mirror of
https://github.com/torvalds/linux
synced 2024-11-05 18:23:50 +00:00
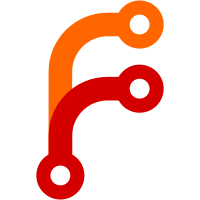
Function was only called in dsos.c with the dso parameter as NULL. Remove the function and specialize for the dso being NULL case removing other unused functions along the way. Signed-off-by: Ian Rogers <irogers@google.com> Cc: Adrian Hunter <adrian.hunter@intel.com> Cc: Ahelenia Ziemiańska <nabijaczleweli@nabijaczleweli.xyz> Cc: Alexander Shishkin <alexander.shishkin@linux.intel.com> Cc: Andi Kleen <ak@linux.intel.com> Cc: Athira Rajeev <atrajeev@linux.vnet.ibm.com> Cc: Ben Gainey <ben.gainey@arm.com> Cc: Changbin Du <changbin.du@huawei.com> Cc: Chengen Du <chengen.du@canonical.com> Cc: Colin Ian King <colin.i.king@gmail.com> Cc: Dima Kogan <dima@secretsauce.net> Cc: Ilkka Koskinen <ilkka@os.amperecomputing.com> Cc: Ingo Molnar <mingo@redhat.com> Cc: James Clark <james.clark@arm.com> Cc: Jiri Olsa <jolsa@kernel.org> Cc: K Prateek Nayak <kprateek.nayak@amd.com> Cc: Kan Liang <kan.liang@linux.intel.com> Cc: Leo Yan <leo.yan@linux.dev> Cc: Li Dong <lidong@vivo.com> Cc: Mark Rutland <mark.rutland@arm.com> Cc: Masami Hiramatsu <mhiramat@kernel.org> Cc: Namhyung Kim <namhyung@kernel.org> Cc: Paran Lee <p4ranlee@gmail.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Song Liu <song@kernel.org> Cc: Sun Haiyong <sunhaiyong@loongson.cn> Cc: Thomas Richter <tmricht@linux.ibm.com> Cc: Tiezhu Yang <yangtiezhu@loongson.cn> Cc: Yanteng Si <siyanteng@loongson.cn> Cc: zhaimingbing <zhaimingbing@cmss.chinamobile.com> Link: https://lore.kernel.org/r/20240504213803.218974-4-irogers@google.com Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
52 lines
1.4 KiB
C
52 lines
1.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __PERF_DSOS
|
|
#define __PERF_DSOS
|
|
|
|
#include <stdbool.h>
|
|
#include <stdio.h>
|
|
#include <linux/list.h>
|
|
#include <linux/rbtree.h>
|
|
#include "rwsem.h"
|
|
|
|
struct dso;
|
|
struct dso_id;
|
|
struct kmod_path;
|
|
struct machine;
|
|
|
|
/*
|
|
* Collection of DSOs as an array for iteration speed, but sorted for O(n)
|
|
* lookup.
|
|
*/
|
|
struct dsos {
|
|
struct rw_semaphore lock;
|
|
struct dso **dsos;
|
|
unsigned int cnt;
|
|
unsigned int allocated;
|
|
bool sorted;
|
|
};
|
|
|
|
void dsos__init(struct dsos *dsos);
|
|
void dsos__exit(struct dsos *dsos);
|
|
|
|
int __dsos__add(struct dsos *dsos, struct dso *dso);
|
|
int dsos__add(struct dsos *dsos, struct dso *dso);
|
|
struct dso *dsos__find(struct dsos *dsos, const char *name, bool cmp_short);
|
|
|
|
struct dso *dsos__findnew_id(struct dsos *dsos, const char *name, struct dso_id *id);
|
|
|
|
bool dsos__read_build_ids(struct dsos *dsos, bool with_hits);
|
|
|
|
size_t dsos__fprintf_buildid(struct dsos *dsos, FILE *fp,
|
|
bool (skip)(struct dso *dso, int parm), int parm);
|
|
size_t dsos__fprintf(struct dsos *dsos, FILE *fp);
|
|
|
|
int dsos__hit_all(struct dsos *dsos);
|
|
|
|
struct dso *dsos__findnew_module_dso(struct dsos *dsos, struct machine *machine,
|
|
struct kmod_path *m, const char *filename);
|
|
|
|
struct dso *dsos__find_kernel_dso(struct dsos *dsos);
|
|
|
|
int dsos__for_each_dso(struct dsos *dsos, int (*cb)(struct dso *dso, void *data), void *data);
|
|
|
|
#endif /* __PERF_DSOS */
|