mirror of
https://github.com/torvalds/linux
synced 2024-09-19 18:46:35 +00:00
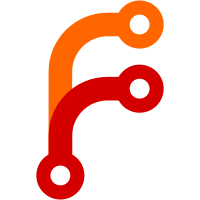
Move the pass1 commit code into the per-item source code files and use the dispatch function to call them. Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com> Reviewed-by: Chandan Babu R <chandanrlinux@gmail.com> Reviewed-by: Christoph Hellwig <hch@lst.de>
92 lines
2.2 KiB
C
92 lines
2.2 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2000-2006 Silicon Graphics, Inc.
|
|
* All Rights Reserved.
|
|
*/
|
|
#include "xfs.h"
|
|
#include "xfs_fs.h"
|
|
#include "xfs_shared.h"
|
|
#include "xfs_format.h"
|
|
#include "xfs_log_format.h"
|
|
#include "xfs_trans_resv.h"
|
|
#include "xfs_mount.h"
|
|
#include "xfs_inode.h"
|
|
#include "xfs_quota.h"
|
|
#include "xfs_trans.h"
|
|
#include "xfs_buf_item.h"
|
|
#include "xfs_trans_priv.h"
|
|
#include "xfs_qm.h"
|
|
#include "xfs_log.h"
|
|
#include "xfs_log_priv.h"
|
|
#include "xfs_log_recover.h"
|
|
|
|
STATIC void
|
|
xlog_recover_dquot_ra_pass2(
|
|
struct xlog *log,
|
|
struct xlog_recover_item *item)
|
|
{
|
|
struct xfs_mount *mp = log->l_mp;
|
|
struct xfs_disk_dquot *recddq;
|
|
struct xfs_dq_logformat *dq_f;
|
|
uint type;
|
|
|
|
if (mp->m_qflags == 0)
|
|
return;
|
|
|
|
recddq = item->ri_buf[1].i_addr;
|
|
if (recddq == NULL)
|
|
return;
|
|
if (item->ri_buf[1].i_len < sizeof(struct xfs_disk_dquot))
|
|
return;
|
|
|
|
type = recddq->d_flags & (XFS_DQ_USER | XFS_DQ_PROJ | XFS_DQ_GROUP);
|
|
ASSERT(type);
|
|
if (log->l_quotaoffs_flag & type)
|
|
return;
|
|
|
|
dq_f = item->ri_buf[0].i_addr;
|
|
ASSERT(dq_f);
|
|
ASSERT(dq_f->qlf_len == 1);
|
|
|
|
xlog_buf_readahead(log, dq_f->qlf_blkno,
|
|
XFS_FSB_TO_BB(mp, dq_f->qlf_len),
|
|
&xfs_dquot_buf_ra_ops);
|
|
}
|
|
|
|
const struct xlog_recover_item_ops xlog_dquot_item_ops = {
|
|
.item_type = XFS_LI_DQUOT,
|
|
.ra_pass2 = xlog_recover_dquot_ra_pass2,
|
|
};
|
|
|
|
/*
|
|
* Recover QUOTAOFF records. We simply make a note of it in the xlog
|
|
* structure, so that we know not to do any dquot item or dquot buffer recovery,
|
|
* of that type.
|
|
*/
|
|
STATIC int
|
|
xlog_recover_quotaoff_commit_pass1(
|
|
struct xlog *log,
|
|
struct xlog_recover_item *item)
|
|
{
|
|
struct xfs_qoff_logformat *qoff_f = item->ri_buf[0].i_addr;
|
|
ASSERT(qoff_f);
|
|
|
|
/*
|
|
* The logitem format's flag tells us if this was user quotaoff,
|
|
* group/project quotaoff or both.
|
|
*/
|
|
if (qoff_f->qf_flags & XFS_UQUOTA_ACCT)
|
|
log->l_quotaoffs_flag |= XFS_DQ_USER;
|
|
if (qoff_f->qf_flags & XFS_PQUOTA_ACCT)
|
|
log->l_quotaoffs_flag |= XFS_DQ_PROJ;
|
|
if (qoff_f->qf_flags & XFS_GQUOTA_ACCT)
|
|
log->l_quotaoffs_flag |= XFS_DQ_GROUP;
|
|
|
|
return 0;
|
|
}
|
|
|
|
const struct xlog_recover_item_ops xlog_quotaoff_item_ops = {
|
|
.item_type = XFS_LI_QUOTAOFF,
|
|
.commit_pass1 = xlog_recover_quotaoff_commit_pass1,
|
|
};
|