mirror of
https://github.com/torvalds/linux
synced 2024-10-03 01:43:05 +00:00
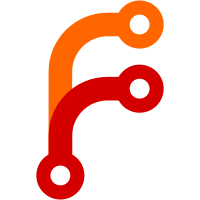
In the cases when gpio_is_valid() is called with unsigned parameter the result is always true in the GPIO library code, hence the check for false won't ever be true. Get rid of such calls. While at it, move GPIO device base to be unsigned to clearly show it won't ever be negative. This requires a new definition for the maximum GPIO number in the system. Signed-off-by: Andy Shevchenko <andriy.shevchenko@linux.intel.com> Reviewed-by: Linus Walleij <linus.walleij@linaro.org> Signed-off-by: Bartosz Golaszewski <bartosz.golaszewski@linaro.org>
75 lines
1.6 KiB
C
75 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/gpio/consumer.h>
|
|
#include <linux/gpio/driver.h>
|
|
|
|
#include <linux/gpio.h>
|
|
|
|
#include "gpiolib.h"
|
|
|
|
/*
|
|
* **DEPRECATED** This function is deprecated and must not be used in new code.
|
|
*/
|
|
void gpio_free(unsigned gpio)
|
|
{
|
|
gpiod_free(gpio_to_desc(gpio));
|
|
}
|
|
EXPORT_SYMBOL_GPL(gpio_free);
|
|
|
|
/**
|
|
* gpio_request_one - request a single GPIO with initial configuration
|
|
* @gpio: the GPIO number
|
|
* @flags: GPIO configuration as specified by GPIOF_*
|
|
* @label: a literal description string of this GPIO
|
|
*
|
|
* **DEPRECATED** This function is deprecated and must not be used in new code.
|
|
*/
|
|
int gpio_request_one(unsigned gpio, unsigned long flags, const char *label)
|
|
{
|
|
struct gpio_desc *desc;
|
|
int err;
|
|
|
|
/* Compatibility: assume unavailable "valid" GPIOs will appear later */
|
|
desc = gpio_to_desc(gpio);
|
|
if (!desc)
|
|
return -EPROBE_DEFER;
|
|
|
|
err = gpiod_request(desc, label);
|
|
if (err)
|
|
return err;
|
|
|
|
if (flags & GPIOF_ACTIVE_LOW)
|
|
set_bit(FLAG_ACTIVE_LOW, &desc->flags);
|
|
|
|
if (flags & GPIOF_DIR_IN)
|
|
err = gpiod_direction_input(desc);
|
|
else
|
|
err = gpiod_direction_output_raw(desc,
|
|
(flags & GPIOF_INIT_HIGH) ? 1 : 0);
|
|
|
|
if (err)
|
|
goto free_gpio;
|
|
|
|
return 0;
|
|
|
|
free_gpio:
|
|
gpiod_free(desc);
|
|
return err;
|
|
}
|
|
EXPORT_SYMBOL_GPL(gpio_request_one);
|
|
|
|
/*
|
|
* **DEPRECATED** This function is deprecated and must not be used in new code.
|
|
*/
|
|
int gpio_request(unsigned gpio, const char *label)
|
|
{
|
|
struct gpio_desc *desc;
|
|
|
|
/* Compatibility: assume unavailable "valid" GPIOs will appear later */
|
|
desc = gpio_to_desc(gpio);
|
|
if (!desc)
|
|
return -EPROBE_DEFER;
|
|
|
|
return gpiod_request(desc, label);
|
|
}
|
|
EXPORT_SYMBOL_GPL(gpio_request);
|