mirror of
https://github.com/torvalds/linux
synced 2024-10-09 12:54:52 +00:00
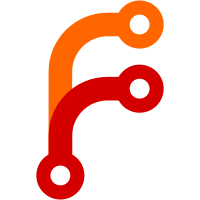
The write-protect pin handling looks like a standard property that could benefit other users if available in the core nvmem framework. Instead of modifying all the memory drivers to check this pin, make the NVMEM subsystem check if the write-protect GPIO being passed through the nvmem_config or defined in the device tree and pull it low whenever writing to the memory. There was a suggestion for introducing the gpiodesc from pdata, but as pdata is already removed it could be replaced by adding it to nvmem_config. Reference: https://lists.96boards.org/pipermail/dev/2018-August/001056.html Signed-off-by: Khouloud Touil <ktouil@baylibre.com> Reviewed-by: Linus Walleij <linus.walleij@linaro.org> Acked-by: Srinivas Kandagatla <srinivas.kandagatla@linaro.org> Signed-off-by: Bartosz Golaszewski <bgolaszewski@baylibre.com>
65 lines
1.6 KiB
C
65 lines
1.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
|
|
#ifndef _DRIVERS_NVMEM_H
|
|
#define _DRIVERS_NVMEM_H
|
|
|
|
#include <linux/device.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/kref.h>
|
|
#include <linux/list.h>
|
|
#include <linux/nvmem-consumer.h>
|
|
#include <linux/nvmem-provider.h>
|
|
#include <linux/gpio/consumer.h>
|
|
|
|
struct nvmem_device {
|
|
struct module *owner;
|
|
struct device dev;
|
|
int stride;
|
|
int word_size;
|
|
int id;
|
|
struct kref refcnt;
|
|
size_t size;
|
|
bool read_only;
|
|
int flags;
|
|
enum nvmem_type type;
|
|
struct bin_attribute eeprom;
|
|
struct device *base_dev;
|
|
struct list_head cells;
|
|
nvmem_reg_read_t reg_read;
|
|
nvmem_reg_write_t reg_write;
|
|
struct gpio_desc *wp_gpio;
|
|
void *priv;
|
|
};
|
|
|
|
#define to_nvmem_device(d) container_of(d, struct nvmem_device, dev)
|
|
#define FLAG_COMPAT BIT(0)
|
|
|
|
#ifdef CONFIG_NVMEM_SYSFS
|
|
const struct attribute_group **nvmem_sysfs_get_groups(
|
|
struct nvmem_device *nvmem,
|
|
const struct nvmem_config *config);
|
|
int nvmem_sysfs_setup_compat(struct nvmem_device *nvmem,
|
|
const struct nvmem_config *config);
|
|
void nvmem_sysfs_remove_compat(struct nvmem_device *nvmem,
|
|
const struct nvmem_config *config);
|
|
#else
|
|
static inline const struct attribute_group **nvmem_sysfs_get_groups(
|
|
struct nvmem_device *nvmem,
|
|
const struct nvmem_config *config)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static inline int nvmem_sysfs_setup_compat(struct nvmem_device *nvmem,
|
|
const struct nvmem_config *config)
|
|
{
|
|
return -ENOSYS;
|
|
}
|
|
static inline void nvmem_sysfs_remove_compat(struct nvmem_device *nvmem,
|
|
const struct nvmem_config *config)
|
|
{
|
|
}
|
|
#endif /* CONFIG_NVMEM_SYSFS */
|
|
|
|
#endif /* _DRIVERS_NVMEM_H */
|