mirror of
https://github.com/torvalds/linux
synced 2024-09-21 11:38:48 +00:00
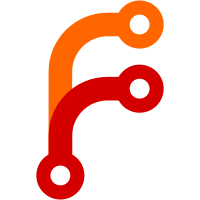
Generate unique values of st_dev per lower layer for non-samefs overlay mount. The unique values are obtained by allocating anonymous bdevs for each of the lowerdirs in the overlayfs instance. The anonymous bdev is going to be returned by stat(2) for lowerdir non-dir entries in non-samefs case. [amir: split from ovl_getattr() and re-structure patches] Signed-off-by: Chandan Rajendra <chandan@linux.vnet.ibm.com> Signed-off-by: Amir Goldstein <amir73il@gmail.com> Signed-off-by: Miklos Szeredi <mszeredi@redhat.com>
92 lines
2.1 KiB
C
92 lines
2.1 KiB
C
/*
|
|
*
|
|
* Copyright (C) 2011 Novell Inc.
|
|
* Copyright (C) 2016 Red Hat, Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 as published by
|
|
* the Free Software Foundation.
|
|
*/
|
|
|
|
struct ovl_config {
|
|
char *lowerdir;
|
|
char *upperdir;
|
|
char *workdir;
|
|
bool default_permissions;
|
|
bool redirect_dir;
|
|
bool index;
|
|
};
|
|
|
|
struct ovl_layer {
|
|
struct vfsmount *mnt;
|
|
dev_t pseudo_dev;
|
|
};
|
|
|
|
struct ovl_path {
|
|
struct ovl_layer *layer;
|
|
struct dentry *dentry;
|
|
};
|
|
|
|
/* private information held for overlayfs's superblock */
|
|
struct ovl_fs {
|
|
struct vfsmount *upper_mnt;
|
|
unsigned numlower;
|
|
struct ovl_layer *lower_layers;
|
|
/* workbasedir is the path at workdir= mount option */
|
|
struct dentry *workbasedir;
|
|
/* workdir is the 'work' directory under workbasedir */
|
|
struct dentry *workdir;
|
|
/* index directory listing overlay inodes by origin file handle */
|
|
struct dentry *indexdir;
|
|
long namelen;
|
|
/* pathnames of lower and upper dirs, for show_options */
|
|
struct ovl_config config;
|
|
/* creds of process who forced instantiation of super block */
|
|
const struct cred *creator_cred;
|
|
bool tmpfile;
|
|
bool noxattr;
|
|
/* sb common to all layers */
|
|
struct super_block *same_sb;
|
|
/* Did we take the inuse lock? */
|
|
bool upperdir_locked;
|
|
bool workdir_locked;
|
|
};
|
|
|
|
/* private information held for every overlayfs dentry */
|
|
struct ovl_entry {
|
|
union {
|
|
struct {
|
|
unsigned long has_upper;
|
|
bool opaque;
|
|
};
|
|
struct rcu_head rcu;
|
|
};
|
|
unsigned numlower;
|
|
struct ovl_path lowerstack[];
|
|
};
|
|
|
|
struct ovl_entry *ovl_alloc_entry(unsigned int numlower);
|
|
|
|
struct ovl_inode {
|
|
struct ovl_dir_cache *cache;
|
|
const char *redirect;
|
|
u64 version;
|
|
unsigned long flags;
|
|
struct inode vfs_inode;
|
|
struct dentry *__upperdentry;
|
|
struct inode *lower;
|
|
|
|
/* synchronize copy up and more */
|
|
struct mutex lock;
|
|
};
|
|
|
|
static inline struct ovl_inode *OVL_I(struct inode *inode)
|
|
{
|
|
return container_of(inode, struct ovl_inode, vfs_inode);
|
|
}
|
|
|
|
static inline struct dentry *ovl_upperdentry_dereference(struct ovl_inode *oi)
|
|
{
|
|
return lockless_dereference(oi->__upperdentry);
|
|
}
|