mirror of
https://github.com/torvalds/linux
synced 2024-07-21 18:51:47 +00:00
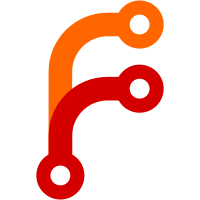
Since commit6c40624930
("bootconfig: Increase max nodes of bootconfig from 1024 to 8192 for DCC support") increased the max number of bootconfig node to 8192, the bootconfig testcase of the max number of nodes fails. To fix this issue, we can not simply increase the number in the test script because the test bootconfig file becomes too big (>32KB). To fix that, we can use a combination of three alphabets (26^3 = 17576). But with that, we can not express the 8193 (just one exceed from the limitation) because it also exceeds the max size of bootconfig. So, the first 26 nodes will just use one alphabet. With this fix, test-bootconfig.sh passes all tests. Link: https://lore.kernel.org/all/167888844790.791176.670805252426835131.stgit@devnote2/ Reported-by: Heinz Wiesinger <pprkut@slackware.com> Link: https://lore.kernel.org/all/2463802.XAFRqVoOGU@amaterasu.liwjatan.org Fixes:6c40624930
("bootconfig: Increase max nodes of bootconfig from 1024 to 8192 for DCC support") Signed-off-by: Masami Hiramatsu (Google) <mhiramat@kernel.org> Reviewed-by: Steven Rostedt (Google) <rostedt@goodmis.org>
196 lines
4 KiB
Bash
Executable file
196 lines
4 KiB
Bash
Executable file
#!/bin/sh
|
|
# SPDX-License-Identifier: GPL-2.0-only
|
|
|
|
echo "Boot config test script"
|
|
|
|
if [ -d "$1" ]; then
|
|
TESTDIR=$1
|
|
else
|
|
TESTDIR=.
|
|
fi
|
|
BOOTCONF=${TESTDIR}/bootconfig
|
|
ALIGN=4
|
|
|
|
INITRD=`mktemp ${TESTDIR}/initrd-XXXX`
|
|
TEMPCONF=`mktemp ${TESTDIR}/temp-XXXX.bconf`
|
|
OUTFILE=`mktemp ${TESTDIR}/tempout-XXXX`
|
|
NG=0
|
|
|
|
cleanup() {
|
|
rm -f $INITRD $TEMPCONF $OUTFILE
|
|
exit $NG
|
|
}
|
|
|
|
trap cleanup EXIT TERM
|
|
|
|
NO=1
|
|
|
|
xpass() { # pass test command
|
|
echo "test case $NO ($*)... "
|
|
if ! ($@ && echo "\t\t[OK]"); then
|
|
echo "\t\t[NG]"; NG=$((NG + 1))
|
|
fi
|
|
NO=$((NO + 1))
|
|
}
|
|
|
|
xfail() { # fail test command
|
|
echo "test case $NO ($*)... "
|
|
if ! (! $@ && echo "\t\t[OK]"); then
|
|
echo "\t\t[NG]"; NG=$((NG + 1))
|
|
fi
|
|
NO=$((NO + 1))
|
|
}
|
|
|
|
echo "Basic command test"
|
|
xpass $BOOTCONF $INITRD
|
|
|
|
echo "Delete command should success without bootconfig"
|
|
xpass $BOOTCONF -d $INITRD
|
|
|
|
dd if=/dev/zero of=$INITRD bs=4096 count=1
|
|
echo "key = value;" > $TEMPCONF
|
|
bconf_size=$(stat -c %s $TEMPCONF)
|
|
initrd_size=$(stat -c %s $INITRD)
|
|
|
|
echo "Apply command test"
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
new_size=$(stat -c %s $INITRD)
|
|
|
|
echo "Show command test"
|
|
xpass $BOOTCONF $INITRD
|
|
|
|
echo "File size check"
|
|
total_size=$(expr $bconf_size + $initrd_size + 9 + 12 + $ALIGN - 1 )
|
|
total_size=$(expr $total_size / $ALIGN)
|
|
total_size=$(expr $total_size \* $ALIGN)
|
|
xpass test $new_size -eq $total_size
|
|
|
|
echo "Apply command repeat test"
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
|
|
echo "File size check"
|
|
xpass test $new_size -eq $(stat -c %s $INITRD)
|
|
|
|
echo "Delete command check"
|
|
xpass $BOOTCONF -d $INITRD
|
|
|
|
echo "File size check"
|
|
new_size=$(stat -c %s $INITRD)
|
|
xpass test $new_size -eq $initrd_size
|
|
|
|
echo "No error messge while applying"
|
|
dd if=/dev/zero of=$INITRD bs=4096 count=1
|
|
printf " \0\0\0 \0\0\0" >> $INITRD
|
|
$BOOTCONF -a $TEMPCONF $INITRD > $OUTFILE 2>&1
|
|
xfail grep -i "failed" $OUTFILE
|
|
xfail grep -i "error" $OUTFILE
|
|
|
|
echo "Max node number check"
|
|
|
|
awk '
|
|
BEGIN {
|
|
for (i = 0; i < 26; i += 1)
|
|
printf("%c\n", 65 + i % 26)
|
|
for (i = 26; i < 8192; i += 1)
|
|
printf("%c%c%c\n", 65 + i % 26, 65 + (i / 26) % 26, 65 + (i / 26 / 26))
|
|
}
|
|
' > $TEMPCONF
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
|
|
echo "badnode" >> $TEMPCONF
|
|
xfail $BOOTCONF -a $TEMPCONF $INITRD
|
|
|
|
echo "Max filesize check"
|
|
|
|
# Max size is 32767 (including terminal byte)
|
|
echo -n "data = \"" > $TEMPCONF
|
|
dd if=/dev/urandom bs=768 count=32 | base64 -w0 >> $TEMPCONF
|
|
echo "\"" >> $TEMPCONF
|
|
xfail $BOOTCONF -a $TEMPCONF $INITRD
|
|
|
|
truncate -s 32764 $TEMPCONF
|
|
echo "\"" >> $TEMPCONF # add 2 bytes + terminal ('\"\n\0')
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
|
|
echo "Adding same-key values"
|
|
cat > $TEMPCONF << EOF
|
|
key = bar, baz
|
|
key += qux
|
|
EOF
|
|
echo > $INITRD
|
|
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
$BOOTCONF $INITRD > $OUTFILE
|
|
xpass grep -q "bar" $OUTFILE
|
|
xpass grep -q "baz" $OUTFILE
|
|
xpass grep -q "qux" $OUTFILE
|
|
|
|
echo "Override same-key values"
|
|
cat > $TEMPCONF << EOF
|
|
key = bar, baz
|
|
key := qux
|
|
EOF
|
|
echo > $INITRD
|
|
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
$BOOTCONF $INITRD > $OUTFILE
|
|
xfail grep -q "bar" $OUTFILE
|
|
xfail grep -q "baz" $OUTFILE
|
|
xpass grep -q "qux" $OUTFILE
|
|
|
|
echo "Double/single quotes test"
|
|
echo "key = '\"string\"';" > $TEMPCONF
|
|
$BOOTCONF -a $TEMPCONF $INITRD
|
|
$BOOTCONF $INITRD > $TEMPCONF
|
|
cat $TEMPCONF
|
|
xpass grep \'\"string\"\' $TEMPCONF
|
|
|
|
echo "Repeat same-key tree"
|
|
cat > $TEMPCONF << EOF
|
|
foo
|
|
bar
|
|
foo { buz }
|
|
EOF
|
|
echo > $INITRD
|
|
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
$BOOTCONF $INITRD > $OUTFILE
|
|
xpass grep -q "bar" $OUTFILE
|
|
|
|
|
|
echo "Remove/keep tailing spaces"
|
|
cat > $TEMPCONF << EOF
|
|
foo = val # comment
|
|
bar = "val2 " # comment
|
|
EOF
|
|
echo > $INITRD
|
|
|
|
xpass $BOOTCONF -a $TEMPCONF $INITRD
|
|
$BOOTCONF $INITRD > $OUTFILE
|
|
xfail grep -q val[[:space:]] $OUTFILE
|
|
xpass grep -q val2[[:space:]] $OUTFILE
|
|
|
|
echo "=== expected failure cases ==="
|
|
for i in samples/bad-* ; do
|
|
xfail $BOOTCONF -a $i $INITRD
|
|
done
|
|
|
|
echo "=== expected success cases ==="
|
|
for i in samples/good-* ; do
|
|
xpass $BOOTCONF -a $i $INITRD
|
|
done
|
|
|
|
|
|
echo
|
|
echo "=== Summary ==="
|
|
echo "# of Passed: $(expr $NO - $NG - 1)"
|
|
echo "# of Failed: $NG"
|
|
|
|
echo
|
|
if [ $NG -eq 0 ]; then
|
|
echo "All tests passed"
|
|
else
|
|
echo "$NG tests failed"
|
|
exit 1
|
|
fi
|