mirror of
https://github.com/torvalds/linux
synced 2024-10-04 10:26:40 +00:00
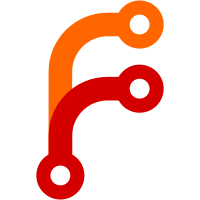
Make kernfs support superblock creation/mount/remount with fs_context. This requires that sysfs, cgroup and intel_rdt, which are built on kernfs, be made to support fs_context also. Notes: (1) A kernfs_fs_context struct is created to wrap fs_context and the kernfs mount parameters are moved in here (or are in fs_context). (2) kernfs_mount{,_ns}() are made into kernfs_get_tree(). The extra namespace tag parameter is passed in the context if desired (3) kernfs_free_fs_context() is provided as a destructor for the kernfs_fs_context struct, but for the moment it does nothing except get called in the right places. (4) sysfs doesn't wrap kernfs_fs_context since it has no parameters to pass, but possibly this should be done anyway in case someone wants to add a parameter in future. (5) A cgroup_fs_context struct is created to wrap kernfs_fs_context and the cgroup v1 and v2 mount parameters are all moved there. (6) cgroup1 parameter parsing error messages are now handled by invalf(), which allows userspace to collect them directly. (7) cgroup1 parameter cleanup is now done in the context destructor rather than in the mount/get_tree and remount functions. Weirdies: (*) cgroup_do_get_tree() calls cset_cgroup_from_root() with locks held, but then uses the resulting pointer after dropping the locks. I'm told this is okay and needs commenting. (*) The cgroup refcount web. This really needs documenting. (*) cgroup2 only has one root? Add a suggestion from Thomas Gleixner in which the RDT enablement code is placed into its own function. [folded a leak fix from Andrey Vagin] Signed-off-by: David Howells <dhowells@redhat.com> cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> cc: Tejun Heo <tj@kernel.org> cc: Li Zefan <lizefan@huawei.com> cc: Johannes Weiner <hannes@cmpxchg.org> cc: cgroups@vger.kernel.org cc: fenghua.yu@intel.com Signed-off-by: Al Viro <viro@zeniv.linux.org.uk>
127 lines
3.4 KiB
C
127 lines
3.4 KiB
C
/*
|
|
* fs/kernfs/kernfs-internal.h - kernfs internal header file
|
|
*
|
|
* Copyright (c) 2001-3 Patrick Mochel
|
|
* Copyright (c) 2007 SUSE Linux Products GmbH
|
|
* Copyright (c) 2007, 2013 Tejun Heo <teheo@suse.de>
|
|
*
|
|
* This file is released under the GPLv2.
|
|
*/
|
|
|
|
#ifndef __KERNFS_INTERNAL_H
|
|
#define __KERNFS_INTERNAL_H
|
|
|
|
#include <linux/lockdep.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/mutex.h>
|
|
#include <linux/xattr.h>
|
|
|
|
#include <linux/kernfs.h>
|
|
#include <linux/fs_context.h>
|
|
|
|
struct kernfs_iattrs {
|
|
struct iattr ia_iattr;
|
|
void *ia_secdata;
|
|
u32 ia_secdata_len;
|
|
|
|
struct simple_xattrs xattrs;
|
|
};
|
|
|
|
/* +1 to avoid triggering overflow warning when negating it */
|
|
#define KN_DEACTIVATED_BIAS (INT_MIN + 1)
|
|
|
|
/* KERNFS_TYPE_MASK and types are defined in include/linux/kernfs.h */
|
|
|
|
/**
|
|
* kernfs_root - find out the kernfs_root a kernfs_node belongs to
|
|
* @kn: kernfs_node of interest
|
|
*
|
|
* Return the kernfs_root @kn belongs to.
|
|
*/
|
|
static inline struct kernfs_root *kernfs_root(struct kernfs_node *kn)
|
|
{
|
|
/* if parent exists, it's always a dir; otherwise, @sd is a dir */
|
|
if (kn->parent)
|
|
kn = kn->parent;
|
|
return kn->dir.root;
|
|
}
|
|
|
|
/*
|
|
* mount.c
|
|
*/
|
|
struct kernfs_super_info {
|
|
struct super_block *sb;
|
|
|
|
/*
|
|
* The root associated with this super_block. Each super_block is
|
|
* identified by the root and ns it's associated with.
|
|
*/
|
|
struct kernfs_root *root;
|
|
|
|
/*
|
|
* Each sb is associated with one namespace tag, currently the
|
|
* network namespace of the task which mounted this kernfs
|
|
* instance. If multiple tags become necessary, make the following
|
|
* an array and compare kernfs_node tag against every entry.
|
|
*/
|
|
const void *ns;
|
|
|
|
/* anchored at kernfs_root->supers, protected by kernfs_mutex */
|
|
struct list_head node;
|
|
};
|
|
#define kernfs_info(SB) ((struct kernfs_super_info *)(SB->s_fs_info))
|
|
|
|
static inline struct kernfs_node *kernfs_dentry_node(struct dentry *dentry)
|
|
{
|
|
if (d_really_is_negative(dentry))
|
|
return NULL;
|
|
return d_inode(dentry)->i_private;
|
|
}
|
|
|
|
extern const struct super_operations kernfs_sops;
|
|
extern struct kmem_cache *kernfs_node_cache;
|
|
|
|
/*
|
|
* inode.c
|
|
*/
|
|
extern const struct xattr_handler *kernfs_xattr_handlers[];
|
|
void kernfs_evict_inode(struct inode *inode);
|
|
int kernfs_iop_permission(struct inode *inode, int mask);
|
|
int kernfs_iop_setattr(struct dentry *dentry, struct iattr *iattr);
|
|
int kernfs_iop_getattr(const struct path *path, struct kstat *stat,
|
|
u32 request_mask, unsigned int query_flags);
|
|
ssize_t kernfs_iop_listxattr(struct dentry *dentry, char *buf, size_t size);
|
|
int __kernfs_setattr(struct kernfs_node *kn, const struct iattr *iattr);
|
|
|
|
/*
|
|
* dir.c
|
|
*/
|
|
extern struct mutex kernfs_mutex;
|
|
extern const struct dentry_operations kernfs_dops;
|
|
extern const struct file_operations kernfs_dir_fops;
|
|
extern const struct inode_operations kernfs_dir_iops;
|
|
|
|
struct kernfs_node *kernfs_get_active(struct kernfs_node *kn);
|
|
void kernfs_put_active(struct kernfs_node *kn);
|
|
int kernfs_add_one(struct kernfs_node *kn);
|
|
struct kernfs_node *kernfs_new_node(struct kernfs_node *parent,
|
|
const char *name, umode_t mode,
|
|
kuid_t uid, kgid_t gid,
|
|
unsigned flags);
|
|
struct kernfs_node *kernfs_find_and_get_node_by_ino(struct kernfs_root *root,
|
|
unsigned int ino);
|
|
|
|
/*
|
|
* file.c
|
|
*/
|
|
extern const struct file_operations kernfs_file_fops;
|
|
|
|
void kernfs_drain_open_files(struct kernfs_node *kn);
|
|
|
|
/*
|
|
* symlink.c
|
|
*/
|
|
extern const struct inode_operations kernfs_symlink_iops;
|
|
|
|
#endif /* __KERNFS_INTERNAL_H */
|