mirror of
https://github.com/torvalds/linux
synced 2024-09-19 18:46:35 +00:00
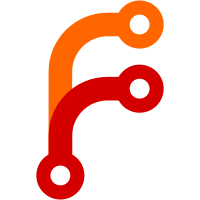
Trying to toggle the resets in a rapid fashion can lead to the changes
not actually arriving at the clock controller block when we expect them
to. This was observed at least on SM8250.
Read back the value after regmap_update_bits to ensure write completion.
Fixes: b36ba30c8a
("clk: qcom: Add reset controller support")
Signed-off-by: Konrad Dybcio <konrad.dybcio@linaro.org>
Link: https://lore.kernel.org/r/20240105-topic-venus_reset-v2-3-c37eba13b5ce@linaro.org
Signed-off-by: Bjorn Andersson <andersson@kernel.org>
60 lines
1.5 KiB
C
60 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* Copyright (c) 2013, The Linux Foundation. All rights reserved.
|
|
*/
|
|
|
|
#include <linux/bitops.h>
|
|
#include <linux/export.h>
|
|
#include <linux/regmap.h>
|
|
#include <linux/reset-controller.h>
|
|
#include <linux/delay.h>
|
|
|
|
#include "reset.h"
|
|
|
|
static int qcom_reset(struct reset_controller_dev *rcdev, unsigned long id)
|
|
{
|
|
struct qcom_reset_controller *rst = to_qcom_reset_controller(rcdev);
|
|
|
|
rcdev->ops->assert(rcdev, id);
|
|
fsleep(rst->reset_map[id].udelay ?: 1); /* use 1 us as default */
|
|
|
|
rcdev->ops->deassert(rcdev, id);
|
|
return 0;
|
|
}
|
|
|
|
static int qcom_reset_set_assert(struct reset_controller_dev *rcdev,
|
|
unsigned long id, bool assert)
|
|
{
|
|
struct qcom_reset_controller *rst;
|
|
const struct qcom_reset_map *map;
|
|
u32 mask;
|
|
|
|
rst = to_qcom_reset_controller(rcdev);
|
|
map = &rst->reset_map[id];
|
|
mask = map->bitmask ? map->bitmask : BIT(map->bit);
|
|
|
|
regmap_update_bits(rst->regmap, map->reg, mask, assert ? mask : 0);
|
|
|
|
/* Read back the register to ensure write completion, ignore the value */
|
|
regmap_read(rst->regmap, map->reg, &mask);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int qcom_reset_assert(struct reset_controller_dev *rcdev, unsigned long id)
|
|
{
|
|
return qcom_reset_set_assert(rcdev, id, true);
|
|
}
|
|
|
|
static int qcom_reset_deassert(struct reset_controller_dev *rcdev, unsigned long id)
|
|
{
|
|
return qcom_reset_set_assert(rcdev, id, false);
|
|
}
|
|
|
|
const struct reset_control_ops qcom_reset_ops = {
|
|
.reset = qcom_reset,
|
|
.assert = qcom_reset_assert,
|
|
.deassert = qcom_reset_deassert,
|
|
};
|
|
EXPORT_SYMBOL_GPL(qcom_reset_ops);
|