mirror of
https://github.com/torvalds/linux
synced 2024-07-21 10:41:44 +00:00
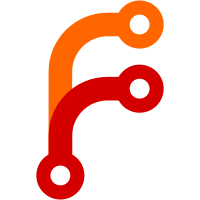
Free page accounting currently happens a bit too high up the call stack, where it has to deal with guard pages, compaction capturing, block stealing and even page isolation. This is subtle and fragile, and makes it difficult to hack on the code. Now that type violations on the freelists have been fixed, push the accounting down to where pages enter and leave the freelist. [hannes@cmpxchg.org: undo unrelated drive-by line wrap] Link: https://lkml.kernel.org/r/20240327185736.GA7597@cmpxchg.org [hannes@cmpxchg.org: remove unused page parameter from account_freepages()] Link: https://lkml.kernel.org/r/20240327185831.GB7597@cmpxchg.org [baolin.wang@linux.alibaba.com: fix free page accounting] Link: https://lkml.kernel.org/r/a2a48baca69f103aa431fd201f8a06e3b95e203d.1712648441.git.baolin.wang@linux.alibaba.com [andriy.shevchenko@linux.intel.com: avoid defining unused function] Link: https://lkml.kernel.org/r/20240423161506.2637177-1-andriy.shevchenko@linux.intel.com Link: https://lkml.kernel.org/r/20240320180429.678181-11-hannes@cmpxchg.org Signed-off-by: Johannes Weiner <hannes@cmpxchg.org> Signed-off-by: Andy Shevchenko <andriy.shevchenko@linux.intel.com> Signed-off-by: Baolin Wang <baolin.wang@linux.alibaba.com> Reviewed-by: Vlastimil Babka <vbabka@suse.cz> Tested-by: Baolin Wang <baolin.wang@linux.alibaba.com> Cc: David Hildenbrand <david@redhat.com> Cc: "Huang, Ying" <ying.huang@intel.com> Cc: Mel Gorman <mgorman@techsingularity.net> Cc: Zi Yan <ziy@nvidia.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
52 lines
1.4 KiB
C
52 lines
1.4 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/mm.h>
|
|
#include <linux/page-isolation.h>
|
|
|
|
unsigned int _debug_guardpage_minorder;
|
|
|
|
bool _debug_pagealloc_enabled_early __read_mostly
|
|
= IS_ENABLED(CONFIG_DEBUG_PAGEALLOC_ENABLE_DEFAULT);
|
|
EXPORT_SYMBOL(_debug_pagealloc_enabled_early);
|
|
DEFINE_STATIC_KEY_FALSE(_debug_pagealloc_enabled);
|
|
EXPORT_SYMBOL(_debug_pagealloc_enabled);
|
|
|
|
DEFINE_STATIC_KEY_FALSE(_debug_guardpage_enabled);
|
|
|
|
static int __init early_debug_pagealloc(char *buf)
|
|
{
|
|
return kstrtobool(buf, &_debug_pagealloc_enabled_early);
|
|
}
|
|
early_param("debug_pagealloc", early_debug_pagealloc);
|
|
|
|
static int __init debug_guardpage_minorder_setup(char *buf)
|
|
{
|
|
unsigned long res;
|
|
|
|
if (kstrtoul(buf, 10, &res) < 0 || res > MAX_PAGE_ORDER / 2) {
|
|
pr_err("Bad debug_guardpage_minorder value\n");
|
|
return 0;
|
|
}
|
|
_debug_guardpage_minorder = res;
|
|
pr_info("Setting debug_guardpage_minorder to %lu\n", res);
|
|
return 0;
|
|
}
|
|
early_param("debug_guardpage_minorder", debug_guardpage_minorder_setup);
|
|
|
|
bool __set_page_guard(struct zone *zone, struct page *page, unsigned int order)
|
|
{
|
|
if (order >= debug_guardpage_minorder())
|
|
return false;
|
|
|
|
__SetPageGuard(page);
|
|
INIT_LIST_HEAD(&page->buddy_list);
|
|
set_page_private(page, order);
|
|
|
|
return true;
|
|
}
|
|
|
|
void __clear_page_guard(struct zone *zone, struct page *page, unsigned int order)
|
|
{
|
|
__ClearPageGuard(page);
|
|
set_page_private(page, 0);
|
|
}
|