mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
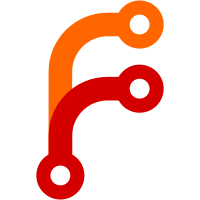
The DT of_device.h and of_platform.h date back to the separate of_platform_bus_type before it as merged into the regular platform bus. As part of that merge prepping Arm DT support 13 years ago, they "temporarily" include each other. They also include platform_device.h and of.h. As a result, there's a pretty much random mix of those include files used throughout the tree. In order to detangle these headers and replace the implicit includes with struct declarations, users need to explicitly include the correct includes. Signed-off-by: Rob Herring <robh@kernel.org> Reviewed-by: Andy Shevchenko <andy.shevchenko@gmail.com> Acked-by: Romain Perier <romain.perier@gmail.com> Signed-off-by: Bartosz Golaszewski <bartosz.golaszewski@linaro.org>
78 lines
1.9 KiB
C
78 lines
1.9 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* GPIO driver for the TS-4800 board
|
|
*
|
|
* Copyright (c) 2016 - Savoir-faire Linux
|
|
*/
|
|
|
|
#include <linux/gpio/driver.h>
|
|
#include <linux/module.h>
|
|
#include <linux/of.h>
|
|
#include <linux/platform_device.h>
|
|
|
|
#define DEFAULT_PIN_NUMBER 16
|
|
#define INPUT_REG_OFFSET 0x00
|
|
#define OUTPUT_REG_OFFSET 0x02
|
|
#define DIRECTION_REG_OFFSET 0x04
|
|
|
|
static int ts4800_gpio_probe(struct platform_device *pdev)
|
|
{
|
|
struct device_node *node;
|
|
struct gpio_chip *chip;
|
|
void __iomem *base_addr;
|
|
int retval;
|
|
u32 ngpios;
|
|
|
|
chip = devm_kzalloc(&pdev->dev, sizeof(struct gpio_chip), GFP_KERNEL);
|
|
if (!chip)
|
|
return -ENOMEM;
|
|
|
|
base_addr = devm_platform_ioremap_resource(pdev, 0);
|
|
if (IS_ERR(base_addr))
|
|
return PTR_ERR(base_addr);
|
|
|
|
node = pdev->dev.of_node;
|
|
if (!node)
|
|
return -EINVAL;
|
|
|
|
retval = of_property_read_u32(node, "ngpios", &ngpios);
|
|
if (retval == -EINVAL)
|
|
ngpios = DEFAULT_PIN_NUMBER;
|
|
else if (retval)
|
|
return retval;
|
|
|
|
retval = bgpio_init(chip, &pdev->dev, 2, base_addr + INPUT_REG_OFFSET,
|
|
base_addr + OUTPUT_REG_OFFSET, NULL,
|
|
base_addr + DIRECTION_REG_OFFSET, NULL, 0);
|
|
if (retval) {
|
|
dev_err(&pdev->dev, "bgpio_init failed\n");
|
|
return retval;
|
|
}
|
|
|
|
chip->ngpio = ngpios;
|
|
|
|
platform_set_drvdata(pdev, chip);
|
|
|
|
return devm_gpiochip_add_data(&pdev->dev, chip, NULL);
|
|
}
|
|
|
|
static const struct of_device_id ts4800_gpio_of_match[] = {
|
|
{ .compatible = "technologic,ts4800-gpio", },
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(of, ts4800_gpio_of_match);
|
|
|
|
static struct platform_driver ts4800_gpio_driver = {
|
|
.driver = {
|
|
.name = "ts4800-gpio",
|
|
.of_match_table = ts4800_gpio_of_match,
|
|
},
|
|
.probe = ts4800_gpio_probe,
|
|
};
|
|
|
|
module_platform_driver_probe(ts4800_gpio_driver, ts4800_gpio_probe);
|
|
|
|
MODULE_AUTHOR("Julien Grossholtz <julien.grossholtz@savoirfairelinux.com>");
|
|
MODULE_DESCRIPTION("TS4800 FPGA GPIO driver");
|
|
MODULE_LICENSE("GPL v2");
|