mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
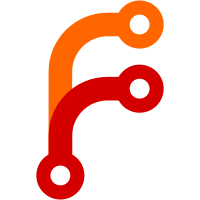
For EVPN non-DF (Designated Forwarder) filtering we need to be able to prevent decapsulated traffic from being flooded to a multi-homed host. Filtering of multicast and broadcast traffic can be achieved using the following flower filter: # tc filter add dev bond0 egress pref 1 proto all flower indev vxlan0 dst_mac 01:00:00:00:00:00/01:00:00:00:00:00 action drop Unlike broadcast and multicast traffic, it is not currently possible to filter unknown unicast traffic. The classification into unknown unicast is performed by the bridge driver, but is not visible to other layers such as tc. Solve this by adding a new 'l2_miss' bit to the tc skb extension. Clear the bit whenever a packet enters the bridge (received from a bridge port or transmitted via the bridge) and set it if the packet did not match an FDB or MDB entry. If there is no skb extension and the bit needs to be cleared, then do not allocate one as no extension is equivalent to the bit being cleared. The bit is not set for broadcast packets as they never perform a lookup and therefore never incur a miss. A bit that is set for every flooded packet would also work for the current use case, but it does not allow us to differentiate between registered and unregistered multicast traffic, which might be useful in the future. To keep the performance impact to a minimum, the marking of packets is guarded by the 'tc_skb_ext_tc' static key. When 'false', the skb is not touched and an skb extension is not allocated. Instead, only a 5 bytes nop is executed, as demonstrated below for the call site in br_handle_frame(). Before the patch: ``` memset(skb->cb, 0, sizeof(struct br_input_skb_cb)); c37b09: 49 c7 44 24 28 00 00 movq $0x0,0x28(%r12) c37b10: 00 00 p = br_port_get_rcu(skb->dev); c37b12: 49 8b 44 24 10 mov 0x10(%r12),%rax memset(skb->cb, 0, sizeof(struct br_input_skb_cb)); c37b17: 49 c7 44 24 30 00 00 movq $0x0,0x30(%r12) c37b1e: 00 00 c37b20: 49 c7 44 24 38 00 00 movq $0x0,0x38(%r12) c37b27: 00 00 ``` After the patch (when static key is disabled): ``` memset(skb->cb, 0, sizeof(struct br_input_skb_cb)); c37c29: 49 c7 44 24 28 00 00 movq $0x0,0x28(%r12) c37c30: 00 00 c37c32: 49 8d 44 24 28 lea 0x28(%r12),%rax c37c37: 48 c7 40 08 00 00 00 movq $0x0,0x8(%rax) c37c3e: 00 c37c3f: 48 c7 40 10 00 00 00 movq $0x0,0x10(%rax) c37c46: 00 #ifdef CONFIG_HAVE_JUMP_LABEL_HACK static __always_inline bool arch_static_branch(struct static_key *key, bool branch) { asm_volatile_goto("1:" c37c47: 0f 1f 44 00 00 nopl 0x0(%rax,%rax,1) br_tc_skb_miss_set(skb, false); p = br_port_get_rcu(skb->dev); c37c4c: 49 8b 44 24 10 mov 0x10(%r12),%rax ``` Subsequent patches will extend the flower classifier to be able to match on the new 'l2_miss' bit and enable / disable the static key when filters that match on it are added / deleted. Signed-off-by: Ido Schimmel <idosch@nvidia.com> Acked-by: Nikolay Aleksandrov <razor@blackwall.org> Acked-by: Jakub Kicinski <kuba@kernel.org> Signed-off-by: Jakub Kicinski <kuba@kernel.org>
460 lines
12 KiB
C
460 lines
12 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Handle incoming frames
|
|
* Linux ethernet bridge
|
|
*
|
|
* Authors:
|
|
* Lennert Buytenhek <buytenh@gnu.org>
|
|
*/
|
|
|
|
#include <linux/slab.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/etherdevice.h>
|
|
#include <linux/netfilter_bridge.h>
|
|
#ifdef CONFIG_NETFILTER_FAMILY_BRIDGE
|
|
#include <net/netfilter/nf_queue.h>
|
|
#endif
|
|
#include <linux/neighbour.h>
|
|
#include <net/arp.h>
|
|
#include <net/dsa.h>
|
|
#include <linux/export.h>
|
|
#include <linux/rculist.h>
|
|
#include "br_private.h"
|
|
#include "br_private_tunnel.h"
|
|
|
|
static int
|
|
br_netif_receive_skb(struct net *net, struct sock *sk, struct sk_buff *skb)
|
|
{
|
|
br_drop_fake_rtable(skb);
|
|
return netif_receive_skb(skb);
|
|
}
|
|
|
|
static int br_pass_frame_up(struct sk_buff *skb)
|
|
{
|
|
struct net_device *indev, *brdev = BR_INPUT_SKB_CB(skb)->brdev;
|
|
struct net_bridge *br = netdev_priv(brdev);
|
|
struct net_bridge_vlan_group *vg;
|
|
|
|
dev_sw_netstats_rx_add(brdev, skb->len);
|
|
|
|
vg = br_vlan_group_rcu(br);
|
|
|
|
/* Reset the offload_fwd_mark because there could be a stacked
|
|
* bridge above, and it should not think this bridge it doing
|
|
* that bridge's work forwarding out its ports.
|
|
*/
|
|
br_switchdev_frame_unmark(skb);
|
|
|
|
/* Bridge is just like any other port. Make sure the
|
|
* packet is allowed except in promisc mode when someone
|
|
* may be running packet capture.
|
|
*/
|
|
if (!(brdev->flags & IFF_PROMISC) &&
|
|
!br_allowed_egress(vg, skb)) {
|
|
kfree_skb(skb);
|
|
return NET_RX_DROP;
|
|
}
|
|
|
|
indev = skb->dev;
|
|
skb->dev = brdev;
|
|
skb = br_handle_vlan(br, NULL, vg, skb);
|
|
if (!skb)
|
|
return NET_RX_DROP;
|
|
/* update the multicast stats if the packet is IGMP/MLD */
|
|
br_multicast_count(br, NULL, skb, br_multicast_igmp_type(skb),
|
|
BR_MCAST_DIR_TX);
|
|
|
|
return NF_HOOK(NFPROTO_BRIDGE, NF_BR_LOCAL_IN,
|
|
dev_net(indev), NULL, skb, indev, NULL,
|
|
br_netif_receive_skb);
|
|
}
|
|
|
|
/* note: already called with rcu_read_lock */
|
|
int br_handle_frame_finish(struct net *net, struct sock *sk, struct sk_buff *skb)
|
|
{
|
|
struct net_bridge_port *p = br_port_get_rcu(skb->dev);
|
|
enum br_pkt_type pkt_type = BR_PKT_UNICAST;
|
|
struct net_bridge_fdb_entry *dst = NULL;
|
|
struct net_bridge_mcast_port *pmctx;
|
|
struct net_bridge_mdb_entry *mdst;
|
|
bool local_rcv, mcast_hit = false;
|
|
struct net_bridge_mcast *brmctx;
|
|
struct net_bridge_vlan *vlan;
|
|
struct net_bridge *br;
|
|
u16 vid = 0;
|
|
u8 state;
|
|
|
|
if (!p)
|
|
goto drop;
|
|
|
|
br = p->br;
|
|
|
|
if (br_mst_is_enabled(br)) {
|
|
state = BR_STATE_FORWARDING;
|
|
} else {
|
|
if (p->state == BR_STATE_DISABLED)
|
|
goto drop;
|
|
|
|
state = p->state;
|
|
}
|
|
|
|
brmctx = &p->br->multicast_ctx;
|
|
pmctx = &p->multicast_ctx;
|
|
if (!br_allowed_ingress(p->br, nbp_vlan_group_rcu(p), skb, &vid,
|
|
&state, &vlan))
|
|
goto out;
|
|
|
|
if (p->flags & BR_PORT_LOCKED) {
|
|
struct net_bridge_fdb_entry *fdb_src =
|
|
br_fdb_find_rcu(br, eth_hdr(skb)->h_source, vid);
|
|
|
|
if (!fdb_src) {
|
|
/* FDB miss. Create locked FDB entry if MAB is enabled
|
|
* and drop the packet.
|
|
*/
|
|
if (p->flags & BR_PORT_MAB)
|
|
br_fdb_update(br, p, eth_hdr(skb)->h_source,
|
|
vid, BIT(BR_FDB_LOCKED));
|
|
goto drop;
|
|
} else if (READ_ONCE(fdb_src->dst) != p ||
|
|
test_bit(BR_FDB_LOCAL, &fdb_src->flags)) {
|
|
/* FDB mismatch. Drop the packet without roaming. */
|
|
goto drop;
|
|
} else if (test_bit(BR_FDB_LOCKED, &fdb_src->flags)) {
|
|
/* FDB match, but entry is locked. Refresh it and drop
|
|
* the packet.
|
|
*/
|
|
br_fdb_update(br, p, eth_hdr(skb)->h_source, vid,
|
|
BIT(BR_FDB_LOCKED));
|
|
goto drop;
|
|
}
|
|
}
|
|
|
|
nbp_switchdev_frame_mark(p, skb);
|
|
|
|
/* insert into forwarding database after filtering to avoid spoofing */
|
|
if (p->flags & BR_LEARNING)
|
|
br_fdb_update(br, p, eth_hdr(skb)->h_source, vid, 0);
|
|
|
|
local_rcv = !!(br->dev->flags & IFF_PROMISC);
|
|
if (is_multicast_ether_addr(eth_hdr(skb)->h_dest)) {
|
|
/* by definition the broadcast is also a multicast address */
|
|
if (is_broadcast_ether_addr(eth_hdr(skb)->h_dest)) {
|
|
pkt_type = BR_PKT_BROADCAST;
|
|
local_rcv = true;
|
|
} else {
|
|
pkt_type = BR_PKT_MULTICAST;
|
|
if (br_multicast_rcv(&brmctx, &pmctx, vlan, skb, vid))
|
|
goto drop;
|
|
}
|
|
}
|
|
|
|
if (state == BR_STATE_LEARNING)
|
|
goto drop;
|
|
|
|
BR_INPUT_SKB_CB(skb)->brdev = br->dev;
|
|
BR_INPUT_SKB_CB(skb)->src_port_isolated = !!(p->flags & BR_ISOLATED);
|
|
|
|
if (IS_ENABLED(CONFIG_INET) &&
|
|
(skb->protocol == htons(ETH_P_ARP) ||
|
|
skb->protocol == htons(ETH_P_RARP))) {
|
|
br_do_proxy_suppress_arp(skb, br, vid, p);
|
|
} else if (IS_ENABLED(CONFIG_IPV6) &&
|
|
skb->protocol == htons(ETH_P_IPV6) &&
|
|
br_opt_get(br, BROPT_NEIGH_SUPPRESS_ENABLED) &&
|
|
pskb_may_pull(skb, sizeof(struct ipv6hdr) +
|
|
sizeof(struct nd_msg)) &&
|
|
ipv6_hdr(skb)->nexthdr == IPPROTO_ICMPV6) {
|
|
struct nd_msg *msg, _msg;
|
|
|
|
msg = br_is_nd_neigh_msg(skb, &_msg);
|
|
if (msg)
|
|
br_do_suppress_nd(skb, br, vid, p, msg);
|
|
}
|
|
|
|
switch (pkt_type) {
|
|
case BR_PKT_MULTICAST:
|
|
mdst = br_mdb_get(brmctx, skb, vid);
|
|
if ((mdst || BR_INPUT_SKB_CB_MROUTERS_ONLY(skb)) &&
|
|
br_multicast_querier_exists(brmctx, eth_hdr(skb), mdst)) {
|
|
if ((mdst && mdst->host_joined) ||
|
|
br_multicast_is_router(brmctx, skb)) {
|
|
local_rcv = true;
|
|
br->dev->stats.multicast++;
|
|
}
|
|
mcast_hit = true;
|
|
} else {
|
|
local_rcv = true;
|
|
br->dev->stats.multicast++;
|
|
}
|
|
break;
|
|
case BR_PKT_UNICAST:
|
|
dst = br_fdb_find_rcu(br, eth_hdr(skb)->h_dest, vid);
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
|
|
if (dst) {
|
|
unsigned long now = jiffies;
|
|
|
|
if (test_bit(BR_FDB_LOCAL, &dst->flags))
|
|
return br_pass_frame_up(skb);
|
|
|
|
if (now != dst->used)
|
|
dst->used = now;
|
|
br_forward(dst->dst, skb, local_rcv, false);
|
|
} else {
|
|
if (!mcast_hit)
|
|
br_flood(br, skb, pkt_type, local_rcv, false, vid);
|
|
else
|
|
br_multicast_flood(mdst, skb, brmctx, local_rcv, false);
|
|
}
|
|
|
|
if (local_rcv)
|
|
return br_pass_frame_up(skb);
|
|
|
|
out:
|
|
return 0;
|
|
drop:
|
|
kfree_skb(skb);
|
|
goto out;
|
|
}
|
|
EXPORT_SYMBOL_GPL(br_handle_frame_finish);
|
|
|
|
static void __br_handle_local_finish(struct sk_buff *skb)
|
|
{
|
|
struct net_bridge_port *p = br_port_get_rcu(skb->dev);
|
|
u16 vid = 0;
|
|
|
|
/* check if vlan is allowed, to avoid spoofing */
|
|
if ((p->flags & BR_LEARNING) &&
|
|
nbp_state_should_learn(p) &&
|
|
!br_opt_get(p->br, BROPT_NO_LL_LEARN) &&
|
|
br_should_learn(p, skb, &vid))
|
|
br_fdb_update(p->br, p, eth_hdr(skb)->h_source, vid, 0);
|
|
}
|
|
|
|
/* note: already called with rcu_read_lock */
|
|
static int br_handle_local_finish(struct net *net, struct sock *sk, struct sk_buff *skb)
|
|
{
|
|
__br_handle_local_finish(skb);
|
|
|
|
/* return 1 to signal the okfn() was called so it's ok to use the skb */
|
|
return 1;
|
|
}
|
|
|
|
static int nf_hook_bridge_pre(struct sk_buff *skb, struct sk_buff **pskb)
|
|
{
|
|
#ifdef CONFIG_NETFILTER_FAMILY_BRIDGE
|
|
struct nf_hook_entries *e = NULL;
|
|
struct nf_hook_state state;
|
|
unsigned int verdict, i;
|
|
struct net *net;
|
|
int ret;
|
|
|
|
net = dev_net(skb->dev);
|
|
#ifdef HAVE_JUMP_LABEL
|
|
if (!static_key_false(&nf_hooks_needed[NFPROTO_BRIDGE][NF_BR_PRE_ROUTING]))
|
|
goto frame_finish;
|
|
#endif
|
|
|
|
e = rcu_dereference(net->nf.hooks_bridge[NF_BR_PRE_ROUTING]);
|
|
if (!e)
|
|
goto frame_finish;
|
|
|
|
nf_hook_state_init(&state, NF_BR_PRE_ROUTING,
|
|
NFPROTO_BRIDGE, skb->dev, NULL, NULL,
|
|
net, br_handle_frame_finish);
|
|
|
|
for (i = 0; i < e->num_hook_entries; i++) {
|
|
verdict = nf_hook_entry_hookfn(&e->hooks[i], skb, &state);
|
|
switch (verdict & NF_VERDICT_MASK) {
|
|
case NF_ACCEPT:
|
|
if (BR_INPUT_SKB_CB(skb)->br_netfilter_broute) {
|
|
*pskb = skb;
|
|
return RX_HANDLER_PASS;
|
|
}
|
|
break;
|
|
case NF_DROP:
|
|
kfree_skb(skb);
|
|
return RX_HANDLER_CONSUMED;
|
|
case NF_QUEUE:
|
|
ret = nf_queue(skb, &state, i, verdict);
|
|
if (ret == 1)
|
|
continue;
|
|
return RX_HANDLER_CONSUMED;
|
|
default: /* STOLEN */
|
|
return RX_HANDLER_CONSUMED;
|
|
}
|
|
}
|
|
frame_finish:
|
|
net = dev_net(skb->dev);
|
|
br_handle_frame_finish(net, NULL, skb);
|
|
#else
|
|
br_handle_frame_finish(dev_net(skb->dev), NULL, skb);
|
|
#endif
|
|
return RX_HANDLER_CONSUMED;
|
|
}
|
|
|
|
/* Return 0 if the frame was not processed otherwise 1
|
|
* note: already called with rcu_read_lock
|
|
*/
|
|
static int br_process_frame_type(struct net_bridge_port *p,
|
|
struct sk_buff *skb)
|
|
{
|
|
struct br_frame_type *tmp;
|
|
|
|
hlist_for_each_entry_rcu(tmp, &p->br->frame_type_list, list)
|
|
if (unlikely(tmp->type == skb->protocol))
|
|
return tmp->frame_handler(p, skb);
|
|
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* Return NULL if skb is handled
|
|
* note: already called with rcu_read_lock
|
|
*/
|
|
static rx_handler_result_t br_handle_frame(struct sk_buff **pskb)
|
|
{
|
|
struct net_bridge_port *p;
|
|
struct sk_buff *skb = *pskb;
|
|
const unsigned char *dest = eth_hdr(skb)->h_dest;
|
|
|
|
if (unlikely(skb->pkt_type == PACKET_LOOPBACK))
|
|
return RX_HANDLER_PASS;
|
|
|
|
if (!is_valid_ether_addr(eth_hdr(skb)->h_source))
|
|
goto drop;
|
|
|
|
skb = skb_share_check(skb, GFP_ATOMIC);
|
|
if (!skb)
|
|
return RX_HANDLER_CONSUMED;
|
|
|
|
memset(skb->cb, 0, sizeof(struct br_input_skb_cb));
|
|
br_tc_skb_miss_set(skb, false);
|
|
|
|
p = br_port_get_rcu(skb->dev);
|
|
if (p->flags & BR_VLAN_TUNNEL)
|
|
br_handle_ingress_vlan_tunnel(skb, p, nbp_vlan_group_rcu(p));
|
|
|
|
if (unlikely(is_link_local_ether_addr(dest))) {
|
|
u16 fwd_mask = p->br->group_fwd_mask_required;
|
|
|
|
/*
|
|
* See IEEE 802.1D Table 7-10 Reserved addresses
|
|
*
|
|
* Assignment Value
|
|
* Bridge Group Address 01-80-C2-00-00-00
|
|
* (MAC Control) 802.3 01-80-C2-00-00-01
|
|
* (Link Aggregation) 802.3 01-80-C2-00-00-02
|
|
* 802.1X PAE address 01-80-C2-00-00-03
|
|
*
|
|
* 802.1AB LLDP 01-80-C2-00-00-0E
|
|
*
|
|
* Others reserved for future standardization
|
|
*/
|
|
fwd_mask |= p->group_fwd_mask;
|
|
switch (dest[5]) {
|
|
case 0x00: /* Bridge Group Address */
|
|
/* If STP is turned off,
|
|
then must forward to keep loop detection */
|
|
if (p->br->stp_enabled == BR_NO_STP ||
|
|
fwd_mask & (1u << dest[5]))
|
|
goto forward;
|
|
*pskb = skb;
|
|
__br_handle_local_finish(skb);
|
|
return RX_HANDLER_PASS;
|
|
|
|
case 0x01: /* IEEE MAC (Pause) */
|
|
goto drop;
|
|
|
|
case 0x0E: /* 802.1AB LLDP */
|
|
fwd_mask |= p->br->group_fwd_mask;
|
|
if (fwd_mask & (1u << dest[5]))
|
|
goto forward;
|
|
*pskb = skb;
|
|
__br_handle_local_finish(skb);
|
|
return RX_HANDLER_PASS;
|
|
|
|
default:
|
|
/* Allow selective forwarding for most other protocols */
|
|
fwd_mask |= p->br->group_fwd_mask;
|
|
if (fwd_mask & (1u << dest[5]))
|
|
goto forward;
|
|
}
|
|
|
|
/* The else clause should be hit when nf_hook():
|
|
* - returns < 0 (drop/error)
|
|
* - returns = 0 (stolen/nf_queue)
|
|
* Thus return 1 from the okfn() to signal the skb is ok to pass
|
|
*/
|
|
if (NF_HOOK(NFPROTO_BRIDGE, NF_BR_LOCAL_IN,
|
|
dev_net(skb->dev), NULL, skb, skb->dev, NULL,
|
|
br_handle_local_finish) == 1) {
|
|
return RX_HANDLER_PASS;
|
|
} else {
|
|
return RX_HANDLER_CONSUMED;
|
|
}
|
|
}
|
|
|
|
if (unlikely(br_process_frame_type(p, skb)))
|
|
return RX_HANDLER_PASS;
|
|
|
|
forward:
|
|
if (br_mst_is_enabled(p->br))
|
|
goto defer_stp_filtering;
|
|
|
|
switch (p->state) {
|
|
case BR_STATE_FORWARDING:
|
|
case BR_STATE_LEARNING:
|
|
defer_stp_filtering:
|
|
if (ether_addr_equal(p->br->dev->dev_addr, dest))
|
|
skb->pkt_type = PACKET_HOST;
|
|
|
|
return nf_hook_bridge_pre(skb, pskb);
|
|
default:
|
|
drop:
|
|
kfree_skb(skb);
|
|
}
|
|
return RX_HANDLER_CONSUMED;
|
|
}
|
|
|
|
/* This function has no purpose other than to appease the br_port_get_rcu/rtnl
|
|
* helpers which identify bridged ports according to the rx_handler installed
|
|
* on them (so there _needs_ to be a bridge rx_handler even if we don't need it
|
|
* to do anything useful). This bridge won't support traffic to/from the stack,
|
|
* but only hardware bridging. So return RX_HANDLER_PASS so we don't steal
|
|
* frames from the ETH_P_XDSA packet_type handler.
|
|
*/
|
|
static rx_handler_result_t br_handle_frame_dummy(struct sk_buff **pskb)
|
|
{
|
|
return RX_HANDLER_PASS;
|
|
}
|
|
|
|
rx_handler_func_t *br_get_rx_handler(const struct net_device *dev)
|
|
{
|
|
if (netdev_uses_dsa(dev))
|
|
return br_handle_frame_dummy;
|
|
|
|
return br_handle_frame;
|
|
}
|
|
|
|
void br_add_frame(struct net_bridge *br, struct br_frame_type *ft)
|
|
{
|
|
hlist_add_head_rcu(&ft->list, &br->frame_type_list);
|
|
}
|
|
|
|
void br_del_frame(struct net_bridge *br, struct br_frame_type *ft)
|
|
{
|
|
struct br_frame_type *tmp;
|
|
|
|
hlist_for_each_entry(tmp, &br->frame_type_list, list)
|
|
if (ft == tmp) {
|
|
hlist_del_rcu(&ft->list);
|
|
return;
|
|
}
|
|
}
|