mirror of
https://github.com/torvalds/linux
synced 2024-09-06 18:01:05 +00:00
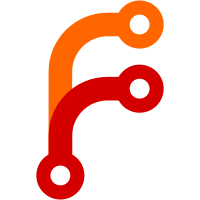
Tidy up the abort generation infrastructure in the following ways: (1) Create an enum and string mapping table to list the reasons an abort might be generated in tracing. (2) Replace the 3-char string with the values from (1) in the places that use that to log the abort source. This gets rid of a memcpy() in the tracepoint. (3) Subsume the rxrpc_rx_eproto tracepoint with the rxrpc_abort tracepoint and use values from (1) to indicate the trace reason. (4) Always make a call to an abort function at the point of the abort rather than stashing the values into variables and using goto to get to a place where it reported. The C optimiser will collapse the calls together as appropriate. The abort functions return a value that can be returned directly if appropriate. Note that this extends into afs also at the points where that generates an abort. To aid with this, the afs sources need to #define RXRPC_TRACE_ONLY_DEFINE_ENUMS before including the rxrpc tracing header because they don't have access to the rxrpc internal structures that some of the tracepoints make use of. Signed-off-by: David Howells <dhowells@redhat.com> cc: Marc Dionne <marc.dionne@auristor.com> cc: linux-afs@lists.infradead.org
77 lines
2.7 KiB
C
77 lines
2.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/* RxRPC kernel service interface definitions
|
|
*
|
|
* Copyright (C) 2007 Red Hat, Inc. All Rights Reserved.
|
|
* Written by David Howells (dhowells@redhat.com)
|
|
*/
|
|
|
|
#ifndef _NET_RXRPC_H
|
|
#define _NET_RXRPC_H
|
|
|
|
#include <linux/rxrpc.h>
|
|
#include <linux/ktime.h>
|
|
|
|
struct key;
|
|
struct sock;
|
|
struct socket;
|
|
struct rxrpc_call;
|
|
enum rxrpc_abort_reason;
|
|
|
|
enum rxrpc_interruptibility {
|
|
RXRPC_INTERRUPTIBLE, /* Call is interruptible */
|
|
RXRPC_PREINTERRUPTIBLE, /* Call can be cancelled whilst waiting for a slot */
|
|
RXRPC_UNINTERRUPTIBLE, /* Call should not be interruptible at all */
|
|
};
|
|
|
|
/*
|
|
* Debug ID counter for tracing.
|
|
*/
|
|
extern atomic_t rxrpc_debug_id;
|
|
|
|
typedef void (*rxrpc_notify_rx_t)(struct sock *, struct rxrpc_call *,
|
|
unsigned long);
|
|
typedef void (*rxrpc_notify_end_tx_t)(struct sock *, struct rxrpc_call *,
|
|
unsigned long);
|
|
typedef void (*rxrpc_notify_new_call_t)(struct sock *, struct rxrpc_call *,
|
|
unsigned long);
|
|
typedef void (*rxrpc_discard_new_call_t)(struct rxrpc_call *, unsigned long);
|
|
typedef void (*rxrpc_user_attach_call_t)(struct rxrpc_call *, unsigned long);
|
|
|
|
void rxrpc_kernel_new_call_notification(struct socket *,
|
|
rxrpc_notify_new_call_t,
|
|
rxrpc_discard_new_call_t);
|
|
struct rxrpc_call *rxrpc_kernel_begin_call(struct socket *,
|
|
struct sockaddr_rxrpc *,
|
|
struct key *,
|
|
unsigned long,
|
|
s64,
|
|
gfp_t,
|
|
rxrpc_notify_rx_t,
|
|
bool,
|
|
enum rxrpc_interruptibility,
|
|
unsigned int);
|
|
int rxrpc_kernel_send_data(struct socket *, struct rxrpc_call *,
|
|
struct msghdr *, size_t,
|
|
rxrpc_notify_end_tx_t);
|
|
int rxrpc_kernel_recv_data(struct socket *, struct rxrpc_call *,
|
|
struct iov_iter *, size_t *, bool, u32 *, u16 *);
|
|
bool rxrpc_kernel_abort_call(struct socket *, struct rxrpc_call *,
|
|
u32, int, enum rxrpc_abort_reason);
|
|
void rxrpc_kernel_end_call(struct socket *, struct rxrpc_call *);
|
|
void rxrpc_kernel_get_peer(struct socket *, struct rxrpc_call *,
|
|
struct sockaddr_rxrpc *);
|
|
bool rxrpc_kernel_get_srtt(struct socket *, struct rxrpc_call *, u32 *);
|
|
int rxrpc_kernel_charge_accept(struct socket *, rxrpc_notify_rx_t,
|
|
rxrpc_user_attach_call_t, unsigned long, gfp_t,
|
|
unsigned int);
|
|
void rxrpc_kernel_set_tx_length(struct socket *, struct rxrpc_call *, s64);
|
|
bool rxrpc_kernel_check_life(const struct socket *, const struct rxrpc_call *);
|
|
u32 rxrpc_kernel_get_epoch(struct socket *, struct rxrpc_call *);
|
|
void rxrpc_kernel_set_max_life(struct socket *, struct rxrpc_call *,
|
|
unsigned long);
|
|
|
|
int rxrpc_sock_set_min_security_level(struct sock *sk, unsigned int val);
|
|
int rxrpc_sock_set_security_keyring(struct sock *, struct key *);
|
|
|
|
#endif /* _NET_RXRPC_H */
|