mirror of
https://github.com/torvalds/linux
synced 2024-10-05 10:52:54 +00:00
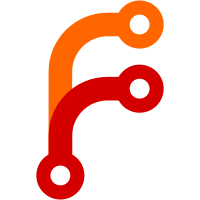
Signals are always delivered to 64-bit tasks with CS set to a long mode segment. In long mode, SS doesn't matter as long as it's a present writable segment. If SS starts out invalid (this can happen if the signal was caused by an IRET fault or was delivered on the way out of set_thread_area or modify_ldt), then IRET to the signal handler can fail, eventually killing the task. The straightforward fix would be to simply reset SS when delivering a signal. That breaks DOSEMU, though: 64-bit builds of DOSEMU rely on SS being set to the faulting SS when signals are delivered. As a compromise, this patch leaves SS alone so long as it's valid. The net effect should be that the behavior of successfully delivered signals is unchanged. Some signals that would previously have failed to be delivered will now be delivered successfully. This has no effect for x32 or 32-bit tasks: their signal handlers were already called with SS == __USER_DS. (On Xen, there's a slight hole: if a task sets SS to a writable *kernel* data segment, then we will fail to identify it as invalid and we'll still kill the task. If anyone cares, this could be fixed with a new paravirt hook.) Signed-off-by: Andy Lutomirski <luto@kernel.org> Acked-by: Borislav Petkov <bp@alien8.de> Cc: Al Viro <viro@zeniv.linux.org.uk> Cc: Andy Lutomirski <luto@amacapital.net> Cc: Brian Gerst <brgerst@gmail.com> Cc: Cyrill Gorcunov <gorcunov@gmail.com> Cc: Denys Vlasenko <dvlasenk@redhat.com> Cc: H. Peter Anvin <hpa@zytor.com> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Oleg Nesterov <oleg@redhat.com> Cc: Pavel Emelyanov <xemul@parallels.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Stas Sergeev <stsp@list.ru> Cc: Thomas Gleixner <tglx@linutronix.de> Link: http://lkml.kernel.org/r/163c6e1eacde41388f3ff4d2fe6769be651d7b6e.1455664054.git.luto@kernel.org Signed-off-by: Ingo Molnar <mingo@kernel.org>
125 lines
3.4 KiB
C
125 lines
3.4 KiB
C
/* Written 2000 by Andi Kleen */
|
|
#ifndef _ASM_X86_DESC_DEFS_H
|
|
#define _ASM_X86_DESC_DEFS_H
|
|
|
|
/*
|
|
* Segment descriptor structure definitions, usable from both x86_64 and i386
|
|
* archs.
|
|
*/
|
|
|
|
#ifndef __ASSEMBLY__
|
|
|
|
#include <linux/types.h>
|
|
|
|
/*
|
|
* FIXME: Accessing the desc_struct through its fields is more elegant,
|
|
* and should be the one valid thing to do. However, a lot of open code
|
|
* still touches the a and b accessors, and doing this allow us to do it
|
|
* incrementally. We keep the signature as a struct, rather than an union,
|
|
* so we can get rid of it transparently in the future -- glommer
|
|
*/
|
|
/* 8 byte segment descriptor */
|
|
struct desc_struct {
|
|
union {
|
|
struct {
|
|
unsigned int a;
|
|
unsigned int b;
|
|
};
|
|
struct {
|
|
u16 limit0;
|
|
u16 base0;
|
|
unsigned base1: 8, type: 4, s: 1, dpl: 2, p: 1;
|
|
unsigned limit: 4, avl: 1, l: 1, d: 1, g: 1, base2: 8;
|
|
};
|
|
};
|
|
} __attribute__((packed));
|
|
|
|
#define GDT_ENTRY_INIT(flags, base, limit) { { { \
|
|
.a = ((limit) & 0xffff) | (((base) & 0xffff) << 16), \
|
|
.b = (((base) & 0xff0000) >> 16) | (((flags) & 0xf0ff) << 8) | \
|
|
((limit) & 0xf0000) | ((base) & 0xff000000), \
|
|
} } }
|
|
|
|
enum {
|
|
GATE_INTERRUPT = 0xE,
|
|
GATE_TRAP = 0xF,
|
|
GATE_CALL = 0xC,
|
|
GATE_TASK = 0x5,
|
|
};
|
|
|
|
/* 16byte gate */
|
|
struct gate_struct64 {
|
|
u16 offset_low;
|
|
u16 segment;
|
|
unsigned ist : 3, zero0 : 5, type : 5, dpl : 2, p : 1;
|
|
u16 offset_middle;
|
|
u32 offset_high;
|
|
u32 zero1;
|
|
} __attribute__((packed));
|
|
|
|
#define PTR_LOW(x) ((unsigned long long)(x) & 0xFFFF)
|
|
#define PTR_MIDDLE(x) (((unsigned long long)(x) >> 16) & 0xFFFF)
|
|
#define PTR_HIGH(x) ((unsigned long long)(x) >> 32)
|
|
|
|
enum {
|
|
DESC_TSS = 0x9,
|
|
DESC_LDT = 0x2,
|
|
DESCTYPE_S = 0x10, /* !system */
|
|
};
|
|
|
|
/* LDT or TSS descriptor in the GDT. 16 bytes. */
|
|
struct ldttss_desc64 {
|
|
u16 limit0;
|
|
u16 base0;
|
|
unsigned base1 : 8, type : 5, dpl : 2, p : 1;
|
|
unsigned limit1 : 4, zero0 : 3, g : 1, base2 : 8;
|
|
u32 base3;
|
|
u32 zero1;
|
|
} __attribute__((packed));
|
|
|
|
#ifdef CONFIG_X86_64
|
|
typedef struct gate_struct64 gate_desc;
|
|
typedef struct ldttss_desc64 ldt_desc;
|
|
typedef struct ldttss_desc64 tss_desc;
|
|
#define gate_offset(g) ((g).offset_low | ((unsigned long)(g).offset_middle << 16) | ((unsigned long)(g).offset_high << 32))
|
|
#define gate_segment(g) ((g).segment)
|
|
#else
|
|
typedef struct desc_struct gate_desc;
|
|
typedef struct desc_struct ldt_desc;
|
|
typedef struct desc_struct tss_desc;
|
|
#define gate_offset(g) (((g).b & 0xffff0000) | ((g).a & 0x0000ffff))
|
|
#define gate_segment(g) ((g).a >> 16)
|
|
#endif
|
|
|
|
struct desc_ptr {
|
|
unsigned short size;
|
|
unsigned long address;
|
|
} __attribute__((packed)) ;
|
|
|
|
#endif /* !__ASSEMBLY__ */
|
|
|
|
/* Access rights as returned by LAR */
|
|
#define AR_TYPE_RODATA (0 * (1 << 9))
|
|
#define AR_TYPE_RWDATA (1 * (1 << 9))
|
|
#define AR_TYPE_RODATA_EXPDOWN (2 * (1 << 9))
|
|
#define AR_TYPE_RWDATA_EXPDOWN (3 * (1 << 9))
|
|
#define AR_TYPE_XOCODE (4 * (1 << 9))
|
|
#define AR_TYPE_XRCODE (5 * (1 << 9))
|
|
#define AR_TYPE_XOCODE_CONF (6 * (1 << 9))
|
|
#define AR_TYPE_XRCODE_CONF (7 * (1 << 9))
|
|
#define AR_TYPE_MASK (7 * (1 << 9))
|
|
|
|
#define AR_DPL0 (0 * (1 << 13))
|
|
#define AR_DPL3 (3 * (1 << 13))
|
|
#define AR_DPL_MASK (3 * (1 << 13))
|
|
|
|
#define AR_A (1 << 8) /* "Accessed" */
|
|
#define AR_S (1 << 12) /* If clear, "System" segment */
|
|
#define AR_P (1 << 15) /* "Present" */
|
|
#define AR_AVL (1 << 20) /* "AVaiLable" (no HW effect) */
|
|
#define AR_L (1 << 21) /* "Long mode" for code segments */
|
|
#define AR_DB (1 << 22) /* D/B, effect depends on type */
|
|
#define AR_G (1 << 23) /* "Granularity" (limit in pages) */
|
|
|
|
#endif /* _ASM_X86_DESC_DEFS_H */
|