mirror of
https://github.com/torvalds/linux
synced 2024-09-06 09:51:23 +00:00
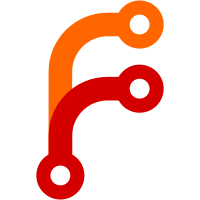
The control queue can be used by different parts of the driver to send commands to the device. Control messages can be either synchronous or asynchronous. The lifetime of a message is controlled by a reference count. Introduce a module parameter to set the message completion timeout: msg_timeout_ms [=1000] Signed-off-by: Anton Yakovlev <anton.yakovlev@opensynergy.com> Link: https://lore.kernel.org/r/20210302164709.3142702-4-anton.yakovlev@opensynergy.com Signed-off-by: Takashi Iwai <tiwai@suse.de>
73 lines
1.7 KiB
C
73 lines
1.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* virtio-snd: Virtio sound device
|
|
* Copyright (C) 2021 OpenSynergy GmbH
|
|
*/
|
|
#ifndef VIRTIO_SND_CARD_H
|
|
#define VIRTIO_SND_CARD_H
|
|
|
|
#include <linux/slab.h>
|
|
#include <linux/virtio.h>
|
|
#include <sound/core.h>
|
|
#include <uapi/linux/virtio_snd.h>
|
|
|
|
#include "virtio_ctl_msg.h"
|
|
|
|
#define VIRTIO_SND_CARD_DRIVER "virtio-snd"
|
|
#define VIRTIO_SND_CARD_NAME "VirtIO SoundCard"
|
|
|
|
/**
|
|
* struct virtio_snd_queue - Virtqueue wrapper structure.
|
|
* @lock: Used to synchronize access to a virtqueue.
|
|
* @vqueue: Underlying virtqueue.
|
|
*/
|
|
struct virtio_snd_queue {
|
|
spinlock_t lock;
|
|
struct virtqueue *vqueue;
|
|
};
|
|
|
|
/**
|
|
* struct virtio_snd - VirtIO sound card device.
|
|
* @vdev: Underlying virtio device.
|
|
* @queues: Virtqueue wrappers.
|
|
* @card: ALSA sound card.
|
|
* @ctl_msgs: Pending control request list.
|
|
* @event_msgs: Device events.
|
|
*/
|
|
struct virtio_snd {
|
|
struct virtio_device *vdev;
|
|
struct virtio_snd_queue queues[VIRTIO_SND_VQ_MAX];
|
|
struct snd_card *card;
|
|
struct list_head ctl_msgs;
|
|
struct virtio_snd_event *event_msgs;
|
|
};
|
|
|
|
/* Message completion timeout in milliseconds (module parameter). */
|
|
extern u32 virtsnd_msg_timeout_ms;
|
|
|
|
static inline struct virtio_snd_queue *
|
|
virtsnd_control_queue(struct virtio_snd *snd)
|
|
{
|
|
return &snd->queues[VIRTIO_SND_VQ_CONTROL];
|
|
}
|
|
|
|
static inline struct virtio_snd_queue *
|
|
virtsnd_event_queue(struct virtio_snd *snd)
|
|
{
|
|
return &snd->queues[VIRTIO_SND_VQ_EVENT];
|
|
}
|
|
|
|
static inline struct virtio_snd_queue *
|
|
virtsnd_tx_queue(struct virtio_snd *snd)
|
|
{
|
|
return &snd->queues[VIRTIO_SND_VQ_TX];
|
|
}
|
|
|
|
static inline struct virtio_snd_queue *
|
|
virtsnd_rx_queue(struct virtio_snd *snd)
|
|
{
|
|
return &snd->queues[VIRTIO_SND_VQ_RX];
|
|
}
|
|
|
|
#endif /* VIRTIO_SND_CARD_H */
|