mirror of
https://github.com/torvalds/linux
synced 2024-07-23 03:29:48 +00:00
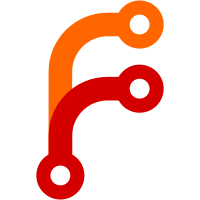
Based on 1 normalized pattern(s): this program include file is free software you can redistribute it and or modify it under the terms of the gnu general public license as published by the free software foundation either version 2 of the license or at your option any later version this program include file is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with this program in the main directory of the linux [ntfs] distribution in the file copying if not write to the free software foundation inc 59 temple place suite 330 boston ma 02111 1307 usa extracted by the scancode license scanner the SPDX license identifier GPL-2.0-or-later has been chosen to replace the boilerplate/reference in 43 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Richard Fontana <rfontana@redhat.com> Reviewed-by: Allison Randal <allison@lohutok.net> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190520075212.517001706@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
90 lines
2.8 KiB
C
90 lines
2.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* time.h - NTFS time conversion functions. Part of the Linux-NTFS project.
|
|
*
|
|
* Copyright (c) 2001-2005 Anton Altaparmakov
|
|
*/
|
|
|
|
#ifndef _LINUX_NTFS_TIME_H
|
|
#define _LINUX_NTFS_TIME_H
|
|
|
|
#include <linux/time.h> /* For current_kernel_time(). */
|
|
#include <asm/div64.h> /* For do_div(). */
|
|
|
|
#include "endian.h"
|
|
|
|
#define NTFS_TIME_OFFSET ((s64)(369 * 365 + 89) * 24 * 3600 * 10000000)
|
|
|
|
/**
|
|
* utc2ntfs - convert Linux UTC time to NTFS time
|
|
* @ts: Linux UTC time to convert to NTFS time
|
|
*
|
|
* Convert the Linux UTC time @ts to its corresponding NTFS time and return
|
|
* that in little endian format.
|
|
*
|
|
* Linux stores time in a struct timespec64 consisting of a time64_t tv_sec
|
|
* and a long tv_nsec where tv_sec is the number of 1-second intervals since
|
|
* 1st January 1970, 00:00:00 UTC and tv_nsec is the number of 1-nano-second
|
|
* intervals since the value of tv_sec.
|
|
*
|
|
* NTFS uses Microsoft's standard time format which is stored in a s64 and is
|
|
* measured as the number of 100-nano-second intervals since 1st January 1601,
|
|
* 00:00:00 UTC.
|
|
*/
|
|
static inline sle64 utc2ntfs(const struct timespec64 ts)
|
|
{
|
|
/*
|
|
* Convert the seconds to 100ns intervals, add the nano-seconds
|
|
* converted to 100ns intervals, and then add the NTFS time offset.
|
|
*/
|
|
return cpu_to_sle64((s64)ts.tv_sec * 10000000 + ts.tv_nsec / 100 +
|
|
NTFS_TIME_OFFSET);
|
|
}
|
|
|
|
/**
|
|
* get_current_ntfs_time - get the current time in little endian NTFS format
|
|
*
|
|
* Get the current time from the Linux kernel, convert it to its corresponding
|
|
* NTFS time and return that in little endian format.
|
|
*/
|
|
static inline sle64 get_current_ntfs_time(void)
|
|
{
|
|
struct timespec64 ts;
|
|
|
|
ktime_get_coarse_real_ts64(&ts);
|
|
return utc2ntfs(ts);
|
|
}
|
|
|
|
/**
|
|
* ntfs2utc - convert NTFS time to Linux time
|
|
* @time: NTFS time (little endian) to convert to Linux UTC
|
|
*
|
|
* Convert the little endian NTFS time @time to its corresponding Linux UTC
|
|
* time and return that in cpu format.
|
|
*
|
|
* Linux stores time in a struct timespec64 consisting of a time64_t tv_sec
|
|
* and a long tv_nsec where tv_sec is the number of 1-second intervals since
|
|
* 1st January 1970, 00:00:00 UTC and tv_nsec is the number of 1-nano-second
|
|
* intervals since the value of tv_sec.
|
|
*
|
|
* NTFS uses Microsoft's standard time format which is stored in a s64 and is
|
|
* measured as the number of 100 nano-second intervals since 1st January 1601,
|
|
* 00:00:00 UTC.
|
|
*/
|
|
static inline struct timespec64 ntfs2utc(const sle64 time)
|
|
{
|
|
struct timespec64 ts;
|
|
|
|
/* Subtract the NTFS time offset. */
|
|
u64 t = (u64)(sle64_to_cpu(time) - NTFS_TIME_OFFSET);
|
|
/*
|
|
* Convert the time to 1-second intervals and the remainder to
|
|
* 1-nano-second intervals.
|
|
*/
|
|
ts.tv_nsec = do_div(t, 10000000) * 100;
|
|
ts.tv_sec = t;
|
|
return ts;
|
|
}
|
|
|
|
#endif /* _LINUX_NTFS_TIME_H */
|