mirror of
https://github.com/torvalds/linux
synced 2024-09-30 16:29:47 +00:00
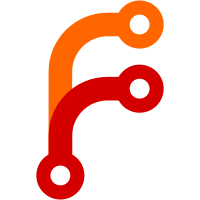
This commit adds fixed tracepoints to the ext3 code. It is based on ext4 tracepoints, however due to the differences of both file systems, there are some tracepoints missing (those for delaloc and for multi-block allocator) and there are some ext3 specific as well (for reservation windows). Here is a list: ext3_free_inode ext3_request_inode ext3_allocate_inode ext3_evict_inode ext3_drop_inode ext3_mark_inode_dirty ext3_write_begin ext3_ordered_write_end ext3_writeback_write_end ext3_journalled_write_end ext3_ordered_writepage ext3_writeback_writepage ext3_journalled_writepage ext3_readpage ext3_releasepage ext3_invalidatepage ext3_discard_blocks ext3_request_blocks ext3_allocate_blocks ext3_free_blocks ext3_sync_file_enter ext3_sync_file_exit ext3_sync_fs ext3_rsv_window_add ext3_discard_reservation ext3_alloc_new_reservation ext3_reserved ext3_forget ext3_read_block_bitmap ext3_direct_IO_enter ext3_direct_IO_exit ext3_unlink_enter ext3_unlink_exit ext3_truncate_enter ext3_truncate_exit ext3_get_blocks_enter ext3_get_blocks_exit ext3_load_inode Signed-off-by: Lukas Czerner <lczerner@redhat.com> Cc: Jan Kara <jack@suse.cz> Signed-off-by: Jan Kara <jack@suse.cz>
105 lines
3 KiB
C
105 lines
3 KiB
C
/*
|
|
* linux/fs/ext3/fsync.c
|
|
*
|
|
* Copyright (C) 1993 Stephen Tweedie (sct@redhat.com)
|
|
* from
|
|
* Copyright (C) 1992 Remy Card (card@masi.ibp.fr)
|
|
* Laboratoire MASI - Institut Blaise Pascal
|
|
* Universite Pierre et Marie Curie (Paris VI)
|
|
* from
|
|
* linux/fs/minix/truncate.c Copyright (C) 1991, 1992 Linus Torvalds
|
|
*
|
|
* ext3fs fsync primitive
|
|
*
|
|
* Big-endian to little-endian byte-swapping/bitmaps by
|
|
* David S. Miller (davem@caip.rutgers.edu), 1995
|
|
*
|
|
* Removed unnecessary code duplication for little endian machines
|
|
* and excessive __inline__s.
|
|
* Andi Kleen, 1997
|
|
*
|
|
* Major simplications and cleanup - we only need to do the metadata, because
|
|
* we can depend on generic_block_fdatasync() to sync the data blocks.
|
|
*/
|
|
|
|
#include <linux/time.h>
|
|
#include <linux/blkdev.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/writeback.h>
|
|
#include <linux/jbd.h>
|
|
#include <linux/ext3_fs.h>
|
|
#include <linux/ext3_jbd.h>
|
|
#include <trace/events/ext3.h>
|
|
|
|
/*
|
|
* akpm: A new design for ext3_sync_file().
|
|
*
|
|
* This is only called from sys_fsync(), sys_fdatasync() and sys_msync().
|
|
* There cannot be a transaction open by this task.
|
|
* Another task could have dirtied this inode. Its data can be in any
|
|
* state in the journalling system.
|
|
*
|
|
* What we do is just kick off a commit and wait on it. This will snapshot the
|
|
* inode to disk.
|
|
*/
|
|
|
|
int ext3_sync_file(struct file *file, int datasync)
|
|
{
|
|
struct inode *inode = file->f_mapping->host;
|
|
struct ext3_inode_info *ei = EXT3_I(inode);
|
|
journal_t *journal = EXT3_SB(inode->i_sb)->s_journal;
|
|
int ret, needs_barrier = 0;
|
|
tid_t commit_tid;
|
|
|
|
J_ASSERT(ext3_journal_current_handle() == NULL);
|
|
|
|
trace_ext3_sync_file_enter(file, datasync);
|
|
|
|
if (inode->i_sb->s_flags & MS_RDONLY)
|
|
return 0;
|
|
|
|
|
|
/*
|
|
* data=writeback,ordered:
|
|
* The caller's filemap_fdatawrite()/wait will sync the data.
|
|
* Metadata is in the journal, we wait for a proper transaction
|
|
* to commit here.
|
|
*
|
|
* data=journal:
|
|
* filemap_fdatawrite won't do anything (the buffers are clean).
|
|
* ext3_force_commit will write the file data into the journal and
|
|
* will wait on that.
|
|
* filemap_fdatawait() will encounter a ton of newly-dirtied pages
|
|
* (they were dirtied by commit). But that's OK - the blocks are
|
|
* safe in-journal, which is all fsync() needs to ensure.
|
|
*/
|
|
if (ext3_should_journal_data(inode)) {
|
|
ret = ext3_force_commit(inode->i_sb);
|
|
goto out;
|
|
}
|
|
|
|
if (datasync)
|
|
commit_tid = atomic_read(&ei->i_datasync_tid);
|
|
else
|
|
commit_tid = atomic_read(&ei->i_sync_tid);
|
|
|
|
if (test_opt(inode->i_sb, BARRIER) &&
|
|
!journal_trans_will_send_data_barrier(journal, commit_tid))
|
|
needs_barrier = 1;
|
|
log_start_commit(journal, commit_tid);
|
|
ret = log_wait_commit(journal, commit_tid);
|
|
|
|
/*
|
|
* In case we didn't commit a transaction, we have to flush
|
|
* disk caches manually so that data really is on persistent
|
|
* storage
|
|
*/
|
|
if (needs_barrier)
|
|
blkdev_issue_flush(inode->i_sb->s_bdev, GFP_KERNEL, NULL);
|
|
|
|
out:
|
|
trace_ext3_sync_file_exit(inode, ret);
|
|
return ret;
|
|
}
|