mirror of
https://github.com/torvalds/linux
synced 2024-09-16 09:05:45 +00:00
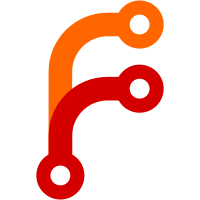
We usually copy all bits that a request needs from the userspace for async execution, so the userspace can keep them on the stack. However, send zerocopy violates this pattern for addresses and may reloads it e.g. from io-wq. Save the address if any in ->async_data as usual. Reported-by: Stefan Metzmacher <metze@samba.org> Signed-off-by: Pavel Begunkov <asml.silence@gmail.com> Link: https://lore.kernel.org/r/d7512d7aa9abcd36e9afe1a4d292a24cb2d157e5.1661342812.git.asml.silence@gmail.com [axboe: fold in incremental fix] Signed-off-by: Jens Axboe <axboe@kernel.dk>
65 lines
2.1 KiB
C
65 lines
2.1 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
|
|
#include <linux/net.h>
|
|
#include <linux/uio.h>
|
|
|
|
#include "alloc_cache.h"
|
|
|
|
#if defined(CONFIG_NET)
|
|
struct io_async_msghdr {
|
|
union {
|
|
struct iovec fast_iov[UIO_FASTIOV];
|
|
struct {
|
|
struct iovec fast_iov_one;
|
|
__kernel_size_t controllen;
|
|
int namelen;
|
|
__kernel_size_t payloadlen;
|
|
};
|
|
struct io_cache_entry cache;
|
|
};
|
|
/* points to an allocated iov, if NULL we use fast_iov instead */
|
|
struct iovec *free_iov;
|
|
struct sockaddr __user *uaddr;
|
|
struct msghdr msg;
|
|
struct sockaddr_storage addr;
|
|
};
|
|
|
|
struct io_async_connect {
|
|
struct sockaddr_storage address;
|
|
};
|
|
|
|
int io_shutdown_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_shutdown(struct io_kiocb *req, unsigned int issue_flags);
|
|
|
|
int io_sendzc_prep_async(struct io_kiocb *req);
|
|
int io_sendmsg_prep_async(struct io_kiocb *req);
|
|
void io_sendmsg_recvmsg_cleanup(struct io_kiocb *req);
|
|
int io_sendmsg_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_sendmsg(struct io_kiocb *req, unsigned int issue_flags);
|
|
int io_send(struct io_kiocb *req, unsigned int issue_flags);
|
|
|
|
int io_recvmsg_prep_async(struct io_kiocb *req);
|
|
int io_recvmsg_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_recvmsg(struct io_kiocb *req, unsigned int issue_flags);
|
|
int io_recv(struct io_kiocb *req, unsigned int issue_flags);
|
|
|
|
int io_accept_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_accept(struct io_kiocb *req, unsigned int issue_flags);
|
|
|
|
int io_socket_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_socket(struct io_kiocb *req, unsigned int issue_flags);
|
|
|
|
int io_connect_prep_async(struct io_kiocb *req);
|
|
int io_connect_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
int io_connect(struct io_kiocb *req, unsigned int issue_flags);
|
|
|
|
int io_sendzc(struct io_kiocb *req, unsigned int issue_flags);
|
|
int io_sendzc_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe);
|
|
|
|
void io_netmsg_cache_free(struct io_cache_entry *entry);
|
|
#else
|
|
static inline void io_netmsg_cache_free(struct io_cache_entry *entry)
|
|
{
|
|
}
|
|
#endif
|