mirror of
https://github.com/torvalds/linux
synced 2024-07-21 02:23:16 +00:00
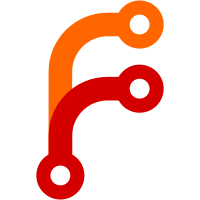
Support to inject result for NOP so that we can inject failure from userspace. It is very helpful for covering failure handling code in io_uring core change. With nop flags, it becomes possible to add more test features on NOP in future. Suggested-by: Jens Axboe <axboe@kernel.dk> Signed-off-by: Ming Lei <ming.lei@redhat.com> Link: https://lore.kernel.org/r/20240510035031.78874-3-ming.lei@redhat.com Signed-off-by: Jens Axboe <axboe@kernel.dk>
44 lines
961 B
C
44 lines
961 B
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/kernel.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/file.h>
|
|
#include <linux/io_uring.h>
|
|
|
|
#include <uapi/linux/io_uring.h>
|
|
|
|
#include "io_uring.h"
|
|
#include "nop.h"
|
|
|
|
struct io_nop {
|
|
/* NOTE: kiocb has the file as the first member, so don't do it here */
|
|
struct file *file;
|
|
int result;
|
|
};
|
|
|
|
int io_nop_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe)
|
|
{
|
|
unsigned int flags;
|
|
struct io_nop *nop = io_kiocb_to_cmd(req, struct io_nop);
|
|
|
|
flags = READ_ONCE(sqe->nop_flags);
|
|
if (flags & ~IORING_NOP_INJECT_RESULT)
|
|
return -EINVAL;
|
|
|
|
if (flags & IORING_NOP_INJECT_RESULT)
|
|
nop->result = READ_ONCE(sqe->len);
|
|
else
|
|
nop->result = 0;
|
|
return 0;
|
|
}
|
|
|
|
int io_nop(struct io_kiocb *req, unsigned int issue_flags)
|
|
{
|
|
struct io_nop *nop = io_kiocb_to_cmd(req, struct io_nop);
|
|
|
|
if (nop->result < 0)
|
|
req_set_fail(req);
|
|
io_req_set_res(req, nop->result, 0);
|
|
return IOU_OK;
|
|
}
|