mirror of
https://github.com/torvalds/linux
synced 2024-07-22 11:10:46 +00:00
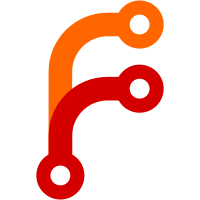
There is a discussion about the name of hugetlb_vmemmap_alloc/free in thread [1]. The suggestion suggested by David is rename "alloc/free" to "optimize/restore" to make functionalities clearer to users, "optimize" means the function will optimize vmemmap pages, while "restore" means restoring its vmemmap pages discared before. This commit does this. Another discussion is the confusion RESERVE_VMEMMAP_NR isn't used explicitly for vmemmap_addr but implicitly for vmemmap_end in hugetlb_vmemmap_alloc/free. David suggested we can compute what hugetlb_vmemmap_init() does now at runtime. We do not need to worry for the overhead of computing at runtime since the calculation is simple enough and those functions are not in a hot path. This commit has the following improvements: 1) The function suffixed name ("optimize/restore") is more expressive. 2) The logic becomes less weird in hugetlb_vmemmap_optimize/restore(). 3) The hugetlb_vmemmap_init() does not need to be exported anymore. 4) A ->optimize_vmemmap_pages field in struct hstate is killed. 5) There is only one place where checks is_power_of_2(sizeof(struct page)) instead of two places. 6) Add more comments for hugetlb_vmemmap_optimize/restore(). 7) For external users, hugetlb_optimize_vmemmap_pages() is used for detecting if the HugeTLB's vmemmap pages is optimizable originally. In this commit, it is killed and we introduce a new helper hugetlb_vmemmap_optimizable() to replace it. The name is more expressive. Link: https://lore.kernel.org/all/20220404074652.68024-2-songmuchun@bytedance.com/ [1] Link: https://lkml.kernel.org/r/20220628092235.91270-7-songmuchun@bytedance.com Signed-off-by: Muchun Song <songmuchun@bytedance.com> Reviewed-by: Mike Kravetz <mike.kravetz@oracle.com> Cc: Anshuman Khandual <anshuman.khandual@arm.com> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: David Hildenbrand <david@redhat.com> Cc: Jonathan Corbet <corbet@lwn.net> Cc: Oscar Salvador <osalvador@suse.de> Cc: Will Deacon <will@kernel.org> Cc: Xiongchun Duan <duanxiongchun@bytedance.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
61 lines
1.6 KiB
C
61 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* HugeTLB Vmemmap Optimization (HVO)
|
|
*
|
|
* Copyright (c) 2020, ByteDance. All rights reserved.
|
|
*
|
|
* Author: Muchun Song <songmuchun@bytedance.com>
|
|
*/
|
|
#ifndef _LINUX_HUGETLB_VMEMMAP_H
|
|
#define _LINUX_HUGETLB_VMEMMAP_H
|
|
#include <linux/hugetlb.h>
|
|
|
|
#ifdef CONFIG_HUGETLB_PAGE_OPTIMIZE_VMEMMAP
|
|
int hugetlb_vmemmap_restore(const struct hstate *h, struct page *head);
|
|
void hugetlb_vmemmap_optimize(const struct hstate *h, struct page *head);
|
|
|
|
/*
|
|
* Reserve one vmemmap page, all vmemmap addresses are mapped to it. See
|
|
* Documentation/vm/vmemmap_dedup.rst.
|
|
*/
|
|
#define HUGETLB_VMEMMAP_RESERVE_SIZE PAGE_SIZE
|
|
|
|
static inline unsigned int hugetlb_vmemmap_size(const struct hstate *h)
|
|
{
|
|
return pages_per_huge_page(h) * sizeof(struct page);
|
|
}
|
|
|
|
/*
|
|
* Return how many vmemmap size associated with a HugeTLB page that can be
|
|
* optimized and can be freed to the buddy allocator.
|
|
*/
|
|
static inline unsigned int hugetlb_vmemmap_optimizable_size(const struct hstate *h)
|
|
{
|
|
int size = hugetlb_vmemmap_size(h) - HUGETLB_VMEMMAP_RESERVE_SIZE;
|
|
|
|
if (!is_power_of_2(sizeof(struct page)))
|
|
return 0;
|
|
return size > 0 ? size : 0;
|
|
}
|
|
#else
|
|
static inline int hugetlb_vmemmap_restore(const struct hstate *h, struct page *head)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline void hugetlb_vmemmap_optimize(const struct hstate *h, struct page *head)
|
|
{
|
|
}
|
|
|
|
static inline unsigned int hugetlb_vmemmap_optimizable_size(const struct hstate *h)
|
|
{
|
|
return 0;
|
|
}
|
|
#endif /* CONFIG_HUGETLB_PAGE_OPTIMIZE_VMEMMAP */
|
|
|
|
static inline bool hugetlb_vmemmap_optimizable(const struct hstate *h)
|
|
{
|
|
return hugetlb_vmemmap_optimizable_size(h) != 0;
|
|
}
|
|
#endif /* _LINUX_HUGETLB_VMEMMAP_H */
|