mirror of
https://github.com/torvalds/linux
synced 2024-07-21 18:51:47 +00:00
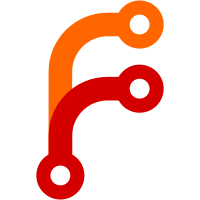
strlcpy() reads the entire source buffer first. This read may exceed the destination size limit. This is both inefficient and can lead to linear read overflows if a source string is not NUL-terminated [1]. In an effort to remove strlcpy() completely [2], replace strlcpy() here with strscpy(). Direct replacement is safe here since return value of -errno is used to check for truncation instead of sizeof(dest). [1] https://www.kernel.org/doc/html/latest/process/deprecated.html#strlcpy [2] https://github.com/KSPP/linux/issues/89 Signed-off-by: Azeem Shaikh <azeemshaikh38@gmail.com> Reviewed-by: Justin Stitt <justinstitt@google.com> Reviewed-by: Kees Cook <keescook@chromium.org> Link: https://lore.kernel.org/r/20230830160806.3821893-1-azeemshaikh38@gmail.com Signed-off-by: Kees Cook <keescook@chromium.org>
55 lines
1.3 KiB
C
55 lines
1.3 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* linux/init/version.c
|
|
*
|
|
* Copyright (C) 1992 Theodore Ts'o
|
|
*
|
|
* May be freely distributed as part of Linux.
|
|
*/
|
|
|
|
#include <generated/compile.h>
|
|
#include <linux/build-salt.h>
|
|
#include <linux/elfnote-lto.h>
|
|
#include <linux/export.h>
|
|
#include <linux/init.h>
|
|
#include <linux/printk.h>
|
|
#include <linux/uts.h>
|
|
#include <linux/utsname.h>
|
|
#include <linux/proc_ns.h>
|
|
|
|
static int __init early_hostname(char *arg)
|
|
{
|
|
size_t bufsize = sizeof(init_uts_ns.name.nodename);
|
|
size_t maxlen = bufsize - 1;
|
|
ssize_t arglen;
|
|
|
|
arglen = strscpy(init_uts_ns.name.nodename, arg, bufsize);
|
|
if (arglen < 0) {
|
|
pr_warn("hostname parameter exceeds %zd characters and will be truncated",
|
|
maxlen);
|
|
}
|
|
return 0;
|
|
}
|
|
early_param("hostname", early_hostname);
|
|
|
|
const char linux_proc_banner[] =
|
|
"%s version %s"
|
|
" (" LINUX_COMPILE_BY "@" LINUX_COMPILE_HOST ")"
|
|
" (" LINUX_COMPILER ") %s\n";
|
|
|
|
BUILD_SALT;
|
|
BUILD_LTO_INFO;
|
|
|
|
/*
|
|
* init_uts_ns and linux_banner contain the build version and timestamp,
|
|
* which are really fixed at the very last step of build process.
|
|
* They are compiled with __weak first, and without __weak later.
|
|
*/
|
|
|
|
struct uts_namespace init_uts_ns __weak;
|
|
const char linux_banner[] __weak;
|
|
|
|
#include "version-timestamp.c"
|
|
|
|
EXPORT_SYMBOL_GPL(init_uts_ns);
|