mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
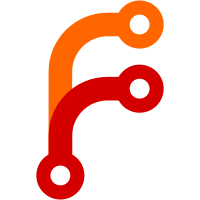
Currently, none of the X1E80100 supported boards upstream have enabled
DP. As for USB, the reason it is not broken when it's obvious that the
offsets are wrong is because the only difference with respect to USB is
the difference in register name. The V6 uses QPHY_V6_PCS_CDR_RESET_TIME
while V6 N4 uses QPHY_V6_N4_PCS_RX_CONFIG. Now, in order for the DP to
work, the DP serdes tables need to be added as they have different
values for V6 N4 when compared to V6 ones, even though they use the same
V6 offsets. While at it, switch swing and pre-emphasis tables to V6 as
well.
Fixes: d7b3579f84
("phy: qcom-qmp-combo: Add x1e80100 USB/DP combo phys")
Co-developed-by: Kuogee Hsieh <quic_khsieh@quicinc.com>
Signed-off-by: Kuogee Hsieh <quic_khsieh@quicinc.com>
Signed-off-by: Abel Vesa <abel.vesa@linaro.org>
Reviewed-by: Dmitry Baryshkov <dmitry.baryshkov@linaro.org>
Link: https://lore.kernel.org/r/20240527-x1e80100-phy-qualcomm-combo-fix-dp-v1-3-be8a0b882117@linaro.org
Signed-off-by: Vinod Koul <vkoul@kernel.org>
3839 lines
136 KiB
C
3839 lines
136 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2017, The Linux Foundation. All rights reserved.
|
|
*/
|
|
|
|
#include <linux/clk.h>
|
|
#include <linux/clk-provider.h>
|
|
#include <linux/delay.h>
|
|
#include <linux/err.h>
|
|
#include <linux/io.h>
|
|
#include <linux/iopoll.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/of.h>
|
|
#include <linux/of_address.h>
|
|
#include <linux/phy/phy.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/regulator/consumer.h>
|
|
#include <linux/reset.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/usb/typec.h>
|
|
#include <linux/usb/typec_mux.h>
|
|
|
|
#include <drm/bridge/aux-bridge.h>
|
|
|
|
#include <dt-bindings/phy/phy-qcom-qmp.h>
|
|
|
|
#include "phy-qcom-qmp-common.h"
|
|
|
|
#include "phy-qcom-qmp.h"
|
|
#include "phy-qcom-qmp-pcs-misc-v3.h"
|
|
#include "phy-qcom-qmp-pcs-usb-v4.h"
|
|
#include "phy-qcom-qmp-pcs-usb-v5.h"
|
|
#include "phy-qcom-qmp-pcs-usb-v6.h"
|
|
|
|
#include "phy-qcom-qmp-dp-com-v3.h"
|
|
|
|
#include "phy-qcom-qmp-dp-phy.h"
|
|
#include "phy-qcom-qmp-dp-phy-v3.h"
|
|
#include "phy-qcom-qmp-dp-phy-v4.h"
|
|
#include "phy-qcom-qmp-dp-phy-v5.h"
|
|
#include "phy-qcom-qmp-dp-phy-v6.h"
|
|
|
|
/* QPHY_V3_DP_COM_RESET_OVRD_CTRL register bits */
|
|
/* DP PHY soft reset */
|
|
#define SW_DPPHY_RESET BIT(0)
|
|
/* mux to select DP PHY reset control, 0:HW control, 1: software reset */
|
|
#define SW_DPPHY_RESET_MUX BIT(1)
|
|
/* USB3 PHY soft reset */
|
|
#define SW_USB3PHY_RESET BIT(2)
|
|
/* mux to select USB3 PHY reset control, 0:HW control, 1: software reset */
|
|
#define SW_USB3PHY_RESET_MUX BIT(3)
|
|
|
|
/* QPHY_V3_DP_COM_PHY_MODE_CTRL register bits */
|
|
#define USB3_MODE BIT(0) /* enables USB3 mode */
|
|
#define DP_MODE BIT(1) /* enables DP mode */
|
|
|
|
/* QPHY_V3_DP_COM_TYPEC_CTRL register bits */
|
|
#define SW_PORTSELECT_VAL BIT(0)
|
|
#define SW_PORTSELECT_MUX BIT(1)
|
|
|
|
#define PHY_INIT_COMPLETE_TIMEOUT 10000
|
|
|
|
/* set of registers with offsets different per-PHY */
|
|
enum qphy_reg_layout {
|
|
/* PCS registers */
|
|
QPHY_SW_RESET,
|
|
QPHY_START_CTRL,
|
|
QPHY_PCS_STATUS,
|
|
QPHY_PCS_AUTONOMOUS_MODE_CTRL,
|
|
QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR,
|
|
QPHY_PCS_POWER_DOWN_CONTROL,
|
|
|
|
QPHY_COM_RESETSM_CNTRL,
|
|
QPHY_COM_C_READY_STATUS,
|
|
QPHY_COM_CMN_STATUS,
|
|
QPHY_COM_BIAS_EN_CLKBUFLR_EN,
|
|
|
|
QPHY_DP_PHY_STATUS,
|
|
QPHY_DP_PHY_VCO_DIV,
|
|
|
|
QPHY_TX_TX_POL_INV,
|
|
QPHY_TX_TX_DRV_LVL,
|
|
QPHY_TX_TX_EMP_POST1_LVL,
|
|
QPHY_TX_HIGHZ_DRVR_EN,
|
|
QPHY_TX_TRANSCEIVER_BIAS_EN,
|
|
|
|
/* Keep last to ensure regs_layout arrays are properly initialized */
|
|
QPHY_LAYOUT_SIZE
|
|
};
|
|
|
|
static const unsigned int qmp_v3_usb3phy_regs_layout[QPHY_LAYOUT_SIZE] = {
|
|
[QPHY_SW_RESET] = QPHY_V3_PCS_SW_RESET,
|
|
[QPHY_START_CTRL] = QPHY_V3_PCS_START_CONTROL,
|
|
[QPHY_PCS_STATUS] = QPHY_V3_PCS_PCS_STATUS,
|
|
[QPHY_PCS_POWER_DOWN_CONTROL] = QPHY_V3_PCS_POWER_DOWN_CONTROL,
|
|
[QPHY_PCS_AUTONOMOUS_MODE_CTRL] = QPHY_V3_PCS_AUTONOMOUS_MODE_CTRL,
|
|
[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR] = QPHY_V3_PCS_LFPS_RXTERM_IRQ_CLEAR,
|
|
|
|
[QPHY_COM_RESETSM_CNTRL] = QSERDES_V3_COM_RESETSM_CNTRL,
|
|
[QPHY_COM_C_READY_STATUS] = QSERDES_V3_COM_C_READY_STATUS,
|
|
[QPHY_COM_CMN_STATUS] = QSERDES_V3_COM_CMN_STATUS,
|
|
[QPHY_COM_BIAS_EN_CLKBUFLR_EN] = QSERDES_V3_COM_BIAS_EN_CLKBUFLR_EN,
|
|
|
|
[QPHY_DP_PHY_STATUS] = QSERDES_V3_DP_PHY_STATUS,
|
|
[QPHY_DP_PHY_VCO_DIV] = QSERDES_V3_DP_PHY_VCO_DIV,
|
|
|
|
[QPHY_TX_TX_POL_INV] = QSERDES_V3_TX_TX_POL_INV,
|
|
[QPHY_TX_TX_DRV_LVL] = QSERDES_V3_TX_TX_DRV_LVL,
|
|
[QPHY_TX_TX_EMP_POST1_LVL] = QSERDES_V3_TX_TX_EMP_POST1_LVL,
|
|
[QPHY_TX_HIGHZ_DRVR_EN] = QSERDES_V3_TX_HIGHZ_DRVR_EN,
|
|
[QPHY_TX_TRANSCEIVER_BIAS_EN] = QSERDES_V3_TX_TRANSCEIVER_BIAS_EN,
|
|
};
|
|
|
|
static const unsigned int qmp_v45_usb3phy_regs_layout[QPHY_LAYOUT_SIZE] = {
|
|
[QPHY_SW_RESET] = QPHY_V4_PCS_SW_RESET,
|
|
[QPHY_START_CTRL] = QPHY_V4_PCS_START_CONTROL,
|
|
[QPHY_PCS_STATUS] = QPHY_V4_PCS_PCS_STATUS1,
|
|
[QPHY_PCS_POWER_DOWN_CONTROL] = QPHY_V4_PCS_POWER_DOWN_CONTROL,
|
|
|
|
/* In PCS_USB */
|
|
[QPHY_PCS_AUTONOMOUS_MODE_CTRL] = QPHY_V4_PCS_USB3_AUTONOMOUS_MODE_CTRL,
|
|
[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR] = QPHY_V4_PCS_USB3_LFPS_RXTERM_IRQ_CLEAR,
|
|
|
|
[QPHY_COM_RESETSM_CNTRL] = QSERDES_V4_COM_RESETSM_CNTRL,
|
|
[QPHY_COM_C_READY_STATUS] = QSERDES_V4_COM_C_READY_STATUS,
|
|
[QPHY_COM_CMN_STATUS] = QSERDES_V4_COM_CMN_STATUS,
|
|
[QPHY_COM_BIAS_EN_CLKBUFLR_EN] = QSERDES_V4_COM_BIAS_EN_CLKBUFLR_EN,
|
|
|
|
[QPHY_DP_PHY_STATUS] = QSERDES_V4_DP_PHY_STATUS,
|
|
[QPHY_DP_PHY_VCO_DIV] = QSERDES_V4_DP_PHY_VCO_DIV,
|
|
|
|
[QPHY_TX_TX_POL_INV] = QSERDES_V4_TX_TX_POL_INV,
|
|
[QPHY_TX_TX_DRV_LVL] = QSERDES_V4_TX_TX_DRV_LVL,
|
|
[QPHY_TX_TX_EMP_POST1_LVL] = QSERDES_V4_TX_TX_EMP_POST1_LVL,
|
|
[QPHY_TX_HIGHZ_DRVR_EN] = QSERDES_V4_TX_HIGHZ_DRVR_EN,
|
|
[QPHY_TX_TRANSCEIVER_BIAS_EN] = QSERDES_V4_TX_TRANSCEIVER_BIAS_EN,
|
|
};
|
|
|
|
static const unsigned int qmp_v5_5nm_usb3phy_regs_layout[QPHY_LAYOUT_SIZE] = {
|
|
[QPHY_SW_RESET] = QPHY_V5_PCS_SW_RESET,
|
|
[QPHY_START_CTRL] = QPHY_V5_PCS_START_CONTROL,
|
|
[QPHY_PCS_STATUS] = QPHY_V5_PCS_PCS_STATUS1,
|
|
[QPHY_PCS_POWER_DOWN_CONTROL] = QPHY_V5_PCS_POWER_DOWN_CONTROL,
|
|
|
|
/* In PCS_USB */
|
|
[QPHY_PCS_AUTONOMOUS_MODE_CTRL] = QPHY_V5_PCS_USB3_AUTONOMOUS_MODE_CTRL,
|
|
[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR] = QPHY_V5_PCS_USB3_LFPS_RXTERM_IRQ_CLEAR,
|
|
|
|
[QPHY_COM_RESETSM_CNTRL] = QSERDES_V5_COM_RESETSM_CNTRL,
|
|
[QPHY_COM_C_READY_STATUS] = QSERDES_V5_COM_C_READY_STATUS,
|
|
[QPHY_COM_CMN_STATUS] = QSERDES_V5_COM_CMN_STATUS,
|
|
[QPHY_COM_BIAS_EN_CLKBUFLR_EN] = QSERDES_V5_COM_BIAS_EN_CLKBUFLR_EN,
|
|
|
|
[QPHY_DP_PHY_STATUS] = QSERDES_V5_DP_PHY_STATUS,
|
|
[QPHY_DP_PHY_VCO_DIV] = QSERDES_V5_DP_PHY_VCO_DIV,
|
|
|
|
[QPHY_TX_TX_POL_INV] = QSERDES_V5_5NM_TX_TX_POL_INV,
|
|
[QPHY_TX_TX_DRV_LVL] = QSERDES_V5_5NM_TX_TX_DRV_LVL,
|
|
[QPHY_TX_TX_EMP_POST1_LVL] = QSERDES_V5_5NM_TX_TX_EMP_POST1_LVL,
|
|
[QPHY_TX_HIGHZ_DRVR_EN] = QSERDES_V5_5NM_TX_HIGHZ_DRVR_EN,
|
|
[QPHY_TX_TRANSCEIVER_BIAS_EN] = QSERDES_V5_5NM_TX_TRANSCEIVER_BIAS_EN,
|
|
};
|
|
|
|
static const unsigned int qmp_v6_usb3phy_regs_layout[QPHY_LAYOUT_SIZE] = {
|
|
[QPHY_SW_RESET] = QPHY_V6_PCS_SW_RESET,
|
|
[QPHY_START_CTRL] = QPHY_V6_PCS_START_CONTROL,
|
|
[QPHY_PCS_STATUS] = QPHY_V6_PCS_PCS_STATUS1,
|
|
[QPHY_PCS_POWER_DOWN_CONTROL] = QPHY_V6_PCS_POWER_DOWN_CONTROL,
|
|
|
|
/* In PCS_USB */
|
|
[QPHY_PCS_AUTONOMOUS_MODE_CTRL] = QPHY_V6_PCS_USB3_AUTONOMOUS_MODE_CTRL,
|
|
[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR] = QPHY_V6_PCS_USB3_LFPS_RXTERM_IRQ_CLEAR,
|
|
|
|
[QPHY_COM_RESETSM_CNTRL] = QSERDES_V6_COM_RESETSM_CNTRL,
|
|
[QPHY_COM_C_READY_STATUS] = QSERDES_V6_COM_C_READY_STATUS,
|
|
[QPHY_COM_CMN_STATUS] = QSERDES_V6_COM_CMN_STATUS,
|
|
[QPHY_COM_BIAS_EN_CLKBUFLR_EN] = QSERDES_V6_COM_PLL_BIAS_EN_CLK_BUFLR_EN,
|
|
|
|
[QPHY_DP_PHY_STATUS] = QSERDES_V6_DP_PHY_STATUS,
|
|
[QPHY_DP_PHY_VCO_DIV] = QSERDES_V6_DP_PHY_VCO_DIV,
|
|
|
|
[QPHY_TX_TX_POL_INV] = QSERDES_V6_TX_TX_POL_INV,
|
|
[QPHY_TX_TX_DRV_LVL] = QSERDES_V6_TX_TX_DRV_LVL,
|
|
[QPHY_TX_TX_EMP_POST1_LVL] = QSERDES_V6_TX_TX_EMP_POST1_LVL,
|
|
[QPHY_TX_HIGHZ_DRVR_EN] = QSERDES_V6_TX_HIGHZ_DRVR_EN,
|
|
[QPHY_TX_TRANSCEIVER_BIAS_EN] = QSERDES_V6_TX_TRANSCEIVER_BIAS_EN,
|
|
};
|
|
|
|
static const unsigned int qmp_v6_n4_usb3phy_regs_layout[QPHY_LAYOUT_SIZE] = {
|
|
[QPHY_SW_RESET] = QPHY_V6_N4_PCS_SW_RESET,
|
|
[QPHY_START_CTRL] = QPHY_V6_N4_PCS_START_CONTROL,
|
|
[QPHY_PCS_STATUS] = QPHY_V6_N4_PCS_PCS_STATUS1,
|
|
[QPHY_PCS_POWER_DOWN_CONTROL] = QPHY_V6_N4_PCS_POWER_DOWN_CONTROL,
|
|
|
|
/* In PCS_USB */
|
|
[QPHY_PCS_AUTONOMOUS_MODE_CTRL] = QPHY_V6_PCS_USB3_AUTONOMOUS_MODE_CTRL,
|
|
[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR] = QPHY_V6_PCS_USB3_LFPS_RXTERM_IRQ_CLEAR,
|
|
|
|
[QPHY_COM_RESETSM_CNTRL] = QSERDES_V6_COM_RESETSM_CNTRL,
|
|
[QPHY_COM_C_READY_STATUS] = QSERDES_V6_COM_C_READY_STATUS,
|
|
[QPHY_COM_CMN_STATUS] = QSERDES_V6_COM_CMN_STATUS,
|
|
[QPHY_COM_BIAS_EN_CLKBUFLR_EN] = QSERDES_V6_COM_PLL_BIAS_EN_CLK_BUFLR_EN,
|
|
|
|
[QPHY_DP_PHY_STATUS] = QSERDES_V6_DP_PHY_STATUS,
|
|
[QPHY_DP_PHY_VCO_DIV] = QSERDES_V6_DP_PHY_VCO_DIV,
|
|
|
|
[QPHY_TX_TX_POL_INV] = QSERDES_V6_N4_TX_TX_POL_INV,
|
|
[QPHY_TX_TX_DRV_LVL] = QSERDES_V6_N4_TX_TX_DRV_LVL,
|
|
[QPHY_TX_TX_EMP_POST1_LVL] = QSERDES_V6_N4_TX_TX_EMP_POST1_LVL,
|
|
[QPHY_TX_HIGHZ_DRVR_EN] = QSERDES_V6_N4_TX_HIGHZ_DRVR_EN,
|
|
[QPHY_TX_TRANSCEIVER_BIAS_EN] = QSERDES_V6_N4_TX_TRANSCEIVER_BIAS_EN,
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_usb3_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_PLL_IVCO, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SYSCLK_EN_SEL, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_BIAS_EN_CLKBUFLR_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CLK_SELECT, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SYS_CLK_CTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_RESETSM_CNTRL2, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CMN_CONFIG, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SVS_MODE_CLK_SEL, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_HSCLK_SEL, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DEC_START_MODE0, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START1_MODE0, 0xab),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START2_MODE0, 0xea),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START3_MODE0, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CP_CTRL_MODE0, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_INTEGLOOP_GAIN1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_INTEGLOOP_GAIN0_MODE0, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_VCO_TUNE2_MODE0, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_VCO_TUNE1_MODE0, 0xc9),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CORECLK_DIV_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP3_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP2_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP1_MODE0, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP_EN, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CORE_CLK_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP_CFG, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_VCO_TUNE_MAP, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SYSCLK_BUF_ENABLE, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_PER1, 0x31),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_PER2, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_ADJ_PER1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_ADJ_PER2, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_STEP_SIZE1, 0x85),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SSC_STEP_SIZE2, 0x07),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_usb3_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_HIGHZ_DRVR_EN, 0x10),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_RCV_DETECT_LVL_2, 0x12),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_LANE_MODE_1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_RES_CODE_LANE_OFFSET_RX, 0x09),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_RES_CODE_LANE_OFFSET_TX, 0x06),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SVS_MODE_CLK_SEL, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SYSCLK_EN_SEL, 0x37),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SYS_CLK_CTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CLK_ENABLE1, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_SYSCLK_BUF_ENABLE, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CLK_SELECT, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CMN_CONFIG, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_INTEGLOOP_GAIN0_MODE0, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_INTEGLOOP_GAIN1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_VCO_TUNE_MAP, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP3_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_BG_TIMER, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CORECLK_DIV_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_VCO_TUNE_CTRL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_BIAS_EN_CLKBUFLR_EN, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CORE_CLK_EN, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_PLL_IVCO, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_CP_CTRL_MODE0, 0x06),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_dp_serdes_tbl_rbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_HSCLK_SEL, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DEC_START_MODE0, 0x69),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START2_MODE0, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START3_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP1_MODE0, 0x6f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP2_MODE0, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP_EN, 0x00),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_dp_serdes_tbl_hbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_HSCLK_SEL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DEC_START_MODE0, 0x69),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START2_MODE0, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START3_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP1_MODE0, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP2_MODE0, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP_EN, 0x00),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_dp_serdes_tbl_hbr2[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_HSCLK_SEL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DEC_START_MODE0, 0x8c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START2_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START3_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP1_MODE0, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP2_MODE0, 0x1c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP_EN, 0x00),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_dp_serdes_tbl_hbr3[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_HSCLK_SEL, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DEC_START_MODE0, 0x69),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START2_MODE0, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_DIV_FRAC_START3_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP1_MODE0, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP2_MODE0, 0x2a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_COM_LOCK_CMP_EN, 0x08),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TRANSCEIVER_BIAS_EN, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_VMODE_CTRL1, 0x40),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_PRE_STALL_LDO_BOOST_EN, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_INTERFACE_SELECT, 0x3d),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_CLKBUF_ENABLE, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_RESET_TSYNC_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TRAN_DRVR_EMP_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_PARRATE_REC_DETECT_IDLE_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TX_INTERFACE_MODE, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TX_BAND, 0x4),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TX_POL_INV, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TX_DRV_LVL, 0x38),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_TX_EMP_POST1_LVL, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_RES_CODE_LANE_OFFSET_TX, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_TX_RES_CODE_LANE_OFFSET_RX, 0x07),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_usb3_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_UCDR_FASTLOCK_FO_GAIN, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQU_ADAPTOR_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQU_ADAPTOR_CNTRL3, 0x4e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQU_ADAPTOR_CNTRL4, 0x18),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQ_OFFSET_ADAPTOR_CNTRL1, 0x77),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_OFFSET_ADAPTOR_CNTRL2, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_SIGDET_CNTRL, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_SIGDET_DEGLITCH_CNTRL, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_UCDR_SO_SATURATION_AND_ENABLE, 0x75),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v3_usb3_pcs_tbl[] = {
|
|
/* FLL settings */
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNTRL2, 0x83),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNT_VAL_L, 0x09),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNT_VAL_H_TOL, 0xa2),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_MAN_CODE, 0x40),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNTRL1, 0x02),
|
|
|
|
/* Lock Det settings */
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LOCK_DETECT_CONFIG1, 0xd1),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LOCK_DETECT_CONFIG2, 0x1f),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LOCK_DETECT_CONFIG3, 0x47),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_POWER_STATE_CONFIG2, 0x1b),
|
|
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RX_SIGDET_LVL, 0xba),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V0, 0x9f),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V1, 0x9f),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V2, 0xb7),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V3, 0x4e),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V4, 0x65),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_LS, 0x6b),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V0, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V0, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V1, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V1, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V2, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V2, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V3, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V3, 0x1d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V4, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V4, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_LS, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_LS, 0x0d),
|
|
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RATE_SLEW_CNTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_PWRUP_RESET_DLY_TIME_AUXCLK, 0x04),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TSYNC_RSYNC_TIME, 0x44),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_PWRUP_RESET_DLY_TIME_AUXCLK, 0x04),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_P1U2_L, 0xe7),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_P1U2_H, 0x03),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_U3_L, 0x40),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_U3_H, 0x00),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RXEQTRAINING_WAIT_TIME, 0x75),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LFPS_TX_ECSTART_EQTLOCK, 0x86),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RXEQTRAINING_RUN_TIME, 0x13),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm6350_usb3_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_UCDR_FASTLOCK_FO_GAIN, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQU_ADAPTOR_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQU_ADAPTOR_CNTRL3, 0x4e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQU_ADAPTOR_CNTRL4, 0x18),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_EQ_OFFSET_ADAPTOR_CNTRL1, 0x77),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_OFFSET_ADAPTOR_CNTRL2, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_SIGDET_CNTRL, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_SIGDET_DEGLITCH_CNTRL, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_RX_MODE_00, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V3_RX_UCDR_SO_SATURATION_AND_ENABLE, 0x75),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm6350_usb3_pcs_tbl[] = {
|
|
/* FLL settings */
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNTRL2, 0x83),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNT_VAL_L, 0x09),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNT_VAL_H_TOL, 0xa2),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_MAN_CODE, 0x40),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_FLL_CNTRL1, 0x02),
|
|
|
|
/* Lock Det settings */
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LOCK_DETECT_CONFIG1, 0xd1),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LOCK_DETECT_CONFIG2, 0x1f),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LOCK_DETECT_CONFIG3, 0x47),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_POWER_STATE_CONFIG2, 0x1b),
|
|
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RX_SIGDET_LVL, 0xcc),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V0, 0x9f),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V1, 0x9f),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V2, 0xb7),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V3, 0x4e),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_V4, 0x65),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXMGN_LS, 0x6b),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V0, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V0, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V1, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V1, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V2, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V2, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V3, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V3, 0x1d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_V4, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_V4, 0x0d),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M6DB_LS, 0x15),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TXDEEMPH_M3P5DB_LS, 0x0d),
|
|
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RATE_SLEW_CNTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_PWRUP_RESET_DLY_TIME_AUXCLK, 0x04),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_TSYNC_RSYNC_TIME, 0x44),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_PWRUP_RESET_DLY_TIME_AUXCLK, 0x04),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_P1U2_L, 0xe7),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_P1U2_H, 0x03),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_U3_L, 0x40),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RCVR_DTCT_DLY_U3_H, 0x00),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RXEQTRAINING_WAIT_TIME, 0x75),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LFPS_TX_ECSTART_EQTLOCK, 0x86),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_RXEQTRAINING_RUN_TIME, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_LFPS_DET_HIGH_COUNT_VAL, 0x04),
|
|
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V3_PCS_REFGEN_REQ_CONFIG2, 0x60),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8150_usb3_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_PER1, 0x31),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_PER2, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_STEP_SIZE1_MODE0, 0xde),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_STEP_SIZE2_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_STEP_SIZE1_MODE1, 0xde),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SSC_STEP_SIZE2_MODE1, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYSCLK_BUF_ENABLE, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CMN_IPTRIM, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CP_CTRL_MODE0, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CP_CTRL_MODE1, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_RCTRL_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_CCTRL_MODE1, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYSCLK_EN_SEL, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP_EN, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP1_MODE0, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP2_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP1_MODE1, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP2_MODE1, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DEC_START_MODE0, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DEC_START_MODE1, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START1_MODE0, 0xab),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START2_MODE0, 0xea),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START3_MODE0, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE_MAP, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START1_MODE1, 0xab),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START2_MODE1, 0xea),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START3_MODE1, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE1_MODE0, 0x24),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE1_MODE1, 0x24),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE2_MODE1, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_HSCLK_SEL, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CORECLK_DIV_MODE1, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0xca),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x1e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIN_VCOCAL_CMP_CODE1_MODE1, 0xca),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIN_VCOCAL_CMP_CODE2_MODE1, 0x1e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIN_VCOCAL_HSCLK_SEL, 0x11),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8150_usb3_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_TX, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_RX, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_LANE_MODE_1, 0xd5),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RCV_DETECT_LVL_2, 0x12),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_PI_QEC_CTRL, 0x20),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8150_usb3_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SO_GAIN, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_FASTLOCK_FO_GAIN, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SO_SATURATION_AND_ENABLE, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_FASTLOCK_COUNT_LOW, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_FASTLOCK_COUNT_HIGH, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_PI_CONTROLS, 0x99),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_THRESH1, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_THRESH2, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_GAIN1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_GAIN2, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_VGA_CAL_CNTRL1, 0x54),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_VGA_CAL_CNTRL2, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQU_ADAPTOR_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQU_ADAPTOR_CNTRL3, 0x4a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQU_ADAPTOR_CNTRL4, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_IDAC_TSETTLE_LOW, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_IDAC_TSETTLE_HIGH, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQ_OFFSET_ADAPTOR_CNTRL1, 0x77),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_SIGDET_CNTRL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_SIGDET_DEGLITCH_CNTRL, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_LOW, 0xbf),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH, 0xbf),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH2, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH3, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH4, 0x94),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_LOW, 0xdc),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH, 0xdc),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH2, 0x5c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH3, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH4, 0xb3),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_DFE_EN_TIMER, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_DFE_CTLE_POST_CAL_OFFSET, 0x38),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_AUX_DATA_TCOARSE_TFINE, 0xa0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_DCC_CTRL1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_GM_CAL, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_VTH_CODE, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8150_usb3_pcs_tbl[] = {
|
|
/* Lock Det settings */
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG1, 0xd0),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG2, 0x07),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG6, 0x13),
|
|
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_RX_SIGDET_LVL, 0xaa),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_CDR_RESET_TIME, 0x0a),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_ALIGN_DETECT_CONFIG1, 0x88),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_ALIGN_DETECT_CONFIG2, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_PCS_TX_RX_CONFIG, 0x0c),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_EQ_CONFIG1, 0x4b),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_EQ_CONFIG5, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8150_usb3_pcs_usb_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_USB3_LFPS_DET_HIGH_COUNT_VAL, 0xf8),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_USB3_RXEQTRAINING_DFE_TIME_S2, 0x07),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8250_usb3_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_TX, 0x60),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_RX, 0x60),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_OFFSET_TX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_OFFSET_RX, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_LANE_MODE_1, 0xd5),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RCV_DETECT_LVL_2, 0x12),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V4_TX_PI_QEC_CTRL, 0x40, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V4_TX_PI_QEC_CTRL, 0x54, 2),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8250_usb3_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SO_GAIN, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_FASTLOCK_FO_GAIN, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SO_SATURATION_AND_ENABLE, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_FASTLOCK_COUNT_LOW, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_FASTLOCK_COUNT_HIGH, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_PI_CONTROLS, 0x99),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_THRESH1, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_THRESH2, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_GAIN1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_UCDR_SB2_GAIN2, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_VGA_CAL_CNTRL1, 0x54),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_VGA_CAL_CNTRL2, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQU_ADAPTOR_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQU_ADAPTOR_CNTRL3, 0x4a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQU_ADAPTOR_CNTRL4, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_IDAC_TSETTLE_LOW, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_IDAC_TSETTLE_HIGH, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_EQ_OFFSET_ADAPTOR_CNTRL1, 0x77),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_SIGDET_CNTRL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_SIGDET_DEGLITCH_CNTRL, 0x0e),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V4_RX_RX_MODE_00_LOW, 0xff, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V4_RX_RX_MODE_00_LOW, 0x7f, 2),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V4_RX_RX_MODE_00_HIGH, 0x7f, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V4_RX_RX_MODE_00_HIGH, 0xff, 2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH2, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH3, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_00_HIGH4, 0x97),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_LOW, 0xdc),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH, 0xdc),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH2, 0x5c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH3, 0x7b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_RX_MODE_01_HIGH4, 0xb4),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_DFE_EN_TIMER, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_DFE_CTLE_POST_CAL_OFFSET, 0x38),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_AUX_DATA_TCOARSE_TFINE, 0xa0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_DCC_CTRL1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_GM_CAL, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_RX_VTH_CODE, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8250_usb3_pcs_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG1, 0xd0),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG2, 0x07),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG3, 0x20),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG6, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_RX_SIGDET_LVL, 0xa9),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_CDR_RESET_TIME, 0x0a),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_ALIGN_DETECT_CONFIG1, 0x88),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_ALIGN_DETECT_CONFIG2, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_PCS_TX_RX_CONFIG, 0x0c),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_EQ_CONFIG1, 0x4b),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_EQ_CONFIG5, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8250_usb3_pcs_usb_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_USB3_LFPS_DET_HIGH_COUNT_VAL, 0xf8),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_USB3_RXEQTRAINING_DFE_TIME_S2, 0x07),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8350_usb3_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RES_CODE_LANE_TX, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RES_CODE_LANE_RX, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RES_CODE_LANE_OFFSET_TX, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RES_CODE_LANE_OFFSET_RX, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_LANE_MODE_1, 0x35),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_LANE_MODE_3, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_LANE_MODE_4, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_LANE_MODE_5, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RCV_DETECT_LVL_2, 0x12),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_PI_QEC_CTRL, 0x21),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8350_usb3_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_FO_GAIN, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_SO_GAIN, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_FASTLOCK_FO_GAIN, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_SO_SATURATION_AND_ENABLE, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_FASTLOCK_COUNT_LOW, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_FASTLOCK_COUNT_HIGH, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_PI_CONTROLS, 0x99),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_SB2_THRESH1, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_SB2_THRESH2, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_SB2_GAIN1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_UCDR_SB2_GAIN2, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_VGA_CAL_CNTRL1, 0x54),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_VGA_CAL_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_EQU_ADAPTOR_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_EQU_ADAPTOR_CNTRL3, 0x4a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_EQU_ADAPTOR_CNTRL4, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_IDAC_TSETTLE_LOW, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_IDAC_TSETTLE_HIGH, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_EQ_OFFSET_ADAPTOR_CNTRL1, 0x47),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_SIGDET_CNTRL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_SIGDET_DEGLITCH_CNTRL, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_00_LOW, 0xbb),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_00_HIGH, 0x7b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_00_HIGH2, 0xbb),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V5_RX_RX_MODE_00_HIGH3, 0x3d, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V5_RX_RX_MODE_00_HIGH3, 0x3c, 2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_00_HIGH4, 0xdb),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_01_LOW, 0x64),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_01_HIGH, 0x24),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_01_HIGH2, 0xd2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_01_HIGH3, 0x13),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_RX_MODE_01_HIGH4, 0xa9),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_DFE_EN_TIMER, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_DFE_CTLE_POST_CAL_OFFSET, 0x38),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_AUX_DATA_TCOARSE_TFINE, 0xa0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_DCC_CTRL1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_GM_CAL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_RX_VTH_CODE, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8350_usb3_pcs_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_RCVR_DTCT_DLY_P1U2_L, 0xe7),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_RCVR_DTCT_DLY_P1U2_H, 0x03),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG1, 0xd0),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG2, 0x07),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG3, 0x20),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_LOCK_DETECT_CONFIG6, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_RX_SIGDET_LVL, 0xaa),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_CDR_RESET_TIME, 0x0a),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_ALIGN_DETECT_CONFIG1, 0x88),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_ALIGN_DETECT_CONFIG2, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_PCS_TX_RX_CONFIG, 0x0c),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_EQ_CONFIG1, 0x4b),
|
|
QMP_PHY_INIT_CFG(QPHY_V4_PCS_EQ_CONFIG5, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8350_usb3_pcs_usb_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_USB3_RCVR_DTCT_DLY_U3_L, 0x40),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_USB3_RCVR_DTCT_DLY_U3_H, 0x00),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_USB3_LFPS_DET_HIGH_COUNT_VAL, 0xf8),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_USB3_RXEQTRAINING_DFE_TIME_S2, 0x07),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8550_usb3_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE1, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE1, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CP_CTRL_MODE1, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_RCTRL_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CCTRL_MODE1, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CORECLK_DIV_MODE1, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE1, 0x41),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE1, 0x41),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MSB_MODE1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START1_MODE1, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE1, 0x75),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE1, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE1_MODE1, 0x25),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE2_MODE1, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE1, 0x5c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE1, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x5c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE0, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE0, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CP_CTRL_MODE0, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x41),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MSB_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START1_MODE0, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0x75),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE1_MODE0, 0x25),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE2_MODE0, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BG_TIMER, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER1, 0x62),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER2, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_BUF_ENABLE, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_EN_SEL, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_CFG, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_MAP, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CORE_CLK_EN, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CMN_CONFIG_1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_AUTO_GAIN_ADJ_CTRL_1, 0xb6),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_AUTO_GAIN_ADJ_CTRL_2, 0x4b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_AUTO_GAIN_ADJ_CTRL_3, 0x37),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_ADDITIONAL_MISC, 0x0c),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8550_usb3_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RES_CODE_LANE_TX, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RES_CODE_LANE_RX, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RES_CODE_LANE_OFFSET_TX, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RES_CODE_LANE_OFFSET_RX, 0x09),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_LANE_MODE_1, 0xf5),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_LANE_MODE_3, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_LANE_MODE_4, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_LANE_MODE_5, 0x5f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RCV_DETECT_LVL_2, 0x12),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_TX_PI_QEC_CTRL, 0x21, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_TX_PI_QEC_CTRL, 0x05, 2),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8550_usb3_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_FO_GAIN, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_SO_GAIN, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_FASTLOCK_FO_GAIN, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_SO_SATURATION_AND_ENABLE, 0x7f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_FASTLOCK_COUNT_LOW, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_FASTLOCK_COUNT_HIGH, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_PI_CONTROLS, 0x99),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_SB2_THRESH1, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_SB2_THRESH2, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_SB2_GAIN1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_UCDR_SB2_GAIN2, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_AUX_DATA_TCOARSE_TFINE, 0xa0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_VGA_CAL_CNTRL1, 0x54),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_VGA_CAL_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_GM_CAL, 0x13),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_EQU_ADAPTOR_CNTRL2, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_EQU_ADAPTOR_CNTRL3, 0x4a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_EQU_ADAPTOR_CNTRL4, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_IDAC_TSETTLE_LOW, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_IDAC_TSETTLE_HIGH, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_EQ_OFFSET_ADAPTOR_CNTRL1, 0x47),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_SIGDET_CNTRL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_SIGDET_DEGLITCH_CNTRL, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_MODE_01_LOW, 0xdc),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_MODE_01_HIGH, 0x5c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_MODE_01_HIGH2, 0x9c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_MODE_01_HIGH3, 0x1d),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_RX_MODE_01_HIGH4, 0x09),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_DFE_EN_TIMER, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_DFE_CTLE_POST_CAL_OFFSET, 0x38),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_DCC_CTRL1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_VTH_CODE, 0x10),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_SIGDET_CAL_CTRL1, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_RX_SIGDET_CAL_TRIM, 0x08),
|
|
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_LOW, 0x3f, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH, 0xbf, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH2, 0xff, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH3, 0xdf, 1),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH4, 0xed, 1),
|
|
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_LOW, 0xbf, 2),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH, 0xbf, 2),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH2, 0xbf, 2),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH3, 0xdf, 2),
|
|
QMP_PHY_INIT_CFG_LANE(QSERDES_V6_RX_RX_MODE_00_HIGH4, 0xfd, 2),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8550_usb3_pcs_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_LOCK_DETECT_CONFIG1, 0xc4),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_LOCK_DETECT_CONFIG2, 0x89),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_LOCK_DETECT_CONFIG3, 0x20),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_LOCK_DETECT_CONFIG6, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_RX_SIGDET_LVL, 0x99),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_RCVR_DTCT_DLY_P1U2_L, 0xe7),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_RCVR_DTCT_DLY_P1U2_H, 0x03),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_CDR_RESET_TIME, 0x0a),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_ALIGN_DETECT_CONFIG1, 0x88),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_ALIGN_DETECT_CONFIG2, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_PCS_TX_RX_CONFIG, 0x0c),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_EQ_CONFIG1, 0x4b),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_EQ_CONFIG5, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sm8550_usb3_pcs_usb_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_LFPS_DET_HIGH_COUNT_VAL, 0xf8),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_RXEQTRAINING_DFE_TIME_S2, 0x07),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_RCVR_DTCT_DLY_U3_L, 0x40),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_RCVR_DTCT_DLY_U3_H, 0x00),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_POWER_STATE_CONFIG1, 0x68),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v4_dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SVS_MODE_CLK_SEL, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYSCLK_EN_SEL, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYS_CLK_CTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CLK_ENABLE1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYSCLK_BUF_ENABLE, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CLK_SELECT, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_IVCO, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CP_CTRL_MODE0, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CMN_CONFIG, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_INTEGLOOP_GAIN0_MODE0, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_INTEGLOOP_GAIN1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE_MAP, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BG_TIMER, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CORECLK_DIV_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE_CTRL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIAS_EN_CLKBUFLR_EN, 0x17),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CORE_CLK_EN, 0x1f),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v4_dp_serdes_tbl_rbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_HSCLK_SEL, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DEC_START_MODE0, 0x69),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START2_MODE0, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START3_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP1_MODE0, 0x6f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP2_MODE0, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP_EN, 0x04),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v4_dp_serdes_tbl_hbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_HSCLK_SEL, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DEC_START_MODE0, 0x69),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START2_MODE0, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START3_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP1_MODE0, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP2_MODE0, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP_EN, 0x08),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v4_dp_serdes_tbl_hbr2[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_HSCLK_SEL, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DEC_START_MODE0, 0x8c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START2_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START3_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP1_MODE0, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP2_MODE0, 0x1c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP_EN, 0x08),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v4_dp_serdes_tbl_hbr3[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_HSCLK_SEL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DEC_START_MODE0, 0x69),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START2_MODE0, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START3_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP1_MODE0, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP2_MODE0, 0x2a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_LOCK_CMP_EN, 0x08),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v4_dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_VMODE_CTRL1, 0x40),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_PRE_STALL_LDO_BOOST_EN, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_INTERFACE_SELECT, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_CLKBUF_ENABLE, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RESET_TSYNC_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_TRAN_DRVR_EMP_EN, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_PARRATE_REC_DETECT_IDLE_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_TX_INTERFACE_MODE, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_OFFSET_TX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_RES_CODE_LANE_OFFSET_RX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_TX_BAND, 0x4),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_TX_POL_INV, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_TX_DRV_LVL, 0x2a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_TX_TX_EMP_POST1_LVL, 0x20),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v5_dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SVS_MODE_CLK_SEL, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYSCLK_EN_SEL, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYS_CLK_CTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CLK_ENABLE1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_SYSCLK_BUF_ENABLE, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CLK_SELECT, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_IVCO, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_CCTRL_MODE1, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_PLL_RCTRL_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CP_CTRL_MODE0, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CP_CTRL_MODE1, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CMN_CONFIG, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_INTEGLOOP_GAIN0_MODE0, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_INTEGLOOP_GAIN1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE_MAP, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_DIV_FRAC_START1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BG_TIMER, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CORECLK_DIV_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_VCO_TUNE_CTRL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_BIAS_EN_CLKBUFLR_EN, 0x17),
|
|
QMP_PHY_INIT_CFG(QSERDES_V4_COM_CORE_CLK_EN, 0x1f),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v5_dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_VMODE_CTRL1, 0x40),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_PRE_STALL_LDO_BOOST_EN, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_INTERFACE_SELECT, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_CLKBUF_ENABLE, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RESET_TSYNC_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_TRAN_DRVR_EMP_EN, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_PARRATE_REC_DETECT_IDLE_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_TX_INTERFACE_MODE, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RES_CODE_LANE_OFFSET_TX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_RES_CODE_LANE_OFFSET_RX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_TX_TX_BAND, 0x04),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v5_5nm_dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_LANE_MODE_3, 0x51),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_TRANSCEIVER_BIAS_EN, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_VMODE_CTRL1, 0x40),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_PRE_STALL_LDO_BOOST_EN, 0x0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_INTERFACE_SELECT, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_CLKBUF_ENABLE, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_RESET_TSYNC_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_TRAN_DRVR_EMP_EN, 0xf),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_PARRATE_REC_DETECT_IDLE_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_RES_CODE_LANE_OFFSET_TX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_RES_CODE_LANE_OFFSET_RX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_TX_BAND, 0x01),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SVS_MODE_CLK_SEL, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_EN_SEL, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYS_CLK_CTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CLK_ENABLE1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_BUF_ENABLE, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CLK_SELECT, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_IVCO, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CP_CTRL_MODE0, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CMN_CONFIG_1, 0x12),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_INTEGLOOP_GAIN0_MODE0, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_INTEGLOOP_GAIN1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_MAP, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BG_TIMER, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CORE_CLK_DIV_MODE0, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_CTRL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_BIAS_EN_CLK_BUFLR_EN, 0x17),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CORE_CLK_EN, 0x0f),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_n4_dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SVS_MODE_CLK_SEL, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_EN_SEL, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYS_CLK_CTRL, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CLK_ENABLE1, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_BUF_ENABLE, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CLK_SELECT, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_IVCO, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CP_CTRL_MODE0, 0x06),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CMN_CONFIG_1, 0x12),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_INTEGLOOP_GAIN0_MODE0, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_INTEGLOOP_GAIN1_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_MAP, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BG_TIMER, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CORE_CLK_DIV_MODE0, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_CTRL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_BIAS_EN_CLK_BUFLR_EN, 0x17),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CORE_CLK_EN, 0x0f),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_VMODE_CTRL1, 0x40),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_PRE_STALL_LDO_BOOST_EN, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_INTERFACE_SELECT, 0x3b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_CLKBUF_ENABLE, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RESET_TSYNC_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_TRAN_DRVR_EMP_EN, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_PARRATE_REC_DETECT_IDLE_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_TX_INTERFACE_MODE, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RES_CODE_LANE_OFFSET_TX, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_RES_CODE_LANE_OFFSET_RX, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_TX_TX_BAND, 0x4),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_n4_dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_VMODE_CTRL1, 0x40),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_PRE_STALL_LDO_BOOST_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_INTERFACE_SELECT, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_CLKBUF_ENABLE, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_RESET_TSYNC_EN, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_TRAN_DRVR_EMP_EN, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_PARRATE_REC_DETECT_IDLE_EN, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_RES_CODE_LANE_OFFSET_TX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_RES_CODE_LANE_OFFSET_RX, 0x11),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_TX_BAND, 0x1),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_dp_serdes_tbl_rbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x37),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x71),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0c),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_dp_serdes_tbl_hbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x71),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0c),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_dp_serdes_tbl_hbr2[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x46),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x97),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_dp_serdes_tbl_hbr3[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0xc0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x17),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x71),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0c),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_n4_dp_serdes_tbl_rbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x37),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x71),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_ADJ_PER1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER1, 0x6b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER2, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE0, 0x92),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE0, 0x01),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_n4_dp_serdes_tbl_hbr[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x07),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x71),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_ADJ_PER1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER1, 0x6b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER2, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE0, 0x92),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE0, 0x01),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_n4_dp_serdes_tbl_hbr2[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x46),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x97),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x10),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_ADJ_PER1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER1, 0x6b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER2, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE0, 0x18),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE0, 0x02),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl qmp_v6_n4_dp_serdes_tbl_hbr3[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x0b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x17),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE1_MODE0, 0x71),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BIN_VCOCAL_CMP_CODE2_MODE0, 0x0c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_ADJ_PER1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER1, 0x6b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER2, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE0, 0x92),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE0, 0x01),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sc8280xp_usb43dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_PER1, 0x31),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_PER2, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_STEP_SIZE1_MODE0, 0xfd),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_STEP_SIZE2_MODE0, 0x0d),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_STEP_SIZE1_MODE1, 0xfd),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SSC_STEP_SIZE2_MODE1, 0x0d),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SYSCLK_BUF_ENABLE, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_CP_CTRL_MODE0, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_CP_CTRL_MODE1, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_PLL_RCTRL_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_PLL_CCTRL_MODE1, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SYSCLK_EN_SEL, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_LOCK_CMP_EN, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_LOCK_CMP1_MODE0, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_LOCK_CMP2_MODE0, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_LOCK_CMP1_MODE1, 0x34),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_LOCK_CMP2_MODE1, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DEC_START_MODE0, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DEC_START_MSB_MODE0, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DEC_START_MODE1, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DEC_START_MSB_MODE1, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DIV_FRAC_START1_MODE0, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DIV_FRAC_START2_MODE0, 0xd5),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DIV_FRAC_START3_MODE0, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DIV_FRAC_START1_MODE1, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DIV_FRAC_START2_MODE1, 0xd5),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_DIV_FRAC_START3_MODE1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE_MAP, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE1_MODE0, 0xd4),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE2_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE1_MODE1, 0xd4),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE2_MODE1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_HSCLK_SEL, 0x13),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_HSCLK_HS_SWITCH_SEL, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_CORECLK_DIV_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_CORECLK_DIV_MODE1, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_CORE_CLK_EN, 0x60),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_CMN_CONFIG, 0x76),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_PLL_IVCO, 0xff),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_INTEGLOOP_GAIN0_MODE0, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_INTEGLOOP_GAIN0_MODE1, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE_INITVAL2, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_VCO_TUNE_MAXVAL2, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_COM_SVS_MODE_CLK_SEL, 0x0a),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sc8280xp_usb43dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_LANE_MODE_1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_LANE_MODE_2, 0xc2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_LANE_MODE_3, 0x10),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_RES_CODE_LANE_OFFSET_TX, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_TX_RES_CODE_LANE_OFFSET_RX, 0x0a),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sc8280xp_usb43dp_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_SIGDET_CNTRL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_SIGDET_DEGLITCH_CNTRL, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_SIGDET_ENABLES, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B0, 0xd2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B1, 0xd2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B2, 0xdb),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B3, 0x21),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B4, 0x3f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B5, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B6, 0x45),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE_0_1_B7, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B0, 0x6b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B1, 0x63),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B2, 0xb6),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B3, 0x23),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B4, 0x35),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B5, 0x30),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B6, 0x8e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_MODE_RATE2_B7, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_IVCM_CAL_CODE_OVERRIDE, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_IVCM_CAL_CTRL2, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_SUMMER_CAL_SPD_MODE, 0x1b),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_DFE_CTLE_POST_CAL_OFFSET, 0x38),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_UCDR_PI_CONTROLS, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_UCDR_SB2_GAIN2_RATE2, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_RX_IVCM_POSTCAL_OFFSET, 0x7c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_VGA_CAL_CNTRL1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_VGA_CAL_MAN_VAL, 0x0d),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_DFE_DAC_ENABLE1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_DFE_3, 0x45),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_GM_CAL, 0x09),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_UCDR_FO_GAIN_RATE2, 0x09),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_UCDR_SO_GAIN_RATE2, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V5_5NM_RX_Q_PI_INTRINSIC_BIAS_RATE32, 0x3f),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl sc8280xp_usb43dp_pcs_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_RCVR_DTCT_DLY_P1U2_L, 0xe7),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_RCVR_DTCT_DLY_P1U2_H, 0x03),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_LOCK_DETECT_CONFIG1, 0xd0),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_LOCK_DETECT_CONFIG2, 0x07),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_LOCK_DETECT_CONFIG3, 0x20),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_LOCK_DETECT_CONFIG6, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_RX_SIGDET_LVL, 0xaa),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_RX_CONFIG, 0x0a),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_ALIGN_DETECT_CONFIG1, 0x88),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_ALIGN_DETECT_CONFIG2, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_PCS_TX_RX_CONFIG, 0x0c),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_EQ_CONFIG1, 0x4b),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_EQ_CONFIG5, 0x10),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_USB3_LFPS_DET_HIGH_COUNT_VAL, 0xf8),
|
|
QMP_PHY_INIT_CFG(QPHY_V5_PCS_USB3_RXEQTRAINING_DFE_TIME_S2, 0x07),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl x1e80100_usb43dp_serdes_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_EN_CENTER, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER1, 0x62),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_PER2, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE0, 0xc2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE0, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE1_MODE1, 0xc2),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SSC_STEP_SIZE2_MODE1, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_BUF_ENABLE, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CP_CTRL_MODE0, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CP_CTRL_MODE1, 0x02),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_RCTRL_MODE0, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_RCTRL_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CCTRL_MODE0, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CCTRL_MODE1, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SYSCLK_EN_SEL, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_EN, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP_CFG, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE0, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE0, 0x1a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP1_MODE1, 0x16),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_LOCK_CMP2_MODE1, 0x41),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE0, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MSB_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MODE1, 0x82),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DEC_START_MSB_MODE1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START1_MODE0, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE0, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE0, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START1_MODE1, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START2_MODE1, 0x55),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_DIV_FRAC_START3_MODE1, 0x03),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_MAP, 0x14),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE1_MODE0, 0xba),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE2_MODE0, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE1_MODE1, 0xba),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE2_MODE1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_SEL_1, 0x13),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_HSCLK_HS_SWITCH_SEL_1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_CORE_CLK_DIV_MODE0, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CORECLK_DIV_MODE1, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CORE_CLK_EN, 0xa0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_CMN_CONFIG_1, 0x76),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_IVCO, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_PLL_IVCO_MODE1, 0x0f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_INTEGLOOP_GAIN0_MODE0, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_INTEGLOOP_GAIN0_MODE1, 0x20),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_INITVAL2, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_VCO_TUNE_MAXVAL2, 0x01),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_SVS_MODE_CLK_SEL, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_COM_BG_TIMER, 0x0a),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl x1e80100_usb43dp_tx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_LANE_MODE_1, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_LANE_MODE_2, 0x50),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_LANE_MODE_3, 0x50),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_RES_CODE_LANE_OFFSET_TX, 0x1f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_TX_RES_CODE_LANE_OFFSET_RX, 0x0a),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl x1e80100_usb43dp_rx_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_SIGDET_CNTRL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_SIGDET_DEGLITCH_CNTRL, 0x0e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_SIGDET_ENABLES, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B0, 0xc3),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B1, 0xc3),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B2, 0xd8),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B3, 0x9e),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B4, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B5, 0xb6),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE_0_1_B6, 0x64),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B0, 0xd6),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B1, 0xee),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B2, 0x18),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B3, 0x9a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B4, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B5, 0x36),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_MODE_RATE2_B6, 0xe3),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_IVCM_CAL_CODE_OVERRIDE, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_RX_IVCM_CAL_CTRL2, 0x80),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_RX_SUMMER_CAL_SPD_MODE, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_DFE_CTLE_POST_CAL_OFFSET, 0x08),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_UCDR_PI_CONTROLS, 0x15),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_UCDR_PI_CTRL1, 0xd0),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_UCDR_PI_CTRL2, 0x48),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_UCDR_SB2_GAIN2_RATE2, 0x0a),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_RX_IVCM_POSTCAL_OFFSET, 0x7c),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_VGA_CAL_CNTRL1, 0x00),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_VGA_CAL_MAN_VAL, 0x04),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_DFE_DAC_ENABLE1, 0x88),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_DFE_3, 0x45),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_GM_CAL, 0x0d),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_UCDR_FO_GAIN_RATE2, 0x09),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_UCDR_SO_GAIN_RATE2, 0x05),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_Q_PI_INTRINSIC_BIAS_RATE32, 0x2f),
|
|
QMP_PHY_INIT_CFG(QSERDES_V6_N4_RX_RX_BKUP_CTRL1, 0x14),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl x1e80100_usb43dp_pcs_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_RCVR_DTCT_DLY_P1U2_L, 0xe7),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_RCVR_DTCT_DLY_P1U2_H, 0x03),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_LOCK_DETECT_CONFIG1, 0xc4),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_LOCK_DETECT_CONFIG2, 0x89),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_LOCK_DETECT_CONFIG3, 0x20),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_LOCK_DETECT_CONFIG6, 0x13),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_REFGEN_REQ_CONFIG1, 0x21),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_RX_SIGDET_LVL, 0x55),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_RX_CONFIG, 0x0a),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_ALIGN_DETECT_CONFIG1, 0xd4),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_ALIGN_DETECT_CONFIG2, 0x30),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_PCS_TX_RX_CONFIG, 0x0c),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_EQ_CONFIG1, 0x4b),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_N4_PCS_EQ_CONFIG5, 0x10),
|
|
};
|
|
|
|
static const struct qmp_phy_init_tbl x1e80100_usb43dp_pcs_usb_tbl[] = {
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_LFPS_DET_HIGH_COUNT_VAL, 0xf8),
|
|
QMP_PHY_INIT_CFG(QPHY_V6_PCS_USB3_RXEQTRAINING_DFE_TIME_S2, 0x07),
|
|
};
|
|
|
|
/* list of regulators */
|
|
struct qmp_regulator_data {
|
|
const char *name;
|
|
unsigned int enable_load;
|
|
};
|
|
|
|
static struct qmp_regulator_data qmp_phy_vreg_l[] = {
|
|
{ .name = "vdda-phy", .enable_load = 21800 },
|
|
{ .name = "vdda-pll", .enable_load = 36000 },
|
|
};
|
|
|
|
static const u8 qmp_dp_v3_pre_emphasis_hbr3_hbr2[4][4] = {
|
|
{ 0x00, 0x0c, 0x15, 0x1a },
|
|
{ 0x02, 0x0e, 0x16, 0xff },
|
|
{ 0x02, 0x11, 0xff, 0xff },
|
|
{ 0x04, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v3_voltage_swing_hbr3_hbr2[4][4] = {
|
|
{ 0x02, 0x12, 0x16, 0x1a },
|
|
{ 0x09, 0x19, 0x1f, 0xff },
|
|
{ 0x10, 0x1f, 0xff, 0xff },
|
|
{ 0x1f, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v3_pre_emphasis_hbr_rbr[4][4] = {
|
|
{ 0x00, 0x0c, 0x14, 0x19 },
|
|
{ 0x00, 0x0b, 0x12, 0xff },
|
|
{ 0x00, 0x0b, 0xff, 0xff },
|
|
{ 0x04, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v3_voltage_swing_hbr_rbr[4][4] = {
|
|
{ 0x08, 0x0f, 0x16, 0x1f },
|
|
{ 0x11, 0x1e, 0x1f, 0xff },
|
|
{ 0x19, 0x1f, 0xff, 0xff },
|
|
{ 0x1f, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v4_pre_emphasis_hbr3_hbr2[4][4] = {
|
|
{ 0x00, 0x0c, 0x15, 0x1b },
|
|
{ 0x02, 0x0e, 0x16, 0xff },
|
|
{ 0x02, 0x11, 0xff, 0xff },
|
|
{ 0x04, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v4_pre_emphasis_hbr_rbr[4][4] = {
|
|
{ 0x00, 0x0d, 0x14, 0x1a },
|
|
{ 0x00, 0x0e, 0x15, 0xff },
|
|
{ 0x00, 0x0d, 0xff, 0xff },
|
|
{ 0x03, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v4_voltage_swing_hbr_rbr[4][4] = {
|
|
{ 0x08, 0x0f, 0x16, 0x1f },
|
|
{ 0x11, 0x1e, 0x1f, 0xff },
|
|
{ 0x16, 0x1f, 0xff, 0xff },
|
|
{ 0x1f, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v5_pre_emphasis_hbr3_hbr2[4][4] = {
|
|
{ 0x20, 0x2c, 0x35, 0x3b },
|
|
{ 0x22, 0x2e, 0x36, 0xff },
|
|
{ 0x22, 0x31, 0xff, 0xff },
|
|
{ 0x24, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v5_voltage_swing_hbr3_hbr2[4][4] = {
|
|
{ 0x22, 0x32, 0x36, 0x3a },
|
|
{ 0x29, 0x39, 0x3f, 0xff },
|
|
{ 0x30, 0x3f, 0xff, 0xff },
|
|
{ 0x3f, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v5_pre_emphasis_hbr_rbr[4][4] = {
|
|
{ 0x20, 0x2d, 0x34, 0x3a },
|
|
{ 0x20, 0x2e, 0x35, 0xff },
|
|
{ 0x20, 0x2e, 0xff, 0xff },
|
|
{ 0x24, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v5_voltage_swing_hbr_rbr[4][4] = {
|
|
{ 0x28, 0x2f, 0x36, 0x3f },
|
|
{ 0x31, 0x3e, 0x3f, 0xff },
|
|
{ 0x36, 0x3f, 0xff, 0xff },
|
|
{ 0x3f, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v6_voltage_swing_hbr_rbr[4][4] = {
|
|
{ 0x27, 0x2f, 0x36, 0x3f },
|
|
{ 0x31, 0x3e, 0x3f, 0xff },
|
|
{ 0x36, 0x3f, 0xff, 0xff },
|
|
{ 0x3f, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
static const u8 qmp_dp_v6_pre_emphasis_hbr_rbr[4][4] = {
|
|
{ 0x20, 0x2d, 0x34, 0x3a },
|
|
{ 0x20, 0x2e, 0x35, 0xff },
|
|
{ 0x20, 0x2e, 0xff, 0xff },
|
|
{ 0x22, 0xff, 0xff, 0xff }
|
|
};
|
|
|
|
struct qmp_combo;
|
|
|
|
struct qmp_combo_offsets {
|
|
u16 com;
|
|
u16 txa;
|
|
u16 rxa;
|
|
u16 txb;
|
|
u16 rxb;
|
|
u16 usb3_serdes;
|
|
u16 usb3_pcs_misc;
|
|
u16 usb3_pcs;
|
|
u16 usb3_pcs_usb;
|
|
u16 dp_serdes;
|
|
u16 dp_txa;
|
|
u16 dp_txb;
|
|
u16 dp_dp_phy;
|
|
};
|
|
|
|
struct qmp_phy_cfg {
|
|
const struct qmp_combo_offsets *offsets;
|
|
|
|
/* Init sequence for PHY blocks - serdes, tx, rx, pcs */
|
|
const struct qmp_phy_init_tbl *serdes_tbl;
|
|
int serdes_tbl_num;
|
|
const struct qmp_phy_init_tbl *tx_tbl;
|
|
int tx_tbl_num;
|
|
const struct qmp_phy_init_tbl *rx_tbl;
|
|
int rx_tbl_num;
|
|
const struct qmp_phy_init_tbl *pcs_tbl;
|
|
int pcs_tbl_num;
|
|
const struct qmp_phy_init_tbl *pcs_usb_tbl;
|
|
int pcs_usb_tbl_num;
|
|
|
|
const struct qmp_phy_init_tbl *dp_serdes_tbl;
|
|
int dp_serdes_tbl_num;
|
|
const struct qmp_phy_init_tbl *dp_tx_tbl;
|
|
int dp_tx_tbl_num;
|
|
|
|
/* Init sequence for DP PHY block link rates */
|
|
const struct qmp_phy_init_tbl *serdes_tbl_rbr;
|
|
int serdes_tbl_rbr_num;
|
|
const struct qmp_phy_init_tbl *serdes_tbl_hbr;
|
|
int serdes_tbl_hbr_num;
|
|
const struct qmp_phy_init_tbl *serdes_tbl_hbr2;
|
|
int serdes_tbl_hbr2_num;
|
|
const struct qmp_phy_init_tbl *serdes_tbl_hbr3;
|
|
int serdes_tbl_hbr3_num;
|
|
|
|
/* DP PHY swing and pre_emphasis tables */
|
|
const u8 (*swing_hbr_rbr)[4][4];
|
|
const u8 (*swing_hbr3_hbr2)[4][4];
|
|
const u8 (*pre_emphasis_hbr_rbr)[4][4];
|
|
const u8 (*pre_emphasis_hbr3_hbr2)[4][4];
|
|
|
|
/* DP PHY callbacks */
|
|
int (*configure_dp_phy)(struct qmp_combo *qmp);
|
|
void (*configure_dp_tx)(struct qmp_combo *qmp);
|
|
int (*calibrate_dp_phy)(struct qmp_combo *qmp);
|
|
void (*dp_aux_init)(struct qmp_combo *qmp);
|
|
|
|
/* resets to be requested */
|
|
const char * const *reset_list;
|
|
int num_resets;
|
|
/* regulators to be requested */
|
|
const struct qmp_regulator_data *vreg_list;
|
|
int num_vregs;
|
|
|
|
/* array of registers with different offsets */
|
|
const unsigned int *regs;
|
|
|
|
/* true, if PHY needs delay after POWER_DOWN */
|
|
bool has_pwrdn_delay;
|
|
|
|
/* Offset from PCS to PCS_USB region */
|
|
unsigned int pcs_usb_offset;
|
|
|
|
};
|
|
|
|
struct qmp_combo {
|
|
struct device *dev;
|
|
|
|
const struct qmp_phy_cfg *cfg;
|
|
|
|
void __iomem *com;
|
|
|
|
void __iomem *serdes;
|
|
void __iomem *tx;
|
|
void __iomem *rx;
|
|
void __iomem *pcs;
|
|
void __iomem *tx2;
|
|
void __iomem *rx2;
|
|
void __iomem *pcs_misc;
|
|
void __iomem *pcs_usb;
|
|
|
|
void __iomem *dp_serdes;
|
|
void __iomem *dp_tx;
|
|
void __iomem *dp_tx2;
|
|
void __iomem *dp_dp_phy;
|
|
|
|
struct clk *pipe_clk;
|
|
struct clk_bulk_data *clks;
|
|
int num_clks;
|
|
struct reset_control_bulk_data *resets;
|
|
struct regulator_bulk_data *vregs;
|
|
|
|
struct mutex phy_mutex;
|
|
int init_count;
|
|
|
|
struct phy *usb_phy;
|
|
enum phy_mode mode;
|
|
unsigned int usb_init_count;
|
|
|
|
struct phy *dp_phy;
|
|
unsigned int dp_aux_cfg;
|
|
struct phy_configure_opts_dp dp_opts;
|
|
unsigned int dp_init_count;
|
|
|
|
struct clk_fixed_rate pipe_clk_fixed;
|
|
struct clk_hw dp_link_hw;
|
|
struct clk_hw dp_pixel_hw;
|
|
|
|
struct typec_switch_dev *sw;
|
|
enum typec_orientation orientation;
|
|
};
|
|
|
|
static void qmp_v3_dp_aux_init(struct qmp_combo *qmp);
|
|
static void qmp_v3_configure_dp_tx(struct qmp_combo *qmp);
|
|
static int qmp_v3_configure_dp_phy(struct qmp_combo *qmp);
|
|
static int qmp_v3_calibrate_dp_phy(struct qmp_combo *qmp);
|
|
|
|
static void qmp_v4_dp_aux_init(struct qmp_combo *qmp);
|
|
static void qmp_v4_configure_dp_tx(struct qmp_combo *qmp);
|
|
static int qmp_v4_configure_dp_phy(struct qmp_combo *qmp);
|
|
static int qmp_v4_calibrate_dp_phy(struct qmp_combo *qmp);
|
|
|
|
static inline void qphy_setbits(void __iomem *base, u32 offset, u32 val)
|
|
{
|
|
u32 reg;
|
|
|
|
reg = readl(base + offset);
|
|
reg |= val;
|
|
writel(reg, base + offset);
|
|
|
|
/* ensure that above write is through */
|
|
readl(base + offset);
|
|
}
|
|
|
|
static inline void qphy_clrbits(void __iomem *base, u32 offset, u32 val)
|
|
{
|
|
u32 reg;
|
|
|
|
reg = readl(base + offset);
|
|
reg &= ~val;
|
|
writel(reg, base + offset);
|
|
|
|
/* ensure that above write is through */
|
|
readl(base + offset);
|
|
}
|
|
|
|
/* list of clocks required by phy */
|
|
static const char * const qmp_combo_phy_clk_l[] = {
|
|
"aux", "cfg_ahb", "ref", "com_aux",
|
|
};
|
|
|
|
/* list of resets */
|
|
static const char * const msm8996_usb3phy_reset_l[] = {
|
|
"phy", "common",
|
|
};
|
|
|
|
static const char * const sc7180_usb3phy_reset_l[] = {
|
|
"phy",
|
|
};
|
|
|
|
static const struct qmp_combo_offsets qmp_combo_offsets_v3 = {
|
|
.com = 0x0000,
|
|
.txa = 0x1200,
|
|
.rxa = 0x1400,
|
|
.txb = 0x1600,
|
|
.rxb = 0x1800,
|
|
.usb3_serdes = 0x1000,
|
|
.usb3_pcs_misc = 0x1a00,
|
|
.usb3_pcs = 0x1c00,
|
|
.usb3_pcs_usb = 0x1f00,
|
|
.dp_serdes = 0x2000,
|
|
.dp_txa = 0x2200,
|
|
.dp_txb = 0x2600,
|
|
.dp_dp_phy = 0x2a00,
|
|
};
|
|
|
|
static const struct qmp_combo_offsets qmp_combo_offsets_v5 = {
|
|
.com = 0x0000,
|
|
.txa = 0x0400,
|
|
.rxa = 0x0600,
|
|
.txb = 0x0a00,
|
|
.rxb = 0x0c00,
|
|
.usb3_serdes = 0x1000,
|
|
.usb3_pcs_misc = 0x1200,
|
|
.usb3_pcs = 0x1400,
|
|
.usb3_pcs_usb = 0x1700,
|
|
.dp_serdes = 0x2000,
|
|
.dp_dp_phy = 0x2200,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sc7180_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = qmp_v3_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(qmp_v3_usb3_serdes_tbl),
|
|
.tx_tbl = qmp_v3_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(qmp_v3_usb3_tx_tbl),
|
|
.rx_tbl = qmp_v3_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(qmp_v3_usb3_rx_tbl),
|
|
.pcs_tbl = qmp_v3_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(qmp_v3_usb3_pcs_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v3_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v3_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v3_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v3_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v3_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v3_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v3_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v3_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v3_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v3_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v3_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v3_dp_aux_init,
|
|
.configure_dp_tx = qmp_v3_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v3_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v3_calibrate_dp_phy,
|
|
|
|
.reset_list = sc7180_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(sc7180_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v3_usb3phy_regs_layout,
|
|
|
|
.has_pwrdn_delay = true,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sdm845_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = qmp_v3_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(qmp_v3_usb3_serdes_tbl),
|
|
.tx_tbl = qmp_v3_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(qmp_v3_usb3_tx_tbl),
|
|
.rx_tbl = qmp_v3_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(qmp_v3_usb3_rx_tbl),
|
|
.pcs_tbl = qmp_v3_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(qmp_v3_usb3_pcs_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v3_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v3_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v3_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v3_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v3_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v3_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v3_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v3_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v3_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v3_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v3_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v3_dp_aux_init,
|
|
.configure_dp_tx = qmp_v3_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v3_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v3_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v3_usb3phy_regs_layout,
|
|
|
|
.has_pwrdn_delay = true,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sc8180x_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = sm8150_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(sm8150_usb3_serdes_tbl),
|
|
.tx_tbl = sm8150_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(sm8150_usb3_tx_tbl),
|
|
.rx_tbl = sm8150_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sm8150_usb3_rx_tbl),
|
|
.pcs_tbl = sm8150_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sm8150_usb3_pcs_tbl),
|
|
.pcs_usb_tbl = sm8150_usb3_pcs_usb_tbl,
|
|
.pcs_usb_tbl_num = ARRAY_SIZE(sm8150_usb3_pcs_usb_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v4_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v4_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v4_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v4_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v4_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v4_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v4_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v3_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v3_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v3_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v3_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v45_usb3phy_regs_layout,
|
|
.pcs_usb_offset = 0x300,
|
|
|
|
.has_pwrdn_delay = true,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sc8280xp_usb43dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v5,
|
|
|
|
.serdes_tbl = sc8280xp_usb43dp_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(sc8280xp_usb43dp_serdes_tbl),
|
|
.tx_tbl = sc8280xp_usb43dp_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(sc8280xp_usb43dp_tx_tbl),
|
|
.rx_tbl = sc8280xp_usb43dp_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sc8280xp_usb43dp_rx_tbl),
|
|
.pcs_tbl = sc8280xp_usb43dp_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sc8280xp_usb43dp_pcs_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v5_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v5_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v5_5nm_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v5_5nm_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v4_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v4_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v4_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v4_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v5_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v5_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v5_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v5_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v5_5nm_usb3phy_regs_layout,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg x1e80100_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v5,
|
|
|
|
.serdes_tbl = x1e80100_usb43dp_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(x1e80100_usb43dp_serdes_tbl),
|
|
.tx_tbl = x1e80100_usb43dp_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(x1e80100_usb43dp_tx_tbl),
|
|
.rx_tbl = x1e80100_usb43dp_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(x1e80100_usb43dp_rx_tbl),
|
|
.pcs_tbl = x1e80100_usb43dp_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(x1e80100_usb43dp_pcs_tbl),
|
|
.pcs_usb_tbl = x1e80100_usb43dp_pcs_usb_tbl,
|
|
.pcs_usb_tbl_num = ARRAY_SIZE(x1e80100_usb43dp_pcs_usb_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v6_n4_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v6_n4_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v6_n4_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v6_n4_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v6_n4_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v6_n4_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v6_n4_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v6_n4_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v6_n4_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v6_n4_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v6_n4_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v6_n4_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v6_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v6_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v5_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v5_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v6_n4_usb3phy_regs_layout,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sm6350_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = qmp_v3_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(qmp_v3_usb3_serdes_tbl),
|
|
.tx_tbl = qmp_v3_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(qmp_v3_usb3_tx_tbl),
|
|
.rx_tbl = sm6350_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sm6350_usb3_rx_tbl),
|
|
.pcs_tbl = sm6350_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sm6350_usb3_pcs_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v3_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v3_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v3_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v3_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v3_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v3_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v3_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v3_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v3_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v3_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v3_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v3_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v3_dp_aux_init,
|
|
.configure_dp_tx = qmp_v3_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v3_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v3_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v3_usb3phy_regs_layout,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sm8250_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = sm8150_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(sm8150_usb3_serdes_tbl),
|
|
.tx_tbl = sm8250_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(sm8250_usb3_tx_tbl),
|
|
.rx_tbl = sm8250_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sm8250_usb3_rx_tbl),
|
|
.pcs_tbl = sm8250_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sm8250_usb3_pcs_tbl),
|
|
.pcs_usb_tbl = sm8250_usb3_pcs_usb_tbl,
|
|
.pcs_usb_tbl_num = ARRAY_SIZE(sm8250_usb3_pcs_usb_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v4_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v4_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v4_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v4_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v4_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v4_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v4_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v3_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v3_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v3_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v3_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v45_usb3phy_regs_layout,
|
|
.pcs_usb_offset = 0x300,
|
|
|
|
.has_pwrdn_delay = true,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sm8350_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = sm8150_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(sm8150_usb3_serdes_tbl),
|
|
.tx_tbl = sm8350_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(sm8350_usb3_tx_tbl),
|
|
.rx_tbl = sm8350_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sm8350_usb3_rx_tbl),
|
|
.pcs_tbl = sm8350_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sm8350_usb3_pcs_tbl),
|
|
.pcs_usb_tbl = sm8350_usb3_pcs_usb_tbl,
|
|
.pcs_usb_tbl_num = ARRAY_SIZE(sm8350_usb3_pcs_usb_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v4_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v5_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v5_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v4_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v4_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v4_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v4_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v4_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v4_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v4_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v3_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v4_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
.regs = qmp_v45_usb3phy_regs_layout,
|
|
|
|
.has_pwrdn_delay = true,
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sm8550_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = sm8550_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(sm8550_usb3_serdes_tbl),
|
|
.tx_tbl = sm8550_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(sm8550_usb3_tx_tbl),
|
|
.rx_tbl = sm8550_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sm8550_usb3_rx_tbl),
|
|
.pcs_tbl = sm8550_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sm8550_usb3_pcs_tbl),
|
|
.pcs_usb_tbl = sm8550_usb3_pcs_usb_tbl,
|
|
.pcs_usb_tbl_num = ARRAY_SIZE(sm8550_usb3_pcs_usb_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v6_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v6_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v6_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v6_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v6_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v6_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v6_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v5_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v6_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v5_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v5_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.regs = qmp_v6_usb3phy_regs_layout,
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
};
|
|
|
|
static const struct qmp_phy_cfg sm8650_usb3dpphy_cfg = {
|
|
.offsets = &qmp_combo_offsets_v3,
|
|
|
|
.serdes_tbl = sm8550_usb3_serdes_tbl,
|
|
.serdes_tbl_num = ARRAY_SIZE(sm8550_usb3_serdes_tbl),
|
|
.tx_tbl = sm8550_usb3_tx_tbl,
|
|
.tx_tbl_num = ARRAY_SIZE(sm8550_usb3_tx_tbl),
|
|
.rx_tbl = sm8550_usb3_rx_tbl,
|
|
.rx_tbl_num = ARRAY_SIZE(sm8550_usb3_rx_tbl),
|
|
.pcs_tbl = sm8550_usb3_pcs_tbl,
|
|
.pcs_tbl_num = ARRAY_SIZE(sm8550_usb3_pcs_tbl),
|
|
.pcs_usb_tbl = sm8550_usb3_pcs_usb_tbl,
|
|
.pcs_usb_tbl_num = ARRAY_SIZE(sm8550_usb3_pcs_usb_tbl),
|
|
|
|
.dp_serdes_tbl = qmp_v6_dp_serdes_tbl,
|
|
.dp_serdes_tbl_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl),
|
|
.dp_tx_tbl = qmp_v6_dp_tx_tbl,
|
|
.dp_tx_tbl_num = ARRAY_SIZE(qmp_v6_dp_tx_tbl),
|
|
|
|
.serdes_tbl_rbr = qmp_v6_dp_serdes_tbl_rbr,
|
|
.serdes_tbl_rbr_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_rbr),
|
|
.serdes_tbl_hbr = qmp_v6_dp_serdes_tbl_hbr,
|
|
.serdes_tbl_hbr_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_hbr),
|
|
.serdes_tbl_hbr2 = qmp_v6_dp_serdes_tbl_hbr2,
|
|
.serdes_tbl_hbr2_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_hbr2),
|
|
.serdes_tbl_hbr3 = qmp_v6_dp_serdes_tbl_hbr3,
|
|
.serdes_tbl_hbr3_num = ARRAY_SIZE(qmp_v6_dp_serdes_tbl_hbr3),
|
|
|
|
.swing_hbr_rbr = &qmp_dp_v6_voltage_swing_hbr_rbr,
|
|
.pre_emphasis_hbr_rbr = &qmp_dp_v6_pre_emphasis_hbr_rbr,
|
|
.swing_hbr3_hbr2 = &qmp_dp_v5_voltage_swing_hbr3_hbr2,
|
|
.pre_emphasis_hbr3_hbr2 = &qmp_dp_v5_pre_emphasis_hbr3_hbr2,
|
|
|
|
.dp_aux_init = qmp_v4_dp_aux_init,
|
|
.configure_dp_tx = qmp_v4_configure_dp_tx,
|
|
.configure_dp_phy = qmp_v4_configure_dp_phy,
|
|
.calibrate_dp_phy = qmp_v4_calibrate_dp_phy,
|
|
|
|
.regs = qmp_v6_usb3phy_regs_layout,
|
|
.reset_list = msm8996_usb3phy_reset_l,
|
|
.num_resets = ARRAY_SIZE(msm8996_usb3phy_reset_l),
|
|
.vreg_list = qmp_phy_vreg_l,
|
|
.num_vregs = ARRAY_SIZE(qmp_phy_vreg_l),
|
|
};
|
|
|
|
static int qmp_combo_dp_serdes_init(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
void __iomem *serdes = qmp->dp_serdes;
|
|
const struct phy_configure_opts_dp *dp_opts = &qmp->dp_opts;
|
|
|
|
qmp_configure(serdes, cfg->dp_serdes_tbl, cfg->dp_serdes_tbl_num);
|
|
|
|
switch (dp_opts->link_rate) {
|
|
case 1620:
|
|
qmp_configure(serdes, cfg->serdes_tbl_rbr,
|
|
cfg->serdes_tbl_rbr_num);
|
|
break;
|
|
case 2700:
|
|
qmp_configure(serdes, cfg->serdes_tbl_hbr,
|
|
cfg->serdes_tbl_hbr_num);
|
|
break;
|
|
case 5400:
|
|
qmp_configure(serdes, cfg->serdes_tbl_hbr2,
|
|
cfg->serdes_tbl_hbr2_num);
|
|
break;
|
|
case 8100:
|
|
qmp_configure(serdes, cfg->serdes_tbl_hbr3,
|
|
cfg->serdes_tbl_hbr3_num);
|
|
break;
|
|
default:
|
|
/* Other link rates aren't supported */
|
|
return -EINVAL;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void qmp_v3_dp_aux_init(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
writel(DP_PHY_PD_CTL_PWRDN | DP_PHY_PD_CTL_AUX_PWRDN |
|
|
DP_PHY_PD_CTL_PLL_PWRDN | DP_PHY_PD_CTL_DP_CLAMP_EN,
|
|
qmp->dp_dp_phy + QSERDES_DP_PHY_PD_CTL);
|
|
|
|
/* Turn on BIAS current for PHY/PLL */
|
|
writel(QSERDES_V3_COM_BIAS_EN | QSERDES_V3_COM_BIAS_EN_MUX |
|
|
QSERDES_V3_COM_CLKBUF_L_EN | QSERDES_V3_COM_EN_SYSCLK_TX_SEL,
|
|
qmp->dp_serdes + cfg->regs[QPHY_COM_BIAS_EN_CLKBUFLR_EN]);
|
|
|
|
writel(DP_PHY_PD_CTL_PSR_PWRDN, qmp->dp_dp_phy + QSERDES_DP_PHY_PD_CTL);
|
|
|
|
writel(DP_PHY_PD_CTL_PWRDN | DP_PHY_PD_CTL_AUX_PWRDN |
|
|
DP_PHY_PD_CTL_LANE_0_1_PWRDN |
|
|
DP_PHY_PD_CTL_LANE_2_3_PWRDN | DP_PHY_PD_CTL_PLL_PWRDN |
|
|
DP_PHY_PD_CTL_DP_CLAMP_EN,
|
|
qmp->dp_dp_phy + QSERDES_DP_PHY_PD_CTL);
|
|
|
|
writel(QSERDES_V3_COM_BIAS_EN |
|
|
QSERDES_V3_COM_BIAS_EN_MUX | QSERDES_V3_COM_CLKBUF_R_EN |
|
|
QSERDES_V3_COM_CLKBUF_L_EN | QSERDES_V3_COM_EN_SYSCLK_TX_SEL |
|
|
QSERDES_V3_COM_CLKBUF_RX_DRIVE_L,
|
|
qmp->dp_serdes + cfg->regs[QPHY_COM_BIAS_EN_CLKBUFLR_EN]);
|
|
|
|
writel(0x00, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG0);
|
|
writel(0x13, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG1);
|
|
writel(0x24, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG2);
|
|
writel(0x00, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG3);
|
|
writel(0x0a, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG4);
|
|
writel(0x26, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG5);
|
|
writel(0x0a, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG6);
|
|
writel(0x03, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG7);
|
|
writel(0xbb, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG8);
|
|
writel(0x03, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG9);
|
|
qmp->dp_aux_cfg = 0;
|
|
|
|
writel(PHY_AUX_STOP_ERR_MASK | PHY_AUX_DEC_ERR_MASK |
|
|
PHY_AUX_SYNC_ERR_MASK | PHY_AUX_ALIGN_ERR_MASK |
|
|
PHY_AUX_REQ_ERR_MASK,
|
|
qmp->dp_dp_phy + QSERDES_V3_DP_PHY_AUX_INTERRUPT_MASK);
|
|
}
|
|
|
|
static int qmp_combo_configure_dp_swing(struct qmp_combo *qmp)
|
|
{
|
|
const struct phy_configure_opts_dp *dp_opts = &qmp->dp_opts;
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
unsigned int v_level = 0, p_level = 0;
|
|
u8 voltage_swing_cfg, pre_emphasis_cfg;
|
|
int i;
|
|
|
|
for (i = 0; i < dp_opts->lanes; i++) {
|
|
v_level = max(v_level, dp_opts->voltage[i]);
|
|
p_level = max(p_level, dp_opts->pre[i]);
|
|
}
|
|
|
|
if (dp_opts->link_rate <= 2700) {
|
|
voltage_swing_cfg = (*cfg->swing_hbr_rbr)[v_level][p_level];
|
|
pre_emphasis_cfg = (*cfg->pre_emphasis_hbr_rbr)[v_level][p_level];
|
|
} else {
|
|
voltage_swing_cfg = (*cfg->swing_hbr3_hbr2)[v_level][p_level];
|
|
pre_emphasis_cfg = (*cfg->pre_emphasis_hbr3_hbr2)[v_level][p_level];
|
|
}
|
|
|
|
/* TODO: Move check to config check */
|
|
if (voltage_swing_cfg == 0xFF && pre_emphasis_cfg == 0xFF)
|
|
return -EINVAL;
|
|
|
|
/* Enable MUX to use Cursor values from these registers */
|
|
voltage_swing_cfg |= DP_PHY_TXn_TX_DRV_LVL_MUX_EN;
|
|
pre_emphasis_cfg |= DP_PHY_TXn_TX_EMP_POST1_LVL_MUX_EN;
|
|
|
|
writel(voltage_swing_cfg, qmp->dp_tx + cfg->regs[QPHY_TX_TX_DRV_LVL]);
|
|
writel(pre_emphasis_cfg, qmp->dp_tx + cfg->regs[QPHY_TX_TX_EMP_POST1_LVL]);
|
|
writel(voltage_swing_cfg, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_DRV_LVL]);
|
|
writel(pre_emphasis_cfg, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_EMP_POST1_LVL]);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void qmp_v3_configure_dp_tx(struct qmp_combo *qmp)
|
|
{
|
|
const struct phy_configure_opts_dp *dp_opts = &qmp->dp_opts;
|
|
u32 bias_en, drvr_en;
|
|
|
|
if (qmp_combo_configure_dp_swing(qmp) < 0)
|
|
return;
|
|
|
|
if (dp_opts->lanes == 1) {
|
|
bias_en = 0x3e;
|
|
drvr_en = 0x13;
|
|
} else {
|
|
bias_en = 0x3f;
|
|
drvr_en = 0x10;
|
|
}
|
|
|
|
writel(drvr_en, qmp->dp_tx + QSERDES_V3_TX_HIGHZ_DRVR_EN);
|
|
writel(bias_en, qmp->dp_tx + QSERDES_V3_TX_TRANSCEIVER_BIAS_EN);
|
|
writel(drvr_en, qmp->dp_tx2 + QSERDES_V3_TX_HIGHZ_DRVR_EN);
|
|
writel(bias_en, qmp->dp_tx2 + QSERDES_V3_TX_TRANSCEIVER_BIAS_EN);
|
|
}
|
|
|
|
static bool qmp_combo_configure_dp_mode(struct qmp_combo *qmp)
|
|
{
|
|
bool reverse = (qmp->orientation == TYPEC_ORIENTATION_REVERSE);
|
|
const struct phy_configure_opts_dp *dp_opts = &qmp->dp_opts;
|
|
u32 val;
|
|
|
|
val = DP_PHY_PD_CTL_PWRDN | DP_PHY_PD_CTL_AUX_PWRDN |
|
|
DP_PHY_PD_CTL_PLL_PWRDN | DP_PHY_PD_CTL_DP_CLAMP_EN;
|
|
|
|
if (dp_opts->lanes == 4 || reverse)
|
|
val |= DP_PHY_PD_CTL_LANE_0_1_PWRDN;
|
|
if (dp_opts->lanes == 4 || !reverse)
|
|
val |= DP_PHY_PD_CTL_LANE_2_3_PWRDN;
|
|
|
|
writel(val, qmp->dp_dp_phy + QSERDES_DP_PHY_PD_CTL);
|
|
|
|
if (reverse)
|
|
writel(0x4c, qmp->dp_dp_phy + QSERDES_DP_PHY_MODE);
|
|
else
|
|
writel(0x5c, qmp->dp_dp_phy + QSERDES_DP_PHY_MODE);
|
|
|
|
return reverse;
|
|
}
|
|
|
|
static int qmp_combo_configure_dp_clocks(struct qmp_combo *qmp)
|
|
{
|
|
const struct phy_configure_opts_dp *dp_opts = &qmp->dp_opts;
|
|
u32 phy_vco_div;
|
|
unsigned long pixel_freq;
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
switch (dp_opts->link_rate) {
|
|
case 1620:
|
|
phy_vco_div = 0x1;
|
|
pixel_freq = 1620000000UL / 2;
|
|
break;
|
|
case 2700:
|
|
phy_vco_div = 0x1;
|
|
pixel_freq = 2700000000UL / 2;
|
|
break;
|
|
case 5400:
|
|
phy_vco_div = 0x2;
|
|
pixel_freq = 5400000000UL / 4;
|
|
break;
|
|
case 8100:
|
|
phy_vco_div = 0x0;
|
|
pixel_freq = 8100000000UL / 6;
|
|
break;
|
|
default:
|
|
/* Other link rates aren't supported */
|
|
return -EINVAL;
|
|
}
|
|
writel(phy_vco_div, qmp->dp_dp_phy + cfg->regs[QPHY_DP_PHY_VCO_DIV]);
|
|
|
|
clk_set_rate(qmp->dp_link_hw.clk, dp_opts->link_rate * 100000);
|
|
clk_set_rate(qmp->dp_pixel_hw.clk, pixel_freq);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_v3_configure_dp_phy(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
u32 status;
|
|
int ret;
|
|
|
|
qmp_combo_configure_dp_mode(qmp);
|
|
|
|
writel(0x05, qmp->dp_dp_phy + QSERDES_V3_DP_PHY_TX0_TX1_LANE_CTL);
|
|
writel(0x05, qmp->dp_dp_phy + QSERDES_V3_DP_PHY_TX2_TX3_LANE_CTL);
|
|
|
|
ret = qmp_combo_configure_dp_clocks(qmp);
|
|
if (ret)
|
|
return ret;
|
|
|
|
writel(0x04, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG2);
|
|
writel(0x01, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
writel(0x05, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
writel(0x01, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
writel(0x09, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
|
|
writel(0x20, qmp->dp_serdes + cfg->regs[QPHY_COM_RESETSM_CNTRL]);
|
|
|
|
if (readl_poll_timeout(qmp->dp_serdes + cfg->regs[QPHY_COM_C_READY_STATUS],
|
|
status,
|
|
((status & BIT(0)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
writel(0x19, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
|
|
if (readl_poll_timeout(qmp->dp_dp_phy + cfg->regs[QPHY_DP_PHY_STATUS],
|
|
status,
|
|
((status & BIT(1)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
writel(0x18, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
udelay(2000);
|
|
writel(0x19, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
|
|
return readl_poll_timeout(qmp->dp_dp_phy + cfg->regs[QPHY_DP_PHY_STATUS],
|
|
status,
|
|
((status & BIT(1)) > 0),
|
|
500,
|
|
10000);
|
|
}
|
|
|
|
/*
|
|
* We need to calibrate the aux setting here as many times
|
|
* as the caller tries
|
|
*/
|
|
static int qmp_v3_calibrate_dp_phy(struct qmp_combo *qmp)
|
|
{
|
|
static const u8 cfg1_settings[] = { 0x13, 0x23, 0x1d };
|
|
u8 val;
|
|
|
|
qmp->dp_aux_cfg++;
|
|
qmp->dp_aux_cfg %= ARRAY_SIZE(cfg1_settings);
|
|
val = cfg1_settings[qmp->dp_aux_cfg];
|
|
|
|
writel(val, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG1);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void qmp_v4_dp_aux_init(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
writel(DP_PHY_PD_CTL_PWRDN | DP_PHY_PD_CTL_PSR_PWRDN | DP_PHY_PD_CTL_AUX_PWRDN |
|
|
DP_PHY_PD_CTL_PLL_PWRDN | DP_PHY_PD_CTL_DP_CLAMP_EN,
|
|
qmp->dp_dp_phy + QSERDES_DP_PHY_PD_CTL);
|
|
|
|
/* Turn on BIAS current for PHY/PLL */
|
|
writel(0x17, qmp->dp_serdes + cfg->regs[QPHY_COM_BIAS_EN_CLKBUFLR_EN]);
|
|
|
|
writel(0x00, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG0);
|
|
writel(0x13, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG1);
|
|
writel(0xa4, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG2);
|
|
writel(0x00, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG3);
|
|
writel(0x0a, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG4);
|
|
writel(0x26, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG5);
|
|
writel(0x0a, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG6);
|
|
writel(0x03, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG7);
|
|
writel(0xb7, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG8);
|
|
writel(0x03, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG9);
|
|
qmp->dp_aux_cfg = 0;
|
|
|
|
writel(PHY_AUX_STOP_ERR_MASK | PHY_AUX_DEC_ERR_MASK |
|
|
PHY_AUX_SYNC_ERR_MASK | PHY_AUX_ALIGN_ERR_MASK |
|
|
PHY_AUX_REQ_ERR_MASK,
|
|
qmp->dp_dp_phy + QSERDES_V4_DP_PHY_AUX_INTERRUPT_MASK);
|
|
}
|
|
|
|
static void qmp_v4_configure_dp_tx(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
/* Program default values before writing proper values */
|
|
writel(0x27, qmp->dp_tx + cfg->regs[QPHY_TX_TX_DRV_LVL]);
|
|
writel(0x27, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_DRV_LVL]);
|
|
|
|
writel(0x20, qmp->dp_tx + cfg->regs[QPHY_TX_TX_EMP_POST1_LVL]);
|
|
writel(0x20, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_EMP_POST1_LVL]);
|
|
|
|
qmp_combo_configure_dp_swing(qmp);
|
|
}
|
|
|
|
static int qmp_v456_configure_dp_phy(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
u32 status;
|
|
int ret;
|
|
|
|
writel(0x0f, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG_1);
|
|
|
|
qmp_combo_configure_dp_mode(qmp);
|
|
|
|
writel(0x13, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG1);
|
|
writel(0xa4, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG2);
|
|
|
|
writel(0x05, qmp->dp_dp_phy + QSERDES_V4_DP_PHY_TX0_TX1_LANE_CTL);
|
|
writel(0x05, qmp->dp_dp_phy + QSERDES_V4_DP_PHY_TX2_TX3_LANE_CTL);
|
|
|
|
ret = qmp_combo_configure_dp_clocks(qmp);
|
|
if (ret)
|
|
return ret;
|
|
|
|
writel(0x01, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
writel(0x05, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
writel(0x01, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
writel(0x09, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
|
|
writel(0x20, qmp->dp_serdes + cfg->regs[QPHY_COM_RESETSM_CNTRL]);
|
|
|
|
if (readl_poll_timeout(qmp->dp_serdes + cfg->regs[QPHY_COM_C_READY_STATUS],
|
|
status,
|
|
((status & BIT(0)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
if (readl_poll_timeout(qmp->dp_serdes + cfg->regs[QPHY_COM_CMN_STATUS],
|
|
status,
|
|
((status & BIT(0)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
if (readl_poll_timeout(qmp->dp_serdes + cfg->regs[QPHY_COM_CMN_STATUS],
|
|
status,
|
|
((status & BIT(1)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
writel(0x19, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
|
|
if (readl_poll_timeout(qmp->dp_dp_phy + cfg->regs[QPHY_DP_PHY_STATUS],
|
|
status,
|
|
((status & BIT(0)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
if (readl_poll_timeout(qmp->dp_dp_phy + cfg->regs[QPHY_DP_PHY_STATUS],
|
|
status,
|
|
((status & BIT(1)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_v4_configure_dp_phy(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
bool reverse = (qmp->orientation == TYPEC_ORIENTATION_REVERSE);
|
|
const struct phy_configure_opts_dp *dp_opts = &qmp->dp_opts;
|
|
u32 bias0_en, drvr0_en, bias1_en, drvr1_en;
|
|
u32 status;
|
|
int ret;
|
|
|
|
ret = qmp_v456_configure_dp_phy(qmp);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
/*
|
|
* At least for 7nm DP PHY this has to be done after enabling link
|
|
* clock.
|
|
*/
|
|
|
|
if (dp_opts->lanes == 1) {
|
|
bias0_en = reverse ? 0x3e : 0x15;
|
|
bias1_en = reverse ? 0x15 : 0x3e;
|
|
drvr0_en = reverse ? 0x13 : 0x10;
|
|
drvr1_en = reverse ? 0x10 : 0x13;
|
|
} else if (dp_opts->lanes == 2) {
|
|
bias0_en = reverse ? 0x3f : 0x15;
|
|
bias1_en = reverse ? 0x15 : 0x3f;
|
|
drvr0_en = 0x10;
|
|
drvr1_en = 0x10;
|
|
} else {
|
|
bias0_en = 0x3f;
|
|
bias1_en = 0x3f;
|
|
drvr0_en = 0x10;
|
|
drvr1_en = 0x10;
|
|
}
|
|
|
|
writel(drvr0_en, qmp->dp_tx + cfg->regs[QPHY_TX_HIGHZ_DRVR_EN]);
|
|
writel(bias0_en, qmp->dp_tx + cfg->regs[QPHY_TX_TRANSCEIVER_BIAS_EN]);
|
|
writel(drvr1_en, qmp->dp_tx2 + cfg->regs[QPHY_TX_HIGHZ_DRVR_EN]);
|
|
writel(bias1_en, qmp->dp_tx2 + cfg->regs[QPHY_TX_TRANSCEIVER_BIAS_EN]);
|
|
|
|
writel(0x18, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
udelay(2000);
|
|
writel(0x19, qmp->dp_dp_phy + QSERDES_DP_PHY_CFG);
|
|
|
|
if (readl_poll_timeout(qmp->dp_dp_phy + cfg->regs[QPHY_DP_PHY_STATUS],
|
|
status,
|
|
((status & BIT(1)) > 0),
|
|
500,
|
|
10000))
|
|
return -ETIMEDOUT;
|
|
|
|
writel(0x0a, qmp->dp_tx + cfg->regs[QPHY_TX_TX_POL_INV]);
|
|
writel(0x0a, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_POL_INV]);
|
|
|
|
writel(0x27, qmp->dp_tx + cfg->regs[QPHY_TX_TX_DRV_LVL]);
|
|
writel(0x27, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_DRV_LVL]);
|
|
|
|
writel(0x20, qmp->dp_tx + cfg->regs[QPHY_TX_TX_EMP_POST1_LVL]);
|
|
writel(0x20, qmp->dp_tx2 + cfg->regs[QPHY_TX_TX_EMP_POST1_LVL]);
|
|
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* We need to calibrate the aux setting here as many times
|
|
* as the caller tries
|
|
*/
|
|
static int qmp_v4_calibrate_dp_phy(struct qmp_combo *qmp)
|
|
{
|
|
static const u8 cfg1_settings[] = { 0x20, 0x13, 0x23, 0x1d };
|
|
u8 val;
|
|
|
|
qmp->dp_aux_cfg++;
|
|
qmp->dp_aux_cfg %= ARRAY_SIZE(cfg1_settings);
|
|
val = cfg1_settings[qmp->dp_aux_cfg];
|
|
|
|
writel(val, qmp->dp_dp_phy + QSERDES_DP_PHY_AUX_CFG1);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_dp_configure(struct phy *phy, union phy_configure_opts *opts)
|
|
{
|
|
const struct phy_configure_opts_dp *dp_opts = &opts->dp;
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
|
|
memcpy(&qmp->dp_opts, dp_opts, sizeof(*dp_opts));
|
|
if (qmp->dp_opts.set_voltages) {
|
|
cfg->configure_dp_tx(qmp);
|
|
qmp->dp_opts.set_voltages = 0;
|
|
}
|
|
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_dp_calibrate(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
int ret = 0;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
|
|
if (cfg->calibrate_dp_phy)
|
|
ret = cfg->calibrate_dp_phy(qmp);
|
|
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
|
|
return ret;
|
|
}
|
|
|
|
static int qmp_combo_com_init(struct qmp_combo *qmp, bool force)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
void __iomem *com = qmp->com;
|
|
int ret;
|
|
u32 val;
|
|
|
|
if (!force && qmp->init_count++)
|
|
return 0;
|
|
|
|
ret = regulator_bulk_enable(cfg->num_vregs, qmp->vregs);
|
|
if (ret) {
|
|
dev_err(qmp->dev, "failed to enable regulators, err=%d\n", ret);
|
|
goto err_decrement_count;
|
|
}
|
|
|
|
ret = reset_control_bulk_assert(cfg->num_resets, qmp->resets);
|
|
if (ret) {
|
|
dev_err(qmp->dev, "reset assert failed\n");
|
|
goto err_disable_regulators;
|
|
}
|
|
|
|
ret = reset_control_bulk_deassert(cfg->num_resets, qmp->resets);
|
|
if (ret) {
|
|
dev_err(qmp->dev, "reset deassert failed\n");
|
|
goto err_disable_regulators;
|
|
}
|
|
|
|
ret = clk_bulk_prepare_enable(qmp->num_clks, qmp->clks);
|
|
if (ret)
|
|
goto err_assert_reset;
|
|
|
|
qphy_setbits(com, QPHY_V3_DP_COM_POWER_DOWN_CTRL, SW_PWRDN);
|
|
|
|
/* override hardware control for reset of qmp phy */
|
|
qphy_setbits(com, QPHY_V3_DP_COM_RESET_OVRD_CTRL,
|
|
SW_DPPHY_RESET_MUX | SW_DPPHY_RESET |
|
|
SW_USB3PHY_RESET_MUX | SW_USB3PHY_RESET);
|
|
|
|
/* Use software based port select and switch on typec orientation */
|
|
val = SW_PORTSELECT_MUX;
|
|
if (qmp->orientation == TYPEC_ORIENTATION_REVERSE)
|
|
val |= SW_PORTSELECT_VAL;
|
|
writel(val, com + QPHY_V3_DP_COM_TYPEC_CTRL);
|
|
writel(USB3_MODE | DP_MODE, com + QPHY_V3_DP_COM_PHY_MODE_CTRL);
|
|
|
|
/* bring both QMP USB and QMP DP PHYs PCS block out of reset */
|
|
qphy_clrbits(com, QPHY_V3_DP_COM_RESET_OVRD_CTRL,
|
|
SW_DPPHY_RESET_MUX | SW_DPPHY_RESET |
|
|
SW_USB3PHY_RESET_MUX | SW_USB3PHY_RESET);
|
|
|
|
qphy_clrbits(com, QPHY_V3_DP_COM_SWI_CTRL, 0x03);
|
|
qphy_clrbits(com, QPHY_V3_DP_COM_SW_RESET, SW_RESET);
|
|
|
|
qphy_setbits(qmp->pcs, cfg->regs[QPHY_PCS_POWER_DOWN_CONTROL],
|
|
SW_PWRDN);
|
|
|
|
return 0;
|
|
|
|
err_assert_reset:
|
|
reset_control_bulk_assert(cfg->num_resets, qmp->resets);
|
|
err_disable_regulators:
|
|
regulator_bulk_disable(cfg->num_vregs, qmp->vregs);
|
|
err_decrement_count:
|
|
qmp->init_count--;
|
|
|
|
return ret;
|
|
}
|
|
|
|
static int qmp_combo_com_exit(struct qmp_combo *qmp, bool force)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
if (!force && --qmp->init_count)
|
|
return 0;
|
|
|
|
reset_control_bulk_assert(cfg->num_resets, qmp->resets);
|
|
|
|
clk_bulk_disable_unprepare(qmp->num_clks, qmp->clks);
|
|
|
|
regulator_bulk_disable(cfg->num_vregs, qmp->vregs);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_dp_init(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
int ret;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
|
|
ret = qmp_combo_com_init(qmp, false);
|
|
if (ret)
|
|
goto out_unlock;
|
|
|
|
cfg->dp_aux_init(qmp);
|
|
|
|
qmp->dp_init_count++;
|
|
|
|
out_unlock:
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
return ret;
|
|
}
|
|
|
|
static int qmp_combo_dp_exit(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
|
|
qmp_combo_com_exit(qmp, false);
|
|
|
|
qmp->dp_init_count--;
|
|
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_dp_power_on(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
void __iomem *tx = qmp->dp_tx;
|
|
void __iomem *tx2 = qmp->dp_tx2;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
|
|
qmp_combo_dp_serdes_init(qmp);
|
|
|
|
qmp_configure_lane(tx, cfg->dp_tx_tbl, cfg->dp_tx_tbl_num, 1);
|
|
qmp_configure_lane(tx2, cfg->dp_tx_tbl, cfg->dp_tx_tbl_num, 2);
|
|
|
|
/* Configure special DP tx tunings */
|
|
cfg->configure_dp_tx(qmp);
|
|
|
|
/* Configure link rate, swing, etc. */
|
|
cfg->configure_dp_phy(qmp);
|
|
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_dp_power_off(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
|
|
/* Assert DP PHY power down */
|
|
writel(DP_PHY_PD_CTL_PSR_PWRDN, qmp->dp_dp_phy + QSERDES_DP_PHY_PD_CTL);
|
|
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_usb_power_on(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
void __iomem *serdes = qmp->serdes;
|
|
void __iomem *tx = qmp->tx;
|
|
void __iomem *rx = qmp->rx;
|
|
void __iomem *tx2 = qmp->tx2;
|
|
void __iomem *rx2 = qmp->rx2;
|
|
void __iomem *pcs = qmp->pcs;
|
|
void __iomem *pcs_usb = qmp->pcs_usb;
|
|
void __iomem *status;
|
|
unsigned int val;
|
|
int ret;
|
|
|
|
qmp_configure(serdes, cfg->serdes_tbl, cfg->serdes_tbl_num);
|
|
|
|
ret = clk_prepare_enable(qmp->pipe_clk);
|
|
if (ret) {
|
|
dev_err(qmp->dev, "pipe_clk enable failed err=%d\n", ret);
|
|
return ret;
|
|
}
|
|
|
|
/* Tx, Rx, and PCS configurations */
|
|
qmp_configure_lane(tx, cfg->tx_tbl, cfg->tx_tbl_num, 1);
|
|
qmp_configure_lane(tx2, cfg->tx_tbl, cfg->tx_tbl_num, 2);
|
|
|
|
qmp_configure_lane(rx, cfg->rx_tbl, cfg->rx_tbl_num, 1);
|
|
qmp_configure_lane(rx2, cfg->rx_tbl, cfg->rx_tbl_num, 2);
|
|
|
|
qmp_configure(pcs, cfg->pcs_tbl, cfg->pcs_tbl_num);
|
|
|
|
if (pcs_usb)
|
|
qmp_configure(pcs_usb, cfg->pcs_usb_tbl, cfg->pcs_usb_tbl_num);
|
|
|
|
if (cfg->has_pwrdn_delay)
|
|
usleep_range(10, 20);
|
|
|
|
/* Pull PHY out of reset state */
|
|
qphy_clrbits(pcs, cfg->regs[QPHY_SW_RESET], SW_RESET);
|
|
|
|
/* start SerDes and Phy-Coding-Sublayer */
|
|
qphy_setbits(pcs, cfg->regs[QPHY_START_CTRL], SERDES_START | PCS_START);
|
|
|
|
status = pcs + cfg->regs[QPHY_PCS_STATUS];
|
|
ret = readl_poll_timeout(status, val, !(val & PHYSTATUS), 200,
|
|
PHY_INIT_COMPLETE_TIMEOUT);
|
|
if (ret) {
|
|
dev_err(qmp->dev, "phy initialization timed-out\n");
|
|
goto err_disable_pipe_clk;
|
|
}
|
|
|
|
return 0;
|
|
|
|
err_disable_pipe_clk:
|
|
clk_disable_unprepare(qmp->pipe_clk);
|
|
|
|
return ret;
|
|
}
|
|
|
|
static int qmp_combo_usb_power_off(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
clk_disable_unprepare(qmp->pipe_clk);
|
|
|
|
/* PHY reset */
|
|
qphy_setbits(qmp->pcs, cfg->regs[QPHY_SW_RESET], SW_RESET);
|
|
|
|
/* stop SerDes and Phy-Coding-Sublayer */
|
|
qphy_clrbits(qmp->pcs, cfg->regs[QPHY_START_CTRL],
|
|
SERDES_START | PCS_START);
|
|
|
|
/* Put PHY into POWER DOWN state: active low */
|
|
qphy_clrbits(qmp->pcs, cfg->regs[QPHY_PCS_POWER_DOWN_CONTROL],
|
|
SW_PWRDN);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_usb_init(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
int ret;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
ret = qmp_combo_com_init(qmp, false);
|
|
if (ret)
|
|
goto out_unlock;
|
|
|
|
ret = qmp_combo_usb_power_on(phy);
|
|
if (ret) {
|
|
qmp_combo_com_exit(qmp, false);
|
|
goto out_unlock;
|
|
}
|
|
|
|
qmp->usb_init_count++;
|
|
|
|
out_unlock:
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
return ret;
|
|
}
|
|
|
|
static int qmp_combo_usb_exit(struct phy *phy)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
int ret;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
ret = qmp_combo_usb_power_off(phy);
|
|
if (ret)
|
|
goto out_unlock;
|
|
|
|
ret = qmp_combo_com_exit(qmp, false);
|
|
if (ret)
|
|
goto out_unlock;
|
|
|
|
qmp->usb_init_count--;
|
|
|
|
out_unlock:
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
return ret;
|
|
}
|
|
|
|
static int qmp_combo_usb_set_mode(struct phy *phy, enum phy_mode mode, int submode)
|
|
{
|
|
struct qmp_combo *qmp = phy_get_drvdata(phy);
|
|
|
|
qmp->mode = mode;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct phy_ops qmp_combo_usb_phy_ops = {
|
|
.init = qmp_combo_usb_init,
|
|
.exit = qmp_combo_usb_exit,
|
|
.set_mode = qmp_combo_usb_set_mode,
|
|
.owner = THIS_MODULE,
|
|
};
|
|
|
|
static const struct phy_ops qmp_combo_dp_phy_ops = {
|
|
.init = qmp_combo_dp_init,
|
|
.configure = qmp_combo_dp_configure,
|
|
.power_on = qmp_combo_dp_power_on,
|
|
.calibrate = qmp_combo_dp_calibrate,
|
|
.power_off = qmp_combo_dp_power_off,
|
|
.exit = qmp_combo_dp_exit,
|
|
.owner = THIS_MODULE,
|
|
};
|
|
|
|
static void qmp_combo_enable_autonomous_mode(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
void __iomem *pcs_usb = qmp->pcs_usb ?: qmp->pcs;
|
|
void __iomem *pcs_misc = qmp->pcs_misc;
|
|
u32 intr_mask;
|
|
|
|
if (qmp->mode == PHY_MODE_USB_HOST_SS ||
|
|
qmp->mode == PHY_MODE_USB_DEVICE_SS)
|
|
intr_mask = ARCVR_DTCT_EN | ALFPS_DTCT_EN;
|
|
else
|
|
intr_mask = ARCVR_DTCT_EN | ARCVR_DTCT_EVENT_SEL;
|
|
|
|
/* Clear any pending interrupts status */
|
|
qphy_setbits(pcs_usb, cfg->regs[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR], IRQ_CLEAR);
|
|
/* Writing 1 followed by 0 clears the interrupt */
|
|
qphy_clrbits(pcs_usb, cfg->regs[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR], IRQ_CLEAR);
|
|
|
|
qphy_clrbits(pcs_usb, cfg->regs[QPHY_PCS_AUTONOMOUS_MODE_CTRL],
|
|
ARCVR_DTCT_EN | ALFPS_DTCT_EN | ARCVR_DTCT_EVENT_SEL);
|
|
|
|
/* Enable required PHY autonomous mode interrupts */
|
|
qphy_setbits(pcs_usb, cfg->regs[QPHY_PCS_AUTONOMOUS_MODE_CTRL], intr_mask);
|
|
|
|
/* Enable i/o clamp_n for autonomous mode */
|
|
if (pcs_misc)
|
|
qphy_clrbits(pcs_misc, QPHY_V3_PCS_MISC_CLAMP_ENABLE, CLAMP_EN);
|
|
}
|
|
|
|
static void qmp_combo_disable_autonomous_mode(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
void __iomem *pcs_usb = qmp->pcs_usb ?: qmp->pcs;
|
|
void __iomem *pcs_misc = qmp->pcs_misc;
|
|
|
|
/* Disable i/o clamp_n on resume for normal mode */
|
|
if (pcs_misc)
|
|
qphy_setbits(pcs_misc, QPHY_V3_PCS_MISC_CLAMP_ENABLE, CLAMP_EN);
|
|
|
|
qphy_clrbits(pcs_usb, cfg->regs[QPHY_PCS_AUTONOMOUS_MODE_CTRL],
|
|
ARCVR_DTCT_EN | ARCVR_DTCT_EVENT_SEL | ALFPS_DTCT_EN);
|
|
|
|
qphy_setbits(pcs_usb, cfg->regs[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR], IRQ_CLEAR);
|
|
/* Writing 1 followed by 0 clears the interrupt */
|
|
qphy_clrbits(pcs_usb, cfg->regs[QPHY_PCS_LFPS_RXTERM_IRQ_CLEAR], IRQ_CLEAR);
|
|
}
|
|
|
|
static int __maybe_unused qmp_combo_runtime_suspend(struct device *dev)
|
|
{
|
|
struct qmp_combo *qmp = dev_get_drvdata(dev);
|
|
|
|
dev_vdbg(dev, "Suspending QMP phy, mode:%d\n", qmp->mode);
|
|
|
|
if (!qmp->init_count) {
|
|
dev_vdbg(dev, "PHY not initialized, bailing out\n");
|
|
return 0;
|
|
}
|
|
|
|
qmp_combo_enable_autonomous_mode(qmp);
|
|
|
|
clk_disable_unprepare(qmp->pipe_clk);
|
|
clk_bulk_disable_unprepare(qmp->num_clks, qmp->clks);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int __maybe_unused qmp_combo_runtime_resume(struct device *dev)
|
|
{
|
|
struct qmp_combo *qmp = dev_get_drvdata(dev);
|
|
int ret = 0;
|
|
|
|
dev_vdbg(dev, "Resuming QMP phy, mode:%d\n", qmp->mode);
|
|
|
|
if (!qmp->init_count) {
|
|
dev_vdbg(dev, "PHY not initialized, bailing out\n");
|
|
return 0;
|
|
}
|
|
|
|
ret = clk_bulk_prepare_enable(qmp->num_clks, qmp->clks);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = clk_prepare_enable(qmp->pipe_clk);
|
|
if (ret) {
|
|
dev_err(dev, "pipe_clk enable failed, err=%d\n", ret);
|
|
clk_bulk_disable_unprepare(qmp->num_clks, qmp->clks);
|
|
return ret;
|
|
}
|
|
|
|
qmp_combo_disable_autonomous_mode(qmp);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct dev_pm_ops qmp_combo_pm_ops = {
|
|
SET_RUNTIME_PM_OPS(qmp_combo_runtime_suspend,
|
|
qmp_combo_runtime_resume, NULL)
|
|
};
|
|
|
|
static int qmp_combo_vreg_init(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
struct device *dev = qmp->dev;
|
|
int num = cfg->num_vregs;
|
|
int ret, i;
|
|
|
|
qmp->vregs = devm_kcalloc(dev, num, sizeof(*qmp->vregs), GFP_KERNEL);
|
|
if (!qmp->vregs)
|
|
return -ENOMEM;
|
|
|
|
for (i = 0; i < num; i++)
|
|
qmp->vregs[i].supply = cfg->vreg_list[i].name;
|
|
|
|
ret = devm_regulator_bulk_get(dev, num, qmp->vregs);
|
|
if (ret) {
|
|
dev_err(dev, "failed at devm_regulator_bulk_get\n");
|
|
return ret;
|
|
}
|
|
|
|
for (i = 0; i < num; i++) {
|
|
ret = regulator_set_load(qmp->vregs[i].consumer,
|
|
cfg->vreg_list[i].enable_load);
|
|
if (ret) {
|
|
dev_err(dev, "failed to set load at %s\n",
|
|
qmp->vregs[i].supply);
|
|
return ret;
|
|
}
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_reset_init(struct qmp_combo *qmp)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
struct device *dev = qmp->dev;
|
|
int i;
|
|
int ret;
|
|
|
|
qmp->resets = devm_kcalloc(dev, cfg->num_resets,
|
|
sizeof(*qmp->resets), GFP_KERNEL);
|
|
if (!qmp->resets)
|
|
return -ENOMEM;
|
|
|
|
for (i = 0; i < cfg->num_resets; i++)
|
|
qmp->resets[i].id = cfg->reset_list[i];
|
|
|
|
ret = devm_reset_control_bulk_get_exclusive(dev, cfg->num_resets, qmp->resets);
|
|
if (ret)
|
|
return dev_err_probe(dev, ret, "failed to get resets\n");
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_clk_init(struct qmp_combo *qmp)
|
|
{
|
|
struct device *dev = qmp->dev;
|
|
int num = ARRAY_SIZE(qmp_combo_phy_clk_l);
|
|
int i;
|
|
|
|
qmp->clks = devm_kcalloc(dev, num, sizeof(*qmp->clks), GFP_KERNEL);
|
|
if (!qmp->clks)
|
|
return -ENOMEM;
|
|
|
|
for (i = 0; i < num; i++)
|
|
qmp->clks[i].id = qmp_combo_phy_clk_l[i];
|
|
|
|
qmp->num_clks = num;
|
|
|
|
return devm_clk_bulk_get_optional(dev, num, qmp->clks);
|
|
}
|
|
|
|
static void phy_clk_release_provider(void *res)
|
|
{
|
|
of_clk_del_provider(res);
|
|
}
|
|
|
|
/*
|
|
* Register a fixed rate pipe clock.
|
|
*
|
|
* The <s>_pipe_clksrc generated by PHY goes to the GCC that gate
|
|
* controls it. The <s>_pipe_clk coming out of the GCC is requested
|
|
* by the PHY driver for its operations.
|
|
* We register the <s>_pipe_clksrc here. The gcc driver takes care
|
|
* of assigning this <s>_pipe_clksrc as parent to <s>_pipe_clk.
|
|
* Below picture shows this relationship.
|
|
*
|
|
* +---------------+
|
|
* | PHY block |<<---------------------------------------+
|
|
* | | |
|
|
* | +-------+ | +-----+ |
|
|
* I/P---^-->| PLL |---^--->pipe_clksrc--->| GCC |--->pipe_clk---+
|
|
* clk | +-------+ | +-----+
|
|
* +---------------+
|
|
*/
|
|
static int phy_pipe_clk_register(struct qmp_combo *qmp, struct device_node *np)
|
|
{
|
|
struct clk_fixed_rate *fixed = &qmp->pipe_clk_fixed;
|
|
struct clk_init_data init = { };
|
|
char name[64];
|
|
|
|
snprintf(name, sizeof(name), "%s::pipe_clk", dev_name(qmp->dev));
|
|
init.name = name;
|
|
init.ops = &clk_fixed_rate_ops;
|
|
|
|
/* controllers using QMP phys use 125MHz pipe clock interface */
|
|
fixed->fixed_rate = 125000000;
|
|
fixed->hw.init = &init;
|
|
|
|
return devm_clk_hw_register(qmp->dev, &fixed->hw);
|
|
}
|
|
|
|
/*
|
|
* Display Port PLL driver block diagram for branch clocks
|
|
*
|
|
* +------------------------------+
|
|
* | DP_VCO_CLK |
|
|
* | |
|
|
* | +-------------------+ |
|
|
* | | (DP PLL/VCO) | |
|
|
* | +---------+---------+ |
|
|
* | v |
|
|
* | +----------+-----------+ |
|
|
* | | hsclk_divsel_clk_src | |
|
|
* | +----------+-----------+ |
|
|
* +------------------------------+
|
|
* |
|
|
* +---------<---------v------------>----------+
|
|
* | |
|
|
* +--------v----------------+ |
|
|
* | dp_phy_pll_link_clk | |
|
|
* | link_clk | |
|
|
* +--------+----------------+ |
|
|
* | |
|
|
* | |
|
|
* v v
|
|
* Input to DISPCC block |
|
|
* for link clk, crypto clk |
|
|
* and interface clock |
|
|
* |
|
|
* |
|
|
* +--------<------------+-----------------+---<---+
|
|
* | | |
|
|
* +----v---------+ +--------v-----+ +--------v------+
|
|
* | vco_divided | | vco_divided | | vco_divided |
|
|
* | _clk_src | | _clk_src | | _clk_src |
|
|
* | | | | | |
|
|
* |divsel_six | | divsel_two | | divsel_four |
|
|
* +-------+------+ +-----+--------+ +--------+------+
|
|
* | | |
|
|
* v---->----------v-------------<------v
|
|
* |
|
|
* +----------+-----------------+
|
|
* | dp_phy_pll_vco_div_clk |
|
|
* +---------+------------------+
|
|
* |
|
|
* v
|
|
* Input to DISPCC block
|
|
* for DP pixel clock
|
|
*
|
|
*/
|
|
static int qmp_dp_pixel_clk_determine_rate(struct clk_hw *hw, struct clk_rate_request *req)
|
|
{
|
|
switch (req->rate) {
|
|
case 1620000000UL / 2:
|
|
case 2700000000UL / 2:
|
|
/* 5.4 and 8.1 GHz are same link rate as 2.7GHz, i.e. div 4 and div 6 */
|
|
return 0;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
}
|
|
|
|
static unsigned long qmp_dp_pixel_clk_recalc_rate(struct clk_hw *hw, unsigned long parent_rate)
|
|
{
|
|
const struct qmp_combo *qmp;
|
|
const struct phy_configure_opts_dp *dp_opts;
|
|
|
|
qmp = container_of(hw, struct qmp_combo, dp_pixel_hw);
|
|
dp_opts = &qmp->dp_opts;
|
|
|
|
switch (dp_opts->link_rate) {
|
|
case 1620:
|
|
return 1620000000UL / 2;
|
|
case 2700:
|
|
return 2700000000UL / 2;
|
|
case 5400:
|
|
return 5400000000UL / 4;
|
|
case 8100:
|
|
return 8100000000UL / 6;
|
|
default:
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
static const struct clk_ops qmp_dp_pixel_clk_ops = {
|
|
.determine_rate = qmp_dp_pixel_clk_determine_rate,
|
|
.recalc_rate = qmp_dp_pixel_clk_recalc_rate,
|
|
};
|
|
|
|
static int qmp_dp_link_clk_determine_rate(struct clk_hw *hw, struct clk_rate_request *req)
|
|
{
|
|
switch (req->rate) {
|
|
case 162000000:
|
|
case 270000000:
|
|
case 540000000:
|
|
case 810000000:
|
|
return 0;
|
|
default:
|
|
return -EINVAL;
|
|
}
|
|
}
|
|
|
|
static unsigned long qmp_dp_link_clk_recalc_rate(struct clk_hw *hw, unsigned long parent_rate)
|
|
{
|
|
const struct qmp_combo *qmp;
|
|
const struct phy_configure_opts_dp *dp_opts;
|
|
|
|
qmp = container_of(hw, struct qmp_combo, dp_link_hw);
|
|
dp_opts = &qmp->dp_opts;
|
|
|
|
switch (dp_opts->link_rate) {
|
|
case 1620:
|
|
case 2700:
|
|
case 5400:
|
|
case 8100:
|
|
return dp_opts->link_rate * 100000;
|
|
default:
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
static const struct clk_ops qmp_dp_link_clk_ops = {
|
|
.determine_rate = qmp_dp_link_clk_determine_rate,
|
|
.recalc_rate = qmp_dp_link_clk_recalc_rate,
|
|
};
|
|
|
|
static struct clk_hw *qmp_dp_clks_hw_get(struct of_phandle_args *clkspec, void *data)
|
|
{
|
|
struct qmp_combo *qmp = data;
|
|
unsigned int idx = clkspec->args[0];
|
|
|
|
if (idx >= 2) {
|
|
pr_err("%s: invalid index %u\n", __func__, idx);
|
|
return ERR_PTR(-EINVAL);
|
|
}
|
|
|
|
if (idx == 0)
|
|
return &qmp->dp_link_hw;
|
|
|
|
return &qmp->dp_pixel_hw;
|
|
}
|
|
|
|
static int phy_dp_clks_register(struct qmp_combo *qmp, struct device_node *np)
|
|
{
|
|
struct clk_init_data init = { };
|
|
char name[64];
|
|
int ret;
|
|
|
|
snprintf(name, sizeof(name), "%s::link_clk", dev_name(qmp->dev));
|
|
init.ops = &qmp_dp_link_clk_ops;
|
|
init.name = name;
|
|
qmp->dp_link_hw.init = &init;
|
|
ret = devm_clk_hw_register(qmp->dev, &qmp->dp_link_hw);
|
|
if (ret)
|
|
return ret;
|
|
|
|
snprintf(name, sizeof(name), "%s::vco_div_clk", dev_name(qmp->dev));
|
|
init.ops = &qmp_dp_pixel_clk_ops;
|
|
init.name = name;
|
|
qmp->dp_pixel_hw.init = &init;
|
|
ret = devm_clk_hw_register(qmp->dev, &qmp->dp_pixel_hw);
|
|
if (ret)
|
|
return ret;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static struct clk_hw *qmp_combo_clk_hw_get(struct of_phandle_args *clkspec, void *data)
|
|
{
|
|
struct qmp_combo *qmp = data;
|
|
|
|
switch (clkspec->args[0]) {
|
|
case QMP_USB43DP_USB3_PIPE_CLK:
|
|
return &qmp->pipe_clk_fixed.hw;
|
|
case QMP_USB43DP_DP_LINK_CLK:
|
|
return &qmp->dp_link_hw;
|
|
case QMP_USB43DP_DP_VCO_DIV_CLK:
|
|
return &qmp->dp_pixel_hw;
|
|
}
|
|
|
|
return ERR_PTR(-EINVAL);
|
|
}
|
|
|
|
static int qmp_combo_register_clocks(struct qmp_combo *qmp, struct device_node *usb_np,
|
|
struct device_node *dp_np)
|
|
{
|
|
int ret;
|
|
|
|
ret = phy_pipe_clk_register(qmp, usb_np);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = phy_dp_clks_register(qmp, dp_np);
|
|
if (ret)
|
|
return ret;
|
|
|
|
/*
|
|
* Register a single provider for bindings without child nodes.
|
|
*/
|
|
if (usb_np == qmp->dev->of_node)
|
|
return devm_of_clk_add_hw_provider(qmp->dev, qmp_combo_clk_hw_get, qmp);
|
|
|
|
/*
|
|
* Register multiple providers for legacy bindings with child nodes.
|
|
*/
|
|
ret = of_clk_add_hw_provider(usb_np, of_clk_hw_simple_get,
|
|
&qmp->pipe_clk_fixed.hw);
|
|
if (ret)
|
|
return ret;
|
|
|
|
/*
|
|
* Roll a devm action because the clock provider is the child node, but
|
|
* the child node is not actually a device.
|
|
*/
|
|
ret = devm_add_action_or_reset(qmp->dev, phy_clk_release_provider, usb_np);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = of_clk_add_hw_provider(dp_np, qmp_dp_clks_hw_get, qmp);
|
|
if (ret)
|
|
return ret;
|
|
|
|
return devm_add_action_or_reset(qmp->dev, phy_clk_release_provider, dp_np);
|
|
}
|
|
|
|
#if IS_ENABLED(CONFIG_TYPEC)
|
|
static int qmp_combo_typec_switch_set(struct typec_switch_dev *sw,
|
|
enum typec_orientation orientation)
|
|
{
|
|
struct qmp_combo *qmp = typec_switch_get_drvdata(sw);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
|
|
if (orientation == qmp->orientation || orientation == TYPEC_ORIENTATION_NONE)
|
|
return 0;
|
|
|
|
mutex_lock(&qmp->phy_mutex);
|
|
qmp->orientation = orientation;
|
|
|
|
if (qmp->init_count) {
|
|
if (qmp->usb_init_count)
|
|
qmp_combo_usb_power_off(qmp->usb_phy);
|
|
qmp_combo_com_exit(qmp, true);
|
|
|
|
qmp_combo_com_init(qmp, true);
|
|
if (qmp->usb_init_count)
|
|
qmp_combo_usb_power_on(qmp->usb_phy);
|
|
if (qmp->dp_init_count)
|
|
cfg->dp_aux_init(qmp);
|
|
}
|
|
mutex_unlock(&qmp->phy_mutex);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void qmp_combo_typec_unregister(void *data)
|
|
{
|
|
struct qmp_combo *qmp = data;
|
|
|
|
typec_switch_unregister(qmp->sw);
|
|
}
|
|
|
|
static int qmp_combo_typec_switch_register(struct qmp_combo *qmp)
|
|
{
|
|
struct typec_switch_desc sw_desc = {};
|
|
struct device *dev = qmp->dev;
|
|
|
|
sw_desc.drvdata = qmp;
|
|
sw_desc.fwnode = dev->fwnode;
|
|
sw_desc.set = qmp_combo_typec_switch_set;
|
|
qmp->sw = typec_switch_register(dev, &sw_desc);
|
|
if (IS_ERR(qmp->sw)) {
|
|
dev_err(dev, "Unable to register typec switch: %pe\n", qmp->sw);
|
|
return PTR_ERR(qmp->sw);
|
|
}
|
|
|
|
return devm_add_action_or_reset(dev, qmp_combo_typec_unregister, qmp);
|
|
}
|
|
#else
|
|
static int qmp_combo_typec_switch_register(struct qmp_combo *qmp)
|
|
{
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
static int qmp_combo_parse_dt_lecacy_dp(struct qmp_combo *qmp, struct device_node *np)
|
|
{
|
|
struct device *dev = qmp->dev;
|
|
|
|
/*
|
|
* Get memory resources from the DP child node:
|
|
* Resources are indexed as: tx -> 0; rx -> 1; pcs -> 2;
|
|
* tx2 -> 3; rx2 -> 4
|
|
*
|
|
* Note that only tx/tx2 and pcs (dp_phy) are used by the DP
|
|
* implementation.
|
|
*/
|
|
qmp->dp_tx = devm_of_iomap(dev, np, 0, NULL);
|
|
if (IS_ERR(qmp->dp_tx))
|
|
return PTR_ERR(qmp->dp_tx);
|
|
|
|
qmp->dp_dp_phy = devm_of_iomap(dev, np, 2, NULL);
|
|
if (IS_ERR(qmp->dp_dp_phy))
|
|
return PTR_ERR(qmp->dp_dp_phy);
|
|
|
|
qmp->dp_tx2 = devm_of_iomap(dev, np, 3, NULL);
|
|
if (IS_ERR(qmp->dp_tx2))
|
|
return PTR_ERR(qmp->dp_tx2);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_parse_dt_lecacy_usb(struct qmp_combo *qmp, struct device_node *np)
|
|
{
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
struct device *dev = qmp->dev;
|
|
|
|
/*
|
|
* Get memory resources from the USB child node:
|
|
* Resources are indexed as: tx -> 0; rx -> 1; pcs -> 2;
|
|
* tx2 -> 3; rx2 -> 4; pcs_misc (optional) -> 5
|
|
*/
|
|
qmp->tx = devm_of_iomap(dev, np, 0, NULL);
|
|
if (IS_ERR(qmp->tx))
|
|
return PTR_ERR(qmp->tx);
|
|
|
|
qmp->rx = devm_of_iomap(dev, np, 1, NULL);
|
|
if (IS_ERR(qmp->rx))
|
|
return PTR_ERR(qmp->rx);
|
|
|
|
qmp->pcs = devm_of_iomap(dev, np, 2, NULL);
|
|
if (IS_ERR(qmp->pcs))
|
|
return PTR_ERR(qmp->pcs);
|
|
|
|
if (cfg->pcs_usb_offset)
|
|
qmp->pcs_usb = qmp->pcs + cfg->pcs_usb_offset;
|
|
|
|
qmp->tx2 = devm_of_iomap(dev, np, 3, NULL);
|
|
if (IS_ERR(qmp->tx2))
|
|
return PTR_ERR(qmp->tx2);
|
|
|
|
qmp->rx2 = devm_of_iomap(dev, np, 4, NULL);
|
|
if (IS_ERR(qmp->rx2))
|
|
return PTR_ERR(qmp->rx2);
|
|
|
|
qmp->pcs_misc = devm_of_iomap(dev, np, 5, NULL);
|
|
if (IS_ERR(qmp->pcs_misc)) {
|
|
dev_vdbg(dev, "PHY pcs_misc-reg not used\n");
|
|
qmp->pcs_misc = NULL;
|
|
}
|
|
|
|
qmp->pipe_clk = devm_get_clk_from_child(dev, np, NULL);
|
|
if (IS_ERR(qmp->pipe_clk)) {
|
|
return dev_err_probe(dev, PTR_ERR(qmp->pipe_clk),
|
|
"failed to get pipe clock\n");
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_parse_dt_legacy(struct qmp_combo *qmp, struct device_node *usb_np,
|
|
struct device_node *dp_np)
|
|
{
|
|
struct platform_device *pdev = to_platform_device(qmp->dev);
|
|
int ret;
|
|
|
|
qmp->serdes = devm_platform_ioremap_resource(pdev, 0);
|
|
if (IS_ERR(qmp->serdes))
|
|
return PTR_ERR(qmp->serdes);
|
|
|
|
qmp->com = devm_platform_ioremap_resource(pdev, 1);
|
|
if (IS_ERR(qmp->com))
|
|
return PTR_ERR(qmp->com);
|
|
|
|
qmp->dp_serdes = devm_platform_ioremap_resource(pdev, 2);
|
|
if (IS_ERR(qmp->dp_serdes))
|
|
return PTR_ERR(qmp->dp_serdes);
|
|
|
|
ret = qmp_combo_parse_dt_lecacy_usb(qmp, usb_np);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = qmp_combo_parse_dt_lecacy_dp(qmp, dp_np);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = devm_clk_bulk_get_all(qmp->dev, &qmp->clks);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
qmp->num_clks = ret;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qmp_combo_parse_dt(struct qmp_combo *qmp)
|
|
{
|
|
struct platform_device *pdev = to_platform_device(qmp->dev);
|
|
const struct qmp_phy_cfg *cfg = qmp->cfg;
|
|
const struct qmp_combo_offsets *offs = cfg->offsets;
|
|
struct device *dev = qmp->dev;
|
|
void __iomem *base;
|
|
int ret;
|
|
|
|
if (!offs)
|
|
return -EINVAL;
|
|
|
|
base = devm_platform_ioremap_resource(pdev, 0);
|
|
if (IS_ERR(base))
|
|
return PTR_ERR(base);
|
|
|
|
qmp->com = base + offs->com;
|
|
qmp->tx = base + offs->txa;
|
|
qmp->rx = base + offs->rxa;
|
|
qmp->tx2 = base + offs->txb;
|
|
qmp->rx2 = base + offs->rxb;
|
|
|
|
qmp->serdes = base + offs->usb3_serdes;
|
|
qmp->pcs_misc = base + offs->usb3_pcs_misc;
|
|
qmp->pcs = base + offs->usb3_pcs;
|
|
qmp->pcs_usb = base + offs->usb3_pcs_usb;
|
|
|
|
qmp->dp_serdes = base + offs->dp_serdes;
|
|
if (offs->dp_txa) {
|
|
qmp->dp_tx = base + offs->dp_txa;
|
|
qmp->dp_tx2 = base + offs->dp_txb;
|
|
} else {
|
|
qmp->dp_tx = base + offs->txa;
|
|
qmp->dp_tx2 = base + offs->txb;
|
|
}
|
|
qmp->dp_dp_phy = base + offs->dp_dp_phy;
|
|
|
|
ret = qmp_combo_clk_init(qmp);
|
|
if (ret)
|
|
return ret;
|
|
|
|
qmp->pipe_clk = devm_clk_get(dev, "usb3_pipe");
|
|
if (IS_ERR(qmp->pipe_clk)) {
|
|
return dev_err_probe(dev, PTR_ERR(qmp->pipe_clk),
|
|
"failed to get usb3_pipe clock\n");
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static struct phy *qmp_combo_phy_xlate(struct device *dev, const struct of_phandle_args *args)
|
|
{
|
|
struct qmp_combo *qmp = dev_get_drvdata(dev);
|
|
|
|
if (args->args_count == 0)
|
|
return ERR_PTR(-EINVAL);
|
|
|
|
switch (args->args[0]) {
|
|
case QMP_USB43DP_USB3_PHY:
|
|
return qmp->usb_phy;
|
|
case QMP_USB43DP_DP_PHY:
|
|
return qmp->dp_phy;
|
|
}
|
|
|
|
return ERR_PTR(-EINVAL);
|
|
}
|
|
|
|
static int qmp_combo_probe(struct platform_device *pdev)
|
|
{
|
|
struct qmp_combo *qmp;
|
|
struct device *dev = &pdev->dev;
|
|
struct device_node *dp_np, *usb_np;
|
|
struct phy_provider *phy_provider;
|
|
int ret;
|
|
|
|
qmp = devm_kzalloc(dev, sizeof(*qmp), GFP_KERNEL);
|
|
if (!qmp)
|
|
return -ENOMEM;
|
|
|
|
qmp->dev = dev;
|
|
|
|
qmp->orientation = TYPEC_ORIENTATION_NORMAL;
|
|
|
|
qmp->cfg = of_device_get_match_data(dev);
|
|
if (!qmp->cfg)
|
|
return -EINVAL;
|
|
|
|
mutex_init(&qmp->phy_mutex);
|
|
|
|
ret = qmp_combo_reset_init(qmp);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = qmp_combo_vreg_init(qmp);
|
|
if (ret)
|
|
return ret;
|
|
|
|
/* Check for legacy binding with child nodes. */
|
|
usb_np = of_get_child_by_name(dev->of_node, "usb3-phy");
|
|
if (usb_np) {
|
|
dp_np = of_get_child_by_name(dev->of_node, "dp-phy");
|
|
if (!dp_np) {
|
|
of_node_put(usb_np);
|
|
return -EINVAL;
|
|
}
|
|
|
|
ret = qmp_combo_parse_dt_legacy(qmp, usb_np, dp_np);
|
|
} else {
|
|
usb_np = of_node_get(dev->of_node);
|
|
dp_np = of_node_get(dev->of_node);
|
|
|
|
ret = qmp_combo_parse_dt(qmp);
|
|
}
|
|
if (ret)
|
|
goto err_node_put;
|
|
|
|
ret = qmp_combo_typec_switch_register(qmp);
|
|
if (ret)
|
|
goto err_node_put;
|
|
|
|
ret = drm_aux_bridge_register(dev);
|
|
if (ret)
|
|
goto err_node_put;
|
|
|
|
pm_runtime_set_active(dev);
|
|
ret = devm_pm_runtime_enable(dev);
|
|
if (ret)
|
|
goto err_node_put;
|
|
/*
|
|
* Prevent runtime pm from being ON by default. Users can enable
|
|
* it using power/control in sysfs.
|
|
*/
|
|
pm_runtime_forbid(dev);
|
|
|
|
ret = qmp_combo_register_clocks(qmp, usb_np, dp_np);
|
|
if (ret)
|
|
goto err_node_put;
|
|
|
|
qmp->usb_phy = devm_phy_create(dev, usb_np, &qmp_combo_usb_phy_ops);
|
|
if (IS_ERR(qmp->usb_phy)) {
|
|
ret = PTR_ERR(qmp->usb_phy);
|
|
dev_err(dev, "failed to create USB PHY: %d\n", ret);
|
|
goto err_node_put;
|
|
}
|
|
|
|
phy_set_drvdata(qmp->usb_phy, qmp);
|
|
|
|
qmp->dp_phy = devm_phy_create(dev, dp_np, &qmp_combo_dp_phy_ops);
|
|
if (IS_ERR(qmp->dp_phy)) {
|
|
ret = PTR_ERR(qmp->dp_phy);
|
|
dev_err(dev, "failed to create DP PHY: %d\n", ret);
|
|
goto err_node_put;
|
|
}
|
|
|
|
phy_set_drvdata(qmp->dp_phy, qmp);
|
|
|
|
dev_set_drvdata(dev, qmp);
|
|
|
|
if (usb_np == dev->of_node)
|
|
phy_provider = devm_of_phy_provider_register(dev, qmp_combo_phy_xlate);
|
|
else
|
|
phy_provider = devm_of_phy_provider_register(dev, of_phy_simple_xlate);
|
|
|
|
of_node_put(usb_np);
|
|
of_node_put(dp_np);
|
|
|
|
return PTR_ERR_OR_ZERO(phy_provider);
|
|
|
|
err_node_put:
|
|
of_node_put(usb_np);
|
|
of_node_put(dp_np);
|
|
return ret;
|
|
}
|
|
|
|
static const struct of_device_id qmp_combo_of_match_table[] = {
|
|
{
|
|
.compatible = "qcom,sc7180-qmp-usb3-dp-phy",
|
|
.data = &sc7180_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sc7280-qmp-usb3-dp-phy",
|
|
.data = &sm8250_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sc8180x-qmp-usb3-dp-phy",
|
|
.data = &sc8180x_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sc8280xp-qmp-usb43dp-phy",
|
|
.data = &sc8280xp_usb43dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sdm845-qmp-usb3-dp-phy",
|
|
.data = &sdm845_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm6350-qmp-usb3-dp-phy",
|
|
.data = &sm6350_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm8150-qmp-usb3-dp-phy",
|
|
.data = &sc8180x_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm8250-qmp-usb3-dp-phy",
|
|
.data = &sm8250_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm8350-qmp-usb3-dp-phy",
|
|
.data = &sm8350_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm8450-qmp-usb3-dp-phy",
|
|
.data = &sm8350_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm8550-qmp-usb3-dp-phy",
|
|
.data = &sm8550_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,sm8650-qmp-usb3-dp-phy",
|
|
.data = &sm8650_usb3dpphy_cfg,
|
|
},
|
|
{
|
|
.compatible = "qcom,x1e80100-qmp-usb3-dp-phy",
|
|
.data = &x1e80100_usb3dpphy_cfg,
|
|
},
|
|
{ }
|
|
};
|
|
MODULE_DEVICE_TABLE(of, qmp_combo_of_match_table);
|
|
|
|
static struct platform_driver qmp_combo_driver = {
|
|
.probe = qmp_combo_probe,
|
|
.driver = {
|
|
.name = "qcom-qmp-combo-phy",
|
|
.pm = &qmp_combo_pm_ops,
|
|
.of_match_table = qmp_combo_of_match_table,
|
|
},
|
|
};
|
|
|
|
module_platform_driver(qmp_combo_driver);
|
|
|
|
MODULE_AUTHOR("Vivek Gautam <vivek.gautam@codeaurora.org>");
|
|
MODULE_DESCRIPTION("Qualcomm QMP USB+DP combo PHY driver");
|
|
MODULE_LICENSE("GPL v2");
|