mirror of
https://github.com/torvalds/linux
synced 2024-09-24 05:17:36 +00:00
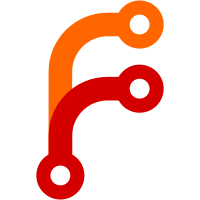
The current print_context_stack helper that does the stack walking job is good for usual stacktraces as it walks through all the stack and reports even addresses that look unreliable, which is nice when we don't have frame pointers for example. But we have users like perf that only require reliable stacktraces, and those may want a more adapted stack walker, so lets make this function a callback in stacktrace_ops that users can tune for their needs. Signed-off-by: Frederic Weisbecker <fweisbec@gmail.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Paul Mackerras <paulus@samba.org> LKML-Reference: <1261024834-5336-1-git-send-regression-fweisbec@gmail.com> Signed-off-by: Ingo Molnar <mingo@elte.hu>
92 lines
1.9 KiB
C
92 lines
1.9 KiB
C
/**
|
|
* @file backtrace.c
|
|
*
|
|
* @remark Copyright 2002 OProfile authors
|
|
* @remark Read the file COPYING
|
|
*
|
|
* @author John Levon
|
|
* @author David Smith
|
|
*/
|
|
|
|
#include <linux/oprofile.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/mm.h>
|
|
#include <asm/ptrace.h>
|
|
#include <asm/uaccess.h>
|
|
#include <asm/stacktrace.h>
|
|
|
|
static void backtrace_warning_symbol(void *data, char *msg,
|
|
unsigned long symbol)
|
|
{
|
|
/* Ignore warnings */
|
|
}
|
|
|
|
static void backtrace_warning(void *data, char *msg)
|
|
{
|
|
/* Ignore warnings */
|
|
}
|
|
|
|
static int backtrace_stack(void *data, char *name)
|
|
{
|
|
/* Yes, we want all stacks */
|
|
return 0;
|
|
}
|
|
|
|
static void backtrace_address(void *data, unsigned long addr, int reliable)
|
|
{
|
|
unsigned int *depth = data;
|
|
|
|
if ((*depth)--)
|
|
oprofile_add_trace(addr);
|
|
}
|
|
|
|
static struct stacktrace_ops backtrace_ops = {
|
|
.warning = backtrace_warning,
|
|
.warning_symbol = backtrace_warning_symbol,
|
|
.stack = backtrace_stack,
|
|
.address = backtrace_address,
|
|
.walk_stack = print_context_stack,
|
|
};
|
|
|
|
struct frame_head {
|
|
struct frame_head *bp;
|
|
unsigned long ret;
|
|
} __attribute__((packed));
|
|
|
|
static struct frame_head *dump_user_backtrace(struct frame_head *head)
|
|
{
|
|
struct frame_head bufhead[2];
|
|
|
|
/* Also check accessibility of one struct frame_head beyond */
|
|
if (!access_ok(VERIFY_READ, head, sizeof(bufhead)))
|
|
return NULL;
|
|
if (__copy_from_user_inatomic(bufhead, head, sizeof(bufhead)))
|
|
return NULL;
|
|
|
|
oprofile_add_trace(bufhead[0].ret);
|
|
|
|
/* frame pointers should strictly progress back up the stack
|
|
* (towards higher addresses) */
|
|
if (head >= bufhead[0].bp)
|
|
return NULL;
|
|
|
|
return bufhead[0].bp;
|
|
}
|
|
|
|
void
|
|
x86_backtrace(struct pt_regs * const regs, unsigned int depth)
|
|
{
|
|
struct frame_head *head = (struct frame_head *)frame_pointer(regs);
|
|
|
|
if (!user_mode_vm(regs)) {
|
|
unsigned long stack = kernel_stack_pointer(regs);
|
|
if (depth)
|
|
dump_trace(NULL, regs, (unsigned long *)stack, 0,
|
|
&backtrace_ops, &depth);
|
|
return;
|
|
}
|
|
|
|
while (depth-- && head)
|
|
head = dump_user_backtrace(head);
|
|
}
|