mirror of
https://github.com/torvalds/linux
synced 2024-07-22 19:21:07 +00:00
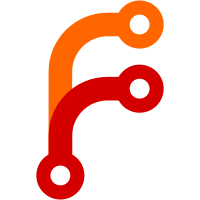
struct worker was laid out with the intent that all fields that are modified for each work item execution are in the first cacheline. However, this hasn't been true for a while with the addition of ->last_func. Let's just collect hot fields together at the top. Move ->sleeping in the hole after ->current_color and move ->lst_func right below. While at it, drop the cacheline comment which isn't useful anymore. Signed-off-by: Tejun Heo <tj@kernel.org> Cc: Lai Jiangshan <jiangshanlai@gmail.com>
81 lines
2.4 KiB
C
81 lines
2.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* kernel/workqueue_internal.h
|
|
*
|
|
* Workqueue internal header file. Only to be included by workqueue and
|
|
* core kernel subsystems.
|
|
*/
|
|
#ifndef _KERNEL_WORKQUEUE_INTERNAL_H
|
|
#define _KERNEL_WORKQUEUE_INTERNAL_H
|
|
|
|
#include <linux/workqueue.h>
|
|
#include <linux/kthread.h>
|
|
#include <linux/preempt.h>
|
|
|
|
struct worker_pool;
|
|
|
|
/*
|
|
* The poor guys doing the actual heavy lifting. All on-duty workers are
|
|
* either serving the manager role, on idle list or on busy hash. For
|
|
* details on the locking annotation (L, I, X...), refer to workqueue.c.
|
|
*
|
|
* Only to be used in workqueue and async.
|
|
*/
|
|
struct worker {
|
|
/* on idle list while idle, on busy hash table while busy */
|
|
union {
|
|
struct list_head entry; /* L: while idle */
|
|
struct hlist_node hentry; /* L: while busy */
|
|
};
|
|
|
|
struct work_struct *current_work; /* L: work being processed */
|
|
work_func_t current_func; /* L: current_work's fn */
|
|
struct pool_workqueue *current_pwq; /* L: current_work's pwq */
|
|
unsigned int current_color; /* L: current_work's color */
|
|
int sleeping; /* None */
|
|
|
|
/* used by the scheduler to determine a worker's last known identity */
|
|
work_func_t last_func; /* L: last work's fn */
|
|
|
|
struct list_head scheduled; /* L: scheduled works */
|
|
|
|
struct task_struct *task; /* I: worker task */
|
|
struct worker_pool *pool; /* A: the associated pool */
|
|
/* L: for rescuers */
|
|
struct list_head node; /* A: anchored at pool->workers */
|
|
/* A: runs through worker->node */
|
|
|
|
unsigned long last_active; /* L: last active timestamp */
|
|
unsigned int flags; /* X: flags */
|
|
int id; /* I: worker id */
|
|
|
|
/*
|
|
* Opaque string set with work_set_desc(). Printed out with task
|
|
* dump for debugging - WARN, BUG, panic or sysrq.
|
|
*/
|
|
char desc[WORKER_DESC_LEN];
|
|
|
|
/* used only by rescuers to point to the target workqueue */
|
|
struct workqueue_struct *rescue_wq; /* I: the workqueue to rescue */
|
|
};
|
|
|
|
/**
|
|
* current_wq_worker - return struct worker if %current is a workqueue worker
|
|
*/
|
|
static inline struct worker *current_wq_worker(void)
|
|
{
|
|
if (in_task() && (current->flags & PF_WQ_WORKER))
|
|
return kthread_data(current);
|
|
return NULL;
|
|
}
|
|
|
|
/*
|
|
* Scheduler hooks for concurrency managed workqueue. Only to be used from
|
|
* sched/ and workqueue.c.
|
|
*/
|
|
void wq_worker_running(struct task_struct *task);
|
|
void wq_worker_sleeping(struct task_struct *task);
|
|
work_func_t wq_worker_last_func(struct task_struct *task);
|
|
|
|
#endif /* _KERNEL_WORKQUEUE_INTERNAL_H */
|