mirror of
https://github.com/torvalds/linux
synced 2024-10-01 08:50:11 +00:00
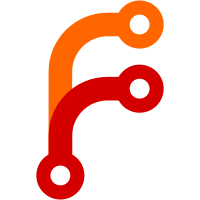
On Fireworks board module of Echo Audio, TSB43Cx43A (IceLynx Micro, iCEM) is used to process payload of isochronous packets. There's an public document of this chip[1]. This document is for firmware programmers to transfer/receive AMDTP with IEC60958 data format, however in clause 2.5, 2.6 and 2.7, we can see system design to utilize the sequence of value in SYT field of CIP header. In clause 2.3, we can see the specification of Audio Master Clock (MCLK) from iCEM. Well, this clock is actually not used for sampling clock. This can be confirmed when corresponding driver transfer random value as the sequence of SYT field. Even if in this case, the unit generates proper sound. Additionally, in unique command set for this board module, the format of CIP is changed; for IEC 61883-6 mode which we use, and for Windows Operating System. In the latter mode, the whole 32 bit field in second CIP header from Windows driver is used to represent counter of packets (NO-DATA code is still used for packets without data blocks). If the master clock was physically used by DSP on the board module, the Windows driver must have transferred correct sequence of SYT field. Furthermore, as long as seeing capacities of AudioFire2, AudioFire4, AudioFire8, AudioFirePre8 and AudioFire12, these models don't support SYT-Match clock source. Summary, we have no need to relate incoming/outgoing packets. This commit drops reusing SYT sequence of incoming packets for outgoing packets. [1] Using TSB43Cx43A: S/PDIF over 1394 (2003, Texus Instruments Incorporated) http://www.ti.com/analog/docs/litabsmultiplefilelist.tsp?literatureNumber=slla148&docCategoryId=1&familyId=361 Signed-off-by: Takashi Sakamoto <o-takashi@sakamocchi.jp> Signed-off-by: Takashi Iwai <tiwai@suse.de>
320 lines
7.3 KiB
C
320 lines
7.3 KiB
C
/*
|
|
* fireworks_stream.c - a part of driver for Fireworks based devices
|
|
*
|
|
* Copyright (c) 2013-2014 Takashi Sakamoto
|
|
*
|
|
* Licensed under the terms of the GNU General Public License, version 2.
|
|
*/
|
|
#include "./fireworks.h"
|
|
|
|
#define CALLBACK_TIMEOUT 100
|
|
|
|
static int
|
|
init_stream(struct snd_efw *efw, struct amdtp_stream *stream)
|
|
{
|
|
struct cmp_connection *conn;
|
|
enum cmp_direction c_dir;
|
|
enum amdtp_stream_direction s_dir;
|
|
int err;
|
|
|
|
if (stream == &efw->tx_stream) {
|
|
conn = &efw->out_conn;
|
|
c_dir = CMP_OUTPUT;
|
|
s_dir = AMDTP_IN_STREAM;
|
|
} else {
|
|
conn = &efw->in_conn;
|
|
c_dir = CMP_INPUT;
|
|
s_dir = AMDTP_OUT_STREAM;
|
|
}
|
|
|
|
err = cmp_connection_init(conn, efw->unit, c_dir, 0);
|
|
if (err < 0)
|
|
goto end;
|
|
|
|
err = amdtp_am824_init(stream, efw->unit, s_dir, CIP_BLOCKING);
|
|
if (err < 0) {
|
|
amdtp_stream_destroy(stream);
|
|
cmp_connection_destroy(conn);
|
|
}
|
|
end:
|
|
return err;
|
|
}
|
|
|
|
static void
|
|
stop_stream(struct snd_efw *efw, struct amdtp_stream *stream)
|
|
{
|
|
amdtp_stream_pcm_abort(stream);
|
|
amdtp_stream_stop(stream);
|
|
|
|
if (stream == &efw->tx_stream)
|
|
cmp_connection_break(&efw->out_conn);
|
|
else
|
|
cmp_connection_break(&efw->in_conn);
|
|
}
|
|
|
|
static int
|
|
start_stream(struct snd_efw *efw, struct amdtp_stream *stream,
|
|
unsigned int sampling_rate)
|
|
{
|
|
struct cmp_connection *conn;
|
|
unsigned int mode, pcm_channels, midi_ports;
|
|
int err;
|
|
|
|
err = snd_efw_get_multiplier_mode(sampling_rate, &mode);
|
|
if (err < 0)
|
|
goto end;
|
|
if (stream == &efw->tx_stream) {
|
|
conn = &efw->out_conn;
|
|
pcm_channels = efw->pcm_capture_channels[mode];
|
|
midi_ports = efw->midi_out_ports;
|
|
} else {
|
|
conn = &efw->in_conn;
|
|
pcm_channels = efw->pcm_playback_channels[mode];
|
|
midi_ports = efw->midi_in_ports;
|
|
}
|
|
|
|
err = amdtp_am824_set_parameters(stream, sampling_rate,
|
|
pcm_channels, midi_ports, false);
|
|
if (err < 0)
|
|
goto end;
|
|
|
|
/* establish connection via CMP */
|
|
err = cmp_connection_establish(conn,
|
|
amdtp_stream_get_max_payload(stream));
|
|
if (err < 0)
|
|
goto end;
|
|
|
|
/* start amdtp stream */
|
|
err = amdtp_stream_start(stream,
|
|
conn->resources.channel,
|
|
conn->speed);
|
|
if (err < 0) {
|
|
stop_stream(efw, stream);
|
|
goto end;
|
|
}
|
|
|
|
/* wait first callback */
|
|
if (!amdtp_stream_wait_callback(stream, CALLBACK_TIMEOUT)) {
|
|
stop_stream(efw, stream);
|
|
err = -ETIMEDOUT;
|
|
}
|
|
end:
|
|
return err;
|
|
}
|
|
|
|
/*
|
|
* This function should be called before starting the stream or after stopping
|
|
* the streams.
|
|
*/
|
|
static void
|
|
destroy_stream(struct snd_efw *efw, struct amdtp_stream *stream)
|
|
{
|
|
struct cmp_connection *conn;
|
|
|
|
if (stream == &efw->tx_stream)
|
|
conn = &efw->out_conn;
|
|
else
|
|
conn = &efw->in_conn;
|
|
|
|
amdtp_stream_destroy(stream);
|
|
cmp_connection_destroy(&efw->out_conn);
|
|
}
|
|
|
|
static int
|
|
check_connection_used_by_others(struct snd_efw *efw, struct amdtp_stream *s)
|
|
{
|
|
struct cmp_connection *conn;
|
|
bool used;
|
|
int err;
|
|
|
|
if (s == &efw->tx_stream)
|
|
conn = &efw->out_conn;
|
|
else
|
|
conn = &efw->in_conn;
|
|
|
|
err = cmp_connection_check_used(conn, &used);
|
|
if ((err >= 0) && used && !amdtp_stream_running(s)) {
|
|
dev_err(&efw->unit->device,
|
|
"Connection established by others: %cPCR[%d]\n",
|
|
(conn->direction == CMP_OUTPUT) ? 'o' : 'i',
|
|
conn->pcr_index);
|
|
err = -EBUSY;
|
|
}
|
|
|
|
return err;
|
|
}
|
|
|
|
int snd_efw_stream_init_duplex(struct snd_efw *efw)
|
|
{
|
|
int err;
|
|
|
|
err = init_stream(efw, &efw->tx_stream);
|
|
if (err < 0)
|
|
goto end;
|
|
/* Fireworks transmits NODATA packets with TAG0. */
|
|
efw->tx_stream.flags |= CIP_EMPTY_WITH_TAG0;
|
|
/* Fireworks has its own meaning for dbc. */
|
|
efw->tx_stream.flags |= CIP_DBC_IS_END_EVENT;
|
|
/* Fireworks reset dbc at bus reset. */
|
|
efw->tx_stream.flags |= CIP_SKIP_DBC_ZERO_CHECK;
|
|
/*
|
|
* But Recent firmwares starts packets with non-zero dbc.
|
|
* Driver version 5.7.6 installs firmware version 5.7.3.
|
|
*/
|
|
if (efw->is_fireworks3 &&
|
|
(efw->firmware_version == 0x5070000 ||
|
|
efw->firmware_version == 0x5070300 ||
|
|
efw->firmware_version == 0x5080000))
|
|
efw->tx_stream.tx_first_dbc = 0x02;
|
|
/* AudioFire9 always reports wrong dbs. */
|
|
if (efw->is_af9)
|
|
efw->tx_stream.flags |= CIP_WRONG_DBS;
|
|
/* Firmware version 5.5 reports fixed interval for dbc. */
|
|
if (efw->firmware_version == 0x5050000)
|
|
efw->tx_stream.tx_dbc_interval = 8;
|
|
|
|
err = init_stream(efw, &efw->rx_stream);
|
|
if (err < 0) {
|
|
destroy_stream(efw, &efw->tx_stream);
|
|
goto end;
|
|
}
|
|
|
|
/* set IEC61883 compliant mode (actually not fully compliant...) */
|
|
err = snd_efw_command_set_tx_mode(efw, SND_EFW_TRANSPORT_MODE_IEC61883);
|
|
if (err < 0) {
|
|
destroy_stream(efw, &efw->tx_stream);
|
|
destroy_stream(efw, &efw->rx_stream);
|
|
}
|
|
end:
|
|
return err;
|
|
}
|
|
|
|
int snd_efw_stream_start_duplex(struct snd_efw *efw, unsigned int rate)
|
|
{
|
|
unsigned int curr_rate;
|
|
int err = 0;
|
|
|
|
/* Need no substreams */
|
|
if (efw->playback_substreams == 0 && efw->capture_substreams == 0)
|
|
goto end;
|
|
|
|
/*
|
|
* Considering JACK/FFADO streaming:
|
|
* TODO: This can be removed hwdep functionality becomes popular.
|
|
*/
|
|
err = check_connection_used_by_others(efw, &efw->rx_stream);
|
|
if (err < 0)
|
|
goto end;
|
|
|
|
/* packet queueing error */
|
|
if (amdtp_streaming_error(&efw->tx_stream))
|
|
stop_stream(efw, &efw->tx_stream);
|
|
if (amdtp_streaming_error(&efw->rx_stream))
|
|
stop_stream(efw, &efw->rx_stream);
|
|
|
|
/* stop streams if rate is different */
|
|
err = snd_efw_command_get_sampling_rate(efw, &curr_rate);
|
|
if (err < 0)
|
|
goto end;
|
|
if (rate == 0)
|
|
rate = curr_rate;
|
|
if (rate != curr_rate) {
|
|
stop_stream(efw, &efw->tx_stream);
|
|
stop_stream(efw, &efw->rx_stream);
|
|
}
|
|
|
|
/* master should be always running */
|
|
if (!amdtp_stream_running(&efw->rx_stream)) {
|
|
err = snd_efw_command_set_sampling_rate(efw, rate);
|
|
if (err < 0)
|
|
goto end;
|
|
|
|
err = start_stream(efw, &efw->rx_stream, rate);
|
|
if (err < 0) {
|
|
dev_err(&efw->unit->device,
|
|
"fail to start AMDTP master stream:%d\n", err);
|
|
goto end;
|
|
}
|
|
}
|
|
|
|
/* start slave if needed */
|
|
if (efw->capture_substreams > 0 &&
|
|
!amdtp_stream_running(&efw->tx_stream)) {
|
|
err = start_stream(efw, &efw->tx_stream, rate);
|
|
if (err < 0) {
|
|
dev_err(&efw->unit->device,
|
|
"fail to start AMDTP slave stream:%d\n", err);
|
|
stop_stream(efw, &efw->rx_stream);
|
|
}
|
|
}
|
|
end:
|
|
return err;
|
|
}
|
|
|
|
void snd_efw_stream_stop_duplex(struct snd_efw *efw)
|
|
{
|
|
if (efw->capture_substreams == 0) {
|
|
stop_stream(efw, &efw->tx_stream);
|
|
|
|
if (efw->playback_substreams == 0)
|
|
stop_stream(efw, &efw->rx_stream);
|
|
}
|
|
}
|
|
|
|
void snd_efw_stream_update_duplex(struct snd_efw *efw)
|
|
{
|
|
if (cmp_connection_update(&efw->out_conn) < 0 ||
|
|
cmp_connection_update(&efw->in_conn) < 0) {
|
|
stop_stream(efw, &efw->rx_stream);
|
|
stop_stream(efw, &efw->tx_stream);
|
|
} else {
|
|
amdtp_stream_update(&efw->rx_stream);
|
|
amdtp_stream_update(&efw->tx_stream);
|
|
}
|
|
}
|
|
|
|
void snd_efw_stream_destroy_duplex(struct snd_efw *efw)
|
|
{
|
|
destroy_stream(efw, &efw->rx_stream);
|
|
destroy_stream(efw, &efw->tx_stream);
|
|
}
|
|
|
|
void snd_efw_stream_lock_changed(struct snd_efw *efw)
|
|
{
|
|
efw->dev_lock_changed = true;
|
|
wake_up(&efw->hwdep_wait);
|
|
}
|
|
|
|
int snd_efw_stream_lock_try(struct snd_efw *efw)
|
|
{
|
|
int err;
|
|
|
|
spin_lock_irq(&efw->lock);
|
|
|
|
/* user land lock this */
|
|
if (efw->dev_lock_count < 0) {
|
|
err = -EBUSY;
|
|
goto end;
|
|
}
|
|
|
|
/* this is the first time */
|
|
if (efw->dev_lock_count++ == 0)
|
|
snd_efw_stream_lock_changed(efw);
|
|
err = 0;
|
|
end:
|
|
spin_unlock_irq(&efw->lock);
|
|
return err;
|
|
}
|
|
|
|
void snd_efw_stream_lock_release(struct snd_efw *efw)
|
|
{
|
|
spin_lock_irq(&efw->lock);
|
|
|
|
if (WARN_ON(efw->dev_lock_count <= 0))
|
|
goto end;
|
|
if (--efw->dev_lock_count == 0)
|
|
snd_efw_stream_lock_changed(efw);
|
|
end:
|
|
spin_unlock_irq(&efw->lock);
|
|
}
|