mirror of
https://github.com/torvalds/linux
synced 2024-09-06 18:01:05 +00:00
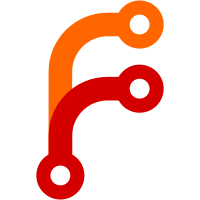
This patch provides functions to lock & unlock access to the "core-other" register region of the CPC. Without performing appropriate locking it is possible for code using this region to be preempted or to race with code on another VPE within the same core, with one changing the core which the "core-other" region is acting upon at an inopportune time for the other. Signed-off-by: Paul Burton <paul.burton@imgtec.com>
79 lines
1.9 KiB
C
79 lines
1.9 KiB
C
/*
|
|
* Copyright (C) 2013 Imagination Technologies
|
|
* Author: Paul Burton <paul.burton@imgtec.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation; either version 2 of the License, or (at your
|
|
* option) any later version.
|
|
*/
|
|
|
|
#include <linux/errno.h>
|
|
|
|
#include <asm/mips-cm.h>
|
|
#include <asm/mips-cpc.h>
|
|
|
|
void __iomem *mips_cpc_base;
|
|
|
|
static DEFINE_PER_CPU_ALIGNED(spinlock_t, cpc_core_lock);
|
|
|
|
static DEFINE_PER_CPU_ALIGNED(unsigned long, cpc_core_lock_flags);
|
|
|
|
phys_t __weak mips_cpc_phys_base(void)
|
|
{
|
|
u32 cpc_base;
|
|
|
|
if (!mips_cm_present())
|
|
return 0;
|
|
|
|
if (!(read_gcr_cpc_status() & CM_GCR_CPC_STATUS_EX_MSK))
|
|
return 0;
|
|
|
|
/* If the CPC is already enabled, leave it so */
|
|
cpc_base = read_gcr_cpc_base();
|
|
if (cpc_base & CM_GCR_CPC_BASE_CPCEN_MSK)
|
|
return cpc_base & CM_GCR_CPC_BASE_CPCBASE_MSK;
|
|
|
|
/* Otherwise, give it the default address & enable it */
|
|
cpc_base = mips_cpc_default_phys_base();
|
|
write_gcr_cpc_base(cpc_base | CM_GCR_CPC_BASE_CPCEN_MSK);
|
|
return cpc_base;
|
|
}
|
|
|
|
int mips_cpc_probe(void)
|
|
{
|
|
phys_t addr;
|
|
unsigned cpu;
|
|
|
|
for_each_possible_cpu(cpu)
|
|
spin_lock_init(&per_cpu(cpc_core_lock, cpu));
|
|
|
|
addr = mips_cpc_phys_base();
|
|
if (!addr)
|
|
return -ENODEV;
|
|
|
|
mips_cpc_base = ioremap_nocache(addr, 0x8000);
|
|
if (!mips_cpc_base)
|
|
return -ENXIO;
|
|
|
|
return 0;
|
|
}
|
|
|
|
void mips_cpc_lock_other(unsigned int core)
|
|
{
|
|
unsigned curr_core;
|
|
preempt_disable();
|
|
curr_core = current_cpu_data.core;
|
|
spin_lock_irqsave(&per_cpu(cpc_core_lock, curr_core),
|
|
per_cpu(cpc_core_lock_flags, curr_core));
|
|
write_cpc_cl_other(core << CPC_Cx_OTHER_CORENUM_SHF);
|
|
}
|
|
|
|
void mips_cpc_unlock_other(void)
|
|
{
|
|
unsigned curr_core = current_cpu_data.core;
|
|
spin_unlock_irqrestore(&per_cpu(cpc_core_lock, curr_core),
|
|
per_cpu(cpc_core_lock_flags, curr_core));
|
|
preempt_enable();
|
|
}
|