mirror of
https://github.com/torvalds/linux
synced 2024-09-30 00:10:51 +00:00
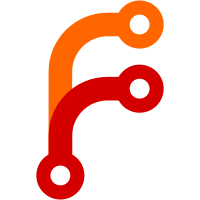
Instead of avoiding CFI entirely on the TLB flush helpers, reorganize the code so that the CFI machinery can deal with it. The important things to take into account are: - functions in asm called indirectly from C need to be defined using SYM_TYPED_FUNC_START() - a reference to the asm function needs to be visible to the compiler, in order to get it to emit the typeid symbol. The latter means that defining the cpu_tlb_fns structs is best done from C code, so that the references in the static initializers will be visible to the compiler. Signed-off-by: Ard Biesheuvel <ardb@kernel.org> Tested-by: Kees Cook <keescook@chromium.org> Reviewed-by: Sami Tolvanen <samitolvanen@google.com> Signed-off-by: Linus Walleij <linus.walleij@linaro.org> Signed-off-by: Russell King (Oracle) <rmk+kernel@armlinux.org.uk>
63 lines
1.5 KiB
ArmAsm
63 lines
1.5 KiB
ArmAsm
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* linux/arch/arm/mm/tlbv4.S
|
|
*
|
|
* Copyright (C) 1997-2002 Russell King
|
|
*
|
|
* ARM architecture version 4 TLB handling functions.
|
|
* These assume a split I/D TLBs, and no write buffer.
|
|
*
|
|
* Processors: ARM720T
|
|
*/
|
|
#include <linux/linkage.h>
|
|
#include <linux/init.h>
|
|
#include <linux/cfi_types.h>
|
|
#include <asm/assembler.h>
|
|
#include <asm/asm-offsets.h>
|
|
#include <asm/tlbflush.h>
|
|
#include "proc-macros.S"
|
|
|
|
.align 5
|
|
/*
|
|
* v4_flush_user_tlb_range(start, end, mm)
|
|
*
|
|
* Invalidate a range of TLB entries in the specified user address space.
|
|
*
|
|
* - start - range start address
|
|
* - end - range end address
|
|
* - mm - mm_struct describing address space
|
|
*/
|
|
.align 5
|
|
SYM_TYPED_FUNC_START(v4_flush_user_tlb_range)
|
|
vma_vm_mm ip, r2
|
|
act_mm r3 @ get current->active_mm
|
|
eors r3, ip, r3 @ == mm ?
|
|
retne lr @ no, we dont do anything
|
|
.v4_flush_kern_tlb_range:
|
|
bic r0, r0, #0x0ff
|
|
bic r0, r0, #0xf00
|
|
1: mcr p15, 0, r0, c8, c7, 1 @ invalidate TLB entry
|
|
add r0, r0, #PAGE_SZ
|
|
cmp r0, r1
|
|
blo 1b
|
|
ret lr
|
|
SYM_FUNC_END(v4_flush_user_tlb_range)
|
|
|
|
/*
|
|
* v4_flush_kern_tlb_range(start, end)
|
|
*
|
|
* Invalidate a range of TLB entries in the specified kernel
|
|
* address range.
|
|
*
|
|
* - start - virtual address (may not be aligned)
|
|
* - end - virtual address (may not be aligned)
|
|
*/
|
|
#ifdef CONFIG_CFI_CLANG
|
|
SYM_TYPED_FUNC_START(v4_flush_kern_tlb_range)
|
|
b .v4_flush_kern_tlb_range
|
|
SYM_FUNC_END(v4_flush_kern_tlb_range)
|
|
#else
|
|
.globl v4_flush_kern_tlb_range
|
|
.equ v4_flush_kern_tlb_range, .v4_flush_kern_tlb_range
|
|
#endif
|