mirror of
https://github.com/torvalds/linux
synced 2024-07-22 03:01:14 +00:00
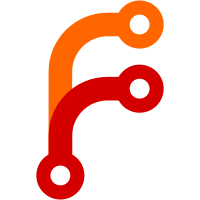
Some laptops without _DSD have the SPI speed set very low in the BIOS. Since the SPI controller uses this speed as its max speed, the SPI transactions are very slow. Firmware download writes to many registers, and if the SPI speed is too slow, it can take a long time to download. For this reason, disable firmware loading if the maximum SPI speed is too low. Without Firmware, audio playback will work, but the volume will be low to ensure safe operation of the CS35L41. Signed-off-by: Stefan Binding <sbinding@opensource.cirrus.com> Cc: <stable@vger.kernel.org> # v6.7+ Link: https://lore.kernel.org/r/20231221132518.3213-3-sbinding@opensource.cirrus.com Signed-off-by: Takashi Iwai <tiwai@suse.de>
64 lines
1.5 KiB
C
64 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
//
|
|
// CS35l41 HDA SPI driver
|
|
//
|
|
// Copyright 2021 Cirrus Logic, Inc.
|
|
//
|
|
// Author: Lucas Tanure <tanureal@opensource.cirrus.com>
|
|
|
|
#include <linux/mod_devicetable.h>
|
|
#include <linux/module.h>
|
|
#include <linux/spi/spi.h>
|
|
|
|
#include "cs35l41_hda.h"
|
|
|
|
static int cs35l41_hda_spi_probe(struct spi_device *spi)
|
|
{
|
|
const char *device_name;
|
|
|
|
/*
|
|
* Compare against the device name so it works for SPI, normal ACPI
|
|
* and for ACPI by serial-multi-instantiate matching cases.
|
|
*/
|
|
if (strstr(dev_name(&spi->dev), "CSC3551"))
|
|
device_name = "CSC3551";
|
|
else
|
|
return -ENODEV;
|
|
|
|
return cs35l41_hda_probe(&spi->dev, device_name, spi_get_chipselect(spi, 0), spi->irq,
|
|
devm_regmap_init_spi(spi, &cs35l41_regmap_spi), SPI);
|
|
}
|
|
|
|
static void cs35l41_hda_spi_remove(struct spi_device *spi)
|
|
{
|
|
cs35l41_hda_remove(&spi->dev);
|
|
}
|
|
|
|
static const struct spi_device_id cs35l41_hda_spi_id[] = {
|
|
{ "cs35l41-hda", 0 },
|
|
{}
|
|
};
|
|
|
|
static const struct acpi_device_id cs35l41_acpi_hda_match[] = {
|
|
{ "CSC3551", 0 },
|
|
{}
|
|
};
|
|
MODULE_DEVICE_TABLE(acpi, cs35l41_acpi_hda_match);
|
|
|
|
static struct spi_driver cs35l41_spi_driver = {
|
|
.driver = {
|
|
.name = "cs35l41-hda",
|
|
.acpi_match_table = cs35l41_acpi_hda_match,
|
|
.pm = &cs35l41_hda_pm_ops,
|
|
},
|
|
.id_table = cs35l41_hda_spi_id,
|
|
.probe = cs35l41_hda_spi_probe,
|
|
.remove = cs35l41_hda_spi_remove,
|
|
};
|
|
module_spi_driver(cs35l41_spi_driver);
|
|
|
|
MODULE_DESCRIPTION("HDA CS35L41 driver");
|
|
MODULE_IMPORT_NS(SND_HDA_SCODEC_CS35L41);
|
|
MODULE_AUTHOR("Lucas Tanure <tanureal@opensource.cirrus.com>");
|
|
MODULE_LICENSE("GPL");
|