
}
|
|
|
|
/*
|
|
* This should be approx 2 Bo*oMips to start (note initial shift), and will
|
|
* still work even if initially too large, it will just take slightly longer
|
|
*/
|
|
unsigned long loops_per_jiffy = (1<<12);
|
|
|
|
EXPORT_SYMBOL(loops_per_jiffy);
|
|
|
|
static int __init debug_kernel(char *str)
|
|
{
|
|
console_loglevel = 10;
|
|
return 0;
|
|
}
|
|
|
|
static int __init quiet_kernel(char *str)
|
|
{
|
|
console_loglevel = 4;
|
|
return 0;
|
|
}
|
|
|
|
early_param("debug", debug_kernel);
|
|
early_param("quiet", quiet_kernel);
|
|
|
|
static int __init loglevel(char *str)
|
|
{
|
|
get_option(&str, &console_loglevel);
|
|
return 0;
|
|
}
|
|
|
|
early_param("loglevel", loglevel);
|
|
|
|
/*
|
|
* Unknown boot options get handed to init, unless they look like
|
|
* failed parameters
|
|
*/
|
|
static int __init unknown_bootoption(char *param, char *val)
|
|
{
|
|
/* Change NUL term back to "=", to make "param" the whole string. */
|
|
if (val) {
|
|
/* param=val or param="val"? */
|
|
if (val == param+strlen(param)+1)
|
|
val[-1] = '=';
|
|
else if (val == param+strlen(param)+2) {
|
|
val[-2] = '=';
|
|
memmove(val-1, val, strlen(val)+1);
|
|
val--;
|
|
} else
|
|
BUG();
|
|
}
|
|
|
|
/* Handle obsolete-style parameters */
|
|
if (obsolete_checksetup(param))
|
|
return 0;
|
|
|
|
/*
|
|
* Preemptive maintenance for "why didn't my misspelled command
|
|
* line work?"
|
|
*/
|
|
if (strchr(param, '.') && (!val || strchr(param, '.') < val)) {
|
|
printk(KERN_ERR "Unknown boot option `%s': ignoring\n", param);
|
|
return 0;
|
|
}
|
|
|
|
if (panic_later)
|
|
return 0;
|
|
|
|
if (val) {
|
|
/* Environment option */
|
|
unsigned int i;
|
|
for (i = 0; envp_init[i]; i++) {
|
|
if (i == MAX_INIT_ENVS) {
|
|
panic_later = "Too many boot env vars at `%s'";
|
|
panic_param = param;
|
|
}
|
|
if (!strncmp(param, envp_init[i], val - param))
|
|
break;
|
|
}
|
|
envp_init[i] = param;
|
|
} else {
|
|
/* Command line option */
|
|
unsigned int i;
|
|
for (i = 0; argv_init[i]; i++) {
|
|
if (i == MAX_INIT_ARGS) {
|
|
panic_later = "Too many boot init vars at `%s'";
|
|
panic_param = param;
|
|
}
|
|
}
|
|
argv_init[i] = param;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
#ifdef CONFIG_DEBUG_PAGEALLOC
|
|
int __read_mostly debug_pagealloc_enabled = 0;
|
|
#endif
|
|
|
|
static int __init init_setup(char *str)
|
|
{
|
|
unsigned int i;
|
|
|
|
execute_command = str;
|
|
/*
|
|
* In case LILO is going to boot us with default command line,
|
|
* it prepends "auto" before the whole cmdline which makes
|
|
* the shell think it should execute a script with such name.
|
|
* So we ignore all arguments entered _before_ init=... [MJ]
|
|
*/
|
|
for (i = 1; i < MAX_INIT_ARGS; i++)
|
|
argv_init[i] = NULL;
|
|
return 1;
|
|
}
|
|
__setup("init=", init_setup);
|
|
|
|
static int __init rdinit_setup(char *str)
|
|
{
|
|
unsigned int i;
|
|
|
|
ramdisk_execute_command = str;
|
|
/* See "auto" comment in init_setup */
|
|
for (i = 1; i < MAX_INIT_ARGS; i++)
|
|
argv_init[i] = NULL;
|
|
return 1;
|
|
}
|
|
__setup("rdinit=", rdinit_setup);
|
|
|
|
#ifndef CONFIG_SMP
|
|
|
|
#ifdef CONFIG_X86_LOCAL_APIC
|
|
static void __init smp_init(void)
|
|
{
|
|
APIC_init_uniprocessor();
|
|
}
|
|
#else
|
|
#define smp_init() do { } while (0)
|
|
#endif
|
|
|
|
static inline void setup_per_cpu_areas(void) { }
|
|
static inline void setup_nr_cpu_ids(void) { }
|
|
static inline void smp_prepare_cpus(unsigned int maxcpus) { }
|
|
|
|
#else
|
|
|
|
#if NR_CPUS > BITS_PER_LONG
|
|
cpumask_t cpu_mask_all __read_mostly = CPU_MASK_ALL;
|
|
EXPORT_SYMBOL(cpu_mask_all);
|
|
#endif
|
|
|
|
/* Setup number of possible processor ids */
|
|
int nr_cpu_ids __read_mostly = NR_CPUS;
|
|
EXPORT_SYMBOL(nr_cpu_ids);
|
|
|
|
/* An arch may set nr_cpu_ids earlier if needed, so this would be redundant */
|
|
static void __init setup_nr_cpu_ids(void)
|
|
{
|
|
int cpu, highest_cpu = 0;
|
|
|
|
for_each_possible_cpu(cpu)
|
|
highest_cpu = cpu;
|
|
|
|
nr_cpu_ids = highest_cpu + 1;
|
|
}
|
|
|
|
#ifndef CONFIG_HAVE_SETUP_PER_CPU_AREA
|
|
unsigned long __per_cpu_offset[NR_CPUS] __read_mostly;
|
|
|
|
EXPORT_SYMBOL(__per_cpu_offset);
|
|
|
|
static void __init setup_per_cpu_areas(void)
|
|
{
|
|
unsigned long size, i;
|
|
char *ptr;
|
|
unsigned long nr_possible_cpus = num_possible_cpus();
|
|
|
|
/* Copy section for each CPU (we discard the original) */
|
|
size = ALIGN(PERCPU_ENOUGH_ROOM, PAGE_SIZE);
|
|
ptr = alloc_bootmem_pages(size * nr_possible_cpus);
|
|
|
|
for_each_possible_cpu(i) {
|
|
__per_cpu_offset[i] = ptr - __per_cpu_start;
|
|
memcpy(ptr, __per_cpu_start, __per_cpu_end - __per_cpu_start);
|
|
ptr += size;
|
|
}
|
|
}
|
|
#endif /* CONFIG_HAVE_SETUP_PER_CPU_AREA */
|
|
|
|
/* Called by boot processor to activate the rest. */
|
|
static void __init smp_init(void)
|
|
{
|
|
unsigned int cpu;
|
|
|
|
/*
|
|
* Set up the current CPU as possible to migrate to.
|
|
* The other ones will be done by cpu_up/cpu_down()
|
|
*/
|
|
cpu = smp_processor_id();
|
|
cpu_set(cpu, cpu_active_map);
|
|
|
|
/* FIXME: This should be done in userspace --RR */
|
|
for_each_present_cpu(cpu) {
|
|
if (num_online_cpus() >= setup_max_cpus)
|
|
break;
|
|
if (!cpu_online(cpu))
|
|
cpu_up(cpu);
|
|
}
|
|
|
|
/* Any cleanup work */
|
|
printk(KERN_INFO "Brought up %ld CPUs\n", (long)num_online_cpus());
|
|
smp_cpus_done(setup_max_cpus);
|
|
}
|
|
|
|
#endif
|
|
|
|
/*
|
|
* We need to store the untouched command line for future reference.
|
|
* We also need to store the touched command line since the parameter
|
|
* parsing is performed in place, and we should allow a component to
|
|
* store reference of name/value for future reference.
|
|
*/
|
|
static void __init setup_command_line(char *command_line)
|
|
{
|
|
saved_command_line = alloc_bootmem(strlen (boot_command_line)+1);
|
|
static_command_line = alloc_bootmem(strlen (command_line)+1);
|
|
strcpy (saved_command_line, boot_command_line);
|
|
strcpy (static_command_line, command_line);
|
|
}
|
|
|
|
/*
|
|
* We need to finalize in a non-__init function or else race conditions
|
|
* between the root thread and the init thread may cause start_kernel to
|
|
* be reaped by free_initmem before the root thread has proceeded to
|
|
* cpu_idle.
|
|
*
|
|
* gcc-3.4 accidentally inlines this function, so use noinline.
|
|
*/
|
|
|
|
static void noinline __init_refok rest_init(void)
|
|
__releases(kernel_lock)
|
|
{
|
|
int pid;
|
|
|
|
kernel_thread(kernel_init, NULL, CLONE_FS | CLONE_SIGHAND);
|
|
numa_default_policy();
|
|
pid = kernel_thread(kthreadd, NULL, CLONE_FS | CLONE_FILES);
|
|
kthreadd_task = find_task_by_pid_ns(pid, &init_pid_ns);
|
|
unlock_kernel();
|
|
|
|
/*
|
|
* The boot idle thread must execute schedule()
|
|
* at least once to get things moving:
|
|
*/
|
|
init_idle_bootup_task(current);
|
|
preempt_enable_no_resched();
|
|
schedule();
|
|
preempt_disable();
|
|
|
|
/* Call into cpu_idle with preempt disabled */
|
|
cpu_idle();
|
|
}
|
|
|
|
/* Check for early params. */
|
|
static int __init do_early_param(char *param, char *val)
|
|
{
|
|
struct obs_kernel_param *p;
|
|
|
|
for (p = __setup_start; p < __setup_end; p++) {
|
|
if ((p->early && strcmp(param, p->str) == 0) ||
|
|
(strcmp(param, "console") == 0 &&
|
|
strcmp(p->str, "earlycon") == 0)
|
|
) {
|
|
if (p->setup_func(val) != 0)
|
|
printk(KERN_WARNING
|
|
"Malformed early option '%s'\n", param);
|
|
}
|
|
}
|
|
/* We accept everything at this stage. */
|
|
return 0;
|
|
}
|
|
|
|
/* Arch code calls this early on, or if not, just before other parsing. */
|
|
void __init parse_early_param(void)
|
|
{
|
|
static __initdata int done = 0;
|
|
static __initdata char tmp_cmdline[COMMAND_LINE_SIZE];
|
|
|
|
if (done)
|
|
return;
|
|
|
|
/* All fall through to do_early_param. */
|
|
strlcpy(tmp_cmdline, boot_command_line, COMMAND_LINE_SIZE);
|
|
parse_args("early options", tmp_cmdline, NULL, 0, do_early_param);
|
|
done = 1;
|
|
}
|
|
|
|
/*
|
|
* Activate the first processor.
|
|
*/
|
|
|
|
static void __init boot_cpu_init(void)
|
|
{
|
|
int cpu = smp_processor_id();
|
|
/* Mark the boot cpu "present", "online" etc for SMP and UP case */
|
|
cpu_set(cpu, cpu_online_map);
|
|
cpu_set(cpu, cpu_present_map);
|
|
cpu_set(cpu, cpu_possible_map);
|
|
}
|
|
|
|
void __init __weak smp_setup_processor_id(void)
|
|
{
|
|
}
|
|
|
|
void __init __weak thread_info_cache_init(void)
|
|
{
|
|
}
|
|
|
|
asmlinkage void __init start_kernel(void)
|
|
{
|
|
char * command_line;
|
|
extern struct kernel_param __start___param[], __stop___param[];
|
|
|
|
smp_setup_processor_id();
|
|
|
|
/*
|
|
* Need to run as early as possible, to initialize the
|
|
* lockdep hash:
|
|
*/
|
|
unwind_init();
|
|
lockdep_init();
|
|
debug_objects_early_init();
|
|
cgroup_init_early();
|
|
|
|
local_irq_disable();
|
|
early_boot_irqs_off();
|
|
early_init_irq_lock_class();
|
|
|
|
/*
|
|
* Interrupts are still disabled. Do necessary setups, then
|
|
* enable them
|
|
*/
|
|
lock_kernel();
|
|
tick_init();
|
|
boot_cpu_init();
|
|
page_address_init();
|
|
printk(KERN_NOTICE);
|
|
printk(linux_banner);
|
|
setup_arch(&command_line);
|
|
mm_init_owner(&init_mm, &init_task);
|
|
setup_command_line(command_line);
|
|
unwind_setup();
|
|
setup_per_cpu_areas();
|
|
setup_nr_cpu_ids();
|
|
smp_prepare_boot_cpu(); /* arch-specific boot-cpu hooks */
|
|
|
|
/*
|
|
* Set up the scheduler prior starting any interrupts (such as the
|
|
* timer interrupt). Full topology setup happens at smp_init()
|
|
* time - but meanwhile we still have a functioning scheduler.
|
|
*/
|
|
sched_init();
|
|
/*
|
|
* Disable preemption - early bootup scheduling is extremely
|
|
* fragile until we cpu_idle() for the first time.
|
|
*/
|
|
preempt_disable();
|
|
build_all_zonelists();
|
|
page_alloc_init();
|
|
printk(KERN_NOTICE "Kernel command line: %s\n", boot_command_line);
|
|
parse_early_param();
|
|
parse_args("Booting kernel", static_command_line, __start___param,
|
|
__stop___param - __start___param,
|
|
&unknown_bootoption);
|
|
if (!irqs_disabled()) {
|
|
printk(KERN_WARNING "start_kernel(): bug: interrupts were "
|
|
"enabled *very* early, fixing it\n");
|
|
local_irq_disable();
|
|
}
|
|
sort_main_extable();
|
|
trap_init();
|
|
rcu_init();
|
|
init_IRQ();
|
|
pidhash_init();
|
|
init_timers();
|
|
hrtimers_init();
|
|
softirq_init();
|
|
timekeeping_init();
|
|
time_init();
|
|
sched_clock_init();
|
|
profile_init();
|
|
if (!irqs_disabled())
|
|
printk("start_kernel(): bug: interrupts were enabled early\n");
|
|
early_boot_irqs_on();
|
|
local_irq_enable();
|
|
|
|
/*
|
|
* HACK ALERT! This is early. We're enabling the console before
|
|
* we've done PCI setups etc, and console_init() must be aware of
|
|
* this. But we do want output early, in case something goes wrong.
|
|
*/
|
|
console_init();
|
|
if (panic_later)
|
|
panic(panic_later, panic_param);
|
|
|
|
lockdep_info();
|
|
|
|
/*
|
|
* Need to run this when irqs are enabled, because it wants
|
|
* to self-test [hard/soft]-irqs on/off lock inversion bugs
|
|
* too:
|
|
*/
|
|
locking_selftest();
|
|
|
|
#ifdef CONFIG_BLK_DEV_INITRD
|
|
if (initrd_start && !initrd_below_start_ok &&
|
|
page_to_pfn(virt_to_page((void *)initrd_start)) < min_low_pfn) {
|
|
printk(KERN_CRIT "initrd overwritten (0x%08lx < 0x%08lx) - "
|
|
"disabling it.\n",
|
|
page_to_pfn(virt_to_page((void *)initrd_start)),
|
|
min_low_pfn);
|
|
initrd_start = 0;
|
|
}
|
|
#endif
|
|
vmalloc_init();
|
|
vfs_caches_init_early();
|
|
cpuset_init_early();
|
|
mem_init();
|
|
enable_debug_pagealloc();
|
|
cpu_hotplug_init();
|
|
kmem_cache_init();
|
|
debug_objects_mem_init();
|
|
idr_init_cache();
|
|
setup_per_cpu_pageset();
|
|
numa_policy_init();
|
|
if (late_time_init)
|
|
late_time_init();
|
|
calibrate_delay();
|
|
pidmap_init();
|
|
pgtable_cache_init();
|
|
prio_tree_init();
|
|
anon_vma_init();
|
|
#ifdef CONFIG_X86
|
|
if (efi_enabled)
|
|
efi_enter_virtual_mode();
|
|
#endif
|
|
thread_info_cache_init();
|
|
fork_init(num_physpages);
|
|
proc_caches_init();
|
|
buffer_init();
|
|
unnamed_dev_init();
|
|
key_init();
|
|
security_init();
|
|
vfs_caches_init(num_physpages);
|
|
radix_tree_init();
|
|
signals_init();
|
|
/* rootfs populating might need page-writeback */
|
|
page_writeback_init();
|
|
#ifdef CONFIG_PROC_FS
|
|
proc_root_init();
|
|
#endif
|
|
cgroup_init();
|
|
cpuset_init();
|
|
taskstats_init_early();
|
|
delayacct_init();
|
|
|
|
check_bugs();
|
|
|
|
acpi_early_init(); /* before LAPIC and SMP init */
|
|
|
|
/* Do the rest non-__init'ed, we're now alive */
|
|
rest_init();
|
|
}
|
|
|
|
static int initcall_debug;
|
|
|
|
static int __init initcall_debug_setup(char *str)
|
|
{
|
|
initcall_debug = 1;
|
|
return 1;
|
|
}
|
|
__setup("initcall_debug", initcall_debug_setup);
|
|
|
|
int do_one_initcall(initcall_t fn)
|
|
{
|
|
int count = preempt_count();
|
|
ktime_t t0, t1, delta;
|
|
char msgbuf[64];
|
|
int result;
|
|
|
|
if (initcall_debug) {
|
|
printk("calling %pF @ %i\n", fn, task_pid_nr(current));
|
|
t0 = ktime_get();
|
|
}
|
|
|
|
result = fn();
|
|
|
|
if (initcall_debug) {
|
|
t1 = ktime_get();
|
|
delta = ktime_sub(t1, t0);
|
|
|
|
printk("initcall %pF returned %d after %Ld msecs\n",
|
|
fn, result,
|
|
(unsigned long long) delta.tv64 >> 20);
|
|
}
|
|
|
|
msgbuf[0] = 0;
|
|
|
|
if (result && result != -ENODEV && initcall_debug)
|
|
sprintf(msgbuf, "error code %d ", result);
|
|
|
|
if (preempt_count() != count) {
|
|
strlcat(msgbuf, "preemption imbalance ", sizeof(msgbuf));
|
|
preempt_count() = count;
|
|
}
|
|
if (irqs_disabled()) {
|
|
strlcat(msgbuf, "disabled interrupts ", sizeof(msgbuf));
|
|
local_irq_enable();
|
|
}
|
|
if (msgbuf[0]) {
|
|
printk("initcall %pF returned with %s\n", fn, msgbuf);
|
|
}
|
|
|
|
return result;
|
|
}
|
|
|
|
|
|
extern initcall_t __initcall_start[], __initcall_end[], __early_initcall_end[];
|
|
|
|
static void __init do_initcalls(void)
|
|
{
|
|
initcall_t *call;
|
|
|
|
for (call = __early_initcall_end; call < __initcall_end; call++)
|
|
do_one_initcall(*call);
|
|
|
|
/* Make sure there is no pending stuff from the initcall sequence */
|
|
flush_scheduled_work();
|
|
}
|
|
|
|
/*
|
|
* Ok, the machine is now initialized. None of the devices
|
|
* have been touched yet, but the CPU subsystem is up and
|
|
* running, and memory and process management works.
|
|
*
|
|
* Now we can finally start doing some real work..
|
|
*/
|
|
static void __init do_basic_setup(void)
|
|
{
|
|
rcu_init_sched(); /* needed by module_init stage. */
|
|
/* drivers will send hotplug events */
|
|
init_workqueues();
|
|
usermodehelper_init();
|
|
driver_init();
|
|
init_irq_proc();
|
|
do_initcalls();
|
|
}
|
|
|
|
static void __init do_pre_smp_initcalls(void)
|
|
{
|
|
initcall_t *call;
|
|
|
|
for (call = __initcall_start; call < __early_initcall_end; call++)
|
|
do_one_initcall(*call);
|
|
}
|
|
|
|
static void run_init_process(char *init_filename)
|
|
{
|
|
argv_init[0] = init_filename;
|
|
kernel_execve(init_filename, argv_init, envp_init);
|
|
}
|
|
|
|
/* This is a non __init function. Force it to be noinline otherwise gcc
|
|
* makes it inline to init() and it becomes part of init.text section
|
|
*/
|
|
static int noinline init_post(void)
|
|
{
|
|
free_initmem();
|
|
unlock_kernel();
|
|
mark_rodata_ro();
|
|
system_state = SYSTEM_RUNNING;
|
|
numa_default_policy();
|
|
|
|
if (sys_open((const char __user *) "/dev/console", O_RDWR, 0) < 0)
|
|
printk(KERN_WARNING "Warning: unable to open an initial console.\n");
|
|
|
|
(void) sys_dup(0);
|
|
(void) sys_dup(0);
|
|
|
|
current->signal->flags |= SIGNAL_UNKILLABLE;
|
|
|
|
if (ramdisk_execute_command) {
|
|
run_init_process(ramdisk_execute_command);
|
|
printk(KERN_WARNING "Failed to execute %s\n",
|
|
ramdisk_execute_command);
|
|
}
|
|
|
|
/*
|
|
* We try each of these until one succeeds.
|
|
*
|
|
* The Bourne shell can be used instead of init if we are
|
|
* trying to recover a really broken machine.
|
|
*/
|
|
if (execute_command) {
|
|
run_init_process(execute_command);
|
|
printk(KERN_WARNING "Failed to execute %s. Attempting "
|
|
"defaults...\n", execute_command);
|
|
}
|
|
run_init_process("/sbin/init");
|
|
run_init_process("/etc/init");
|
|
run_init_process("/bin/init");
|
|
run_init_process("/bin/sh");
|
|
|
|
panic("No init found. Try passing init= option to kernel.");
|
|
}
|
|
|
|
static int __init kernel_init(void * unused)
|
|
{
|
|
lock_kernel();
|
|
/*
|
|
* init can run on any cpu.
|
|
*/
|
|
set_cpus_allowed_ptr(current, CPU_MASK_ALL_PTR);
|
|
/*
|
|
* Tell the world that we're going to be the grim
|
|
* reaper of innocent orphaned children.
|
|
*
|
|
* We don't want people to have to make incorrect
|
|
* assumptions about where in the task array this
|
|
* can be found.
|
|
*/
|
|
init_pid_ns.child_reaper = current;
|
|
|
|
cad_pid = task_pid(current);
|
|
|
|
smp_prepare_cpus(setup_max_cpus);
|
|
|
|
do_pre_smp_initcalls();
|
|
|
|
smp_init();
|
|
sched_init_smp();
|
|
|
|
cpuset_init_smp();
|
|
|
|
do_basic_setup();
|
|
|
|
/*
|
|
* check if there is an early userspace init. If yes, let it do all
|
|
* the work
|
|
*/
|
|
|
|
if (!ramdisk_execute_command)
|
|
ramdisk_execute_command = "/init";
|
|
|
|
if (sys_access((const char __user *) ramdisk_execute_command, 0) != 0) {
|
|
ramdisk_execute_command = NULL;
|
|
prepare_namespace();
|
|
}
|
|
|
|
/*
|
|
* Ok, we have completed the initial bootup, and
|
|
* we're essentially up and running. Get rid of the
|
|
* initmem segments and start the user-mode stuff..
|
|
*/
|
|
init_post();
|
|
return 0;
|
|
}
|