mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
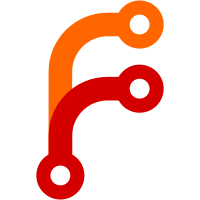
Fix cgroup v1 interference when non-root cgroup v2 BPF programs are used. Back in the days, commitbd1060a1d6
("sock, cgroup: add sock->sk_cgroup") embedded per-socket cgroup information into sock->sk_cgrp_data and in order to save 8 bytes in struct sock made both mutually exclusive, that is, when cgroup v1 socket tagging (e.g. net_cls/net_prio) is used, then cgroup v2 falls back to the root cgroup in sock_cgroup_ptr() (&cgrp_dfl_root.cgrp). The assumption made was "there is no reason to mix the two and this is in line with how legacy and v2 compatibility is handled" as stated inbd1060a1d6
. However, with Kubernetes more widely supporting cgroups v2 as well nowadays, this assumption no longer holds, and the possibility of the v1/v2 mixed mode with the v2 root fallback being hit becomes a real security issue. Many of the cgroup v2 BPF programs are also used for policy enforcement, just to pick _one_ example, that is, to programmatically deny socket related system calls like connect(2) or bind(2). A v2 root fallback would implicitly cause a policy bypass for the affected Pods. In production environments, we have recently seen this case due to various circumstances: i) a different 3rd party agent and/or ii) a container runtime such as [0] in the user's environment configuring legacy cgroup v1 net_cls tags, which triggered implicitly mentioned root fallback. Another case is Kubernetes projects like kind [1] which create Kubernetes nodes in a container and also add cgroup namespaces to the mix, meaning programs which are attached to the cgroup v2 root of the cgroup namespace get attached to a non-root cgroup v2 path from init namespace point of view. And the latter's root is out of reach for agents on a kind Kubernetes node to configure. Meaning, any entity on the node setting cgroup v1 net_cls tag will trigger the bypass despite cgroup v2 BPF programs attached to the namespace root. Generally, this mutual exclusiveness does not hold anymore in today's user environments and makes cgroup v2 usage from BPF side fragile and unreliable. This fix adds proper struct cgroup pointer for the cgroup v2 case to struct sock_cgroup_data in order to address these issues; this implicitly also fixes the tradeoffs being made back then with regards to races and refcount leaks as stated inbd1060a1d6
, and removes the fallback, so that cgroup v2 BPF programs always operate as expected. [0] https://github.com/nestybox/sysbox/ [1] https://kind.sigs.k8s.io/ Fixes:bd1060a1d6
("sock, cgroup: add sock->sk_cgroup") Signed-off-by: Daniel Borkmann <daniel@iogearbox.net> Signed-off-by: Alexei Starovoitov <ast@kernel.org> Acked-by: Stanislav Fomichev <sdf@google.com> Acked-by: Tejun Heo <tj@kernel.org> Link: https://lore.kernel.org/bpf/20210913230759.2313-1-daniel@iogearbox.net
296 lines
6.4 KiB
C
296 lines
6.4 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* net/core/netprio_cgroup.c Priority Control Group
|
|
*
|
|
* Authors: Neil Horman <nhorman@tuxdriver.com>
|
|
*/
|
|
|
|
#define pr_fmt(fmt) KBUILD_MODNAME ": " fmt
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/types.h>
|
|
#include <linux/string.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/cgroup.h>
|
|
#include <linux/rcupdate.h>
|
|
#include <linux/atomic.h>
|
|
#include <linux/sched/task.h>
|
|
|
|
#include <net/rtnetlink.h>
|
|
#include <net/pkt_cls.h>
|
|
#include <net/sock.h>
|
|
#include <net/netprio_cgroup.h>
|
|
|
|
#include <linux/fdtable.h>
|
|
|
|
/*
|
|
* netprio allocates per-net_device priomap array which is indexed by
|
|
* css->id. Limiting css ID to 16bits doesn't lose anything.
|
|
*/
|
|
#define NETPRIO_ID_MAX USHRT_MAX
|
|
|
|
#define PRIOMAP_MIN_SZ 128
|
|
|
|
/*
|
|
* Extend @dev->priomap so that it's large enough to accommodate
|
|
* @target_idx. @dev->priomap.priomap_len > @target_idx after successful
|
|
* return. Must be called under rtnl lock.
|
|
*/
|
|
static int extend_netdev_table(struct net_device *dev, u32 target_idx)
|
|
{
|
|
struct netprio_map *old, *new;
|
|
size_t new_sz, new_len;
|
|
|
|
/* is the existing priomap large enough? */
|
|
old = rtnl_dereference(dev->priomap);
|
|
if (old && old->priomap_len > target_idx)
|
|
return 0;
|
|
|
|
/*
|
|
* Determine the new size. Let's keep it power-of-two. We start
|
|
* from PRIOMAP_MIN_SZ and double it until it's large enough to
|
|
* accommodate @target_idx.
|
|
*/
|
|
new_sz = PRIOMAP_MIN_SZ;
|
|
while (true) {
|
|
new_len = (new_sz - offsetof(struct netprio_map, priomap)) /
|
|
sizeof(new->priomap[0]);
|
|
if (new_len > target_idx)
|
|
break;
|
|
new_sz *= 2;
|
|
/* overflowed? */
|
|
if (WARN_ON(new_sz < PRIOMAP_MIN_SZ))
|
|
return -ENOSPC;
|
|
}
|
|
|
|
/* allocate & copy */
|
|
new = kzalloc(new_sz, GFP_KERNEL);
|
|
if (!new)
|
|
return -ENOMEM;
|
|
|
|
if (old)
|
|
memcpy(new->priomap, old->priomap,
|
|
old->priomap_len * sizeof(old->priomap[0]));
|
|
|
|
new->priomap_len = new_len;
|
|
|
|
/* install the new priomap */
|
|
rcu_assign_pointer(dev->priomap, new);
|
|
if (old)
|
|
kfree_rcu(old, rcu);
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* netprio_prio - return the effective netprio of a cgroup-net_device pair
|
|
* @css: css part of the target pair
|
|
* @dev: net_device part of the target pair
|
|
*
|
|
* Should be called under RCU read or rtnl lock.
|
|
*/
|
|
static u32 netprio_prio(struct cgroup_subsys_state *css, struct net_device *dev)
|
|
{
|
|
struct netprio_map *map = rcu_dereference_rtnl(dev->priomap);
|
|
int id = css->id;
|
|
|
|
if (map && id < map->priomap_len)
|
|
return map->priomap[id];
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* netprio_set_prio - set netprio on a cgroup-net_device pair
|
|
* @css: css part of the target pair
|
|
* @dev: net_device part of the target pair
|
|
* @prio: prio to set
|
|
*
|
|
* Set netprio to @prio on @css-@dev pair. Should be called under rtnl
|
|
* lock and may fail under memory pressure for non-zero @prio.
|
|
*/
|
|
static int netprio_set_prio(struct cgroup_subsys_state *css,
|
|
struct net_device *dev, u32 prio)
|
|
{
|
|
struct netprio_map *map;
|
|
int id = css->id;
|
|
int ret;
|
|
|
|
/* avoid extending priomap for zero writes */
|
|
map = rtnl_dereference(dev->priomap);
|
|
if (!prio && (!map || map->priomap_len <= id))
|
|
return 0;
|
|
|
|
ret = extend_netdev_table(dev, id);
|
|
if (ret)
|
|
return ret;
|
|
|
|
map = rtnl_dereference(dev->priomap);
|
|
map->priomap[id] = prio;
|
|
return 0;
|
|
}
|
|
|
|
static struct cgroup_subsys_state *
|
|
cgrp_css_alloc(struct cgroup_subsys_state *parent_css)
|
|
{
|
|
struct cgroup_subsys_state *css;
|
|
|
|
css = kzalloc(sizeof(*css), GFP_KERNEL);
|
|
if (!css)
|
|
return ERR_PTR(-ENOMEM);
|
|
|
|
return css;
|
|
}
|
|
|
|
static int cgrp_css_online(struct cgroup_subsys_state *css)
|
|
{
|
|
struct cgroup_subsys_state *parent_css = css->parent;
|
|
struct net_device *dev;
|
|
int ret = 0;
|
|
|
|
if (css->id > NETPRIO_ID_MAX)
|
|
return -ENOSPC;
|
|
|
|
if (!parent_css)
|
|
return 0;
|
|
|
|
rtnl_lock();
|
|
/*
|
|
* Inherit prios from the parent. As all prios are set during
|
|
* onlining, there is no need to clear them on offline.
|
|
*/
|
|
for_each_netdev(&init_net, dev) {
|
|
u32 prio = netprio_prio(parent_css, dev);
|
|
|
|
ret = netprio_set_prio(css, dev, prio);
|
|
if (ret)
|
|
break;
|
|
}
|
|
rtnl_unlock();
|
|
return ret;
|
|
}
|
|
|
|
static void cgrp_css_free(struct cgroup_subsys_state *css)
|
|
{
|
|
kfree(css);
|
|
}
|
|
|
|
static u64 read_prioidx(struct cgroup_subsys_state *css, struct cftype *cft)
|
|
{
|
|
return css->id;
|
|
}
|
|
|
|
static int read_priomap(struct seq_file *sf, void *v)
|
|
{
|
|
struct net_device *dev;
|
|
|
|
rcu_read_lock();
|
|
for_each_netdev_rcu(&init_net, dev)
|
|
seq_printf(sf, "%s %u\n", dev->name,
|
|
netprio_prio(seq_css(sf), dev));
|
|
rcu_read_unlock();
|
|
return 0;
|
|
}
|
|
|
|
static ssize_t write_priomap(struct kernfs_open_file *of,
|
|
char *buf, size_t nbytes, loff_t off)
|
|
{
|
|
char devname[IFNAMSIZ + 1];
|
|
struct net_device *dev;
|
|
u32 prio;
|
|
int ret;
|
|
|
|
if (sscanf(buf, "%"__stringify(IFNAMSIZ)"s %u", devname, &prio) != 2)
|
|
return -EINVAL;
|
|
|
|
dev = dev_get_by_name(&init_net, devname);
|
|
if (!dev)
|
|
return -ENODEV;
|
|
|
|
rtnl_lock();
|
|
|
|
ret = netprio_set_prio(of_css(of), dev, prio);
|
|
|
|
rtnl_unlock();
|
|
dev_put(dev);
|
|
return ret ?: nbytes;
|
|
}
|
|
|
|
static int update_netprio(const void *v, struct file *file, unsigned n)
|
|
{
|
|
struct socket *sock = sock_from_file(file);
|
|
|
|
if (sock)
|
|
sock_cgroup_set_prioidx(&sock->sk->sk_cgrp_data,
|
|
(unsigned long)v);
|
|
return 0;
|
|
}
|
|
|
|
static void net_prio_attach(struct cgroup_taskset *tset)
|
|
{
|
|
struct task_struct *p;
|
|
struct cgroup_subsys_state *css;
|
|
|
|
cgroup_taskset_for_each(p, css, tset) {
|
|
void *v = (void *)(unsigned long)css->id;
|
|
|
|
task_lock(p);
|
|
iterate_fd(p->files, 0, update_netprio, v);
|
|
task_unlock(p);
|
|
}
|
|
}
|
|
|
|
static struct cftype ss_files[] = {
|
|
{
|
|
.name = "prioidx",
|
|
.read_u64 = read_prioidx,
|
|
},
|
|
{
|
|
.name = "ifpriomap",
|
|
.seq_show = read_priomap,
|
|
.write = write_priomap,
|
|
},
|
|
{ } /* terminate */
|
|
};
|
|
|
|
struct cgroup_subsys net_prio_cgrp_subsys = {
|
|
.css_alloc = cgrp_css_alloc,
|
|
.css_online = cgrp_css_online,
|
|
.css_free = cgrp_css_free,
|
|
.attach = net_prio_attach,
|
|
.legacy_cftypes = ss_files,
|
|
};
|
|
|
|
static int netprio_device_event(struct notifier_block *unused,
|
|
unsigned long event, void *ptr)
|
|
{
|
|
struct net_device *dev = netdev_notifier_info_to_dev(ptr);
|
|
struct netprio_map *old;
|
|
|
|
/*
|
|
* Note this is called with rtnl_lock held so we have update side
|
|
* protection on our rcu assignments
|
|
*/
|
|
|
|
switch (event) {
|
|
case NETDEV_UNREGISTER:
|
|
old = rtnl_dereference(dev->priomap);
|
|
RCU_INIT_POINTER(dev->priomap, NULL);
|
|
if (old)
|
|
kfree_rcu(old, rcu);
|
|
break;
|
|
}
|
|
return NOTIFY_DONE;
|
|
}
|
|
|
|
static struct notifier_block netprio_device_notifier = {
|
|
.notifier_call = netprio_device_event
|
|
};
|
|
|
|
static int __init init_cgroup_netprio(void)
|
|
{
|
|
register_netdevice_notifier(&netprio_device_notifier);
|
|
return 0;
|
|
}
|
|
subsys_initcall(init_cgroup_netprio);
|